Crush Performance Issues on iPhones: Key Code Tweaks for Flawless Apps
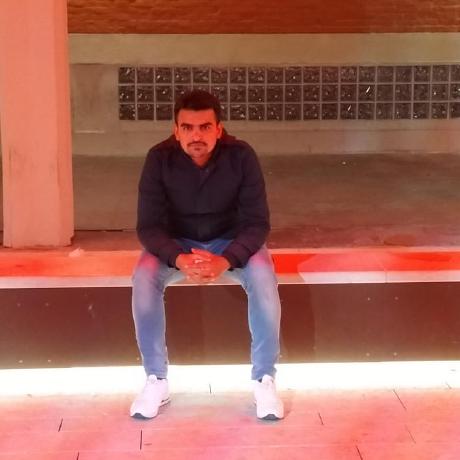
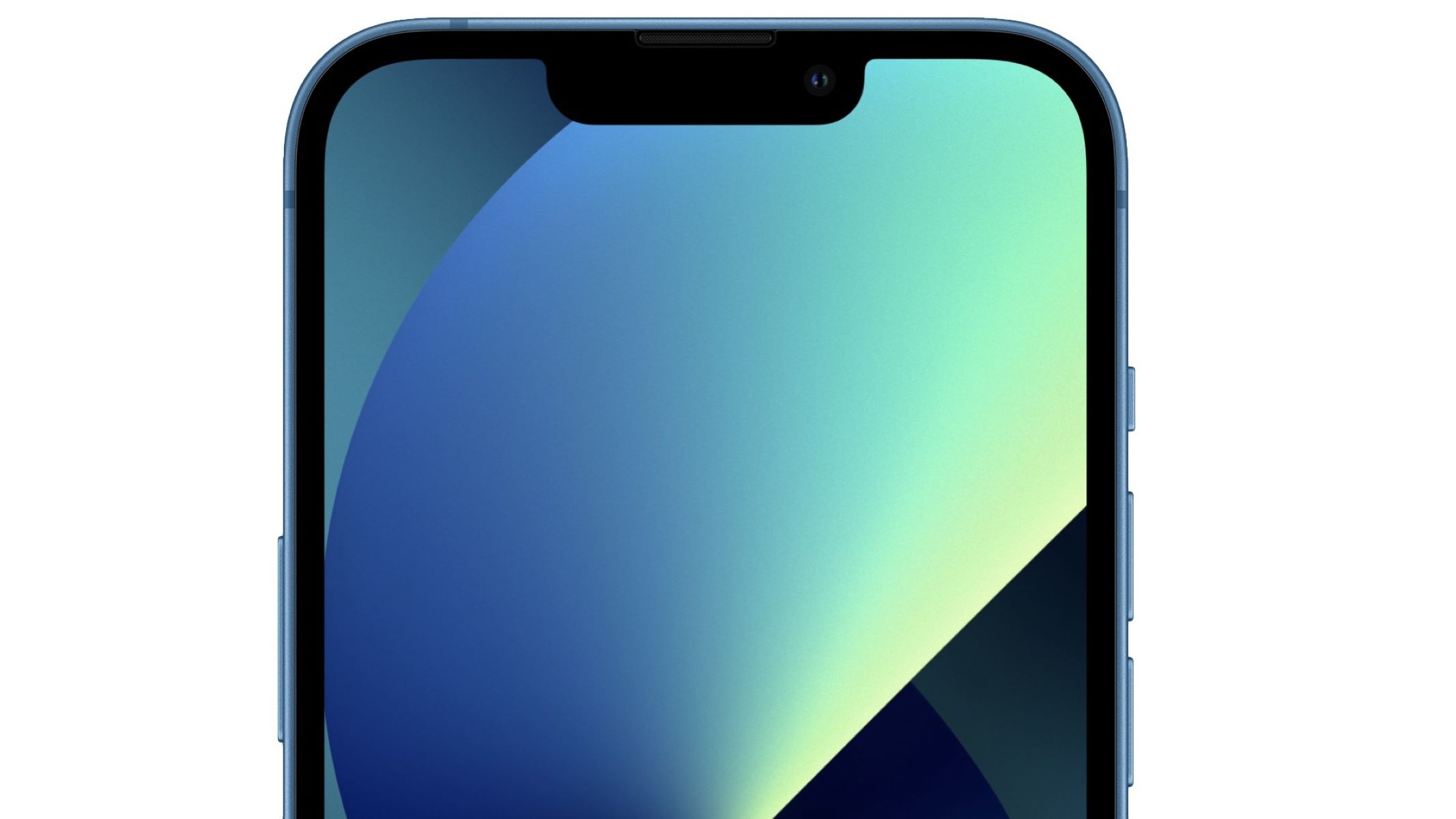
As the iPhone continues to dominate the smartphone market, developers face the challenge of ensuring that their applications run efficiently on iOS devices. Whether you are building a native app or a web application using JavaScript and frameworks like Vue.js, understanding iPhone hardware and iOS-specific constraints is essential for delivering a seamless user experience. This article provides actionable code examples of best practices and common mistakes to avoid, helping developers optimize their applications for iPhones.
