Remember Everything: Building a Flashcard App with Spaced Repetition 🧠

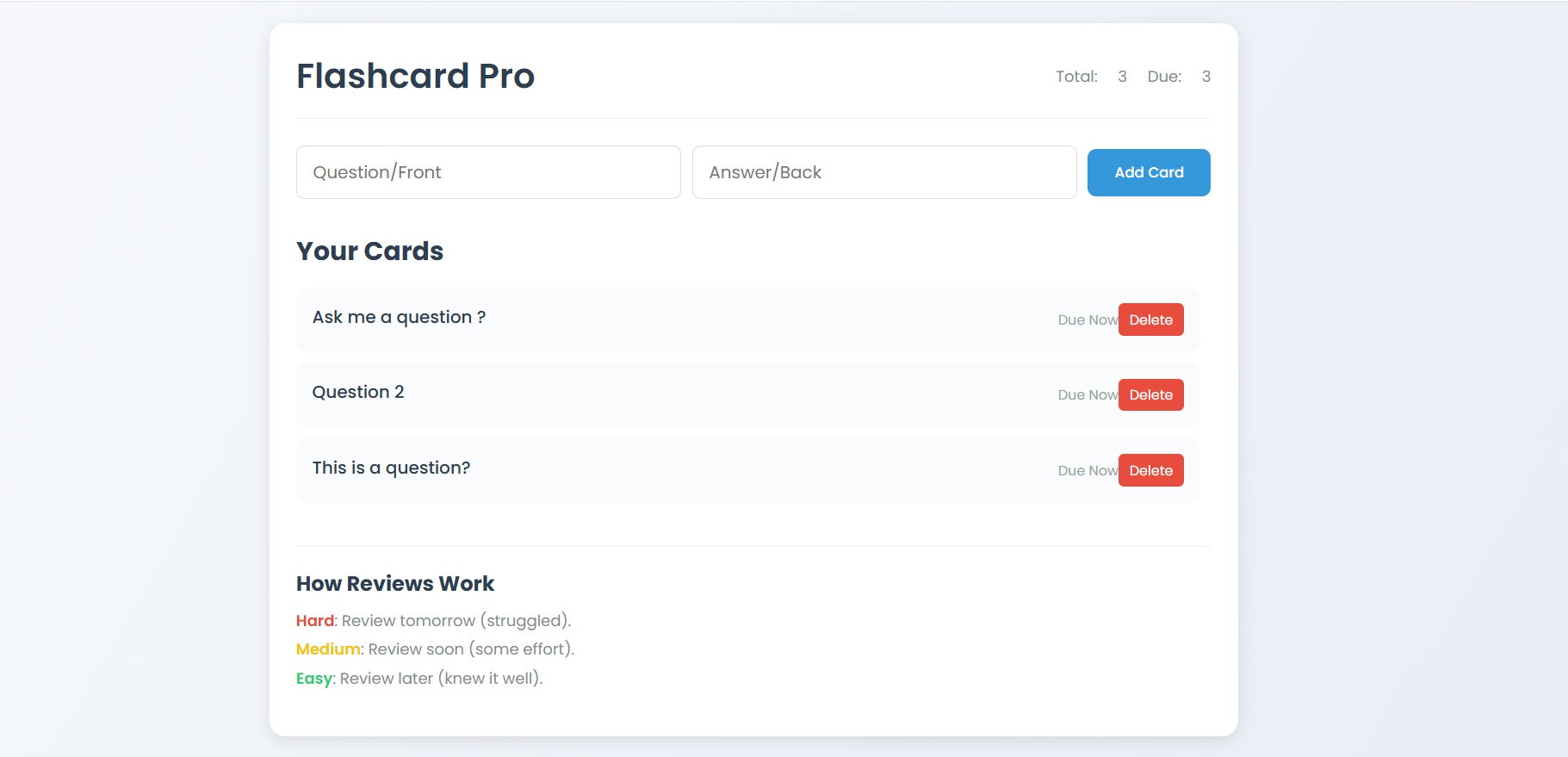
Have you ever spent hours memorizing information only to forget it days later? Whether you're studying for exams, learning a language, or mastering programming concepts, retaining information can be challenging. But what if your study tool could adapt to your learning patterns, showing you difficult concepts more frequently and easy ones less often?
