Step-by-Step Guide to Implement Real-time Sockets in Express.js with Next.js

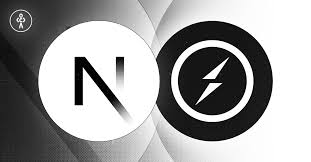
Real-time applications are becoming more common, from chat applications to live notifications and collaborative tools. In this blog, we will explore how to integrate sockets with Express.js using socket.io
to enable real-time communication and connect it with a Next.js client.
Understanding Sockets and Socket.IO
Sockets allow bidirectional communication between a client and a server. socket.io
is a popular library that simplifies working with WebSockets by providing event-driven APIs and fallbacks for older browsers.
Setting Up Sockets in Express.js
Step 1: Initialize an Express.js Application
First, install Express and socket.io
:
npm init -y
npm install express socket.io cors
Create a basic Express server:
const express = require("express");
const http = require("http");
const { Server } = require("socket.io");
const cors = require("cors");
const app = express();
const server = http.createServer(app);
const io = new Server(server, {
cors: {
origin: "*",
},
});
app.use(cors());
app.get("/", (req, res) => {
res.send("Sockets with Express.js");
});
server.listen(5000, () => {
console.log("Server is running on port 5000");
});
Step 2: Implement Socket Event Handling
Add event listeners for socket connections:
io.on("connection", (socket) => {
console.log("A user connected with socketId:", socket.id);
socket.on("message", (data) => {
console.log("Message received:", data);
io.emit("message", data);
});
socket.on("disconnect", () => {
console.log("A user disconnected with socketId", socket.id);
});
});
Optional: Adding Authentication to Your Socket App
If your application requires authentication, you can implement it in your socket.io
server using middleware. Add the following code before the previous socket setup:
io.use(async (socket, next) => {
try {
const token = socket.handshake.auth.token;
const decoded = verifyToken(token);
if (!decoded) {
return next(new Error("Authentication failed: Invalid token"));
}
const { id: userId } = decoded;
socket.userId = userId;
if (userId) {
socket.join(userId);
return next();
}
return next(new Error("Authentication failed: Missing token"));
} catch (error) {
console.error("Error in socket middleware:", error);
return next(new Error("Internal server error"));
}
});
Step 3: Setting Up a Next.js Project
First, create a new Next.js project if you don’t already have one:
yarn create next-app my-socket-app
cd my-socket-app
yarn
Then, install socket.io-client
to connect with the backend socket server:
yarn add socket.io-client
Optional, If you’re using authentication, install next-auth
as well:
yarn add next-auth
Now, your Next.js project is ready for socket integration.
The Right Way to Manage Sockets in React/Nextjs (SocketContextProvider)
A common mistake many junior developers make is initializing the socket instance inside multiple components. This leads to multiple socket connections being opened, causing unnecessary network usage, performance issues, and even unexpected behavior like duplicate event listeners.
To solve this, we create a SocketContextProvider globally. This ensures that there is only one socket connection shared across the entire application. By managing the socket instance at a higher level, we maintain a single source of truth, prevent duplicate connections, and make it easier to manage socket events efficiently across different components.
"use client";
import { SOCKET_URL } from "@/app/utils/constants";
// import { useSession } from "next-auth/react";
import React, { createContext, useContext, useEffect, useState } from "react";
import { io } from "socket.io-client";
const SocketContext = createContext(null);
export const useSocketContext = () => {
const context = useContext(SocketContext);
if (!context) {
throw new Error("useSocketContext must be used within a SocketContextProvider");
}
return context;
};
export const SocketContextProvider = ({ children }) => {
const [socket, setSocket] = useState(null);
//const { data } = useSession();
useEffect(() => {
if (data?.user?.accessToken) {
const newSocket = io(SOCKET_URL, {
// Optional: Use this if you need to authenticate users.
// auth: { token: data.user.accessToken },
reconnectionAttempts: 100,
reconnectionDelay: 3000,
reconnection: true,
autoConnect: true,
transports: ["websocket"],
});
setSocket(newSocket);
console.log("Connecting...", newSocket.connected);
return () => {
newSocket.disconnect();
};
}
}, [data?.user?.accessToken]);
return (
<SocketContext.Provider value={{ socket }}>
{children}
</SocketContext.Provider>
);
};
Updating layout.js
to Include SocketContextProvider
import { SocketContextProvider } from "@/app/context/SocketContextProvider";
import NextAuthProvider from "@/app/context/session-provider";
export const metadata: Metadata = {
title: "Socket Chat-app",
description: "A Simple Chatting Application",
};
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<NextAuthProvider>
<SocketContextProvider>
{children}
</SocketContextProvider>
</NextAuthProvider>
</body>
</html>
);
}
Using the Socket Context in Components
Now, you can use the socket in any component by consuming the context:
"use client";
import { useState , useEffect } from "react";
import { useSocketContext } from "@/app/context/SocketContextProvider";
export default function Chat() {
const { socket } = useSocketContext();
const [message, setMessage] = useState("");
const [messages, setMessages] = useState([]);
useEffect(() => {
if (!socket) return;
const handleMessage = (data) => {
setMessages((prev) => [...prev, data]);
};
socket.on("message", handleMessage);
// Cleanup listener on unmount
return () => {
socket.off("message", handleMessage);
};
}, [socket]);
const sendMessage = () => {
if (message.trim() !== "") {
socket?.emit("message", message);
setMessage("");
}
};
return (
<div>
<h1>Next.js Chat App</h1>
<input
type="text"
value={message}
onChange={(e) => setMessage(e.target.value)}
placeholder="Enter message"
/>
<button onClick={sendMessage}>Send</button>
<ul>
{messages.map((msg, index) => (
<li key={index}>{msg}</li>
))}
</ul>
</div>
);
}
Step 4: Run the Application
Start the Express server:
node server.js
Run the Next.js application:
yarn dev
Navigate to the Next.js app, and test sending messages in real-time!
Conclusion
Using socket.io
with Express.js and Next.js enables real-time communication for chat apps, live notifications, and more. This setup provides an easy way to integrate sockets into a modern web application.
Happy coding!
Subscribe to my newsletter
Read articles from Ayush Dixit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ayush Dixit
Ayush Dixit
I'm a passionate MERN stack developer who loves building websites and apps. I enjoy solving problems and bringing ideas to life through code. I believe in learning something new every day to improve my skills and keep up with the latest technologies. I’m always excited to work with other developers, share knowledge, and contribute to open-source projects. Let’s connect and create something great together!