Building a High-Performance Social Media Intelligence Feed: Lessons from the Trenches
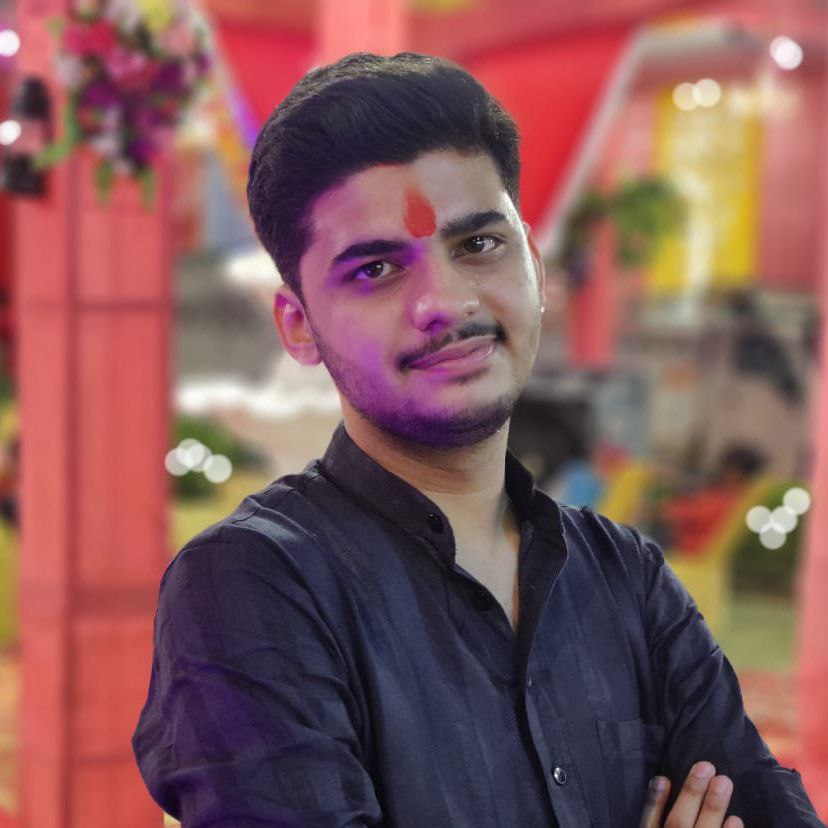
Table of contents
- The Challenge: Building a Cross-Platform Social Feed
- Problem 1: Laggy Navigation and Browser Overwhelm
- Problem 2: Feed Rendering Performance
- Problem 3: Infinite Scrolling Done Right
- Problem 4: Dynamic Heights for Various Media
- The Data Management Strategy: TanStack Query
- Persisting User Experience with Query Parameters
- Key Takeaways
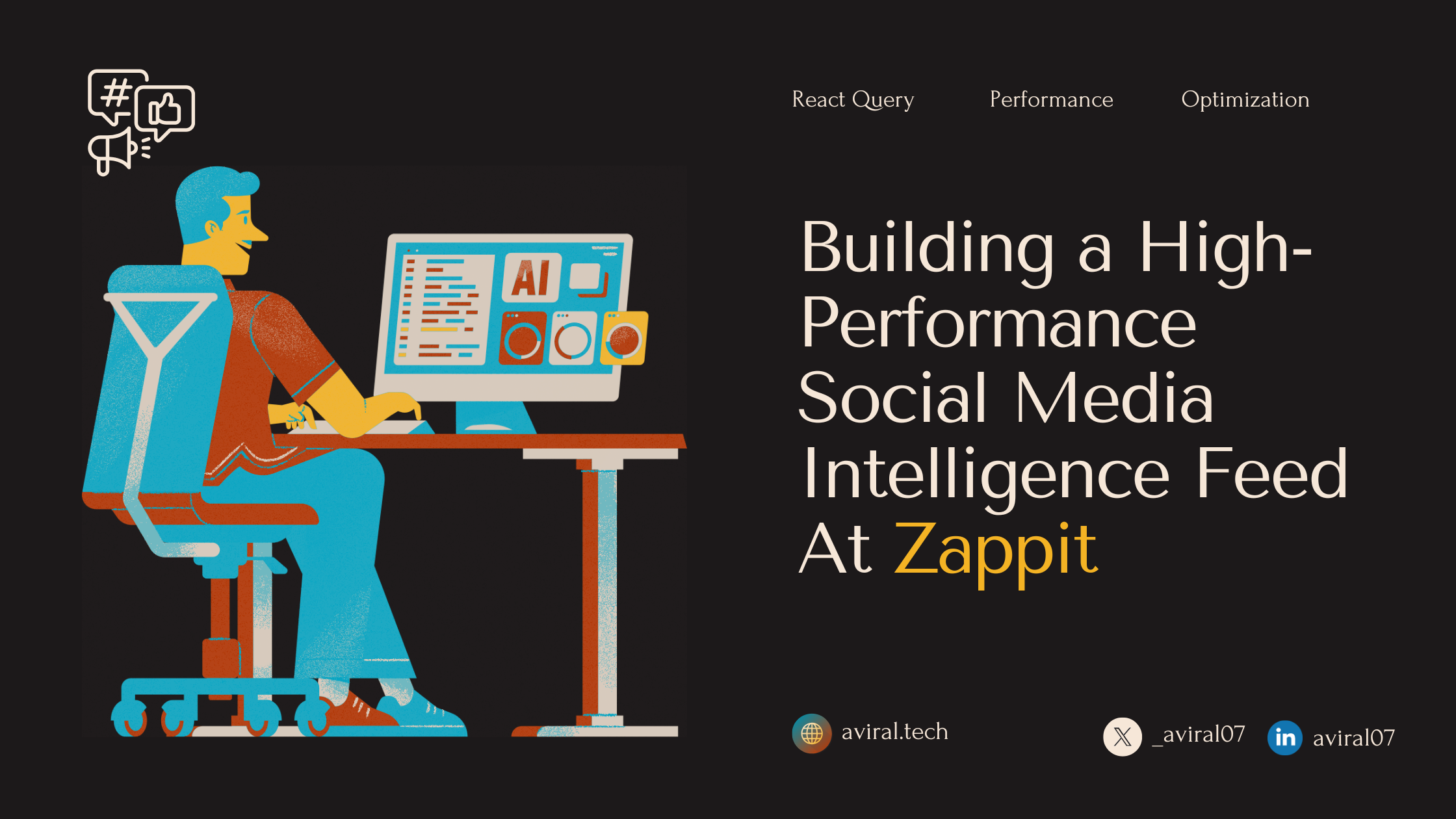
Building a social media feed seems straightforward—until you actually build one. As a Frontend Developer at Zappit.ai, I recently completed work on our Social Media Intelligence feed that shows users posts from their competitors and aspirational brands across multiple platforms (Instagram, Facebook, LinkedIn, Twitter, and Facebook Ads). What initially seemed like a simple UI challenge quickly evolved into a complex performance optimization exercise.
In this blog, I'll walk through how we solved critical performance challenges to build a buttery-smooth social media intelligence feed that handles diverse content formats without sacrificing user experience.
The Challenge: Building a Cross-Platform Social Feed
Our "Competitor Pulse" feed needed to:
Display posts from multiple social platforms in a unified interface
Support infinite scrolling with potentially hundreds of posts
Handle various media formats (images, videos, carousels)
Provide filtering by competitors and platforms
Offer both grid and list views
Maintain performance despite loading heavy media assets
The real challenge wasn't the UI design—it was making it perform well with potentially hundreds of posts, each containing media assets.
Problem 1: Laggy Navigation and Browser Overwhelm
Our initial implementation fetched posts and rendered media as you'd expect. But as users scrolled down the feed and dozens of videos started loading, navigation between routes became painfully slow—with delays of 1-1.2 seconds when switching between pages.
