Understanding Next.js Rendering: A Complete Beginner’s Guide 🚀

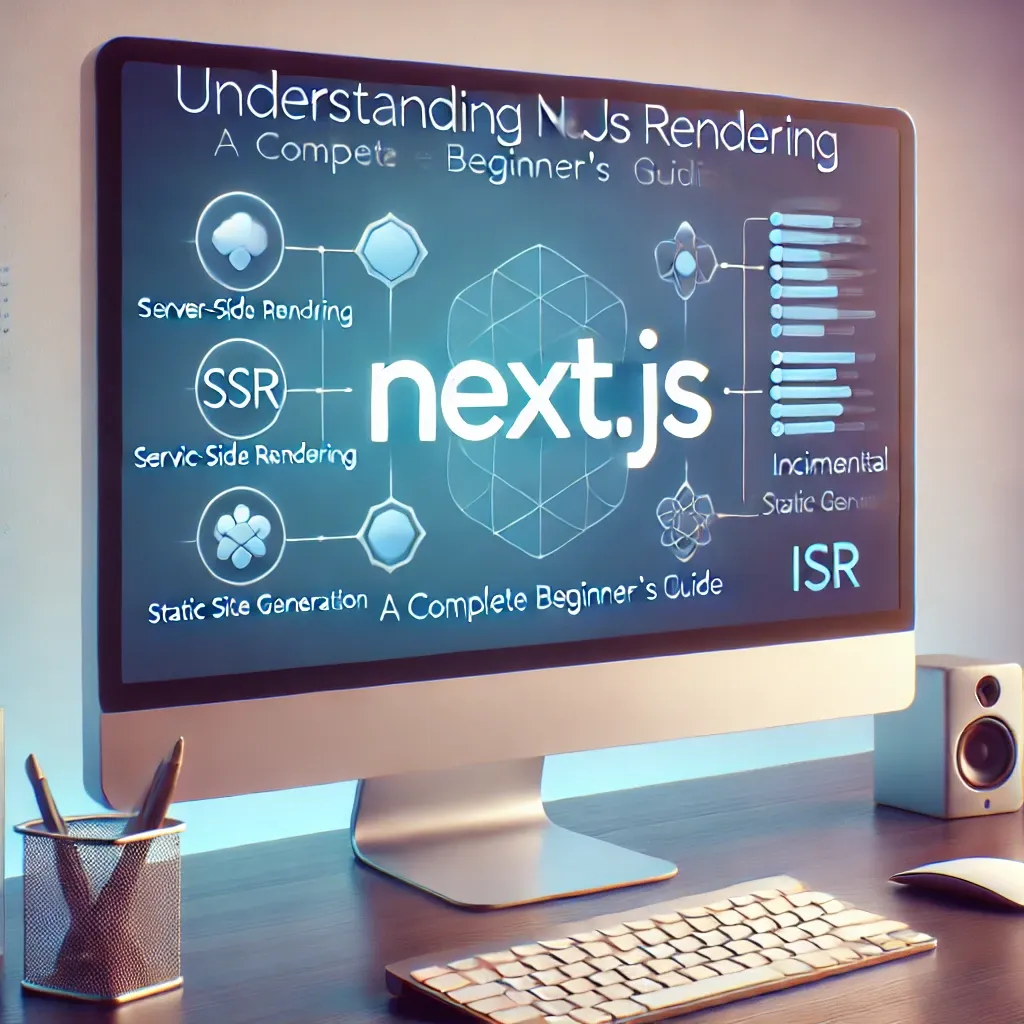
Hey there! Are you just starting with Next.js? Let’s break down the different ways Next.js can serve your web pages in simple terms. Don’t worry if you’re new to this — we’ll take it step by step!
What is Rendering Anyway? 🤔
Before we dive in, let’s understand what rendering means in simple terms:
Rendering is how your website turns your code into actual web pages that users can see.
