How to Set Up and Use Shopify Webhooks in Your Tech Stack
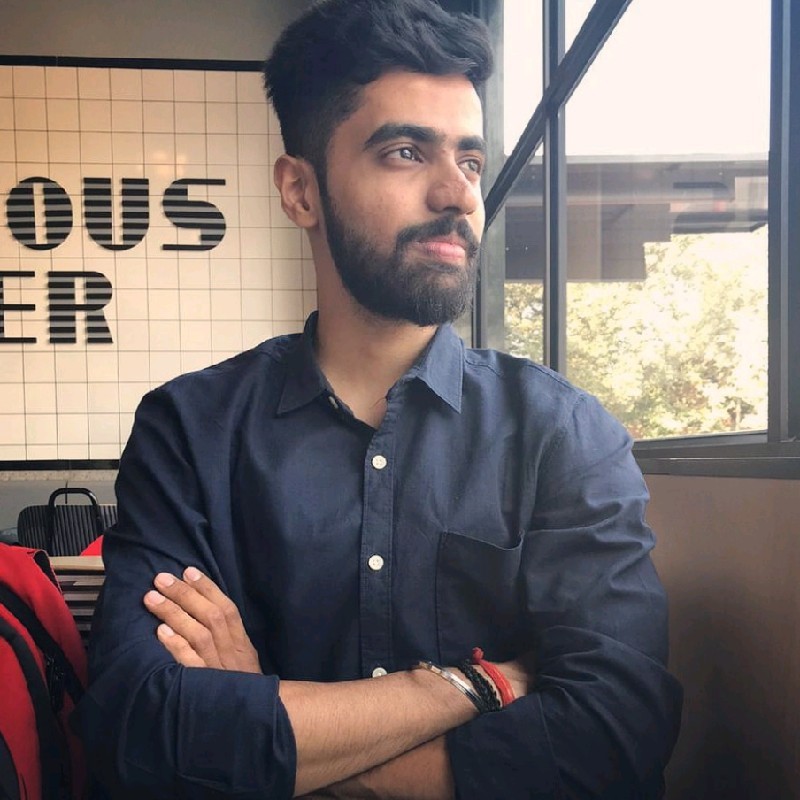
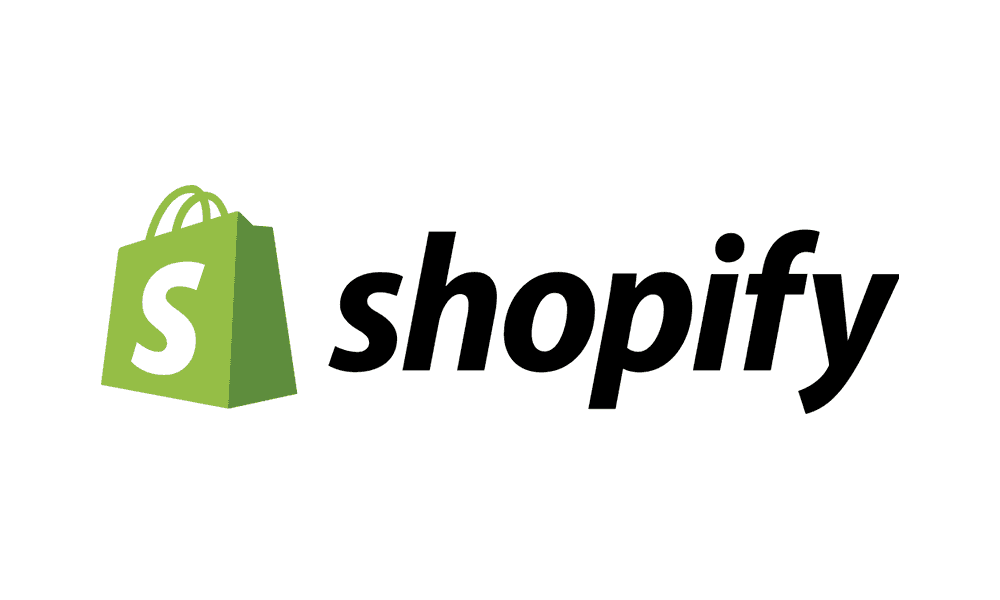
Shopify webhooks are powerful tools that allow developers to automate processes and integrate external systems with Shopify stores. Whether you want to sync orders, manage inventory, or trigger automated workflows, webhooks can help streamline operations in real time.
In this article, you'll learn how to set up Shopify webhooks, test them using ngrok, and integrate them into various tech stacks such as Node.js, Python, PHP, .NET, and more.
๐ What Are Shopify Webhooks?
Shopify webhooks are HTTP callbacks that notify your server whenever specific events occur in your store. These include order creation, product updates, customer sign-ups, and more.
Why Use Webhooks?
Real-time Order Processing โ Sync orders instantly with ERP, CRM, or shipping providers.
Inventory Management โ Keep stock levels updated across multiple platforms.
Automate Workflows โ Send notifications, trigger email campaigns, or log analytics.
Third-party Integrations โ Connect Shopify with apps like Zapier, Slack, and accounting software.
๐ ๏ธ Setting Up Shopify Webhooks (Admin Panel Method)
Go to Shopify Admin โ Settings โ Notifications.
Scroll to Webhooks and click Create webhook.
Choose an Event (e.g., "Order Creation").
Enter a Webhook URL (your server's endpoint).
Choose JSON format.
Click Save.
Whenever the event occurs, Shopify will send a POST
request to your webhook URL with relevant data.
