Understanding Microtask Queue and Callback Queue in JavaScript

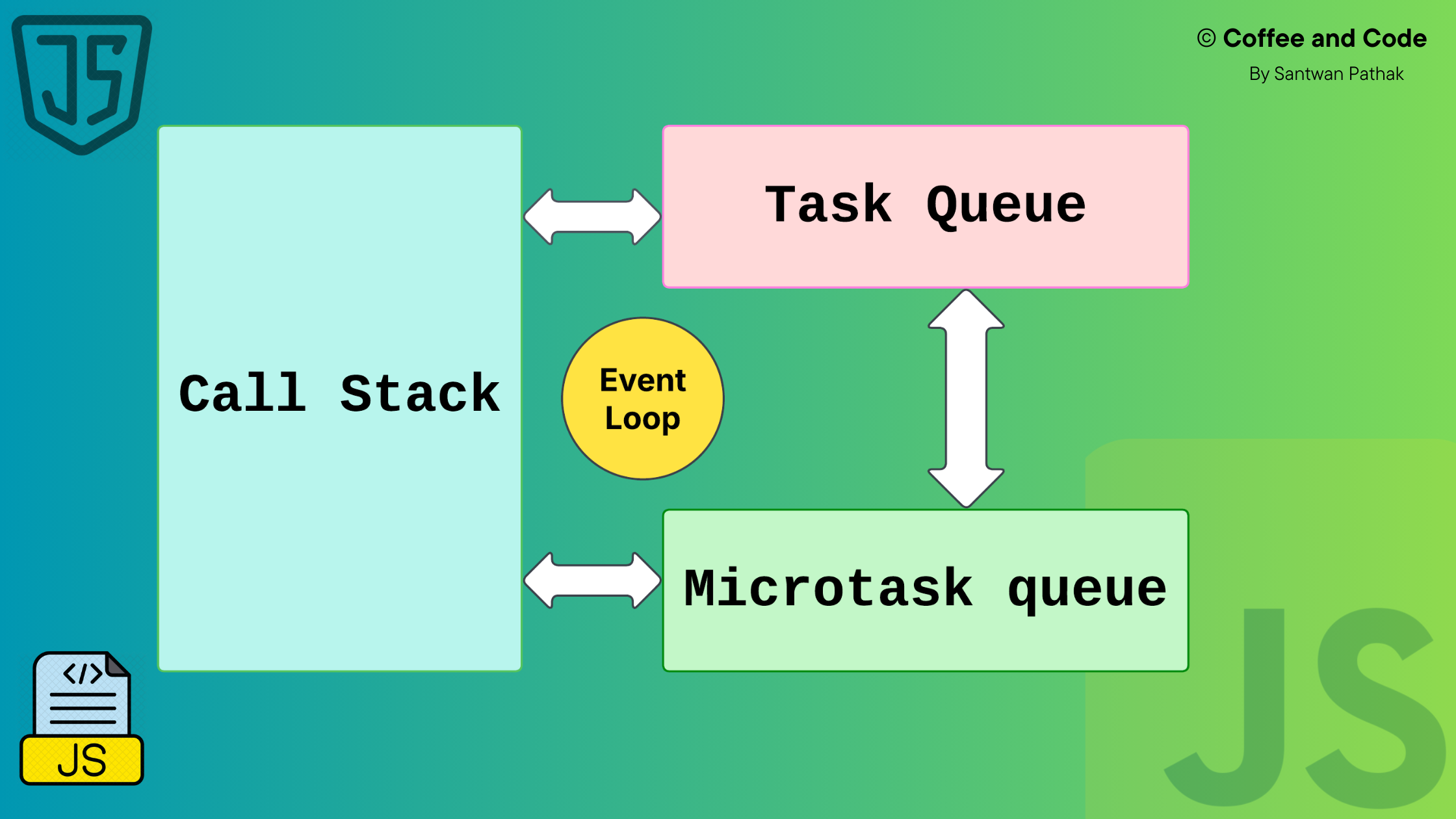
☕ Introduction
JavaScript is a single-threaded language, meaning it executes one operation at a time. However, it manages asynchronous tasks efficiently using the Event Loop, a mechanism that allows JavaScript to handle non-blocking operations seamlessly. The Event Loop interacts with two primary types of queues that determine the execution order of asynchronous tasks:
Microtask Queue (Higher Priority) – This queue is responsible for handling microtasks such as resolved Promises and process.nextTick (in Node.js). Microtasks are executed immediately after the current synchronous execution completes, before moving on to the Callback Queue.