Challenges in Optimizing Apps for iPhone
Building apps for iPhones presents unique challenges, including:
Device Fragmentation: While iPhones have fewer device models compared to Android, variations in performance, screen sizes, and iOS versions still exist.
Memory Constraints: iOS enforces strict memory limits, which can affect performance, especially with web-based applications.
JavaScript Performance: Heavy JavaScript tasks can cause delays or poor performance, especially on lower-end iPhone models.
Apple’s App Store Guidelines: Apps need to meet performance standards to be approved on the App Store.
To address these challenges, developers need to employ best practices in code optimization and architecture.
Code Best Practices for iPhone Optimization
Here are specific "do's" for ensuring optimal performance on iPhones:
1. Use Lazy Loading for Vue.js Routes
Lazy loading in Vue.js helps reduce the initial bundle size by loading only the components required for the current route. This improves the app's startup time, which is crucial on devices with limited resources like the iPhone.
Do:
// Lazy load Vue components using dynamic imports
const routes = [
{
path: '/home',
component: () => import('@/views/Home.vue') // Component is loaded only when the route is accessed
},
{
path: '/about',
component: () => import('@/views/About.vue') // Another route with lazy loading
}
];
By using dynamic imports, Vue.js will only load the necessary components, improving initial load time on iPhones.
2. Use Web Workers for Background Processing
Heavy computations can block the main thread, leading to UI freezes or stutter. Web Workers run JavaScript in the background, offloading computationally expensive tasks and allowing the main thread to remain responsive.
Do:
// Create a new web worker for background computation
const worker = new Worker('worker.js');
worker.postMessage({task: 'processData', data: largeDataset});
worker.onmessage = (event) => {
console.log('Processing complete:', event.data);
};
Inside worker.js
:
onmessage = (e) => {
const result = processData(e.data);
postMessage(result); // Send the result back to the main thread
};
function processData(data) {
// Perform heavy data processing here
return data.map(item => item * 2); // Example computation
}
By using Web Workers, you ensure that intensive tasks don’t block the UI thread, resulting in a smoother experience on iPhones.
3. Use requestAnimationFrame
for Smooth Animations
To achieve smooth, high-performance animations, use requestAnimationFrame, which synchronizes updates with the device’s refresh rate, reducing jank and providing smoother visual effects.
Do:
// Efficient animation loop with requestAnimationFrame
function animate() {
// Animation code here
requestAnimationFrame(animate); // Call animate recursively for continuous animation
}
animate();
This ensures that your animations are processed during the optimal time frame, minimizing jank and improving responsiveness on iPhones.
4. Use Efficient State Management with Vuex or Pinia
For Vue.js applications, managing state efficiently is key to performance. Pinia (or Vuex for older versions of Vue) helps manage the application state in a centralized manner, preventing unnecessary re-renders and improving app performance.
Do:
// Using Pinia for state management
import { defineStore } from 'pinia';
export const useMainStore = defineStore('main', {
state: () => ({
userData: null
}),
actions: {
async fetchUserData() {
this.userData = await fetchUserDataFromAPI();
}
}
});
By using Pinia for state management, you ensure that only the necessary components are re-rendered when state changes, optimizing performance on iPhones.
