[ ] Bracket notation in JavaScript

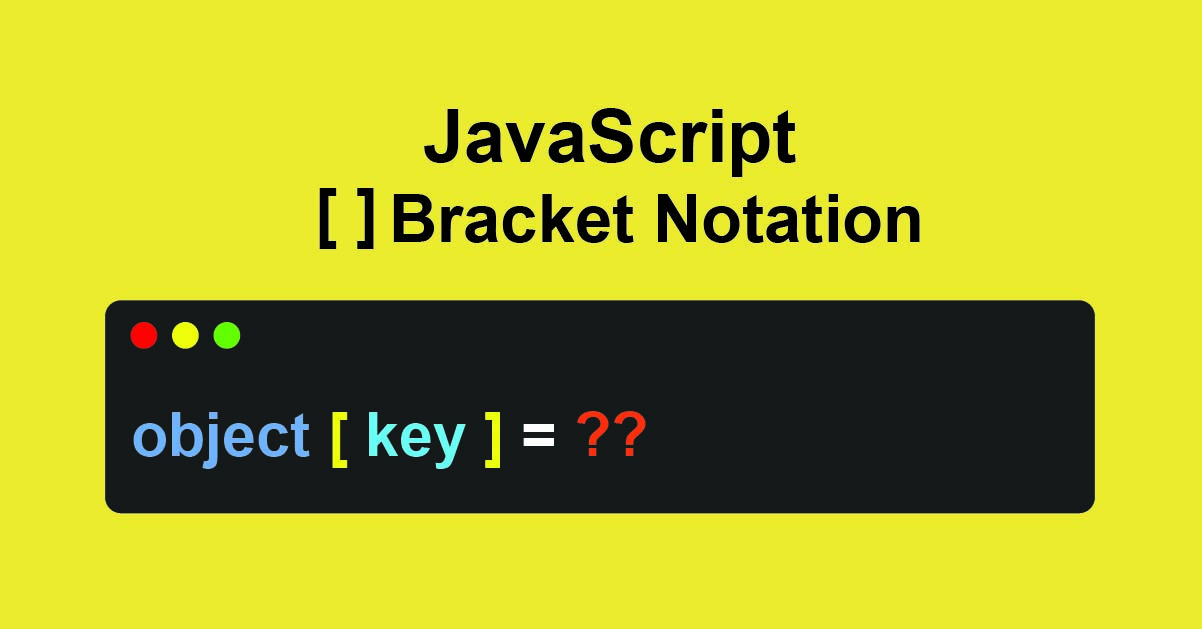
Understanding Bracket Notation in JavaScript
In JavaScript, bracket notation is a powerful way to access and manipulate object properties dynamically. While dot notation is more common, bracket notation provides flexibility, especially when dealing with dynamic keys or special characters.
Why [ ] Bracket notation ?
In JavaScript objects sometimes, we need to access or manipulate object properties dynamically, meaning we don't know beforehand which property we need to access. Instead, we have the property name stored in a variable.
Problem example :
Consider a student object containing fname
, lname
, and age
. We want a function that retrieves any property value based on the key we pass.
const student = {
fname: "Alex",
lname: "Brown",
age: 28,
};
function getValue(key) {
// How do we access the property?
}
// Example usage:
const data = getValue("fname");
console.log(data);
Solution Using Bracket Notation:
Here if we use student.key
, JavaScript will look for a property named key
instead of using the value stored in key
. So, we need to convert the variable into a property reference using bracket notation:
So, for that we need to use [ ] Bracket notation as shown below.
const student = {
fname: "Alex",
lname: "Brown",
age: 28,
};
function getValue(key) {
return student[key];
}
const data = getValue("fname");
console.log(data); // Output: "Alex"
Here, student[key]
is equivalent to student["fname"]
, which is the same as student.fname
.
Use Cases for Bracket Notation:
Dynamic Property Access (as shown in the above example)
When the property name is unknown until runtime:
const key = "name"; console.log(person[key]); // Output: Alex
Property Names with Special Characters or Spaces
Some property names cannot be accessed with dot notation:
const obj = { "first-name": "Alex", "last name": "Brown" }; console.log(obj["first-name"]); // Output: Alex console.log(obj["last name"]); // Output: Brown
Numeric or Symbol Keys
Objects can have numeric keys, which must be accessed using bracket notation:
const scores = { 1: "Gold", 2: "Silver", 3: "Bronze" }; console.log(scores[1]); // Output: Gold
Using Bracket Notation with the Spread Operator
Bracket notation is useful when updating an object dynamically, such as handling form inputs:
let student = {
fname: "Alex",
lname: "Brown",
age: 28,
};
function handleUpdateData(event) {
student = {
...student,
[event.target.name]: event.target.value
};
}
// Example input elements
<input name="fname" type="text" />
<input name="lname" type="text" />
<input name="age" type="text" />
// Event listeners
const inputs = document.querySelectorAll("input");
inputs.forEach(input => input.addEventListener("change", handleUpdateData));
Here, the name
attribute of the input fields matches the keys in the student
object. When a user types in the input field, handleUpdateData
dynamically updates the corresponding property.
Note: name
attributes must have same names as their corresponding keys of the object.
Conclusion
Bracket notation in JavaScript is a crucial tool for working with objects dynamically. While dot notation is more readable and commonly used, bracket notation is indispensable when dealing with dynamic keys, special characters, or numeric properties. Mastering both notations will make your code more flexible and powerful.
Subscribe to my newsletter
Read articles from Vishal Pandit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishal Pandit
Vishal Pandit
I am a software developer