Building a Chain-Agnostic Wallet with Onebalance: A Step-by-Step Guide
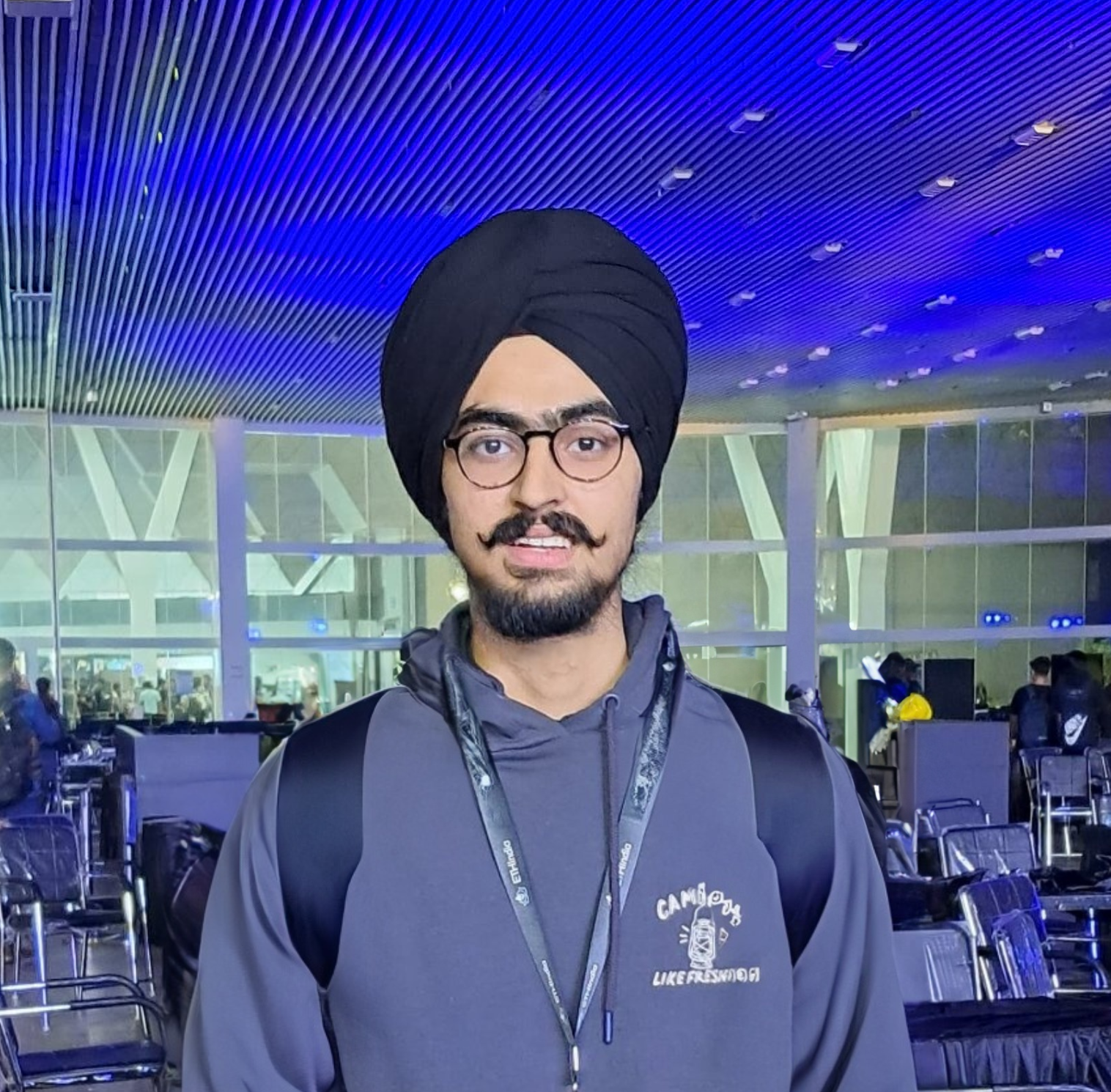
Table of contents
- What is OneBalance
- Getting Started
- Step 0 - Create a NextJS App
- Step 1 - Initialise the Application with Privy
- Step 2 - Set up React Query
- Step 3 - Create a Onebalance Account
- Step 4 - Get the Onebalance Account Balance
- Step 5 - Supported Chains and Assets
- Step 6 - Cross-Chain Transfer of Funds
- Step 7 - Fetch the Status of our Quote
- Step 8 - Cross-Chain Swap of Funds
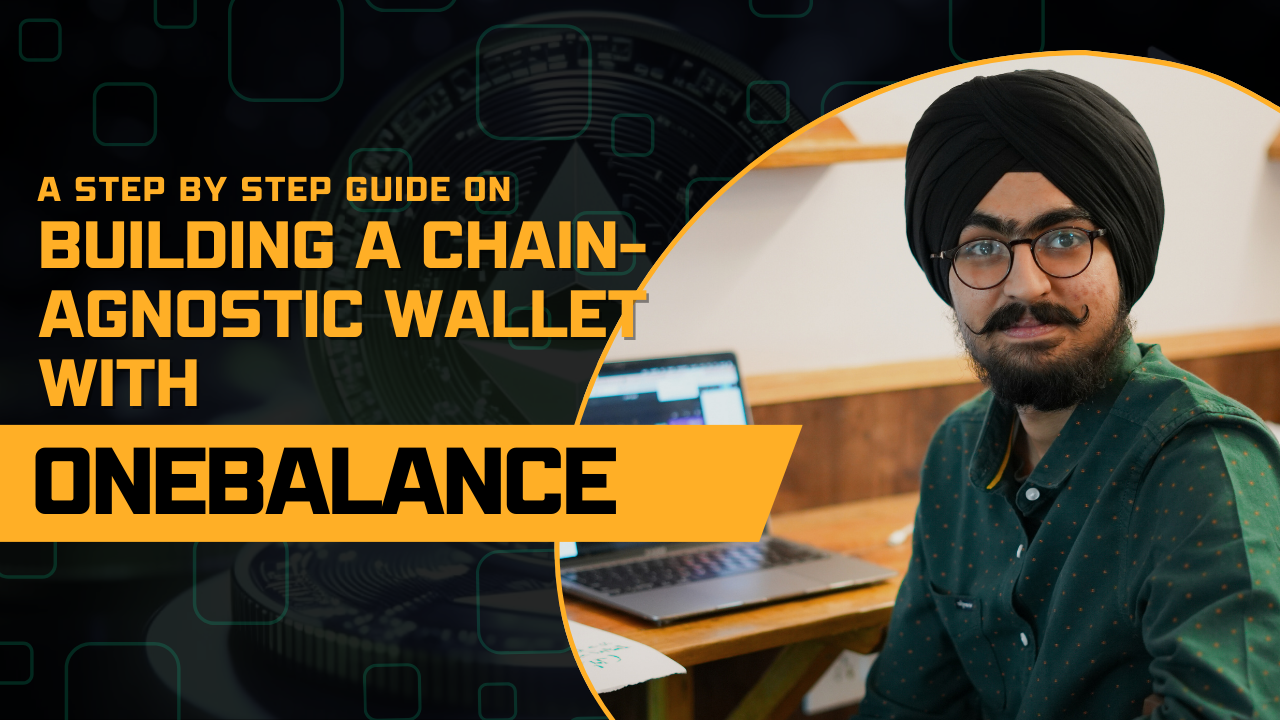
Ethereum started as a single, unified blockchain, offering great usability but struggling with scalability. The rise of Layer 2s (L2s) solved the scaling issue but introduced a new problem—fragmented liquidity and a confusing user experience. With hundreds of chains hosting the same assets, developers face tough choices on where to deploy, while users struggle with high bridge fees, long wait times, and constant asset transfers just to interact with an application.
But what if you didn’t have to choose a chain at all?
Imagine an account that seamlessly works across any EVM chain, Solana, or even Bitcoin without ever bridging funds. Your portfolio becomes an aggregated asset pool, abstracting away the complexity of chains while retaining their use cases.
🚀 Onebalance makes this a reality, shifting the paradigm from a chain-centric to an account-centric world—empowering developers to build frictionless, next-gen applications that can onboard the next billion users.
What is OneBalance
Onebalance is a framework to create Credible accounts that are cheap and fast as EOA and have a global consensus of Smart Wallets so you enjoy all the features like abstraction, social recovery, permission policies, and modern authentication methods across chains.
Clearing up some jargon that you may come across -
Credible Commitment - An unbreakable promise just like what Professor Severus Snape makes in Harry Potter.
Resource Locks - Time-based locking of assets which gets executed if the conditions are met.
Credible Accounts - Accounts living in a secure computer that makes credible commitments.
