Day 17: Deep Dive into Linked Lists & More 🚀

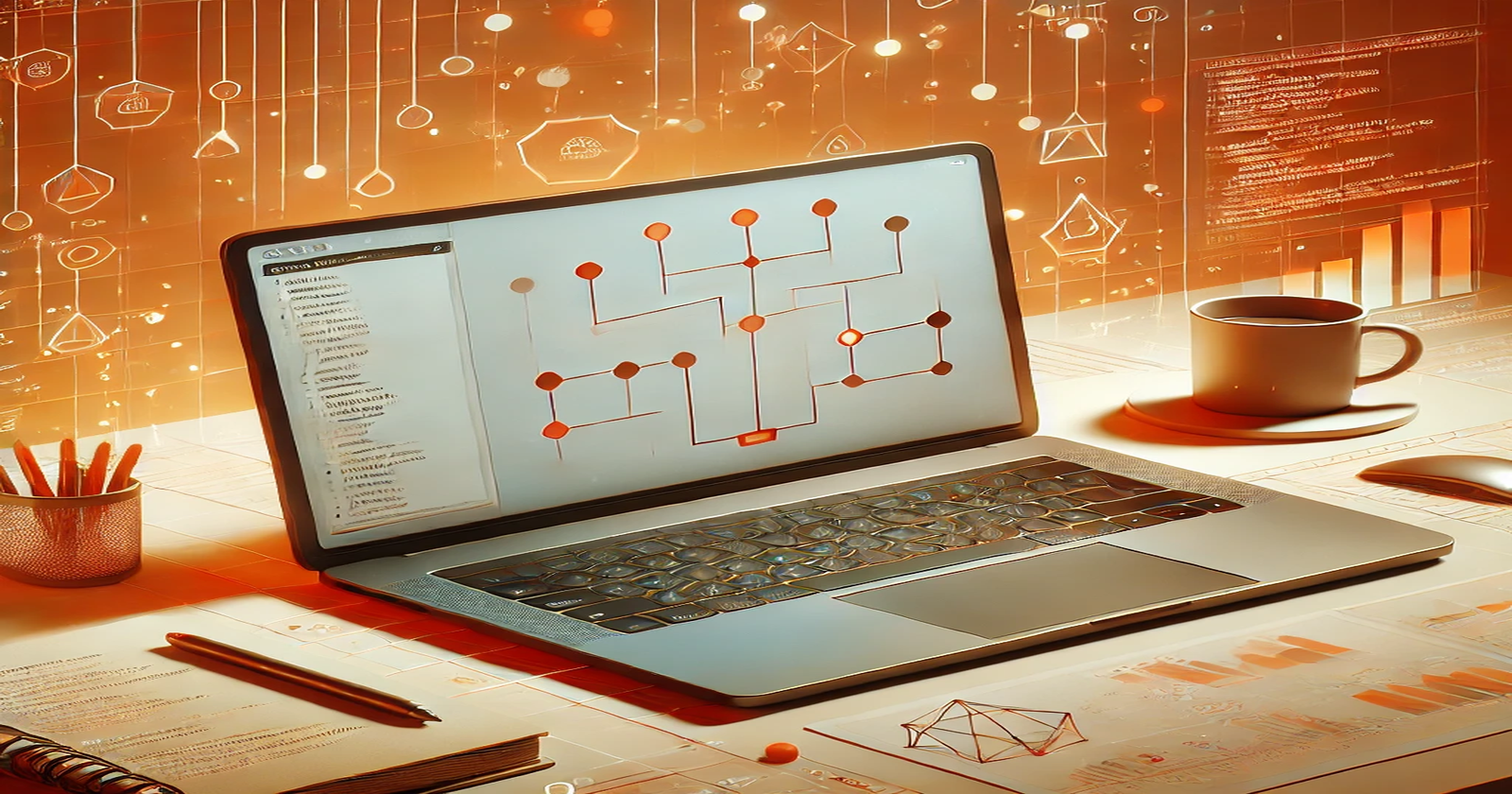
Problems Solved Today
Today's focus was Linked Lists, and I solved many interesting problems. While I was already familiar with the concepts, it had been a while since I last practiced them, so it felt like a fresh challenge.
