Writing Unit Tests in Django: Part 1(URLs)

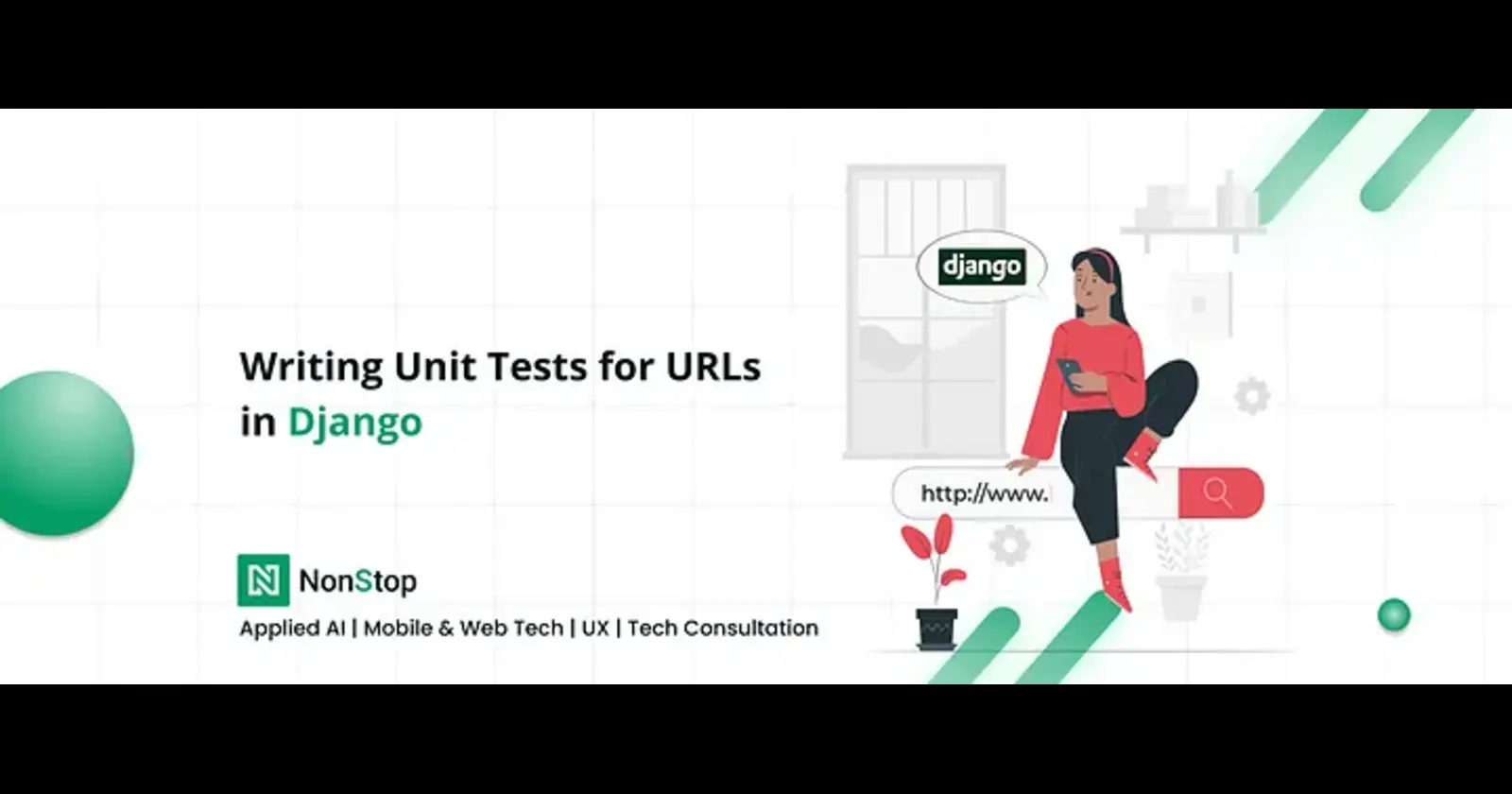
Introduction
In this article, we’ll explore how to write tests in Django to ensure everything works as expected. Before writing tests, you need to set up a tests
directory within your Django app. The tests
folder should include an __init__.py
file, which allows Django to recognize it as a Python package. This setup enables Django to automatically discover and run your tests. Additionally, test files and test methods should begin with the test_
prefix to ensure Django can identify them properly.
Sample ‘tests’ Directory
How to Test URLs?
Testing a URL means, ensuring the URL maps to the correct view.
# urls.py file
from django.urls import path
from . import views
urlpatterns = [
path('', views.blog_list, name='list'),
path('add/', views.BlogCreateView.as_view(), name='add'),
path('<int:id>/', views.blog_detail, name='detail')
]
# test_urls.py file
from django.urls import reverse, resolve
from django.test import SimpleTestCase
from blog.views import blog_list, blog_detail, BlogCreateView
class TestUrls(SimpleTestCase):
def test_list_url_resolves(self):
url = reverse('list')
self.assertEqual(resolve(url).func, blog_list)
def test_detail_url_resolves(self):
url = reverse('detail', args=[1])
# ID has to passed to this url
self.assertEqual(resolve(url).func, blog_detail)
def test_create_url_resolves(self):
url = reverse('add')
self.assertEqual(resolve(url).func.__name__, BlogCreateView.__name__)
# or
self.assertEqual(resolve(url).func.view_class, BlogCreateView)
Explanation
We have three URLs: two using function-based views (blog_list
and blog_detail
) and one using a class-based view (BlogCreateView
).
For testing these URLs, we created a Test Class that inherits from Django’s test module (SimpleTestCase
)since our tests don't involve database operations or transactions. The Test Class contains three test cases, each verifying that the URL correctly maps to its associated view. Instead of hardcoding the URLs, I used reverse()
and URL name. This approach makes it easier to maintain: if a URL changes, we only need to update it in one place. We then use the assertEqual()
to check that the resolved URL points to the correct view.
Run Tests
To run the tests, open up the terminal, navigate to the Project Directory, and run the below command:
python manage.py test <app name>
Thank you for your time.
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.