π Chapter 45:Mastering Java Collections Framework: The Power Behind Efficient Data Management! π
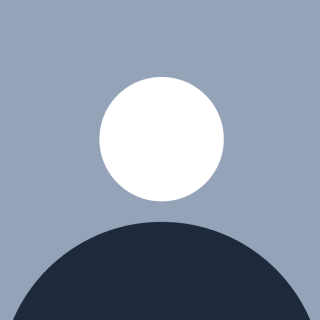
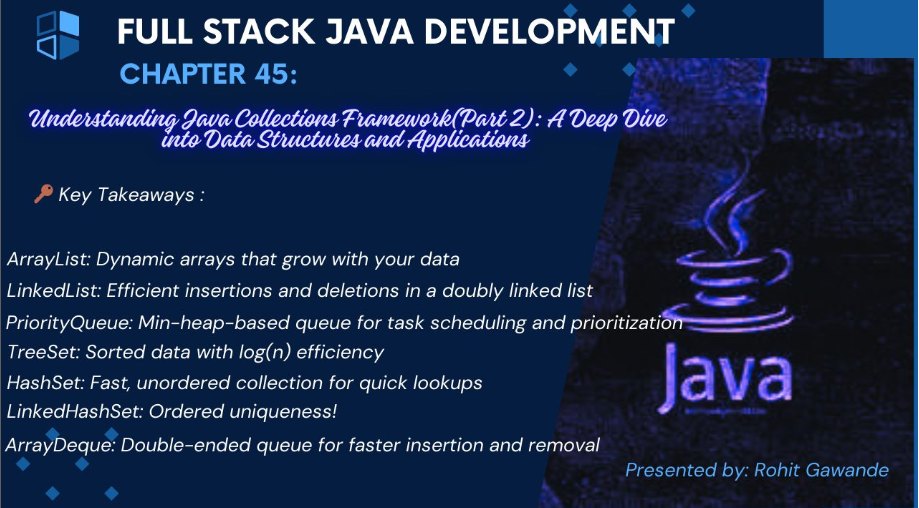
Understanding Java Collections Framework: A Deep Dive into Data Structures and Applications
In Java programming, managing and manipulating groups of objects is a common task β whether itβs storing user data, processing tasks, or organizing elements for computation. To simplify this, Java offers the Collections Framework β a powerful, well-structured set of classes and interfaces designed to handle data structures and algorithms efficiently.
The Collections Framework provides a unified architecture for representing and manipulating collections, making it easier to:
Store dynamic amounts of data (like lists, sets, and maps)
Perform complex operations (sorting, searching, and iteration)
Improve code readability and reusability
In this chapter, we'll dive into the core concepts of collections, explore key interfaces such as List, Set, and Queue, and uncover how classes like ArrayList, LinkedList, and PriorityQueue work under the hood. Whether you're building a simple task scheduler or a complex data processor, mastering collections will elevate your Java programming skills.
Introduction to Collections Framework
In Java, the Collections Framework is a unified architecture that provides various interfaces and classes to handle groups of objects. Introduced in Java 1.2, it offers a comprehensive, flexible, and efficient way to manage data in your programs. The framework defines several interfaces such as List
, Set
, Queue
, and Map
, each with multiple implementations that suit various needs.
Overview of Major Collection Classes
The Collections Framework consists of seven major classes, each of which serves a unique purpose and is backed by a specific data structure. These include:
ArrayList (Dynamic Array)
LinkedList (Doubly Linked List)
ArrayDeque (Double-ended Queue)
PriorityQueue (Min-Heap)
TreeSet (Self-balancing Binary Search Tree)
HashSet (Hash Table-based Set)
LinkedHashSet (HashSet with predictable iteration order)
Each of these classes implements the Collection
interface and provides different advantages based on their underlying data structures. In this post, we'll explore these classes in detail, dive into their characteristics, and understand their use cases.
π Understanding Priority Queue in Java
1. Introduction to Priority Queue
The PriorityQueue class in Java is part of the Java Collections Framework and is present in the java.util package.
