ODC with Loop Components - Adaptive Card Refresh

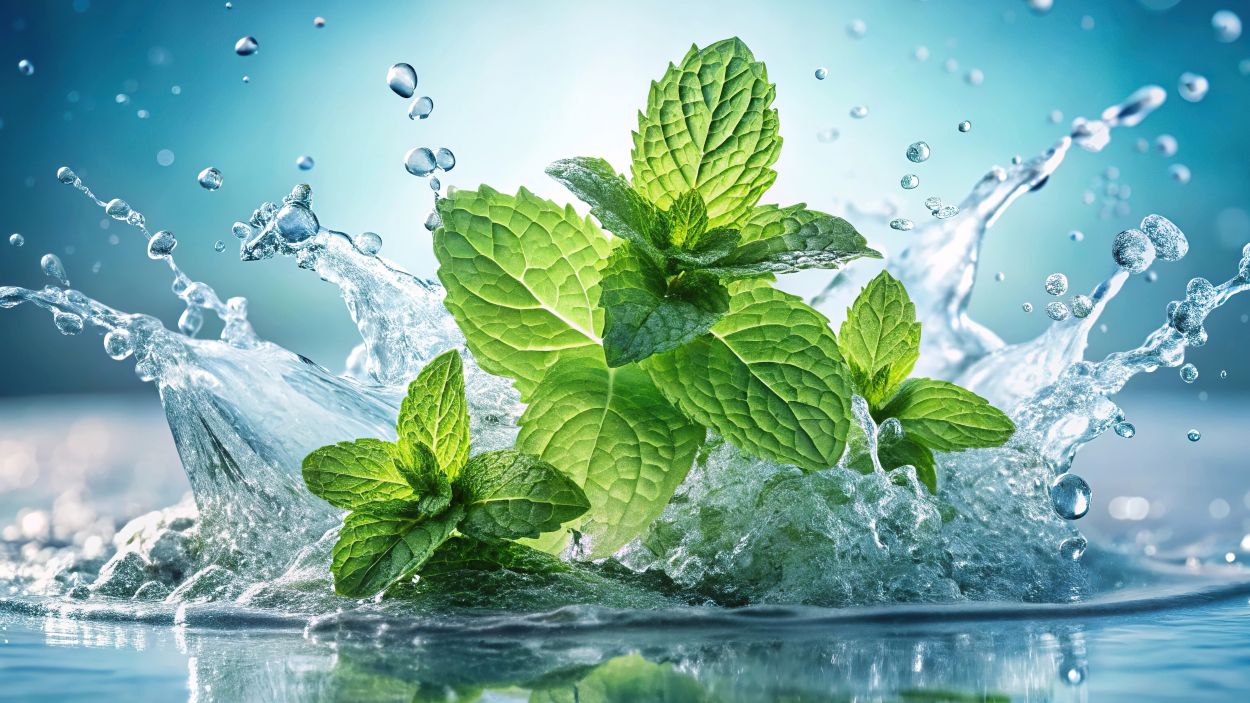
At the end of the previous part of this series, we were able to copy a link from our OutSystems Developer Cloud application, paste it into a Microsoft Teams conversation, and have our messaging endpoint "unfurl" this link into an Adaptive Card Loop component. However, the cards displayed in a Microsoft Teams conversation are still static, meaning they do not update when the data in our application changes, such as the price of an auction. One of the key characteristics of a Loop component is that it is "Live," meaning it automatically updates its content when the bound data changes.
In this tutorial, we explore how to refresh an Adaptive Card-based Loop component using a Universal Action for Adaptive Cards. Universal Actions are Adaptive Card actions that send an Activity of type “invoke” to the messaging endpoint with additional data and Refresh is a special type of a Universal Action.
Demo Application
This article series includes a demo application called "ODC with Loop Components Demo," available on ODC Forge. Be sure to download the version of the application that matches each article in this series.
For this article, you need to install Version 0.2 from ODC Forge.
In the ODC Portal, go to Forge - All Assets.
Search for "ODC with Loop Components Demo".
Click on the Asset (Do not click on Install on the tile!).
Switch to the Version tab and click on Install next to Version 0.2.
Version 0.2 depends on other Forge components:
OAuthTokenExchange - An external logic library that helps retrieve access tokens easily. In this tutorial however we use an action to retrieve the discovery document from Microsoft Entra only.