Today, we'll build exactly that: a smart flashcard application that uses spaced repetition—a learning technique that optimizes memory retention by strategically scheduling reviews. Let's turn forgetting into remembering! ✨
What is Spaced Repetition? 🕒
Spaced repetition is a learning technique that uses increasing time intervals between reviews of previously learned material. It's based on the psychological spacing effect, which shows that we learn more effectively when we space out our learning over time rather than cramming.
Here's how it works:
Concepts you find difficult appear more frequently
Concepts you know well appear less frequently
The system adapts to your personal memory patterns
This approach is scientifically proven to enhance long-term retention and make your study time more efficient. Rather than reviewing everything equally, you focus more on what you don't know well. Smart, right? 🤓
You can try the live demo here: Flashcard Pro
Our Application: Flashcard Pro 📚
Our application, "Flashcard Pro," allows users to:
Create custom flashcards with questions and answers
Review cards using the spaced repetition algorithm
Track their progress with simple statistics
Store all data locally in the browser
Let's break down how we built this application step by step!
Building the Structure: HTML 🏗️
First, let's understand the basic structure of our application:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flashcard Pro</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<header>
<h1>Flashcard Pro</h1>
<div class="stats">
<span>Total: <span id="total-cards">0</span></span>
<span>Due: <span id="due-cards">0</span></span>
</div>
</header>
<div class="create-section">
<div class="input-group">
<input type="text" id="front" placeholder="Question/Front">
<input type="text" id="back" placeholder="Answer/Back">
<button id="add-card">Add Card</button>
</div>
</div>
<div class="cards-section">
<h2>Your Cards</h2>
<div class="cards-list" id="cards-list">
<!-- Cards will be dynamically inserted here -->
</div>
<div class="review-controls" id="review-controls" style="display: none;">
<button class="review-btn" data-difficulty="1">Hard</button>
<button class="review-btn" data-difficulty="2">Medium</button>
<button class="review-btn" data-difficulty="4">Easy</button>
</div>
</div>
<div class="explanation-section">
<h3>How Reviews Work</h3>
<p><span class="hard-text">Hard</span>: Review tomorrow (struggled).</p>
<p><span class="medium-text">Medium</span>: Review soon (some effort).</p>
<p><span class="easy-text">Easy</span>: Review later (knew it well).</p>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Our HTML creates several key sections:
A header with the app title and statistics about total cards and due cards
A creation section with inputs for the front and back of new flashcards
A cards section where all flashcards are displayed and can be reviewed
An explanation section that clarifies how the review system works
The structure is clean and straightforward, which makes it easy for users to navigate and interact with the application.