Credible Commitment Machine - Computer where accounts live that handles all the resource locks. eg. Trusted Execution Environments (TEEs), Multi-Party Computation/chain signatures (MPC), Smart Contract Accounts (SCAs), and in protocol virtual machine changes.
Learning Outcomes
Following this guide, you’ll learn how to supercharge your NextJS DApp with cross-chain magic UX using Onebalance.
We will create a Chain Agnostic Wallet to
Create your Onebalance Account using Privy
Get the Aggregated balance of your Account across the chain
Transfer the funds from your Onebalance account
Cross-chain swap from your Onebalace Account
I am omitting the UI part of the application but will integrate the flow of the application.
If you are stuck at any point, you can use this Github Repo for reference → Onewallet
Prerequisites
Before starting, ensure that you have the following:
Node.js (v18 or higher)
npm or Yarn
Basic experience with React (It’s a NextJS Project)
If you're missing any of the above:
Download Node.js here
Learn React from its official documentation.
Getting Started
Tech Stack:
NextJS: For Full Stack Framework.
Privy: For WalletConnect integration.
Viem: For interacting with EVM chains.
React Query: For data fetching.
Tailwind CSS: For styling.
ShadCN: UI components.
TypeScript: Type Safety
I have tried to make the application simple by removing any UI components, if you are uncomfortable, you can implement useEffect
instead of React Query and plain old Javascript over TS.
Step 0 - Create a NextJS App
Start by creating a NextJS project with Typescript and Tailwind CSS.
npx create-next-app@14 one-wallet --typescript --tailwind --eslint --app --src-dir --import-alias "@/*"
Step 1 - Initialise the Application with Privy
Next, let’s set up Privy, which helps manage authentication and embedded wallets seamlessly. This enables users to onboard quickly without needing an external wallet.
Install the dependencies.
npm install @privy-io/react-auth@latest
Create a
.env.local
file in the root directory add your Privy App ID (which you can get from Privy Dashboard):NEXT_PUBLIC_PRIVY_APP_ID=<your-privy-app-id>
Now, initialize Privy in a new provider component. This will allow us to manage authentication globally across the app.