LiquidFluid - An external logic library that merges data with templates based on the Shopify Liquid Templating language.
UriParser - An external logic library that parses a given URI/URL.
After updating the demo application in your environment, you can try it out right away because the App Manifest—the configuration file that added the app to Microsoft Teams—has not changed. Note that only new Loop components will refresh, while all previously added cards in a channel will stay the same.
Recap
In part one of the series, our messaging endpoint only responded to Activities of type "invoke" with the name "composeExtension/queryLink." To create this response, we used a single liquid template (based on the Shopify Liquid templating language) and merged it with auction data.
The response looked like this
{
"composeExtension": {
"type": "result",
"attachmentLayout": "list",
"attachments": [
{
"preview": <<Preview Adaptive Card>>
"content": <<Full Adaptive Card>>
}
]
}
}
“preview” contains a simplified Adaptive Card (a thumbnail card), while “content” holds the full details of the Adaptive Card. Consider this response as a one-time message from your Messaging Endpoint to initially display an Adaptive Card in a channel.
"One-time" means we cannot reuse the same liquid template for future responses, such as refreshing a card (covered in this tutorial) or responding to user interactions (covered in the next tutorial). Future responses must include only the full Adaptive Card, which means just the “content” object from the response shown above.
In summary
composeExtension/queryLink - Respond with a “composeExtension” object containing an attachment with a preview and full card.
other - Respond with an Adaptive Card only.
To accomodate this we have to split our single liquid template into multiple templates. Let’s get started.
Templates
Open the ODC with Loop Components Demo application in ODC Studio and go to Data - Resources - Templates.
Note the various liquid templates in the folder, download them locally and inspect them with a text editor.
AuctionCard - This template represents a full Adaptive Card for an auction.
AuctionCardPreview - This is the preview version of the auction card.
AuctionQueryLinkResponse - This template is used to create a composeExtension response for link unfurling requests. It combines the results of the AuctionCard and AuctionCardPreview templates.
StatusValueResponse - This template is combined with the result of an Auction card and is used for follow-up responses of an auction card, such as when refreshing a card. It includes a "status code," a "type," and a "value”. In our case the value will always be an Adaptive Card.
Render AuctionCard and AuctionCardPreview
Go to Logic - Server Actions - Loop and check both RenderAuctionCard and RenderAuctionCardPreview. They are similar, but they use different templates and have a different input parameter structure.
First, the card payload data is serialized and then merged with the liquid template in Resources. Finally, the rendered template is returned.
Render QueryLinkResponse
Inspect the RenderQueryLinkResponse server action. This action creates the queryLink response by combining the results of RenderAuctionCard and RenderAuctionCardPreview into a liquid template.
RenderAuctionPreviewCard - Returns the rendered preview card.
RenderAuctionCard - Returns the full auction card.
UnfurlPayload - Assigns both the preview and full card to a local structure instance of AuctionQueryLink.
SerializeUnfurlPayload - Serializes the payload to a string (LiquidFluid only accepts JSON data as a string to merge with a template).
RenderTemplate - Renders the AuctionQueryLinkResponse template.
Render StatusValueResponse
As mentioned above, subsequent responses for an existing card must only include the Adaptive Card. However, that card must be wrapped in a response structure that also returns a status code and type. The RenderStatusValueResponse server action wraps a rendered auction card in the required response structure.
The process here is straightforward, and you should already be familiar with it. Here is an example of what this action produces.