Common Mistakes to Avoid
To further enhance performance, developers should avoid the following common mistakes:
1. Avoid Synchronous JavaScript Calls
Synchronous operations block the main thread, causing the UI to freeze. This is especially problematic on mobile devices like iPhones with limited resources.
Don't:
// Synchronous code that blocks the main thread
const response = await fetch('/api/data'); // Blocks UI until the response is received
console.log(response);
Solution: Use asynchronous code to prevent blocking the main thread.
// Correct asynchronous implementation
async function fetchData() {
const response = await fetch('/api/data');
const data = await response.json();
console.log(data);
}
fetchData();
By avoiding blocking operations, you ensure that the iPhone’s UI thread remains free for smooth interactions.
2. Don’t Forget to Clean Up Resources
Memory leaks can degrade performance over time, especially on iPhones with lower memory resources. Failing to clean up intervals, timeouts, and event listeners can lead to memory issues.
Don't:
// Forgetting to clear timeouts or intervals
const interval = setInterval(() => {
console.log('This is running indefinitely');
}, 1000);
// Forgetting to clear the interval causes a memory leak
Solution: Always clean up resources when they are no longer needed.
// Correct: Always clear intervals when no longer needed
clearInterval(interval);
Proper resource management ensures that your app runs efficiently without draining device memory.
3. Don’t Overload the DOM
Rendering too many DOM elements can slow down performance, especially on mobile devices like iPhones. Overloading the DOM can cause unnecessary reflows, impacting performance.
Don't:
<!-- Don't overload the DOM with unnecessary elements -->
<div id="app">
<div v-for="item in largeList" :key="item.id">{{ item.name }}</div>
</div>
Solution: Use virtualization or lazy loading to render only the visible DOM elements, improving performance.
// Use vue-virtual-scroller for rendering only visible elements
import VirtualList from 'vue-virtual-scroller';
<VirtualList :items="largeList" :item-size="50">
<template v-slot="{ item }">
<div>{{ item.name }}</div>
</template>
</VirtualList>
By only rendering the visible items, you reduce the number of DOM elements and optimize rendering on iPhones.
4. Don’t Overuse Animations
Heavy use of animations can cause performance issues, especially if not optimized for mobile devices. Excessive animations or animations that aren’t GPU-accelerated can result in lag.
Don't:
// Avoid using non-optimized animations
element.style.transition = 'transform 1s ease'; // This can slow down performance on iPhones
Solution: Use CSS animations with hardware acceleration (e.g., using transform and opacity).
/* Optimized CSS animation using hardware acceleration */
.element {
transition: transform 0.3s ease;
transform: translate3d(0, 0, 0); /* Use translate3d for GPU acceleration */
}
Optimized animations ensure better performance on iPhones, providing smoother interactions.
Conclusion
Optimizing your application for iPhone performance involves leveraging efficient coding practices and optimizing resources. By following best practices such as lazy loading, using web workers for background tasks, and employing efficient state management, developers can significantly improve performance. Avoiding common pitfalls like synchronous JavaScript calls, memory leaks, and excessive DOM rendering ensures that your app performs well on iPhones, providing a smooth and responsive user experience.
Testing on real iPhones and adhering to Apple’s guidelines for performance will help you create an app that stands out in the competitive mobile landscape. By taking these steps, your application will run smoothly, enhancing user satisfaction and engagement.
For further reading, consider exploring resources on performance optimization in web apps and mobile development:
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
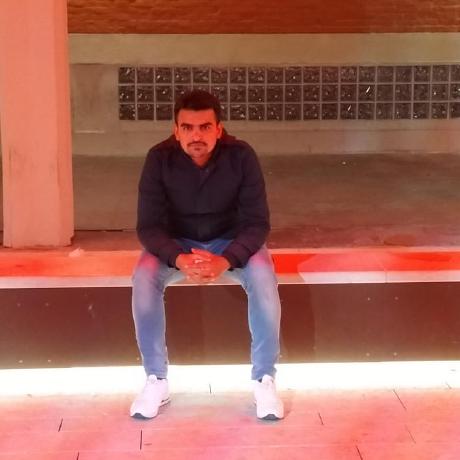
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.