The Diagnosis
We discovered the browser was struggling with multiple concurrent fetch requests for media assets. Even after navigating away from the feed, these requests continued in the background, causing significant lag.
The Solution: Abort Controller
We implemented an AbortController pattern to cancel all pending media requests when a user navigates away from the feed:
let currentPageController = new AbortController();
const interruptedResources = new Map();
export const abortAllMediaNetworkRequests = () => {
console.log("Aborting all pending network requests");
// Abort any fetch requests
currentPageController.abort("Navigation occurred");
currentPageController = new AbortController();
// For images - track interrupted loads
const pendingImages = document.querySelectorAll("img:not([complete])");
pendingImages.forEach((img) => {
const imageElement = img as HTMLImageElement;
if (!imageElement.complete) {
// Store original URL and loading progress
interruptedResources.set((img as HTMLImageElement).src, {
type: "image",
elementId: img.id || null,
className: img.className,
timestamp: Date.now(),
});
// Stop the loading by replacing with tiny blank GIF
imageElement.src =
"data:image/gif;base64,R0lGODlhAQABAAAAACH5BAEKAAEALAAAAAABAAEAAAICTAEAOw==";
}
});
// Handle videos
const videos = document.querySelectorAll("video");
videos.forEach((video) => {
if (video.networkState === 2) { // NETWORK_LOADING
interruptedResources.set(video.src, {
type: "video",
elementId: video.id || null,
className: video.className,
currentTime: video.currentTime,
timestamp: Date.now(),
});
video.pause();
video.src = "";
video.load();
}
});
}
This function runs during navigation events and:
Aborts any in-progress fetch requests
Interrupts loading images by replacing their source
Stops and unloads any loading videos
Even handles SVG elements and their resources
The results were dramatic—navigation speed improved from 1-1.2 seconds back to near-instant responses.