It implements the Queue interface and follows the rules of a Min-Heap Data Structure to store elements.
2. Important Characteristics
Min-Heap Property: Elements with the lowest value have the highest priority and are placed at the top of the heap.
Insertion Order: Unlike ArrayList, LinkedList, and ArrayDequeβwhich maintain insertion orderβPriorityQueue does NOT maintain insertion order.
Index-based Access: Accessing elements by index (like
list.get(index)
) is not allowed in PriorityQueue.Duplicate Elements: Duplicates are allowed in a PriorityQueue.
β‘ How Min-Heap Works in Priority Queue
A Min-Heap organizes elements in a binary tree structure, ensuring that:
The root node contains the smallest element.
Each parent node is smaller than its child nodes.
Letβs break down how elements are added and arranged in the Priority Queue using the following sequence:
Input:100, 50, 150, 25, 75, 125, 175
Steps:
Add 100:
100 is the first element, so it becomes the root node (since itβs the only node).
π’ Root: 100
Add 50:
50 is added to the left of 100 and compared with 100.
Since 50 < 100, a swap happens β 50 goes up, 100 comes down.
π’ Tree:
50 100
Add 150:
150 is added to the right of 50.
Since 150 > 50, no swap occurs.
π’ Tree:
50 100 150
Add 25:
25 is added to the left of 100.
25 < 100 β swap happens between 25 and 100.
Then, 25 < 50 β swap happens again, so 25 becomes the root.
π’ Tree:
25 50 150 100
Add 75:
75 is added to the right of 100.
Since 75 > 50 and 25, no swaps are needed.
π’ Tree:
25 50 150 100 75
Add 125:
125 is added to the left of 150.
125 < 150 β swap happens between 125 and 150.
π’ Tree:
25 50 125 100 75 150
Add 175:
175 is added to the right of 150.
Since 175 > 150, no swaps are done.
π’ Final Min-Heap:
25 50 125 100 75 150 175
ποΈ Java Code Implementation
import java.util.*;
public class Collection9 {
public static void main(String[] args) {
PriorityQueue<Integer> pq = new PriorityQueue<>();
// Adding elements to Priority Queue
pq.add(100);
pq.add(50);
pq.add(150);
pq.add(25);
pq.add(75);
pq.add(125);
pq.add(175);
// Printing Priority Queue (min-heap order)
System.out.println(pq); // Output: [25, 50, 125, 100, 75, 150, 175]
// Adding a duplicate element
pq.add(25);
System.out.println(pq); // Output: [25, 25, 125, 50, 75, 150, 175, 100]
}
}
Output:
[25, 50, 125, 100, 75, 150, 175]
[25, 25, 125, 50, 75, 150, 175, 100]
π₯ Key Takeaways (Conclusion):
Data Structure: Every collection class in Java follows an internal data structure.
Priority Queue: Uses the Min-Heap Data Structure.
Element Order: The smallest element (i.e., highest priority) always appears at the top of the heap or beginning of the collection.
Insertion Order: Not maintained β elements are arranged based on priority (min-heap rules).
Swapping Mechanism: Lower numbers move up the tree, and higher numbers move down.
Duplicates: Allowed β you can add the same value multiple times.
Access: Index-based access is not supported β elements are accessed in priority order.
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
π Tech Enthusiast | Full Stack Developer | System Design Explorer π» Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iβm Rohit Gawande! πI am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iβm Currently Doing πΉ Writing an in-depth System Design Series to help developers master complex design concepts.πΉ Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.πΉ Exploring advanced Java concepts and modern web technologies. What You Can Expect Here β¨ Detailed technical blogs with examples, diagrams, and real-world use cases.β¨ Practical guides on Java, System Design, and Full Stack Development.β¨ Community-driven discussions to learn and grow together. Letβs Connect! π GitHub β Explore my projects and contributions.πΌ LinkedIn β Connect for opportunities and collaborations.π LeetCode β Check out my problem-solving journey. π‘ "Learning is a journey, not a destination. Letβs grow together!" Feel free to customize or add more based on your preferences! π