Discover React 19 Hooks for Easier Async State and Data Management

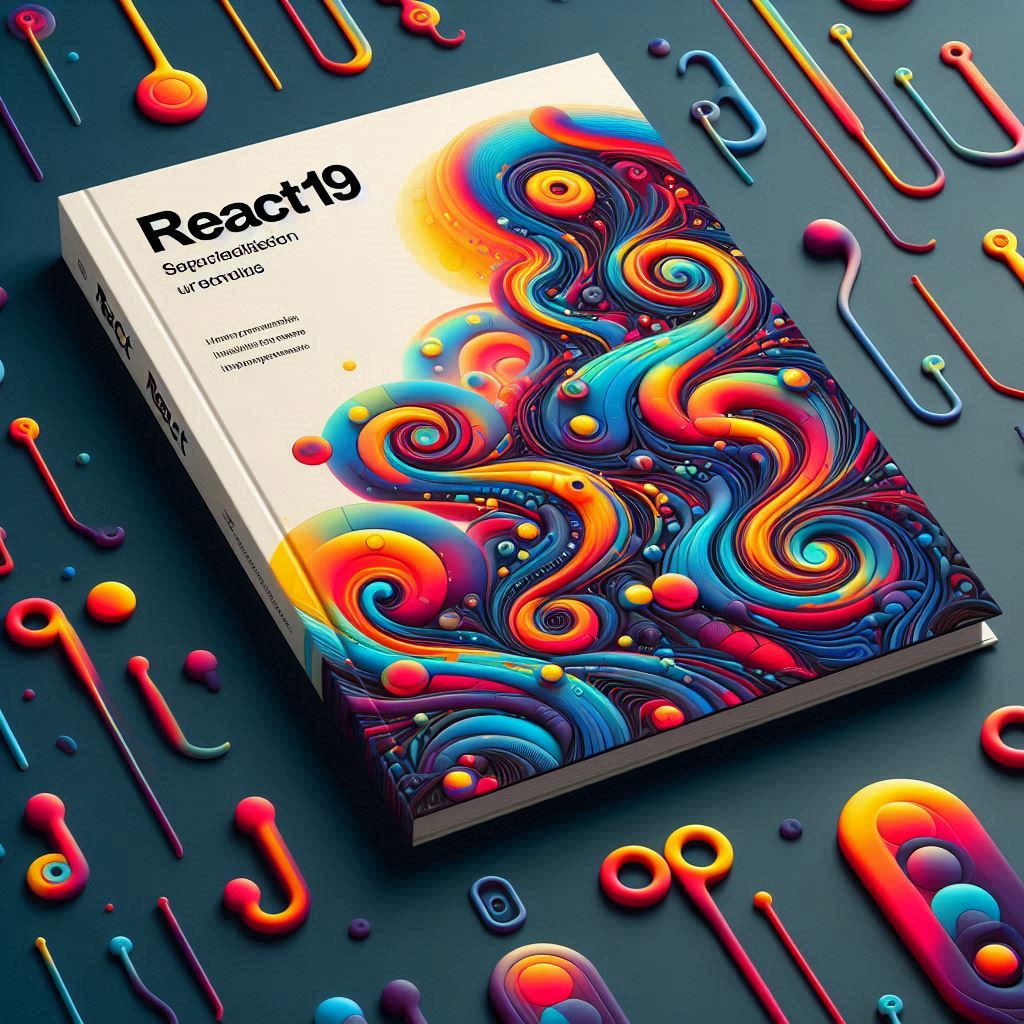
React 19 is here, and it’s not just about performance improvements and server-side rendering. With a host of innovative hooks, React 19 simplifies asynchronous interactions, state management, and even data fetching. In this article, we’ll explore some of the most exciting hooks introduced in React 19 and see how they can transform the way you build modern React applications.
Why React 19’s Hooks Matter
React has always been about writing your UI as a function of state. With the new hooks in React 19, handling asynchronous operations and optimistic UI updates has never been easier. These hooks not only reduce boilerplate but also lead to cleaner, more maintainable code. Whether you’re updating a form, handling a data mutation, or fetching asynchronous data, the new hooks empower you to write more intuitive code.
1. useActionState(): Simplifying Async Mutations
Traditionally, handling async mutations—like form submissions—required manually managing loading states, error handling, and state updates. With the new useActionState() hook, you can encapsulate these concerns in a single, composable API.
What It Does:
Manages Pending State: Automatically toggles a pending state during an async action.
Returns Results: Provides access to the final result of the action (error or success data).