// /providers/privy-provider.tsx "use client"; import { PrivyProvider } from "@privy-io/react-auth"; export function PrivyClientProvider({ children, }: { children: React.ReactNode; }) { return ( <PrivyProvider appId={process.env.NEXT_PUBLIC_PRIVY_APP_ID!} config={{ loginMethods: ["email", "wallet"], // Allow login via email or external wallet embeddedWallets: { ethereum: { createOnLogin: "users-without-wallets", // defaults to 'off' }, }, appearance: { theme: "dark", accentColor: "#676FFF", showWalletLoginFirst: true, }, }} > {children} </PrivyProvider> ); }
Now, wrap the PrivyProvider inside the body.
// /app/layout.tsx. ... import { PrivyClientProvider } from "@/providers/privy-provider"; export default function RootLayout({ children, }: { children: React.ReactNode; }) { return ( <html lang="en"> <body className={inter.className}> <PrivyClientProvider>{children}</PrivyClientProvider> </body> </html> ); }
Create a Login Component
Now let’s build a simple login button. This will handle user authentication and redirect them to the dashboard upon login.
// /components/auth/login-button.tsx "use client"; import { usePrivy } from "@privy-io/react-auth"; import { useRouter } from "next/navigation"; import { useEffect } from "react"; import { Button } from "@/components/ui/button"; export function LoginButton() { const { login, ready, authenticated } = usePrivy(); const router = useRouter(); useEffect(() => { if (ready && authenticated) { router.push("/dashboard"); // Redirects to the Dashboard page once you login } }, [ready, authenticated, router]); return ( <Button onClick={login} disabled={!ready || authenticated} size="lg" className="w-full bg-blue-600 hover:bg-blue-700 text-white font-medium py-3 rounded-lg" > Connect Wallet </Button> ); }
Step 2 - Set up React Query
Since we will be interacting with a lot of APIs, I am using React query to simplify it, you can skip this and implement all this in useEffect
for simplicity but I recommend using it.
Let’s install the dependencies.
npm i @tanstack/react-query
Wrap the provider in the App.
// /app/layout.tsx ... import { QueryClientProvider } from "@/providers/query-provider"; export default function RootLayout({ children, }: { children: React.ReactNode; }) { return ( <html lang="en"> <body className={inter.className}> <QueryClientProvider> <PrivyClientProvider>{children}</PrivyClientProvider> </QueryClientProvider> </body> </html> ); }
Now you are good to use React Query in the Application.
Step 3 - Create a Onebalance Account
Now comes the core part of the tutorial—creating a OneBalance Account.
OneBalance as of now utilizes ERC-4337 (Account Abstraction) to create smart contract wallets. The benefit of this approach is that we can predict the wallet’s address even before deploying it on-chain, allowing us to send funds or perform off-chain operations in advance.
1. Get the API Key
To interact with OneBalance, you need an API Key. You can obtain it by:
Contacting the OneBalance team
Checking the OneBalance API Documentation
2. Set Up Environment Variables
Open your .env.local
file and add the following:
# OneBalance API Configuration
ONEBALANCE_BASE_URL=<Base-URL>
ONEBALANCE_API_KEY=<API-Key>
# Privy Configuration
NEXT_PUBLIC_PRIVY_APP_ID=<Privy-App-ID>
Note: The
ONEBALANCE_BASE_URL
should point to OneBalance's API endpoint.
3. Predict the OneBalance Address
Since OneBalance accounts use ERC-4337, we can predict the address before even deploying.
These details are needed for creation:
Session Address - Your Privy’s Embedded Wallet address
Admin Address - OneBalance allows an admin address to be set as a backup for account recovery. If you don't need an admin, set it to Zero Address (0x0000...0000).
4. Create a Server-Side Function to Fetch the Predicted Address
Now, let’s create a server-side function to predict the OneBalance address.
// /app/actions/account.ts
'use server';
import { PredictAddressRequest, PredictAddressResponse } from '@/types/account';
import { ADMIN_ADDRESS } from '@/constants'; // Set it to Zero Address if no admin needed.
export async function predictAddress(sessionAddress: string): Promise<PredictAddressResponse> {
const response = await fetch(`${process.env.ONEBALANCE_BASE_URL}/api/account/predict-address`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'x-api-key': process.env.ONEBALANCE_API_KEY!
},
body: JSON.stringify({
sessionAddress, // The Privy embedded wallet address
adminAddress: ADMIN_ADDRESS,
} as PredictAddressRequest),
});
if (!response.ok) {
throw new Error('Failed to predict address');
}
return response.json();
}
5. Create a React Hook to Fetch the Address
We will create a React hook eventually fetching it using React query to handle all the data.
// /hooks/useAccount.ts
import { useQuery } from '@tanstack/react-query';
import { useWallets } from '@privy-io/react-auth';
import { predictAddress } from '@/app/actions/account';
export function useAccount() {
const { wallets } = useWallets();
const sessionAddress = wallets.find(wallet => wallet.walletClientType === 'privy')?.address; // Wallet address for Privy Embedded Wallet
const { data, isLoading, error } = useQuery({
queryKey: ['account', 'predict-address', sessionAddress],
queryFn: async () => {
if (!sessionAddress) {
throw new Error('Session address is required');
}
return await predictAddress(sessionAddress);
},
enabled: !!sessionAddress, // Only fetch if sessionAddress exists
});
return {
sessionAddress,
predictedAddress: data?.predictedAddress,
isLoading,
error
};
}
Now that we have the useAccount
hook, you can use it anywhere in your app to access the predicted address.
Step 4 - Get the Onebalance Account Balance
Once we have access to the address, let’s get the cross-chain account balance.
The best part is that u do not need to fetch it individually as we can get it straightaway by a simple API call from Onebalance.
Let’s create the action to fetch the balance.