๐ Setting Up Webhooks Using Shopify API
For a programmatic approach, use Shopify's Admin API:
Create a Webhook via API
POST https://{your-shop-name}.myshopify.com/admin/api/2024-01/webhooks.json
Request Body:
{
"webhook": {
"topic": "orders/create",
"address": "https://your-webhook-url.com/webhook/orders",
"format": "json"
}
}
Headers:
Content-Type: application/json
X-Shopify-Access-Token: {your-access-token}
๐ Testing Webhooks with ngrok
Since Shopify webhooks require a public URL, you can use ngrok to tunnel requests to your local server:
Steps:
Install ngrok:
brew install ngrok # For macOS
Run your local server (Node.js example):
const express = require("express"); const app = express(); app.use(express.json()); app.post("/webhooks/orders", (req, res) => { console.log("Received Order Webhook:", req.body); res.status(200).send("Webhook Received"); }); app.listen(3000, () => console.log("Server running on port 3000"));
Expose your local server using ngrok:
ngrok http 3000
Copy the HTTPS URL from ngrok's output and use it as your webhook URL in Shopify.
๐ Shopify Webhooks in Different Tech Stacks
Webhooks work with various technologies. Below are quick examples of handling a Shopify webhook in different programming languages.
๐ Python (Flask)
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route("/webhooks/orders", methods=["POST"])
def shopify_webhook():
data = request.get_json()
print("Order received:", data)
return "Webhook Received", 200
if __name__ == "__main__":
app.run(port=5000)
๐ PHP (Laravel)
Route::post('/webhooks/orders', function () {
$data = json_decode(file_get_contents('php://input'), true);
Log::info('Shopify Webhook:', $data);
return response('Webhook Received', 200);
});
๐ .NET (C# / ASP.NET Core)
[HttpPost("/webhooks/orders")]
public IActionResult ShopifyWebhook([FromBody] dynamic data)
{
Console.WriteLine("Order Received: " + data);
return Ok("Webhook Received");
}
๐ Securing Shopify Webhooks
To ensure that webhook requests come from Shopify, validate them using HMAC SHA-256.
Example in Node.js:
const crypto = require("crypto");
function verifyWebhook(req, res, next) {
const secret = "your-shopify-app-secret";
const hmac = req.headers["x-shopify-hmac-sha256"];
const body = JSON.stringify(req.body);
const generatedHmac = crypto
.createHmac("sha256", secret)
.update(body, "utf8")
.digest("base64");
if (generatedHmac === hmac) {
console.log("โ
Webhook verified!");
next();
} else {
console.log("โ Webhook verification failed!");
res.status(401).send("Unauthorized");
}
}
Use this middleware before your webhook route for added security.
๐ ๏ธ Real-Life Use Cases of Shopify Webhooks
โ
Sync Orders & Inventory โ Send orders to shipping providers or fulfillment centers.
โ
CRM & Marketing Automation โ Capture customer data for email marketing.
โ
Fraud Detection โ Flag high-risk orders for manual review.
โ
Mobile App Updates โ Notify apps when new products are added.
๐ Conclusion
Shopify webhooks are a game-changer for developers and store owners who need real-time automation and integrations. By following this guide, you can: โ Set up webhooks in Shopify Admin & API โ Test them using ngrok โ Implement webhook handlers in Node.js, Python, PHP, .NET, etc. โ Secure them with HMAC verification
Start integrating webhooks today and automate your Shopify workflows like a pro! ๐
Subscribe to my newsletter
Read articles from Shikhar Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
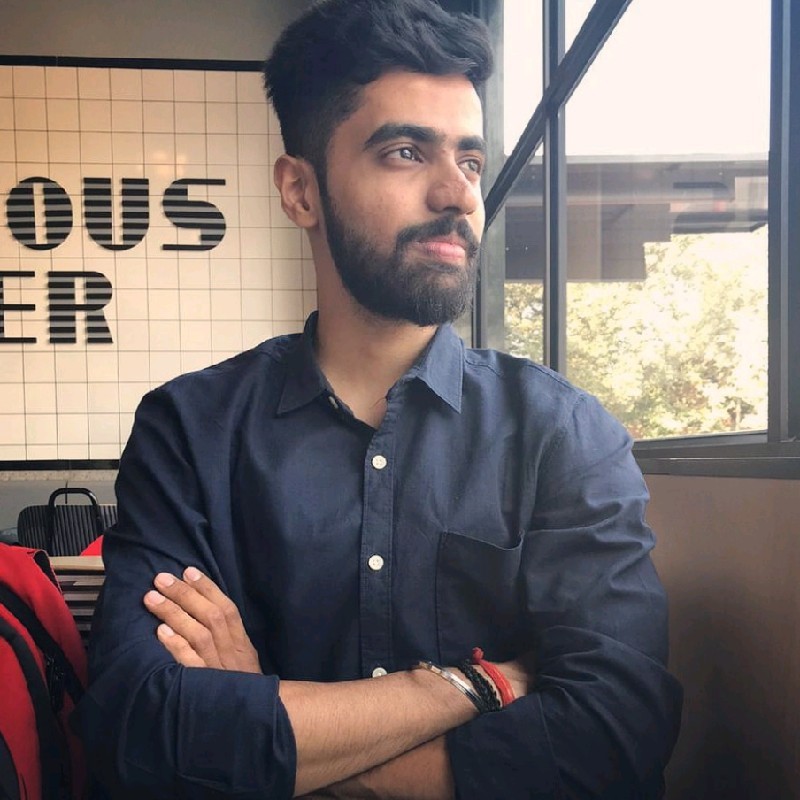