Example Usage:
import { useActionState } from 'react';
function UpdateName() {
const [error, submitAction, isPending] = useActionState(
async (prevState, formData) => {
const newName = formData.get("name");
// Imagine updateName is a function that sends data to your server.
const err = await updateName(newName);
return err; // Return error if any, or null on success.
},
null
);
const handleSubmit = (e) => {
e.preventDefault();
submitAction(new FormData(e.target));
};
return (
<form onSubmit={handleSubmit}>
<input type="text" name="name" placeholder="Enter your name" />
<button type="submit" disabled={isPending}>
{isPending ? "Updating..." : "Update Name"}
</button>
{error && <p style={{ color: "red" }}>{error}</p>}
</form>
);
}
This hook streamlines your async data mutations, reducing the need for verbose state management.
2. useFormStatus(): Access Form Status Without Prop Drilling
In complex forms or design systems, you often need to share form state (like whether the form is pending) across nested components. useFormStatus() comes to the rescue by letting child components access the parent form’s status directly.
Example Usage:
import { useFormStatus } from 'react-dom';
function SubmitButton() {
const { pending } = useFormStatus();
return (
<button type="submit" disabled={pending}>
{pending ? "Processing..." : "Submit"}
</button>
);
}
This hook eliminates the need to pass form state through multiple layers, keeping your component hierarchy clean and simple.
3. useOptimistic(): Enhancing User Experience with Immediate Feedback
Optimistic UI updates are essential for a snappy user experience. useOptimistic() allows you to render an updated UI state immediately while the actual asynchronous operation is underway—and automatically reverts the change if something goes wrong.
Example Usage:
function UpdateProfile({ currentName, onNameChange }) {
const [optimisticName, setOptimisticName] = useOptimistic(currentName);
const handleSubmit = async (e) => {
e.preventDefault();
const newName = new FormData(e.target).get("name");
setOptimisticName(newName); // Optimistically update UI
const error = await updateProfileName(newName);
if (error) {
// Handle rollback or show error message as needed
} else {
onNameChange(newName);
}
};
return (
<form onSubmit={handleSubmit}>
<p>Your name: {optimisticName}</p>
<input type="text" name="name" defaultValue={currentName} />
<button type="submit">Update Name</button>
</form>
);
}
With useOptimistic, users get instant feedback on their actions, making your app feel faster and more responsive.
4. The New “use()” API: Cleaner Asynchronous Data Fetching
React 19 introduces an experimental API called use() that lets you read asynchronous resources like Promises directly within your render function. This means you can simplify your code by eliminating extra state variables or lifecycle hooks for handling data fetching.
Example Usage:
import { use, Suspense } from 'react';
function Comments({ commentsPromise }) {
// Suspends rendering until the promise resolves.
const comments = use(commentsPromise);
return (
<div>
{comments.map((comment) => (
<p key={comment.id}>{comment.text}</p>
))}
</div>
);
}
function Page({ commentsPromise }) {
return (
<Suspense fallback={<div>Loading comments...</div>}>
<Comments commentsPromise={commentsPromise} />
</Suspense>
);
}
The use() API allows you to write more declarative and concise code when working with asynchronous data.
Wrapping Up
React 19 brings a suite of innovative hooks that significantly simplify common asynchronous patterns in React development. Whether it’s managing state transitions with useActionState(), reducing prop drilling with useFormStatus(), delivering instant feedback with useOptimistic(), or streamlining data fetching with the new use() API, these hooks pave the way for cleaner, more efficient code.
By adopting these new hooks, you can reduce boilerplate, enhance user experience, and make your applications more resilient to asynchronous challenges. I encourage you to experiment with these hooks in your own projects and share your experiences with the community.
References:
React 19: New Features and Updates - GeeksforGeeks
Last Updated: January 24, 2025
URL: https://www.geeksforgeeks.org/react-19-new-features-and-updates/React v19 Blog Post by The React Team
Published: December 05, 2024
URL: https://react.dev/blog/2024/12/05/react-19
Subscribe to my newsletter
Read articles from Om Shree directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Om Shree
Om Shree
I specialize in HTML5, CSS3, and JavaScript (ES6+), leveraging React.js ⚛️ and Tailwind CSS 🎨 to build scalable, high-performance web applications. With a keen eye for intuitive design, accessibility, and SEO optimization . 💡 Passionate about integrating emerging technologies like AI 🤖 and Three.js 🎥 to push the boundaries of interactivity. I also mentor aspiring developers and thrive in fast-paced, collaborative environments. 📚 EdTech enthusiast—eager to drive transformative digital solutions that elevate learning experiences. Let's connect! 🤝