Insert and Remove a Footnote in Word Documents Using Python

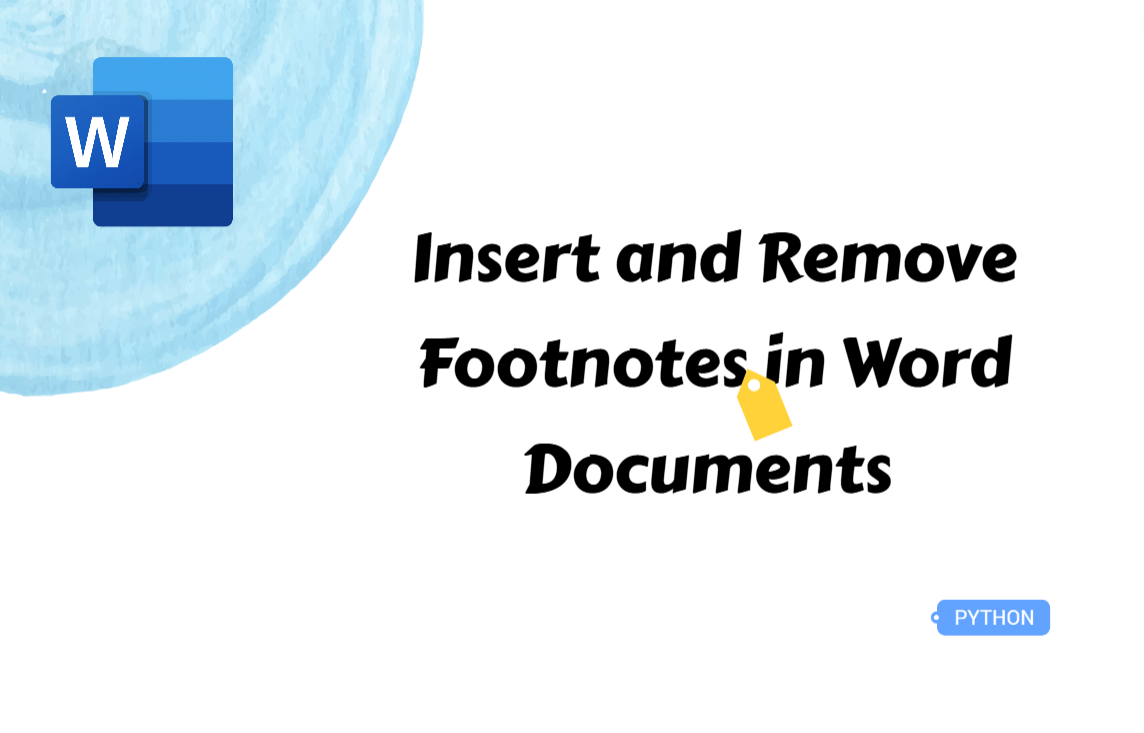
Footnotes allow you to add additional explanations, references, or sources to specific words or sentences without disrupting the main text's layout and logical flow. Typically appearing in the footer, footnotes help maintain document clarity while providing detailed context. However, manually inserting and managing them can be tedious and time-consuming. To streamline this process, this article will explore how to insert and remove footnotes in Word documents using Python, enabling you to create well-structured, professional documents with greater efficiency.
Python Libraries for Inserting and Removing Footnotes in Word
To efficiently insert and remove footnotes in Word documents using Python, a third-party library is the recommended solution. However, the options for open-source libraries are limited. For example, python-docx allows for basic footnote insertion but does not support deletion and only works with .docx files.
In contrast, commercial libraries such as Spire.Doc and Aspose.Words offer more advanced functionality. Beyond inserting and removing footnotes, they support text replacement, formatting, and other detailed document processing tasks.
Due to its intuitive API and reliable technical support, this article will use Spire.Doc to demonstrate the implementation steps and provide code examples. You can install it using the pip command: pip install Spire.Doc
.
How to Insert a Footnote for a Paragraph in Word Documents
In some cases, an entire paragraph may be a cited source, and instead of marking it within the main text, you can reference it in a footnote for better readability. Adding a footnote to a specific paragraph in a Word document involves locating the paragraph and using the Paragraph.AppendFootnote()
method to insert the footnote. Additionally, you can customize its content, font, and formatting to match your document’s style. Let’s explore the step-by-step process to achieve this.
Steps to insert a footnote for a paragraph in Word documents:
Create an instance of the Document class.
Load a source Word document from files using the Document.LoadFromFile() method.
Get a section through the Document.Sections[] property and access the specified paragraph with the Section.Paragraphs[] property.
Insert a footnote at the end of the paragraph using the Paragraph.AppendFootnote() method.
Customize the content, font, and color of the footnote.
Save the updated Word document as a new one through the Document.SaveToFile() method.
Here is a Python example showing how to insert a footnote for the fifth paragraph in a Word document:
from spire.doc import *
from spire.doc.common import *
# Create a Document instance
document = Document()
# Load a sample Word document
document.LoadFromFile("/sample.docx")
# Get the first section
section = document.Sections[0]
# Get a specified paragraph in the section
paragraph = section.Paragraphs[5]
# Add a footnote at the end of the paragraph
footnote = paragraph.AppendFootnote(FootnoteType.Footnote)
# Set the text content of the footnote
text = footnote.TextBody.AddParagraph().AppendText("This is a sample footnote.")