Problem 2: Feed Rendering Performance
As users scrolled deeper into the feed, performance degraded significantly due to:
Too many DOM nodes in the document
Too many concurrent media downloads
Event handlers on many elements
The Solution: Lazy Loading + React Suspense
I implemented a two-pronged approach:
- Lazy-load components to reduce initial JavaScript payload:
// Instead of direct imports:
// import PostComponent from './PostComponent';
// We used lazy loading:
const PostComponent = lazy(() => import('./PostComponent'));
// And rendered with Suspense:
<Suspense fallback={<PostSkeleton />}>
<PostComponent
post={postData}
/>
</Suspense>
- Implement proper media lazy-loading to prevent downloading videos until needed:
// Video component with lazy loading
const VideoPlayer = ({ videoUrl }) => {
const videoRef = useRef(null);
const [isVisible, setIsVisible] = useState(false);
// Using Intersection Observer to detect when video is visible
useEffect(() => {
const observer = new IntersectionObserver(
([entry]) => {
setIsVisible(entry.isIntersecting);
},
{ threshold: 0.1 }
);
if (videoRef.current) {
observer.observe(videoRef.current);
}
return () => {
if (videoRef.current) {
observer.unobserve(videoRef.current);
}
};
}, []);
return (
<div ref={videoRef}>
{isVisible && (
<video
controls
preload="metadata"
src={isVisible ? videoUrl : undefined}
/>
)}
</div>
);
};
The impact was dramatic: videos would only start loading when they entered the viewport, making scrolling through the feed substantially smoother.
Problem 3: Infinite Scrolling Done Right
Implementing infinite scrolling is simple in theory but challenging to get right in practice. Common pitfalls include:
Triggering loads too early or too late
Making too many API calls if the user scrolls quickly
Maintaining scroll position when new content loads
The Solution: IntersectionObserver + Debouncing
I combined the IntersectionObserver API with a debounced load function to prevent API hammering:
// Custom hook for intersection observer
const [isIntersecting, ref] = useIntersectionObserver<HTMLDivElement>({
threshold: 0.1,
rootMargin: "300px 0px", // Load more before user reaches bottom
});
// Debounced load function implementation
const debouncedLoadMore = useCallback(
debounce(() => {
if (hasMore && !loadMoreQuery.isLoading && !isLoadingFeed) {
loadMoreQuery.refetch();
}
}, 500),
[hasMore, loadMoreQuery.isLoading, isLoadingFeed, lastTimestamps]
);
// Using the hook to trigger more loads
useEffect(() => {
if (isIntersecting) {
debouncedLoadMore();
}
// Cleanup
return () => {
(debouncedLoadMore as any).cancel();
};
}, [isIntersecting, debouncedLoadMore]);
This approach ensures:
We only load new content when the user is approaching the end of the current content
We don't make redundant API calls if the user scrolls quickly
We maintain a smooth scrolling experience without "hiccups"
Problem 4: Dynamic Heights for Various Media
Social media content comes in all shapes and sizes. Managing layout shifts caused by dynamically loaded images and videos was crucial for a polished UX.
The Solution: Width + Aspect Ratio
Rather than waiting for media to load to calculate heights, we leveraged aspect ratios:
const DynamicHeightImage = ({ src, aspectRatio, width }) => {
// Calculate height based on width and aspect ratio
// aspectRatio = width / height, so height = width / aspectRatio
const height = width && aspectRatio ? Math.floor(width / aspectRatio) : null;
return (
<img
src={src}
className="w-full object-cover"
style={{ height: height ? `${height}px` : 'auto' }}
loading="lazy"
alt="Post content"
/>
);
};
// Usage example
const PostMedia = ({ media, containerWidth }) => {
// Get media details
const { url, aspectRatio } = media;
return (
<div className="post-media-container">
<DynamicHeightImage
src={url}
aspectRatio={aspectRatio}
width={containerWidth}
/>
</div>
);
};
This technique:
Takes the image source, aspect ratio, and container width as props
Calculates the appropriate height by dividing width by aspect ratio
Sets a fixed height in pixels based on the calculation.