// app/actions/balance.ts 'use server'; import { AggregatedBalance } from '@/types/balance'; export async function getAggregatedBalance(address: string): Promise<AggregatedBalance> { const response = await fetch( `${process.env.ONEBALANCE_BASE_URL}/api/balances/aggregated-balance?address=${address}`, { headers: { 'x-api-key': process.env.ONEBALANCE_API_KEY! } } ); if (!response.ok) { throw new Error('Failed to fetch balance'); } return response.json(); }
Let’s create that React hook which will give us all the Account information including our Onebalance Address.
// /hooks/useBalance.ts import { useQuery } from '@tanstack/react-query'; import { getAggregatedBalance } from '@/app/actions/balance'; import type { AggregatedBalance } from '@/types/balance'; import { useAccount } from './useAccount'; export function useBalance() { const { predictedAddress, isLoading: isLoadingAccount, error: accountError } = useAccount(); const { data, isLoading: isLoadingBalance, error: balanceError } = useQuery<AggregatedBalance>({ queryKey: ['balance', predictedAddress], queryFn: async () => { if (!predictedAddress) { throw new Error('Address is required'); } return await getAggregatedBalance(predictedAddress); }, enabled: !!predictedAddress, }); return { predictedAddress, balance: data, isLoading: isLoadingAccount || isLoadingBalance, error: accountError || balanceError }; }
Now that we have the useBalance
hook, you can use it anywhere in your app to display the account balance. Just call it in your components and render the balance accordingly.
Step 5 - Supported Chains and Assets
Before adding any funds to this Onebalance account, we need to check the supported chains and supported assets so we don’t lose funds by sending a non-supported token.
A simple GET request to fetch all the assets.
// app/actions/assets.ts 'use server'; import { Assets } from '@/types/asset'; export async function getSupportedAssets(): Promise<Assets> { try { const response = await fetch(`${process.env.ONEBALANCE_BASE_URL}/api/assets/list`, { method: 'GET', headers: { 'x-api-key': process.env.ONEBALANCE_API_KEY!, 'Content-Type': 'application/json', 'Accept': 'application/json' } }); if (!response.ok) { throw new Error(`Failed to fetch assets: ${response.status}`); } return response.json(); } catch (error) { console.error('Error in getSupportedAssets:', error); throw error; } }
Now let’s create that React hook which will give us the list of supported assets.
// /hooks/useAsset.ts import { useQuery } from '@tanstack/react-query'; import { getSupportedAssets } from '@/app/actions/asset'; import type { Asset, Assets } from '@/types/asset'; export function useAsset() { const { data, isLoading, error } = useQuery<Assets>({ queryKey: ['assets', 'supported'], queryFn: async () => { try { const result = await getSupportedAssets(); return result; } catch (error) { throw error; } }, enabled: true, retry: false, }); // Helper to get asset info by ID const getAssetById = (assetId: string): Asset | undefined => { return data?.find(asset => asset.aggregatedAssetId === assetId); }; // Helper to get asset by symbol const getAssetBySymbol = (symbol: string): Asset | undefined => { return data?.find(asset => asset.symbol.toLowerCase() === symbol.toLowerCase()); }; return { assets: data, isLoading, error, getAssetById, getAssetBySymbol, }; }
This list of assets from useAsset
hook will be useful next to perform transfer and cross-chain swaps.
Step 6 - Cross-Chain Transfer of Funds
Before transferring any funds, we must ensure that we can move assets between chains and withdraw funds to our EOA. This process involves fetching a transfer quote, signing the operation, and executing it securely.
1. Understanding the Transfer Process
Get the Quote:
Fetch the potential outcome of the trade (e.g., transferring
0.1 ETH on Base
toUSD on Arbitrum
).Receive details such as the amount to be received and resource lock time.
Sign the Quote:
- Use our Embedded Wallet to sign the ERC-4337 User Operations.
Execution:
Resource Locking: While the trade is being processed,
0.1 ETH
on Base is locked.Solver Fulfillment: A solver attempts to fulfill the request.
Settlement:
If successful, the equivalent amount in USD on Arbitrum is received.
The locked ETH is sent to the solver.
If unsuccessful, the funds are unlocked.
2. Fetching the Transfer Quote
The following function fetches a transfer quote from the OneBalance API.
// app/actions/transferQuote.ts
'use server';
import type { TransferQuoteRequest, Quote } from '@/types/quote';
const API_BASE_URL = process.env.ONEBALANCE_BASE_URL;
const API_KEY = process.env.ONEBALANCE_API_KEY;
export async function getTransferQuote(request: TransferQuoteRequest): Promise<Quote> {
try {
console.log(request);
const response = await fetch(`${API_BASE_URL}/api/quotes/transfer-quote`, {
method: 'POST',
headers: {
'x-api-key': API_KEY!,
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: JSON.stringify(request)
});
if (!response.ok) {
const error = await response.json();
console.error('Error in getTransferQuote:', error);
throw new Error(`Failed to fetch transfer quote: ${response.status}`);
}
const data = await response.json();
console.log(data);
return data;
} catch (error) {
console.error('Error in getTransferQuote:', error);
throw error;
}
}
3. React Hook: Fetching the Quote
A custom React hook that leverages React Query to fetch the quote.
// /hooks/useTransferQuote.ts
import { useQuery } from '@tanstack/react-query';
import type { TransferQuoteRequest, Quote } from '@/types/quote';
import { getTransferQuote } from '@/app/actions/transferQuote';
export function useTransferQuote(request: TransferQuoteRequest | null) {
const { data: quote, isLoading, error } = useQuery<Quote>({
queryKey: ['transfer-quote', request],
queryFn: async () => {
if (!request) {
throw new Error('Transfer quote request is required');
}
return await getTransferQuote(request);
},
enabled: !!request,
});
return {
quote,
isLoading,
error,
};
}
4. Constructing the Transfer Quote Request
The transfer request includes:
Session Address: Embedded wallet managing OneBalance Account.
