Redux Toolkit with React: Building a Todo App.

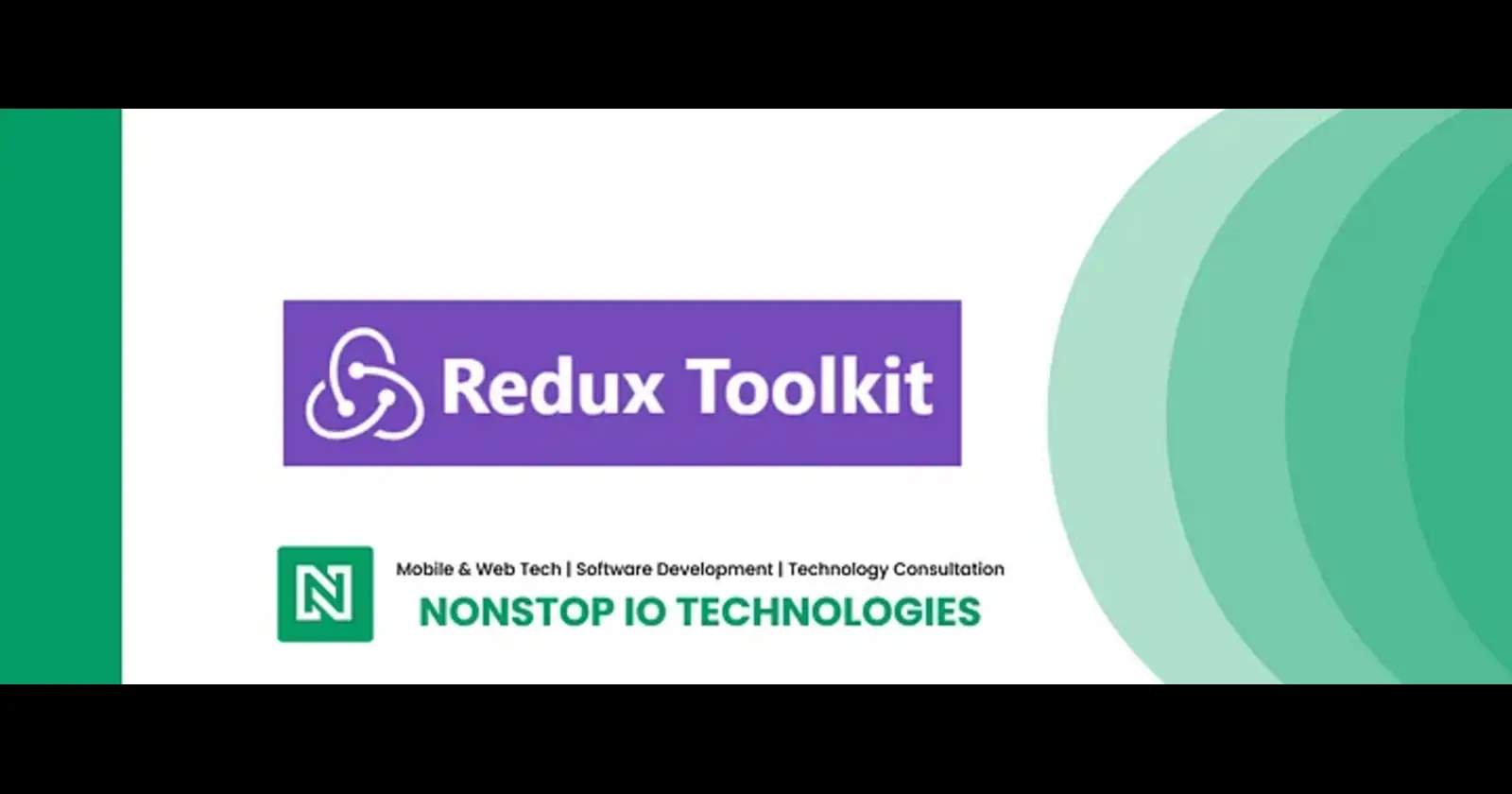
The Redux Toolkit package is intended to be the standard way to write Redux logic. It was initially created to help address three common concerns about Redux:
Configuring a Redux store is too complicated.
I have to add a lot of packages to get Redux to do anything useful.
Redux requires too much boilerplate code.
Building a Todo App.
Step 1: Create a redux store
we need to import the necessary dependencies. In your project, you may have a todosSlice.js
file where you define your Redux slice (reducers and actions). Here's how you import configureStore
and your todoReducer.
ConfigureStore is provided by Redux Toolkit and is used to configure and create a Redux store.
Step 2: Defining a Redux Slice for Your ToDo App
we need to import the necessary dependencies, including createSlice
and nanoid
. createSlice
simplifies the process of creating reducers, actions, and initial state. nanoid
is used to generate unique IDs for our ToDo items. Next, we define an initial state for our Redux slice and then create the Redux slice utilizing the createSlice
function.
Step 3: Create the AddTodo Component
We build the AddTodo
React component. It manages input, dispatches the addTodo
action from our Redux slice, and clears the input field when adding new ToDo items to our app.
Step 4: Create the Todo Component
import { useSelector, useDispatch } from 'react-redux';
import { removeTodo } from '../features/todos/todosSlice';
function Todos() {
const todos = useSelector((state) => state.todos);
const dispatch = useDispatch();
return (
<>
<div>Todos</div>
<ul className='list-none'>
{todos.map((todo) => (
<li
className='mt-4 flex justify-between items-center bg-zinc-800 px-4 py-2 rounded'
key={todo.id}>
<div className='text-white'>{todo.text}</div>
<button
onClick={() => dispatch(removeTodo(todo.id))}
className='text-white bg-red-500 border-0 py-1 px-4 focus:outline-none hover:bg-red-600 rounded text-md'>
<svg
xmlns='http://www.w3.org/2000/svg'
fill='none'
viewBox='0 0 24 24'
strokeWidth={1.5}
stroke='currentColor'
className='w-6 h-6'>
<path
strokeLinecap='round'
strokeLinejoin='round'
d='M14.74 9l-.346 9m-4.788 0L9.26 9m9.968-3.21c.342.052.682.107 1.022.166m-1.022-.165L18.16 19.673a2.25 2.25 0 01-2.244 2.077H8.084a2.25 2.25 0 01-2.244-2.077L4.772 5.79m14.456 0a48.108 48.108 0 00-3.478-.397m-12 .562c.34-.059.68-.114 1.022-.165m0 0a48.11 48.11 0 013.478-.397m7.5 0v-.916c0-1.18-.91-2.164-2.09-2.201a51.964 51.964 0 00-3.32 0c-1.18.037-2.09 1.022-2.09 2.201v.916m7.5 0a48.667 48.667 0 00-7.5 0'
/>
</svg>
</button>
</li>
))}
</ul>
</>
);
}
export default Todos;
The todo component dynamically renders a list of ToDo items and includes the ‘AddTodo’ component to enable users to add new tasks. Users can also remove tasks with a click using the removeTodo
.
Reference link: https://redux-toolkit.js.org/
Github link: https://github.com/navneetnicky/React_Redux/tree/main/reduxToolkit
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.