It’s like baking a cake — you can either bake it when someone orders (Server-Side Rendering) or have it ready in advance (Static Site Generation).
Server-Side Rendering (SSR): Fresh Content Every Time 🔄
What is SSR?
// pages/news.js
export async function getServerSideProps() {
// This code runs on the server every time someone visits the page
const response = await fetch('https://api.example.com/latest-news');
const news = await response.json();
return {
props: {
news, // This data will be passed to your page
fetchTime: new Date().toLocaleString() // Current time
}
};
}
// Your page component
function NewsPage({ news, fetchTime }) {
return (
<div>
<h1>Latest News</h1>
<p>Last updated: {fetchTime}</p>
{news.map(article => (
<div key={article.id}>
<h2>{article.title}</h2>
<p>{article.summary}</p>
</div>
))}
</div>
);
}
export default NewsPage;
When Should You Use SSR?
Think of SSR as perfect for :
Dashboard pages (need latest data)
User-specific pages (like profiles)
Social media feeds (always changing)
Static Site Generation (SSG): Build Once, Use Many Times 📚
What is SSG?
SSG is like preparing meals in advance. Your pages are created when you build your website, not when users visit. This makes them super fast!
// pages/blog/[post].js export async function getStaticPaths() { // This tells Next.js which pages to create at build time const posts = [ { id: 1, title: 'First Post' }, { id: 2, title: 'Second Post' } ]; return { paths: posts.map(post => ({ params: { post: post.id.toString() } })), fallback: false // Show 404 for unknown posts }; } export async function getStaticProps({ params }) { // This runs at build time const post = await getBlogPost(params.post); // Your function to get post data return { props: { post } }; } function BlogPost({ post }) { return ( <article> <h1>{post.title}</h1> <div>{post.content}</div> </article> ); } export default BlogPost;
When to Use SSG?
Perfect for:
Blog posts (content doesn’t change often)
Documentation pages
Product pages in e-commerce
The Best of Both Worlds: Incremental Static Regeneration (ISR) 🌟
Sometimes you want static pages that update occasionally. That’s where ISR comes in! It’s like having a robot chef who remakes the dish every few hours.
// pages/products/[id].js
export async function getStaticProps({ params }) {
const product = await getProduct(params.id);
return {
props: {
product
},
revalidate: 3600 // Update this page every hour (3600 seconds)
};
}
Simple Example Project: Let’s Build! 🛠️
Here’s a tiny project combining both approaches:
// pages/index.js - Static Homepage
export async function getStaticProps() {
return {
props: {
welcomeMessage: "Welcome to our site!",
buildTime: new Date().toISOString()
}
};
}
// pages/profile/[username].js - Dynamic User Profile
export async function getServerSideProps({ params }) {
const { username } = params;
const userData = await fetch(`/api/users/${username}`);
const user = await userData.json();
return {
props: {
user,
visitTime: new Date().toISOString()
}
};
}
Making the Right Choice 🎯
Here’s a simple way to decide:
Does your page need real-time data? → Use SSR
Is your content the same for everyone and changes rarely? → Use SSG
Need occasional updates? → Use ISR
Common Beginner Mistakes to Avoid
Don’t fetch data inside components when you can use getStaticProps or getServerSideProps
function BadExample() { const [data, setData] = useState(null); useEffect(() => { fetch('/api/data').then(res => res.json()).then(setData); }, []); } // âś… Do this instead export async function getStaticProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data } }; }
Remember: getServerSideProps runs on every request, so use it only when necessary.
Wrapping Up 🎉
Now you know:
SSR creates pages on demand (perfect for real-time data)
SSG creates pages at build time (super fast!)
ISR lets you update static pages automatically
Start with SSG for most pages, and use SSR only when you need real-time data. It’s that simple!
Keep coding and experimenting! The best way to learn is by building something yourself. 🚀
Subscribe to my newsletter
Read articles from Supriya Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