Predicted Address: The OneBalance Account Address.
Recipient Account ID: CAIP-10 formatted address (
namespace:reference:address
).Amount & Asset ID: The amount and aggregated asset ID of token to be transferred.
We will send these parameters to our hook to get the quote.
// components/Transfer.tsx
import React, { useState, useMemo } from "react";
import { useTransferQuote } from "@/hooks/useTransferQuote";
import { useAccount } from "@/hooks/useAccount";
....
export function Transfer() {
const { predictedAddress, sessionAddress } = useAccount();
// React States to store the receipient transfer data from any input component
const [selectedAsset, setSelectedAsset] = useState("");
const [amount, setAmount] = useState("");
const [recipientAddress, setRecipientAddress] = useState("");
const [selectedChain, setSelectedChain] = useState("");
// Prepare quote request
const quoteRequest = useMemo<TransferQuoteRequest | null>(() => {
if (
!selectedAsset ||
!BigInt(amount) ||
!recipientAddress ||
!predictedAddress ||
!sessionAddress
) {
return null;
}
// Format recipient account ID according to CAIP-10: namespace:reference:address
const namespace = "eip155"; // Ethereum namespace
const reference = selectedChain || "1"; // Default to mainnet if no chain selected
const recipientAccountId = `${namespace}:${reference}:${recipientAddress}; //` (Unable to add this ` without comment due to hashnode formatting)
return {
account: {
sessionAddress: sessionAddress,
adminAddress: ADMIN_ADDRESS,
accountAddress: predictedAddress,
},
aggregatedAssetId: selectedAsset,
amount,
recipientAccountId,
};
}, [
selectedAsset,
amount,
recipientAddress,
selectedChain,
predictedAddress,
sessionAddress,
]);
const { quote, isLoading: isLoadingQuote } = useTransferQuote(quoteRequest);
return { ...}
5. Executing the Quote
We need to execute the quote received so let’s create the action to make the API request.
// app/actions/executeQuote.ts
'use server'
const API_BASE_URL = process.env.ONEBALANCE_BASE_URL;
const API_KEY = process.env.ONEBALANCE_API_KEY;
import { Quote } from '@/types/quote';
export async function executeQuote(quote: Quote) {
const response = await fetch(`${API_BASE_URL}/api/quotes/execute-quote`, {
method: 'POST',
headers: {
'x-api-key': API_KEY!,
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: JSON.stringify(quote),
});
if (!response.ok) {
const error = await response.json();
throw new Error(error.message || 'Failed to execute quote');
}
return response.json();
}
6. Signing the Transaction with Embedded Wallet
Once we get the quote back, we have to deal with executing the quote which requires signing the transaction user operations from your Session Address which in our case is Privy’s Embedded wallet.
We have a specific field in our quote response of chain operation named- typedDataToSign
to sign from our embedded wallet.
Gas is too abstracted with Onebalance so you just need to sign the transaction which is the only part you need to pay attention.
This blurb of code looks really complex but in a nutshell, it just signs the user Operations of ERC4337 Onebalance Account from our Privy wallet.
import { Address, createWalletClient, custom, Hash } from 'viem';
import type { Quote, ChainOperation } from '@/types/quote';
const signTypedDataWithPrivy = (embeddedWallet: ConnectedWallet) => async (typedData: any): Promise<Hash> => {
const provider = await embeddedWallet.getEthereumProvider();
const walletClient = createWalletClient({
transport: custom(provider),
account: embeddedWallet.address as Address,
});
return walletClient.signTypedData(typedData);
};
const signOperation = (embeddedWallet: ConnectedWallet) => async (operation: ChainOperation): Promise<ChainOperation> => {
const signature = await signTypedDataWithPrivy(embeddedWallet)(operation.typedDataToSign);
return {
...operation,
userOp: { ...operation.userOp, signature },
};
};
export const signQuote = async (quote: Quote, embeddedWallet: ConnectedWallet) => {
const signWithEmbeddedWallet = signOperation(embeddedWallet);
const signedQuote = {
...quote,
};
signedQuote.originChainsOperations = await Promise.all(
quote.originChainsOperations.map(signWithEmbeddedWallet)
);
if (quote.destinationChainOperation) {
signedQuote.destinationChainOperation = await signWithEmbeddedWallet(
quote.destinationChainOperation
);
}
return signedQuote;
};
7. Create the Execute Hook
At last, we create the hook which checks if wallets are present and connected to first sign the quote and then execute them.
// /hooks/useExecuteQuote.ts
import { useMutation } from '@tanstack/react-query';
import { useWallets, ConnectedWallet } from '@privy-io/react-auth';
import { Address, createWalletClient, custom, Hash } from 'viem';
import { executeQuote } from '@/app/actions/executeQuote';
import type { Quote, ChainOperation } from '@/types/quote';
import { signQuote} from '@/helper'
export function useExecuteQuote() {
const { wallets } = useWallets();
return useMutation({
mutationFn: async (quote: Quote) => {
const privyWallet = wallets.find(wallet => wallet.walletClientType === 'privy');
if (!privyWallet) {
throw new Error('Privy wallet not found');
}
const signedQuote = await signQuote(quote, privyWallet);
return executeQuote(signedQuote);
},
});
}
8. Using the Execute Hook
Let’s see how we can use this hook in action.
