Introduction to GraphQL: Easy Guide You Need

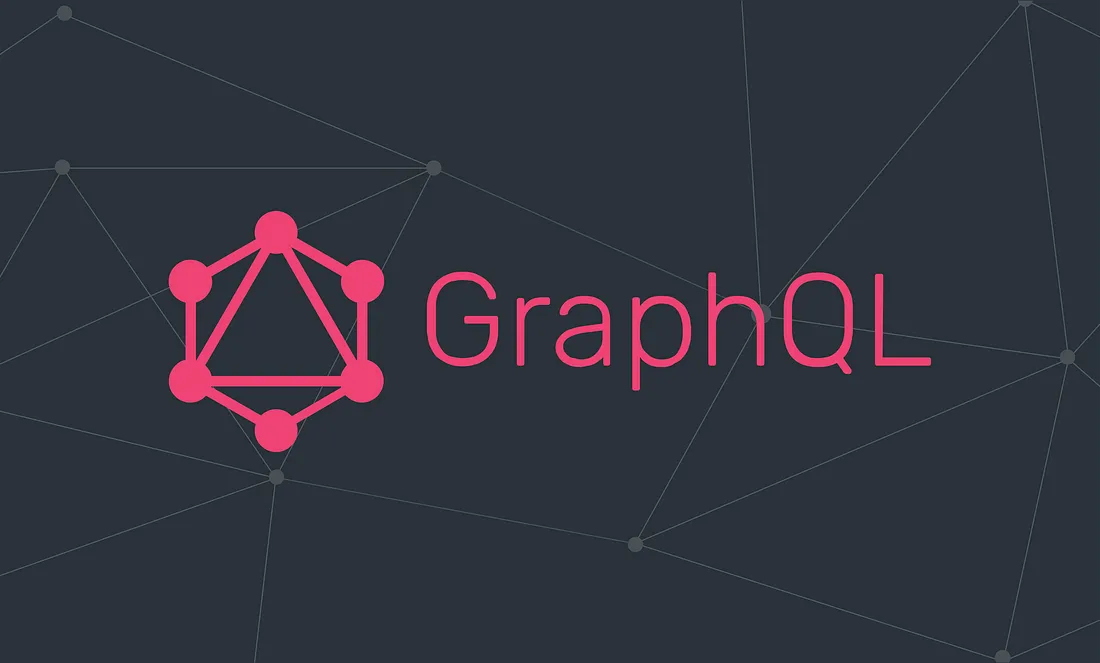
What is GraphQL?
GraphQL is a query language for APIs and a runtime for executing those queries. It allows you to ask for exactly what you need and nothing more. Confused? Let me simplify: Imagine you’re ordering food at a restaurant. Instead of being served a pre-made plate, you tell the waiter exactly what you want, down to the specific ingredients. That’s GraphQL for your APIs — it puts the power in the client’s hands to request only the data they need.
GraphQL was developed by Meta (formerly Facebook) and is now open source, meaning anyone can use it or contribute to its development.
How Does GraphQL Work?
GraphQL acts as a middleman between the client (like your front-end app) and the server. It allows the client to:
Ask for specific data: No more over-fetching or under-fetching of data. You get exactly what you request.
Access multiple resources in one query: Instead of making multiple API calls, GraphQL lets you combine everything into a single query.
Use a schema: GraphQL uses a structured schema to define what data is available and how it can be accessed. Think of it as a blueprint for your API.
Key Benefits of GraphQL
Customizable Queries: Clients request exactly what they need.
Efficient: Combines multiple data sources into one query.
Real-time Data: Supports subscriptions for real-time updates.
Strongly Typed: Ensures clarity and consistency with a schema.
GraphQL vs REST APIs
REST API: Like a buffet. You serve yourself and often take more or less than you need.
GraphQL: Like a restaurant with waiters. You request exactly what you want, and it’s served to you.
Example:
REST API:
// GET user details
GET /users/5
// Response
{
"id": 5,
"name": "John",
"email": "john@example.com",
"posts": ["Post 1", "Post 2"]
}
GraphQL:
query {
user(id: 5) {
name,
email
}
}
// Response
{
"data": {
"user": {
"name": "John",
"email": "john@example.com"
}
}
}
With GraphQL, you only get the name
and email
fields, skipping the rest.
Building a Simple GraphQL API
Let’s create a simple GraphQL query using Express. Imagine a learning management system (LMS):
Database Model
const courses = [
{ id: 1, title: "GraphQL Basics", instructor: "Alice" },
{ id: 2, title: "Advanced GraphQL", instructor: "Bob" },
];
GraphQL Server Code
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
// Define schema
const schema = buildSchema(`
type Course {
id: ID
title: String
instructor: String
}
type Query {
course(id: ID!): Course
}
`);// Resolver functions
const root = {
course: ({ id }) => courses.find(course => course.id == id),
};// Create Express app
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true, // Enables GraphiQL tool
}));app.listen(4000, () => console.log('Server running on http://localhost:4000/graphql'));
Example Query
query {
course(id: 1) {
title
instructor
}
}
// Response
{
"data": {
"course": {
"title": "GraphQL Basics",
"instructor": "Alice"
}
}
}
Subscriptions and Mutations
Subscriptions: Enable real-time updates. For example, live chat or notifications.
Mutations: Used to create, update, or delete data.
Example of a Mutation
mutation {
addCourse(title: "New Course", instructor: "Charlie") {
id
title
}
}
Limitations of GraphQL
Complex Backend: Requires careful setup and maintenance.
Debugging Challenges: Errors can be harder to trace.
Caching Difficulties: Unlike REST, GraphQL doesn’t use HTTP caching out of the box.
Increased Setup: Both client and server need GraphQL support.
Key Terminology in GraphQL
Query: A request to fetch data from the server.
Mutation: An operation to modify data (create, update, or delete).
Schema: A blueprint defining the structure of data available in the API.
Resolver: A function that retrieves the data for a query or mutation.
Subscription: A feature for real-time updates from the server.
When to Use GraphQL
GraphQL shines when:
Your application handles millions of API calls daily.
You need real-time data updates.
Clients require customized data fetching.
But for simpler use cases or small-scale projects, REST APIs might be a better fit.
Conclusion
GraphQL is a modern and powerful alternative to REST APIs. While it offers incredible flexibility and efficiency, it comes with its own set of challenges. If your project demands precise data fetching, reduced API calls, or real-time updates, give GraphQL a try — it might just be the right tool for the job!
References
https://graphql.org/learn/
https://www.youtube.com/watch?v=yWzKJPw_VzM
Subscribe to my newsletter
Read articles from Agrim Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
