Chapter 46: Collections in Java (Part 3) | Full Stack Java Development
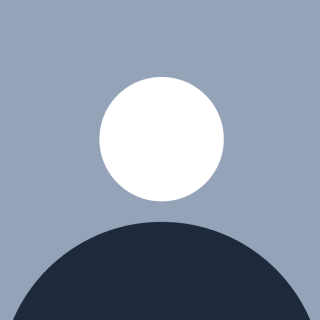
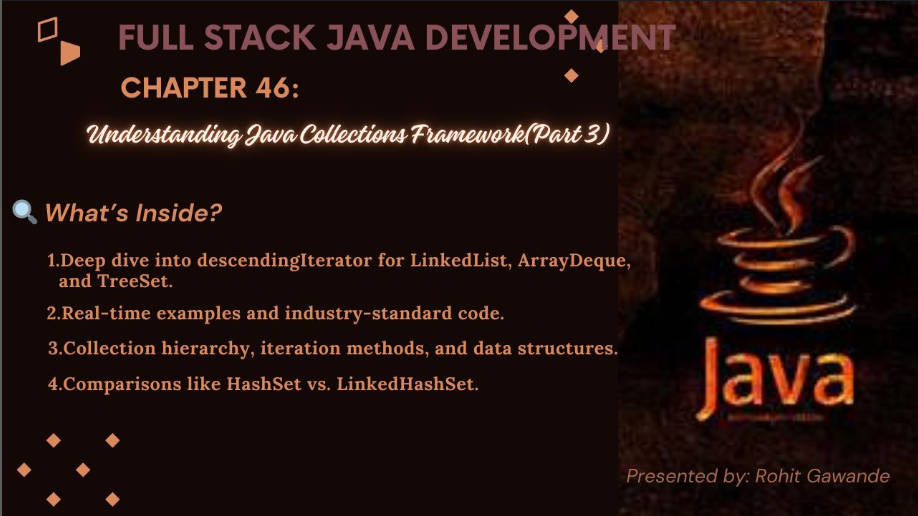
2.2 DescendingIterator
Introduction
The descendingIterator()
method allows traversal of elements in reverse order. It is available in:
LinkedList
ArrayDeque
TreeSet
How DescendingIterator Works
The iterator starts from the last element and moves backward.
Uses
hasNext()
andnext()
methods to traverse the collection.Returns elements as
Object
, requiring explicit downcasting when needed.
Example: Accessing Elements in Reverse Order
import java.util.Iterator;
import java.util.LinkedList;
public class Collection15 {
public static void main(String[] args) {
LinkedList<Integer> ll1 = new LinkedList<>();
ll1.add(10);
ll1.add(20);
ll1.add(30);
ll1.add(40);
ll1.add(50);
System.out.println(ll1); // Output: [10, 20, 30, 40, 50]
Iterator<Integer> ditr = ll1.descendingIterator();
while (ditr.hasNext()) {
System.out.print(ditr.next() + " "); // Output: 50 40 30 20 10
}
}
}
Real-World Use Case: Why Collections in Java?
Java collects data from HTML or user input and stores it in collections.
A separate class manages database interactions.
Data retrieval occurs via Java programs querying the database.
Collections like
ArrayList
store and transfer data between layers (e.g., servlet to HTML).
1. Vector Class
Part of legacy collections.
Uses
Enumeration
instead ofIterator
.Methods:
hasMoreElements()
andnextElement()
.
Example: Accessing Objects with Enumeration
import java.util.Vector;
import java.util.Enumeration;
public class Collection16 {
public static void main(String[] args) {
Vector<Integer> v = new Vector<>();
v.add(10);
v.add(20);
v.add(30);
v.add(40);
System.out.println(v); // Output: [10, 20, 30, 40]
Enumeration<Integer> em = v.elements();
while (em.hasMoreElements()) {
System.out.print(em.nextElement() + " "); // Output: 10 20 30 40
}
}
}
2. Java Collection Framework Hierarchy
Iterable
|
|
Collection
________________________|_____________________________
| | |
List(Interface) Queue(Interface) Set(Interface)
| | | | |
| | PriorityQueue(class) | |--HashSet(class)
|-ArrayList(class) | | |
| Deque(Interface) | |---LinkedList(class)
|-LinkedList(class)-----| |
| | |
|-Vector(class) ArrayDeque(class) |
| extends |
Stack SortedSet(Interface)
|
Navigable(Interface)
|
TreeSet
Key Points
LinkedList
implements bothList
andDeque
interfaces.Deque
extendsQueue
, adding specialized methods.PriorityQueue
has fewer methods thanArrayDeque
because it directly implementsQueue
.TreeSet
implementsNavigableSet
for sorted elements.
3. Accessing Objects in Collections
Classes | For-loop | For-each loop | Iterator | ListIterator | DescendingIterator | Enumeration |
ArrayList | Yes | Yes | Yes | Yes | No | No |
LinkedList | Yes | Yes | Yes | Yes | Yes | No |
ArrayDeque | No | Yes | Yes | No | Yes | No |
PriorityQueue | No | Yes | Yes | No | No | No |
TreeSet | No | Yes | Yes | No | Yes | No |
HashSet | No | Yes | Yes | No | No | No |
LinkedHashSet | No | Yes | Yes | No | No | No |
4. Summary of Java Collections
Classes | Internal Data Structure | Order Preserved | Null Allowed | Duplicates Allowed |
ArrayList | Dynamic Array | Yes | Yes | Yes |
LinkedList | Doubly Linked List | Yes | Yes | Yes |
ArrayDeque | Double-ended Queue | Yes | No | Yes |
PriorityQueue | Min-Heap | No | No | Yes |
TreeSet | Balanced BST | No | No | No |
HashSet | HashTable | No | Yes | No |
LinkedHashSet | HashTable + LinkedList | Yes | Yes | No |
Notes
TreeSet
sorts elements automatically; hence, it does not allownull
.PriorityQueue
follows a min-heap structure, preventingnull
values.HashSet
does not guarantee order, butLinkedHashSet
preserves insertion order.
5. Tight Coupling vs. Loose Coupling in Java Collections
Example: Using Loose and Tight Coupling
import java.util.*;
public class Collection17 {
public static void main(String[] args) {
ArrayList<Integer> al = new ArrayList<>(); // Tight coupling
List<Integer> list = new ArrayList<>(); // Loose coupling (Interface reference)
Queue<Integer> q = new ArrayDeque<>();
List<Integer> li = new LinkedList<>();
Deque<Integer> ql = new LinkedList<>();
Collection<Integer> c = new LinkedList<>();
// peek() is present in Deque interface
ql.peek();
HashSet<Integer> hs = new HashSet<>(); // Tight coupling
Set<Integer> se = new HashSet<>(); // Loose coupling
}
}
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
π Tech Enthusiast | Full Stack Developer | System Design Explorer π» Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iβm Rohit Gawande! πI am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iβm Currently Doing πΉ Writing an in-depth System Design Series to help developers master complex design concepts.πΉ Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.πΉ Exploring advanced Java concepts and modern web technologies. What You Can Expect Here β¨ Detailed technical blogs with examples, diagrams, and real-world use cases.β¨ Practical guides on Java, System Design, and Full Stack Development.β¨ Community-driven discussions to learn and grow together. Letβs Connect! π GitHub β Explore my projects and contributions.πΌ LinkedIn β Connect for opportunities and collaborations.π LeetCode β Check out my problem-solving journey. π‘ "Learning is a journey, not a destination. Letβs grow together!" Feel free to customize or add more based on your preferences! π