// components/Transfer.tsx
...
import { useExecuteQuote } from "@/hooks/useExecuteQuote";
...
export function Transfer() {
const executeQuoteMutation = useExecuteQuote();
// React States to store the receipient transfer data from any input component
const [executedQuoteId, setExecutedQuoteId] = useState<string | null>(null);
const { quote, isLoading: isLoadingQuote } = useTransferQuote(quoteRequest);
const handleExecuteTransfer = async () => {
if (!quote) return;
try {
// Get execution details which includes chain operations
executeQuoteMutation.mutate(quote);
if (quote.id) {
console.log("Setting executed quote ID:", quote.id);
setExecutedQuoteId(quote.id);
} else {
console.error("No quoteId in execution result");
throw new Error("Failed to get quote ID from execution");
}
} catch (error) {
setExecutionError(
error instanceof Error
? error.message
: "Failed to get execution details"
);
} finally {
setIsLoadingExecution(false);
}
};
Now that’s how we transfer our tokens on Onebalance Account across any chain.
Step 7 - Fetch the Status of our Quote
Once we execute our quote, we receive a Quote ID that can be queried to check the transaction status across both chains.
First, we'll create an action to fetch the transaction status from the OneBalance API.
// /app/actions/transactionDetails.ts 'use server' import type { TransactionStatus } from '@/types/transaction'; const API_BASE_URL = process.env.ONEBALANCE_BASE_URL; const API_KEY = process.env.ONEBALANCE_API_KEY; export async function getExecutionDetails(quoteId: string): Promise<TransactionStatus> { const response = await fetch(`${API_BASE_URL}/api/status/get-execution-status?quoteId=${quoteId}`, { method: 'GET', headers: { 'x-api-key': API_KEY!, 'Accept': 'application/json' }, }); if (!response.ok) { const error = await response.json(); throw new Error(error.message || 'Failed to get execution details'); } return response.json(); }
Next, we create a React Query hook to fetch and track the status of our transaction.
// /hooks/useTransactionStatus.ts import { useQuery } from "@tanstack/react-query"; import { getExecutionDetails } from "@/app/actions/transactionDetails"; import { TransactionStatus } from "@/types/transaction"; export function useTransactionStatus({ quoteId }: { quoteId?: string }) { console.log('useTransactionStatus hook called with quoteId:', quoteId); return useQuery<TransactionStatus | undefined>({ queryKey: ['transactionStatus', quoteId], queryFn: async () => { if (!quoteId) throw new Error('Quote ID is required'); console.log('Fetching transaction status for quoteId:', quoteId); const result = await getExecutionDetails(quoteId); console.log('Transaction status result:', result); return result; }, enabled: !!quoteId, refetchInterval: (query) => { const data = query.state.data; if (!data) return false; const shouldRefetch = data.status === 'PENDING'; console.log('Should refetch?', shouldRefetch, 'Current status:', data.status); return shouldRefetch ? 5000 : false; }, }); }
Finally, we build a simple UI component to display the transaction status, including:
Chain operation status
Origin chain operation details
Destination chain operation details
// /components/TransactionStatus.tsx
import { useTransactionStatus } from "@/hooks/useTransactionStatus";
export const TransactionStatus = ({
quoteId,
onReset,
}: {
quoteId?: string;
onReset: () => void;
}) => {
console.log('TransactionStatus component rendered with quoteId:', quoteId);
const { data, isLoading, error } = useTransactionStatus({
quoteId,
});
console.log('Transaction status data:', data);
console.log('Transaction status loading:', isLoading);
console.log('Transaction status error:', error);
if (!quoteId) {
console.log('No quoteId provided');
return <p>No transaction ID available</p>;
}
if (isLoading) {
return <p className="animate-pulse">Fetching transaction status...</p>;
}
if (error) {
console.error('Transaction status error:', error);
return <p className="text-red-500">Error fetching transaction status: {error.message}</p>;
}
if (!data) {
return <p>No transaction data available</p>;
}
return (
<div className="mt-4 p-4 rounded bg-gray-50">
<h2 className="text-xl font-medium mb-4">Transaction Status</h2>
<dl className="space-y-4">
<div className="flex gap-2">
<dt className="font-medium">Status:</dt>
<dd className={`
${data.status === 'COMPLETED' ? 'text-green-600' : ''}
${data.status === 'PENDING' ? 'text-yellow-600' : ''}
${data.status === 'FAILED' ? 'text-red-600' : ''}
`}>
{data.status}
</dd>
</div>
{data.originChainOperations.length > 0 && (
<div className="border-t pt-2">
<dt className="font-medium mb-2">Origin Chain Operations:</dt>
<dd>
<ul className="space-y-2">
{data.originChainOperations.map((operation) => (
<li key={operation.hash} className="text-sm">
<a
href={operation.explorerUrl}
target="_blank"
rel="noopener noreferrer"
className="text-blue-500 hover:text-blue-600 break-all"
>
{operation.hash} (Chain {operation.chainId})
</a>
</li>
))}
</ul>
</dd>
</div>
)}
{data.destinationChainOperations.length > 0 && (
<div className="border-t pt-2">
<dt className="font-medium mb-2">Destination Chain Operations:</dt>
<dd>
<ul className="space-y-2">
{data.destinationChainOperations.map((operation) => (
<li key={operation.hash} className="text-sm">
<a
href={operation.explorerUrl}
target="_blank"
rel="noopener noreferrer"
className="text-blue-500 hover:text-blue-600 break-all"
>
{operation.hash} (Chain {operation.chainId})
</a>
</li>
))}
</ul>
</dd>
</div>
)}
</dl>
<button
onClick={onReset}
className="mt-4 px-6 py-2 bg-blue-500 text-white rounded hover:bg-blue-600 transition-colors"
>
Back to swap
</button>
</div>
);
};
We can integrate this and show this component when our execution is a success.