List of Solved Problems & Key Learnings:
1️⃣ Reverse Linked List ✅ (Easy)
A classic problem that tests your understanding of pointer manipulation. The iterative approach using three pointers (prev, current, next) felt natural, while the recursive solution was an elegant alternative.
2️⃣ Merge Two Sorted Lists ✅ (Easy)
This is essentially the merge step of Merge Sort applied to linked lists. Used a dummy node and a two-pointer approach to efficiently merge the two lists.
3️⃣ Linked List Cycle ✅ (Easy)
Implemented Floyd’s Cycle Detection Algorithm (Tortoise & Hare), which efficiently detects a cycle using two pointers moving at different speeds. A fundamental technique in linked list problems!
4️⃣ Reorder List ✅ (Medium)
A tricky problem where I had to split the list into two halves, reverse the second half, and then merge them alternately. Required good observation skills and precise pointer manipulation.
5️⃣ Remove Nth Node From End of List ✅ (Medium)
Solved using two pointers where one pointer moves n
steps ahead, and then both move together until the first reaches the end. This helps efficiently find and delete the target node in one pass.
6️⃣ Copy List With Random Pointer ✅ (Medium)
One of the most interesting linked list problems! Used hash maps for an O(n) space solution, and later implemented the O(1) space trick by modifying the existing list structure.
7️⃣ Add Two Numbers ✅ (Medium)
Implemented digit-wise addition of two numbers represented as linked lists, similar to how we do manual addition. Handled carry propagation carefully while traversing the lists.
8️⃣ Find The Duplicate Number ✅ (Medium)
A mind-blowing problem that can be solved using linked list cycle detection techniques even though it's an array-based problem! Without watching NeetCode.io’s explanation, I wouldn’t have thought of using Floyd’s Algorithm here.
9️⃣ LRU Cache ✅ (Medium)
Implemented a Least Recently Used (LRU) Cache using a combination of a HashMap and Doubly Linked List to achieve O(1) time complexity for both get() and put() operations. A must-know problem for system design interviews!
🔟 Merge K Sorted Lists ✅ (Hard)
At first, this problem seemed daunting, but once I realized that it was just an extension of merging two sorted lists, was able to solve it pretty cleanly.
11️⃣ Reverse Nodes In K Group ✅ (Hard)
One of the hardest linked list problems I’ve done so far. Required me to recursively reverse K nodes at a time, carefully handling pointer connections. I still need to revisit this one to fully grasp the recursive approach.
Interesting Observations & Key Takeaways
1️⃣ Finding the Duplicate Number 🧐
This problem was mind-blowing. I never imagined that a linked list concept could be represented in an array! Without watching NeetCode.io's explanation, I wouldn’t have decoded why a linked list approach is even needed here. It was an eye-opener!
2️⃣ Two Pointers & Floyd’s Cycle Detection Algorithm 🔁
Most linked list problems involve two-pointer techniques, and I noticed that Floyd's algorithm is a recurring pattern in cycle-related problems. This was a great reinforcement of a classic technique.
3️⃣ LeetCode Difficulty Ratings 🤔
I still don't fully understand how LeetCode assigns difficulty levels.
Merge K Sorted Lists (Hard) → Once I understood the trick, it wasn’t too bad.
Reverse Nodes in K Group (Hard) → This one took me some time to grasp, and I still need to revisit it for a better understanding.
Would love to explore more about how these problems are categorized!
Areas for Improvement & Next Steps
✅ Solving problems is fun, but I need to change my approach. Currently, I rely a bit too much on YouTube solutions 😅.
📌 Going forward, I’ll push myself to solve problems with minimal help, as that’s the best way to test my true understanding and improve my problem-solving skills.
Beyond DSA: DevOps, PySpark & Node.js
Apart from DSA, I also made progress on:
🔹 PySpark – Started exploring how it works in Python.
🔹 DevOps – Still haven't fully started, but I made some progress in planning my approach.
🔹 Node.js Server – Made some improvements to the backend.
Overall, a great and productive day! 💪🔥
Time to keep up the momentum and tackle more challenges tomorrow! 🚀
Subscribe to my newsletter
Read articles from Tennis Kumar C directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