# Set the text font and color
text.CharacterFormat.FontName = "Arial"
text.CharacterFormat.FontSize = 12
text.CharacterFormat.TextColor = Color.get_DarkBlue()
# Set the font and color of the footnote reference mark
footnote.MarkerCharacterFormat.FontName = "Calibri"
footnote.MarkerCharacterFormat.FontSize = 15
footnote.MarkerCharacterFormat.Bold = True
footnote.MarkerCharacterFormat.TextColor = Color.get_DarkCyan()
# Save the result document
document.SaveToFile("/AddFootnoteForParagraph.docx", FileFormat.Docx2016)
document.Close()
How to Insert a Footnote for Text in Word Filles
When your text includes elements that need further explanation—such as a famous name, a technical term, or an acronym—footnotes provide a clear and unobtrusive way to add context. Unlike adding a footnote to an entire paragraph, inserting one for specific text requires first locating the target word or phrase. This can be done using the Document.FindString()
method to find the first match, followed by adding a footnote at that position. The following part provides a step-by-step guide and code examples to demonstrate this process.
Steps to insert a footnote for specified text in Word documents:
Create a Document class and read a Word document through the Document.LoadFromFile() method.
Find the specified text using the Document.FindString() method.
Get the text as a text range with the TextSelection.GetAsOneRange() method.
Get the paragraph where the text range is located through the TextRange.OwnerParagraph property.
Get the index position of the text range in the paragraph with the Paragraph.ChildObjects.IndexOf() method.
Add a footnote for the paragraph using the Paragraph.AppendFootnote() method, and then insert a footnote after the specified text with the Paragraph.ChildObjects.Insert() method.
Set the content, font, and color of the footnote.
Save the updated Word document as a new one through the Document.SaveToFile() method.
Below is a code example showing how to insert a footnote for “artistic community” in a Word file:
from spire.doc import *
from spire.doc.common import *
# Create a Document instance
document = Document()
# Load a sample Word document
document.LoadFromFile("/sample.docx")
# Find a specific text
selection = document.FindString("artistic community", False, True)
# Get the found text as a single text range
textRange = selection.GetAsOneRange()
# Get the paragraph where the text range is located
paragraph = textRange.OwnerParagraph
# Get the index position of the text range in the paragraph
index = paragraph.ChildObjects.IndexOf(textRange)
# Add a footnote to the paragraph
footnote = paragraph.AppendFootnote(FootnoteType.Footnote)
# Insert the footnote after the text range
paragraph.ChildObjects.Insert(index + 1, footnote)
# Set the text content of the footnote
text = footnote.TextBody.AddParagraph().AppendText("Sample Footnote")
# Set the text font and color
text.CharacterFormat.FontName = "Arial"
text.CharacterFormat.FontSize = 12
text.CharacterFormat.TextColor = Color.get_DarkBlue()
# Set the font and color of the footnote reference mark
footnote.MarkerCharacterFormat.FontName = "Calibri"
footnote.MarkerCharacterFormat.FontSize = 15
footnote.MarkerCharacterFormat.Bold = True
footnote.MarkerCharacterFormat.TextColor = Color.get_DarkGreen()
# Save the result document
document.SaveToFile("/AddFootnoteForText.docx", FileFormat.Docx2016)
document.Close()
How to Remove Footnotes from Word Documents
When footnotes are no longer needed, or you want to update your document, removing them efficiently can save time. With Spire.Doc, you can use the Paragraph.ChildObjects.RemoveAt()
method to delete footnotes in bulk, eliminating the need for manual deletion. Let's walk through the detailed step-by-step guide.
Steps to remove footnotes from a Word document:
Create an object of the Document class.
Load a Word document with footnotes through the Document.LoadFromFile() method.
Get a certain section with the Document.Sections[] property.
Loop through paragraphs in the section to find footnotes.
- Remove each footnote using the Paragraph.ChildObjects.RemoveAt() method.
Save the modified Word document.
Here is the code example of removing all footnotes from a Word file:
from spire.doc import *
from spire.doc.common import *
# Create a Document instance
document = Document()
# Load a sample Word document
document.LoadFromFile("/AddFootnoteForParagraph.docx")
# Get the first section
section = document.Sections[0]
# Loop through the paragraphs in the section
for y in range(section.Paragraphs.Count):
para = section.Paragraphs.get_Item(y)
index = -1
i = 0
cnt = para.ChildObjects.Count
while i < cnt:
pBase = para.ChildObjects[i] if isinstance(para.ChildObjects[i], ParagraphBase) else None
if isinstance(pBase, Footnote):
index = i
break
i += 1
if index > -1:
# Remove the footnotes from the paragraph
para.ChildObjects.RemoveAt(index)
# Save the result document
document.SaveToFile("/RemoveFootnotes.docx", FileFormat.Docx)
document.Close()
The Conclusion
This article explored how to insert and remove footnotes in Word documents using Python, covering both adding footnotes to specific paragraphs and inserting them for selected text. To help you understand and implement these techniques, we provided a detailed step-by-step guide along with code examples. By now, you should be ready to automate the process effortlessly with Python. Give it a try and enhance your document editing efficiency!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