... import { useExecuteQuote } from "@/hooks/useExecuteQuote"; import { TransactionStatus } from "./TransactionStatus"; export function Transfer() { const executeQuoteMutation = useExecuteQuote(); const [executedQuoteId, setExecutedQuoteId] = useState<string | null>(null); ... return ( ... {/* Success Message */} {executeQuoteMutation.isSuccess && executedQuoteId && ( <TransactionStatus quoteId={executedQuoteId} onReset={() => { setExecutedQuoteId(null); executeQuoteMutation.reset(); }} /> )} {(executeQuoteMutation.isError || executionError) && ( <div className="mt-2 text-red-600"> Error:{" "} {executionError || executeQuoteMutation.error?.message || "Failed to execute transfer"} </div> )} ); }
Now, we can show our users the transaction hash on both Origin and Destination Chain.
Step 8 - Cross-Chain Swap of Funds
We have now reached the main part of this tutorial: cross-chain swaps. This process is quite similar to a standard transfer.
To perform a swap, simply select the two assets you wish to exchange. You don’t need to worry about which chain they are on, as all assets are aggregated.
For example, swapping 0.1 ETH → USD may result in different outcomes depending on the routing:
ETH on Base → USD on Base
ETH on Base → USD on Arbitrum
ETH on Avalanche → USD.e on Arbitrum
Once we obtain a quote, we just need to sign the typedData
using our session wallet, and the transaction is executed.
Let’s now implement this functionality.
1. Fetching the Swap Quote
We'll start by creating an action to fetch the swap quote.
// app/actions/swapQuote.ts
'use server';
import type { SwapQuoteRequest, SwapQuoteResponse } from '@/types/quote';
const API_BASE_URL = process.env.ONEBALANCE_BASE_URL;
const API_KEY = process.env.ONEBALANCE_API_KEY;
export async function getSwapQuote(request: SwapQuoteRequest): Promise<SwapQuoteResponse> {
try {
console.log(request);
const response = await fetch(`${API_BASE_URL}/api/quotes/swap-quote`, {
method: 'POST',
headers: {
'x-api-key': API_KEY!,
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: JSON.stringify(request)
});
if (!response.ok) {
const error = await response.json();
console.error('Error in getSwapQuote:', error);
throw new Error(`Failed to fetch swap quote: ${response.status}`);
}
return response.json();
} catch (error) {
console.error('Error in getSwapQuote:', error);
throw error;
}
}
2. Creating a Hook for Fetching Swap Quotes
Next, we'll create a React hook that helps fetch the swap quote.
// /hooks/useSwapQuote.ts
import { useQuery } from '@tanstack/react-query';
import type { SwapQuoteRequest, SwapQuoteResponse } from '@/types/quote';
import { getSwapQuote } from '@/app/actions/swapQuote';
export function useSwapQuote(request: SwapQuoteRequest | null) {
const { data: quote, isLoading, error } = useQuery<SwapQuoteResponse>({
queryKey: ['swap-quote', request],
queryFn: async () => {
if (!request) {
throw new Error('Swap quote request is required');
}
return await getSwapQuote(request);
},
enabled: !!request,
});
return {
quote,
isLoading,
error,
};
}
3. Preparing the Swap Quote Request
We will now pass in the swap quote request, which requires the following details:
Session Address → The embedded wallet managing the OneBalance account, responsible for signing transactions.
Admin Address → If an admin is added, otherwise set to a zero address.
Account Address → The OneBalance account address.
FromAggregatedAssetId → The aggregated asset ID of the source token (retrieved using
useAsset
).Amount → The amount of tokens to swap.
ToAggregatedAssetId → The aggregated asset ID of the destination token (retrieved using
useAsset
).
The swap request is similar to a transfer, except that we specify the aggregated asset IDs for the source and destination instead of CAIP-19 standard.
// components/Swap.tsx
import React, { useState, useMemo } from "react";
import { useAsset } from "@/hooks/useAsset";
import { useAccount } from "@/hooks/useAccount";
import { useSwapQuote } from "@/hooks/useSwapQuote";
import type { SwapQuoteRequest } from "@/types/quote";
import { ADMIN_ADDRESS } from "@/constants";
export function Swap() {
const { assets, isLoading: isLoadingAssets } = useAsset();
const { sessionAddress, predictedAddress } = useAccount();
const [fromAsset, setFromAsset] = useState("");
const [toAsset, setToAsset] = useState("");
const [amount, setAmount] = useState("");
// Prepare swap quote request
const quoteRequest = useMemo<SwapQuoteRequest | null>(() => {
if (
!fromAsset ||
!BigInt(amount) ||
!toAsset ||
!predictedAddress ||
!sessionAddress
) {
return null;
}
return {
account: {
sessionAddress: sessionAddress,
adminAddress: ADMIN_ADDRESS,
accountAddress: predictedAddress,
},
fromTokenAmount: amount,
fromAggregatedAssetId: fromAsset,
toAggregatedAssetId: toAsset,
};
}, [fromAsset, toAsset, amount, predictedAddress, sessionAddress]);
const { quote, isLoading: isLoadingQuote } = useSwapQuote(quoteRequest);
...
4. Executing the Swap
Once we receive the quote, we need to execute it by signing the user operations using our session wallet.
It is the same one we used in the Transfer.
Let’s see how we can use this hook in action.