Styling Our App: CSS 🎨
Next, let's make our app visually appealing and user-friendly with CSS:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body {
background: linear-gradient(120deg, #f6f8fc, #e9eef5);
min-height: 100vh;
padding: 20px;
display: flex;
justify-content: center;
}
.container {
background: white;
border-radius: 15px;
padding: 25px;
width: 100%;
max-width: 900px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
}
header {
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 25px;
padding-bottom: 15px;
border-bottom: 1px solid #eee;
}
h1 {
color: #2c3e50;
font-size: 2rem;
font-weight: 600;
}
.stats span {
margin-left: 15px;
color: #7f8c8d;
font-size: 0.9rem;
}
.create-section {
margin-bottom: 30px;
}
.input-group {
display: flex;
gap: 10px;
align-items: center;
}
input {
flex: 1;
padding: 12px 15px;
border: 1px solid #ddd;
border-radius: 8px;
font-size: 1rem;
transition: border-color 0.3s ease;
}
input:focus {
outline: none;
border-color: #3498db;
}
#add-card {
background: #3498db;
color: white;
border: none;
padding: 12px 25px;
border-radius: 8px;
cursor: pointer;
font-weight: 500;
transition: background 0.3s ease;
}
#add-card:hover {
background: #2980b9;
}
.cards-section h2 {
color: #2c3e50;
font-size: 1.5rem;
margin-bottom: 15px;
}
.cards-list {
max-height: 400px;
overflow-y: auto;
padding-right: 10px;
}
.card-item {
background: #f9fbfc;
border-radius: 10px;
padding: 15px;
margin-bottom: 10px;
display: flex;
justify-content: space-between;
align-items: center;
cursor: pointer;
transition: transform 0.2s ease, background 0.2s ease;
}
.card-item:hover {
transform: translateX(5px);
background: #f1f5f9;
}
.card-content {
flex: 1;
}
.card-front {
font-weight: 500;
color: #2c3e50;
margin-bottom: 5px;
}
.card-back {
color: #7f8c8d;
font-size: 0.9rem;
display: none;
}
.card-item.show-back .card-back {
display: block;
}
.card-status {
font-size: 0.8rem;
color: #95a5a6;
margin-left: 15px;
}
.delete-btn {
background: #e74c3c;
color: white;
border: none;
padding: 5px 10px;
border-radius: 5px;
cursor: pointer;
font-size: 0.8rem;
transition: background 0.3s ease;
}
.delete-btn:hover {
background: #c0392b;
}
.review-controls {
margin-top: 20px;
display: flex;
justify-content: center;
gap: 15px;
}
.review-btn {
padding: 10px 20px;
border: none;
border-radius: 8px;
color: white;
cursor: pointer;
font-weight: 500;
transition: transform 0.2s ease;
}
.review-btn[data-difficulty="1"] { background: #e74c3c; }
.review-btn[data-difficulty="2"] { background: #f1c40f; }
.review-btn[data-difficulty="4"] { background: #2ecc71; }
.review-btn:hover {
transform: translateY(-2px);
}
.explanation-section {
margin-top: 30px;
padding-top: 20px;
border-top: 1px solid #eee;
}
.explanation-section h3 {
color: #2c3e50;
font-size: 1.2rem;
margin-bottom: 10px;
}
.explanation-section p {
color: #7f8c8d;
font-size: 0.9rem;
margin-bottom: 5px;
}
.hard-text {
color: #e74c3c;
font-weight: 600;
}
.medium-text {
color: #f1c40f;
font-weight: 600;
}
.easy-text {
color: #2ecc71;
font-weight: 600;
}
Our CSS focuses on several key aspects:
Layout and Color Scheme
We've used a gentle blue gradient background with a clean white container to create a calm, distraction-free learning environment. The container has rounded corners and a subtle shadow for a modern, polished look.
Card Design
Each flashcard has a light background that changes slightly when hovered over, with a small animation that helps users identify interactive elements. When clicked, cards reveal their answer.
Review Controls
The review buttons are color-coded based on difficulty levels:
🔴 Hard (red): For concepts you struggled with
🟡 Medium (yellow): For concepts that required some effort
🟢 Easy (green): For concepts you knew well
These visual cues help users quickly choose the appropriate difficulty level during review.