Callback Queue (Also known as Task Queue) – This queue contains callback functions from setTimeout, setInterval, and other asynchronous APIs. These tasks are executed only after the Microtask Queue is empty and the current execution context has cleared.
Understanding the difference between these queues is essential for writing efficient, non-blocking JavaScript applications.
In this blog, we will explore how JavaScript handles asynchronous execution through these queues, using Promises and setTimeout() as examples.
Execution Order: Microtask Queue vs. Callback Queue
Code Example 1:
console.log("Hi");
setTimeout(() => console.log("Hello after 2s"), 2000); // this means this will execute at a delay of 2 second
Promise.resolve().then(() => console.log("Promise Resolve Hogya"));
console.log("BYE");
Execution Breakdown:
console.log("Hi")
executes first (synchronous task), immediately printing "Hi" to the console.setTimeout()
schedules a callback to execute after 2 seconds but does not run it immediately. It places the task in the Callback Queue.Promise.resolve().then()
schedules a microtask and places it in the Microtask Queue.console.log("BYE")
executes as another synchronous task, printing "BYE" to the console.The Microtask Queue executes next, processing "Promise Resolve Hogya" before moving on to the Callback Queue.
Finally, after 2 seconds, the
setTimeout
callback runs and prints "Hello after 2s".
Use this website to visualize whatever is written here and the output
Output:
Hi
BYE
Promise Resolve Hogya
Hello after 2s
Code Example 2:
console.log("Hi");
setTimeout(() => console.log("Hello after 2s"), 0);
Promise.resolve().then(() => console.log("Promise Resolve Hogya"));
setTimeout(() => console.log("Hello after 2s"), 0);
console.log("BYE");
Execution Breakdown:
console.log("Hi")
executes first, immediately printing "Hi" to the console.setTimeout()
schedules a callback with 0ms delay, placing it in the Callback Queue.Promise.resolve().then()
schedules a microtask and places it in the Microtask Queue.Another
setTimeout()
with 0ms delay is scheduled, adding another callback to the Callback Queue.console.log("BYE")
executes as a synchronous task, printing "BYE" to the console.The Microtask Queue executes, processing "Promise Resolve Hogya" before any setTimeout callbacks.
Finally, both
setTimeout()
callbacks are executed in order.
Output:
Hi
BYE
Promise Resolve Hogya
Hello after 2s
Hello after 2s
Microtask Queue Has Higher Priority
In JavaScript, the Microtask Queue executes before the Callback Queue, even if a microtask is scheduled within another microtask. This can lead to Starvation, where tasks in the Callback Queue get delayed indefinitely.
Code Example 3: Starvation
setTimeout(() => console.log("Hello after 2s"), 0);
Promise.resolve().then(() => {
console.log("Promise Resolve Hogya");
Promise.resolve().then(() => {
console.log("Promise Resolve Hogya");
Promise.resolve().then(() => {
console.log("Promise Resolve Hogya");
});
});
});
setTimeout(() => console.log("Hello after 2s"), 0);
console.log("BYE");
Execution Breakdown:
setTimeout()
schedules two callbacks in the Callback Queue.A Promise is resolved, adding multiple microtasks to the Microtask Queue.
console.log("BYE")
executes.The Microtask Queue executes first, processing all nested Promises before moving to the Callback Queue.
Finally,
setTimeout
callbacks execute.
Output:
BYE
Promise Resolve Hogya
Promise Resolve Hogya
Promise Resolve Hogya
Hello after 2s
Hello after 2s
Why is Starvation a Problem?
Since the Microtask Queue has higher priority, a continuously growing queue of microtasks can prevent the execution of tasks in the Callback Queue (setTimeout, setInterval, etc.). This results in delays and unresponsive behavior in applications.
Summary
JavaScript uses the Microtask Queue (Promises, MutationObservers) and Callback Queue (setTimeout, setInterval, etc.) to handle asynchronous tasks.
Microtask Queue has higher priority than the Callback Queue, meaning it executes first.
Starvation occurs when Microtasks keep executing indefinitely, delaying Callback Queue tasks.
Understanding these concepts is crucial for writing efficient and predictable asynchronous JavaScript code.
Did this blog help you? Share your thoughts in the comments! And Please Do Like 😭❤️
Subscribe to my newsletter
Read articles from Santwan Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santwan Pathak
Santwan Pathak
"A beginner in tech with big aspirations. Passionate about web development, AI, and creating impactful solutions. Always learning, always growing."