Maintains proper media proportions while fitting into feed layout
The Data Management Strategy: TanStack Query
To tie everything together, we needed a robust data management solution. TanStack Query (formerly React Query) was our choice for several reasons:
Built-in caching: Prevents redundant API calls for data we've already fetched
Background refetching: Keeps data fresh without interrupting the user experience
Pagination management: Simplified our infinite scrolling implementation
Loading state management: Provided clean hooks for handling loading states
Our simplified query setup:
// Query for initial feed data
const {
data: feedData,
isLoading: isLoadingFeed,
refetch: refetchFeed,
} = useQuery({
queryKey: getFeedQueryKey(),
queryFn: async () => {
const response = await getInitialSocialFeed(
uniqueId,
getFeedFilters() // Feed Filters to render specfic Posts
);
if (response?.data?.success) {
setLastTimestamps(response.data.data.lastTimestamps);
setHasMore(response.data.data.posts.length > 0);
}
return response.data;
},
enabled: true,
staleTime: 5 * 60 * 1000, // 5 minutes
});
Persisting User Experience with Query Parameters
Another important aspect was maintaining the user's selected filters when they refresh the page or navigate and return. We leveraged URL query parameters to preserve state:
function getTabFromParams(params: URLSearchParams): FEED_TAB {
// Check for tab parameter and return appropriate value
const tabParam = params?.get("postType");
if (tabParam === FEED_TAB.ADS) return FEED_TAB.ADS;
if (tabParam === FEED_TAB.TRENDING) return FEED_TAB.TRENDING;
return FEED_TAB.ORGANIC; // Default
}
// Use the parameters to set initial state
const params = useSearchParams();
const initialTab = getTabFromParams(params!);
const initialPlatforms = initialTab === FEED_TAB.ADS ? ["Ads"] : [];
// Then set state accordingly
const [selectedTab, setSelectedTab] = useState<FEED_TAB>(initialTab);
const [selectedPlatforms, setSelectedPlatforms] = useState<string[]>(initialPlatforms);
Key Takeaways
Building a high-performance social media intelligence feed taught me several valuable lessons:
Mind your media: Lazy load everything, but especially videos
Clean up after yourself: Always abort pending requests when components unmount
Respect the viewport: Only load what's visible or about to be visible
Debounce liberally: User actions like scrolling need to be throttled
Preserve dimensions: Use aspect ratios to maintain layout stability
Cache wisely: Use TanStack Query to avoid redundant API calls
These optimizations transformed our feed from a performance liability into a smooth, native-feeling experience that users can scroll through for hours without performance degradation.
The most important lesson? Performance isn't something you bolt on at the end—it needs to be part of your implementation strategy from day one.
Subscribe to my newsletter
Read articles from Aviral Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
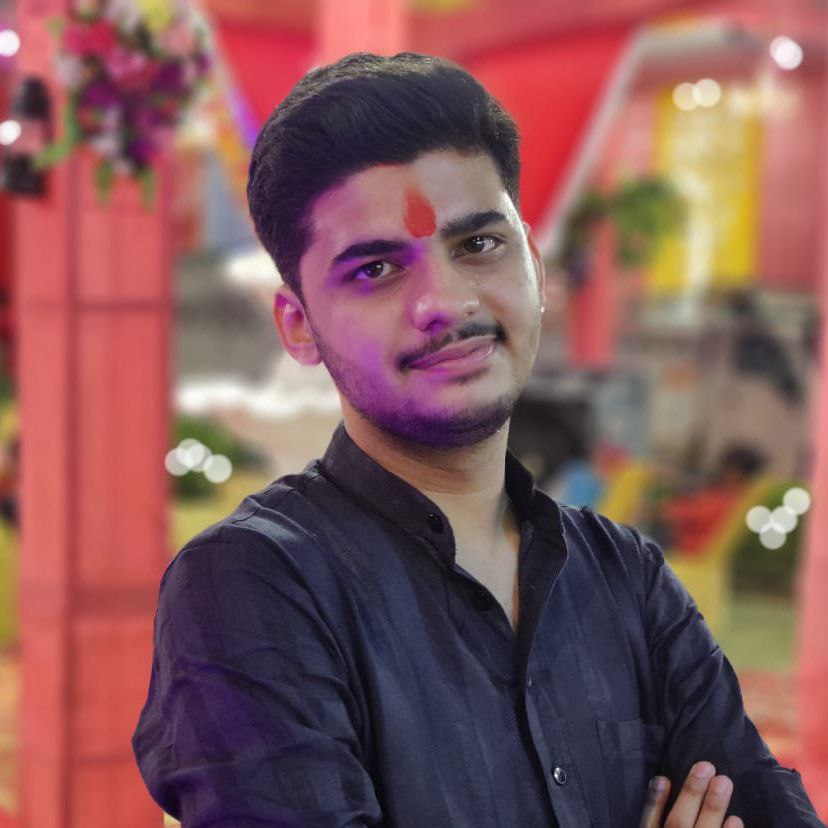
Aviral Sharma
Aviral Sharma
Driven 3rd year BTech (IT) student passionate about front-end development. Eager to leverage skills in React, Redux, JavaScript, Next.js, and Tailwind CSS to contribute to a dynamic team.