The Brain of Our App: JavaScript ⚙️
Now for the most important part—the JavaScript that powers our spaced repetition algorithm and makes the app interactive:
class Flashcard {
constructor(front, back) {
this.front = front;
this.back = back;
this.interval = 1;
this.nextReview = new Date();
this.ease = 2.5;
}
}
class FlashcardApp {
constructor() {
this.cards = JSON.parse(localStorage.getItem('flashcards')) || [];
this.cards = this.cards.map(card => ({
...card,
nextReview: new Date(card.nextReview)
}));
this.selectedCard = null;
this.initElements();
this.bindEvents();
this.renderCards();
this.updateStats();
}
initElements() {
this.frontInput = document.getElementById('front');
this.backInput = document.getElementById('back');
this.addButton = document.getElementById('add-card');
this.cardsList = document.getElementById('cards-list');
this.totalCardsSpan = document.getElementById('total-cards');
this.dueCardsSpan = document.getElementById('due-cards');
this.reviewControls = document.getElementById('review-controls');
this.reviewButtons = document.querySelectorAll('.review-btn');
}
bindEvents() {
if (this.addButton) {
this.addButton.addEventListener('click', () => this.addCard());
} else {
console.error('Add Card button not found in the DOM');
}
this.reviewButtons.forEach(btn =>
btn.addEventListener('click', (e) => this.reviewCard(e.target.dataset.difficulty))
);
}
addCard() {
const front = this.frontInput.value.trim();
const back = this.backInput.value.trim();
if (front && back) {
const card = new Flashcard(front, back);
this.cards.push(card);
this.saveCards();
this.renderCards();
this.updateStats();
this.clearInputs();
} else {
console.log('Please enter both front and back text');
}
}
deleteCard(index) {
this.cards.splice(index, 1);
this.saveCards();
this.renderCards();
this.updateStats();
if (this.selectedCard) {
this.reviewControls.style.display = 'none';
this.selectedCard = null;
}
}
renderCards() {
this.cardsList.innerHTML = '';
const now = new Date();
this.cards.forEach((card, index) => {
const cardElement = document.createElement('div');
cardElement.className = 'card-item';
cardElement.innerHTML = `
<div class="card-content">
<div class="card-front">${card.front}</div>
<div class="card-back">${card.back}</div>
</div>
<div class="card-status">
${card.nextReview <= now ? 'Due Now' : `Due: ${card.nextReview.toLocaleDateString()}`}
</div>
<button class="delete-btn" data-index="${index}">Delete</button>
`;
cardElement.addEventListener('click', (e) => {
if (!e.target.classList.contains('delete-btn')) {
this.selectCard(card, cardElement);
}
});
const deleteBtn = cardElement.querySelector('.delete-btn');
deleteBtn.addEventListener('click', () => this.deleteCard(index));
this.cardsList.appendChild(cardElement);
});
}
selectCard(card, element) {
if (this.selectedCard === element) {
element.classList.toggle('show-back');
return;
}
if (this.selectedCard) {
this.selectedCard.classList.remove('show-back', 'selected');
}
this.selectedCard = element;
element.classList.add('show-back', 'selected');
this.reviewControls.style.display = 'flex';
}
reviewCard(difficulty) {
if (!this.selectedCard) return;
const index = Array.from(this.cardsList.children).indexOf(this.selectedCard);
const card = this.cards[index];
const now = new Date();
if (difficulty >= 3) {
card.ease = Math.max(1.3, card.ease + 0.1 * (difficulty - card.ease));
card.interval = card.interval * card.ease;
} else {
card.ease = Math.max(1.3, card.ease - 0.2);
card.interval = 1;
}
card.nextReview = new Date(now.getTime() + card.interval * 24 * 60 * 60 * 1000);
this.saveCards();
this.renderCards();
this.updateStats();
this.reviewControls.style.display = 'none';
this.selectedCard = null;
}
updateStats() {
const now = new Date();
this.totalCardsSpan.textContent = this.cards.length;
this.dueCardsSpan.textContent = this.cards.filter(card => card.nextReview <= now).length;
}
clearInputs() {
this.frontInput.value = '';
this.backInput.value = '';
}
saveCards() {
localStorage.setItem('flashcards', JSON.stringify(this.cards));
}
}
document.addEventListener('DOMContentLoaded', () => {
try {
new FlashcardApp();
} catch (error) {
console.error('Error initializing FlashcardApp:', error);
}
});
Let's break down the key components of our JavaScript code:
The Flashcard Class
This class represents individual flashcards with:
Front and back content
A review interval (number of days until next review)
A next review date
An "ease" factor that determines how quickly the interval grows
The FlashcardApp Class
This is our main class that handles all app functionality. Let's explore its key methods:
Initialization and Setup
constructor() {
// Loads cards from localStorage
// Sets up DOM elements and event listeners
// Renders existing cards and updates statistics
}
initElements() {
// Gets references to DOM elements
}
bindEvents() {
// Sets up event handlers for buttons and interactions
}
These methods handle the setup process when the app starts. We load any existing cards from localStorage, get references to important DOM elements, and set up event listeners for user interactions.