// /components/Swap.tsx
import { useExecuteQuote } from "@/hooks/useExecuteQuote";
...
export function Swap() {
...
const executeQuoteMutation = useExecuteQuote();
const [executedQuoteId, setExecutedQuoteId] = useState<string | null>(null);
const { quote, isLoading: isLoadingQuote } = useSwapQuote(quoteRequest);
const handleExecuteSwap = async () => {
if (!quote) return;
try {
const result = await executeQuoteMutation.mutateAsync(quote);
if (quote.id) {
setExecutedQuoteId(quote.id);
} else {
console.error("No quoteId in execution result");
throw new Error("Failed to get quote ID from execution");
}
} catch (error) {
console.error("Swap execution error:", error);
}
};
return (...)
}
That's it! We've successfully implemented a cross-chain swap using OneBalance. With this setup, users can seamlessly swap tokens across any chain without worrying about underlying network complexities.
Conclusion
🎉 Congrats, it’s time to touch the grass! 🎉 You’ve just learned to integrate Onebalance into your Chain Agnostic DApp.
To recap, we went through:
✅ Setting up Onebalance
✅ Handling transfers
✅ Executing cross-chain swaps
Now, you're all set to build seamless cross-chain experiences!
Additional Resources:
🔹 Demo: OneWallet
🔹 GitHub Repo: OneWallet
🔹 Onebalance Privy Docs: Getting Started with Onebalance & Privy
🔹 Onebalance Swagger API: API Docs
Remember that BUIDL SHOULD NOT STOP 🏗️.
Subscribe to my newsletter
Read articles from Harpreet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
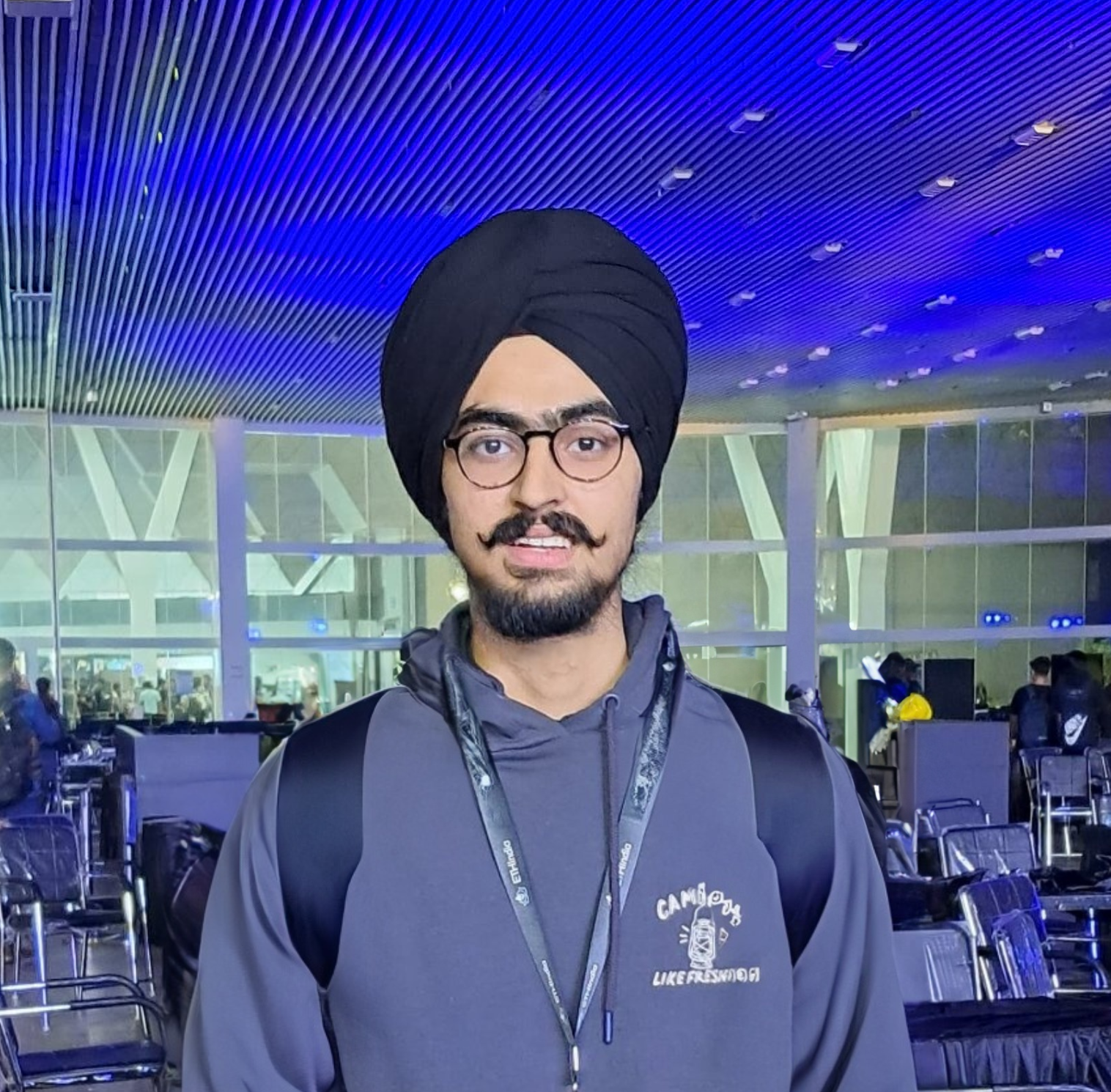
Harpreet Singh
Harpreet Singh
I am a Full Stack Web3 Developer and DevRel enthusiast. Arbitrum Ambassador, Capx Club Captain, Graph Advocate DeveloperDAO Member Scholar at Ora Protocol Arweave India Cohort #1 DevRel University Cohort#4 DXMentorship Mentee