Building Groove-Genie: A Journey of Learning and Growth

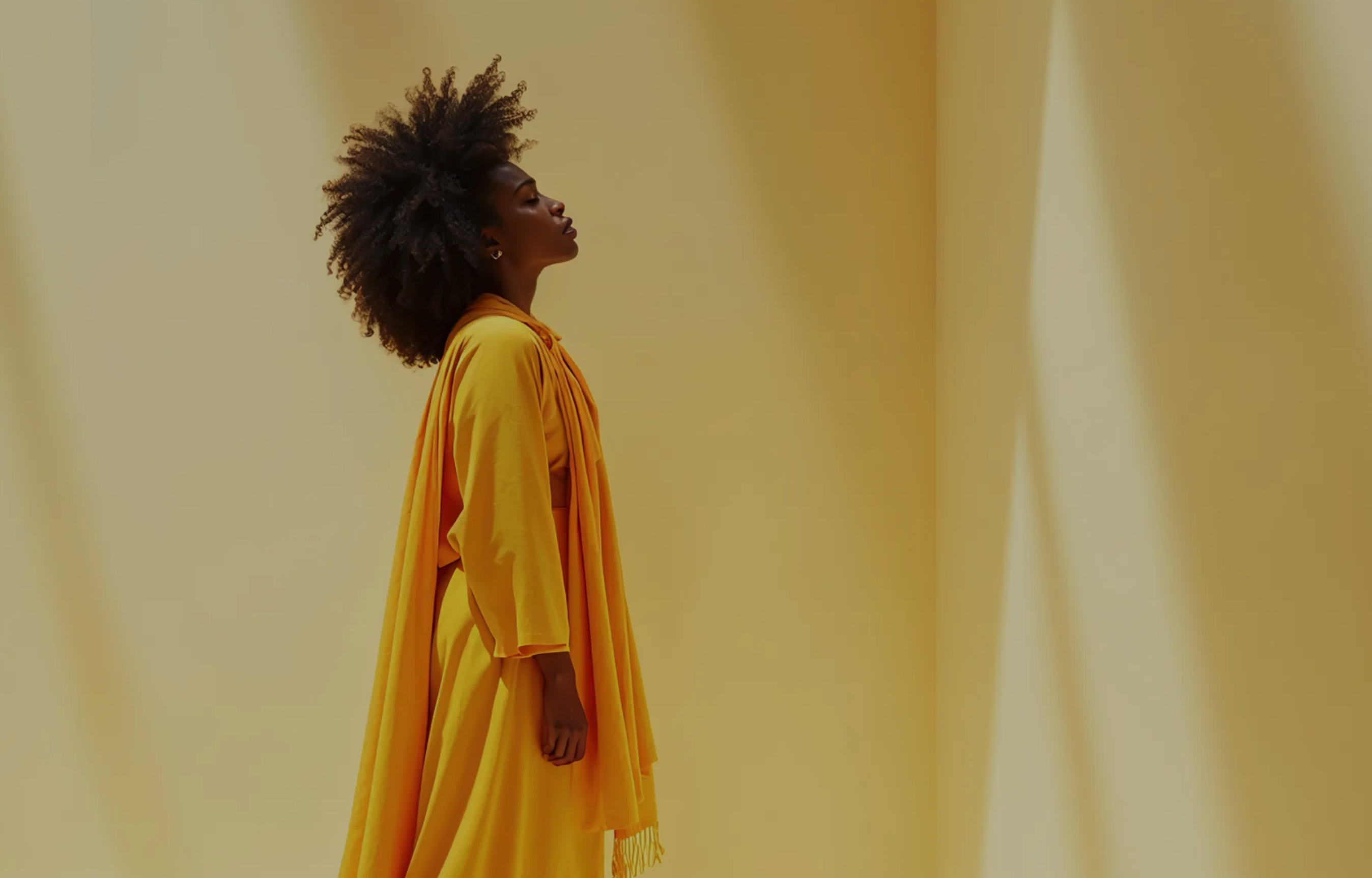
Introduction
Hey there! I'm excited to share my experience of building Groove-Genie, a web application that lets you explore, like, and get recommendations for songs based on your preferences. This project was a labor of love, combining my passion for music and technology. Along the way, I faced numerous challenges, learned valuable lessons, and celebrated some pretty awesome achievements. Let's dive into the journey of creating Groove-Genie!