Creating and Deleting Cards
addCard() {
// Creates a new flashcard from input values
// Adds it to the collection and updates the UI
}
deleteCard(index) {
// Removes a card at the specified index
// Updates the UI and statistics
}
These methods manage the creation and deletion of flashcards, ensuring that the UI and stored data stay in sync.
Card Display and Interaction
renderCards() {
// Creates DOM elements for all cards
// Displays due date information
// Attaches event listeners
}
selectCard(card, element) {
// Handles card selection for review
// Shows the back of the card and review controls
}
These methods handle how cards are displayed and how users interact with them during review.
The Spaced Repetition Algorithm 🧪
The heart of our application is the review mechanism that implements spaced repetition:
reviewCard(difficulty) {
// Gets the selected card
// Adjusts the ease factor based on difficulty
// Calculates the new interval
// Sets the next review date
// Updates the UI
}
This method is called when a user rates a card as Hard, Medium, or Easy. Here's how the algorithm works:
If the card was rated as Easy (difficulty >= 3):
Increase the ease factor slightly (making future intervals longer)
Multiply the current interval by the ease factor
If the card was rated as Hard or Medium:
Decrease the ease factor (making future intervals shorter)
Reset the interval to 1 day
Calculate the next review date based on the new interval
This algorithm ensures that:
Cards you find difficult appear more frequently
Cards you know well appear less frequently
The spacing increases over time as you become more familiar with the content
It's a simplified version of algorithms used in popular spaced repetition systems like Anki, tailored for beginners to understand.
Persistence with localStorage 💾
To make sure our flashcards persist between browser sessions, we use localStorage:
saveCards() {
localStorage.setItem('flashcards', JSON.stringify(this.cards));
}
This method converts our cards array to a JSON string and stores it in the browser's localStorage. When the app loads, we retrieve this data and convert it back to JavaScript objects.
How to Use Flashcard Pro 📝
Using our app is simple:
Create cards: Enter the question on the front and the answer on the back
Review cards: Click on a card to see its answer
Rate your recall: After reviewing, rate how well you remembered the answer:
Hard: You struggled to remember
Medium: You remembered with some effort
Easy: You remembered easily
Trust the system: The app will automatically schedule future reviews based on your ratings
Ideas for Enhancement 🚀
Now that we have a working flashcard app with spaced repetition, here are some ways you could enhance it:
Categories and Tags: Allow users to organize cards by subject or topic
Rich Text Support: Enable formatting, images, and equations
Import/Export: Let users back up their cards or share them with others
Multiple Decks: Support separate collections for different subjects
Advanced Statistics: Show learning progress and forgetting curves
Mobile App: Convert to a PWA for better mobile experience
The Science Behind Spaced Repetition 🔬
Why does spaced repetition work so well? It's based on the forgetting curve, identified by psychologist Hermann Ebbinghaus in the 1880s. This curve shows how information is lost over time when there is no attempt to retain it.
Spaced repetition works by interrupting this forgetting process at strategic intervals, strengthening the memory each time. By spacing out reviews and adjusting intervals based on difficulty, we can dramatically improve long-term retention while minimizing study time.
Many studies have shown that spaced repetition can increase memory retention by 200-400% compared to traditional study methods. Now that's efficient learning! 📈
Conclusion
Building a flashcard app with spaced repetition is not just a fun coding project—it's creating a powerful tool that can genuinely improve how we learn and remember information. The application we've built combines clean design with an effective learning algorithm to help users study more efficiently.
Whether you're learning programming, languages, medicine, or any other knowledge-intensive field, incorporating spaced repetition into your study routine can make a tremendous difference in how much you retain and how quickly you learn.
Happy coding and happy learning! 🎓✨
Subscribe to my newsletter
Read articles from Learn Computer Academy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