The Idea Behind Groove-Genie
The inspiration for Groove-Genie came from my love for discovering new music. I wanted to create a unique experience where users could find new songs based on what they already like. The main features I aimed to include were:
Search Functionality: To find songs, artists, or albums easily.
Song Details: To provide detailed information about each song.
Like and Dislike Songs: To let users express their preferences.
Liked Songs List: To keep track of all liked songs.
Recommendations: To suggest new songs based on liked ones.
Planning and Research
Planning was the first crucial step. I outlined the core features and sketched out wireframes for the user interface. Researching existing music recommendation systems helped me understand the algorithms involved. I decided to use Flask for the backend, HTML, CSS, and JavaScript for the frontend, and pandas and scikit-learn for data handling and recommendations.
Challenges and Learnings
- Data Handling with Pandas:
Challenge: Handling large CSV files and ensuring data integrity was tricky. I dealt with missing values, duplicates, and incorrect data types.
Learning: Data cleaning and preprocessing are crucial. Using pandas, I managed to clean and manipulate the data efficiently.
Here's a snippet of the code used to clean the data:
import pandas as pd
# Load the data
df = pd.read_csv('genres_v2.csv', low_memory=False)
# Remove duplicates and handle missing values
df = df.drop_duplicates(subset="song_name")
df = df.dropna(axis=0)
# Drop non-required columns
df = df[['duration_ms', 'genre', 'song_name']]
2. Implementing Cosine Similarity for Recommendations:
Challenge: Implementing the recommendation algorithm using cosine similarity was a steep learning curve. Understanding the math behind it and coding it was challenging.
Learning: I learned the fundamentals of recommendation algorithms and feature extraction. With scikit-learn’s CountVectorizer and cosine_similarity, I implemented a functional recommendation system.
Here’s how the recommendation system was implemented:
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.metrics.pairwise import cosine_similarity
# Combine all columns into a new 'data' column
df["data"] = df.apply(lambda value: " ".join(value.astype("str")), axis=1)
# Initialize CountVectorizer and calculate cosine similarity
vectorizer = CountVectorizer()
vectorized = vectorizer.fit_transform(df['data'])
similarities = cosine_similarity(vectorized)
# Create a DataFrame with similarity values
similarity_df = pd.DataFrame(similarities, columns=df['song_name'], index=df['song_name']).reset_index()
3. Responsive Design:
Challenge: Making the website look good on all screen sizes was a design challenge. The initial design didn’t adapt well to smaller screens.
Learning: Responsive design techniques like media queries and flexible grids are lifesavers. They helped ensure Groove-Genie looks great on any device.
4. User Experience and Interface Design:
Challenge: Balancing aesthetics with functionality required several iterations and user feedback.
Learning: UI/UX design principles are vital. Incorporating user feedback and improving the layout made the app more user-friendly.
Accomplishments
Core Features Implementation:
- I successfully implemented the core features: search functionality, song details, like/dislike, liked songs list, and recommendations.
Machine Learning Integration:
- Implementing the recommendation system using cosine similarity was a significant achievement, integrating machine learning concepts into a real-world application.
Responsive and User-Friendly Design:
- The responsive design ensures a seamless experience across all devices. The user-friendly interface makes it easy to navigate and interact with the app.
Comprehensive Documentation:
- Documenting the entire project, including setup instructions and usage guidelines, ensures it’s easy to understand and share with others.
Reflections and Future Directions
Reflecting on this project, I realize how planning, research, and iterative development were essential. Each challenge was an opportunity to learn and grow, and each accomplishment reinforced my passion for web development and music technology. Groove-Genie is not just a portfolio project; it’s a testament to my dedication and ability to turn an idea into a functional application.
Looking ahead, I plan to enhance Groove-Genie by adding features like user authentication, playlist creation, and integration with music streaming APIs. These improvements will make the app even more robust and user-centric.
Conclusion
Building Groove-Genie has been a transformative journey filled with trials, learnings, and achievements. Capturing these details now ensures that I can expertly present my project to employers and confidently continue my specialization in Year 2. Groove-Genie reflects my skills, creativity, and passion for creating impactful web applications.
If you’re interested in exploring Groove-Genie or have any questions, feel free to reach out to me!
Contact Information:
Email: wanyamak884@gmail.com
GitHub: sameduTM
Thank you for joining me on this journey!
Subscribe to my newsletter
Read articles from Ken Wanyama Wekesa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