{
"statusCode": 200,
"type": "application/vnd.microsoft.card.adaptive",
"value": {
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"version": "1.6",
"type": "AdaptiveCard",
"metadata": {
"webUrl": "https://<<ODC Stage>>/ODCwithLoopComponentsDemo/AuctionDetail?AuctionId=9f890964-b33d-4817-8fa7-41171c8a0c3c"
},
"refresh": {
"action": {
"type": "Action.Execute",
"title": "Refresh",
"verb": "refreshAuctionCard",
"data": {
"url": "https://<<ODC Stage>>/ODCwithLoopComponentsDemo/AuctionDetail?AuctionId=9f890964-b33d-4817-8fa7-41171c8a0c3c"
}
}
},
"body": [
{
"type": "TextBlock",
"size": "medium",
"weight": "bolder",
"text": "Mein bestes Produkt"
},
{
"type": "ColumnSet",
"columns": [
{
"type": "Column",
"width": "stretch",
"items": [
{
"type": "TextBlock",
"text": "was ich jemands erste",
"wrap": true
}
],
"verticalContentAlignment": "Center",
"spacing": "Medium"
},
{
"type": "Column",
"width": "auto",
"items": [
{
"type": "TextBlock",
"text": "500.0",
"wrap": true,
"size": "ExtraLarge",
"weight": "Bolder"
}
]
}
],
"spacing": "None"
}
]
}
}
Refresh Action
Before we dive into how everything comes together at the messaging endpoint, let's quickly explore how a channel (like Microsoft Teams) knows that an Adaptive Card needs to be refreshed and how our messaging endpoint identifies which auction card should be updated.
Open the AuctionCard template in a text editor.
{
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"version": "1.6",
"type": "AdaptiveCard",
"metadata": {
"webUrl": "{{webUrl}}"
},
"refresh": {
"action": {
"type": "Action.Execute",
"title": "Refresh",
"verb": "refreshAuctionCard",
"data": {
"url": "{{webUrl}}"
}
}
},
"body": ....,
}
What's new is a “refresh” object that defines an action.
type - This is always Action.Execute.
title - Besides automatic card refreshes, Microsoft Teams and other channel applications show a three-dot menu next to the card to manually execute the action. The title you enter here becomes the menu option name.
verb - This is any string that helps us identify which action is requested. At the messaging endpoint, we use this verb to recognize the refresh request.
data - This is additional data sent to the messaging endpoint when the refresh action is requested. Here, we define a single item “url.” The handlebars
{{ webUrl }}
are replaced with the full URL of the Loop component (auction) when merging the template with data in the RenderAuctionCard server action.
Messaging Endpoint
In Logic - Integrations - REST - MassgingEndpoint open the Messages endpoint. In the last part the first switch statement had only one condition for the link unfurling (type of “invoke” and name of “composeExtension/queryLink”).
Adaptive Card-based actions, whether it's a refresh or any other defined action in the card, send an activity request of type "invoke" with a name of "adaptiveCard/action". This invocation is now handled in the second condition added to the switch statement.
Right after DeserializeAdaptiveCardActionActivity is called, which deserializes the request into a structure that provides access to two important pieces of information:
verb - The action verb as defined in the Auction Adaptive Card. We use the verb to identify which action is requested.
data - Additional data defined in the Auction Adaptive Card and passed to the messaging endpoint. Currently, only the Loop component URL is passed.
The next switch statement currently has a single condition that checks the verb and executes MessagingRefreshAuctionAction.
MessagingRefreshAuctionAction
This action generates the response that our messaging endpoint needs to send back to the channel. It includes an Adaptive Card for the auction, wrapped in a Status response structure.
Auction_GetByUrl - This auction parses the given URL (sent via the invoke activity) for the auction identifier. Then it queries the Auction entity and returns the auction details.
RenderAuctionCard - see above. Renders the Adaptive Card for the auction.
RenderStatusValueResponse - see above. Wraps the auction card in a status response structure.
Try
Paste an auction link from the demo application into a Microsoft Teams conversation. This will first display the auction card.
Next, change the auction price in the demo application.
In Microsoft Teams, click on a different conversation, then return to the conversation where you pasted the link. The card will update to show the new price you entered.
Summary
In this tutorial, we explored how to keep Adaptive Card-based Loop components up to date in Microsoft Teams by adding a refresh action to a card and implementing a messaging endpoint action to return the most recent Adaptive Card for an auction.
The steps we implemented also lay the foundation for the next part of the tutorial, where we will add user interactions to the card.
I hope you enjoyed it. Please leave a comment with your feedback or any questions you have.
Subscribe to my newsletter
Read articles from Stefan Weber directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Stefan Weber
Stefan Weber
As a seasoned Senior Director at Telelink Business Services EAD, a leading IT full-service provider headquartered in Sofia, Bulgaria, I lead the charge in our Application Services Practice. In this role, I spearhead the development of tailored software solutions using no-code/low-code platforms and cutting-edge cloud-ready/cloud-native solutions based on the Microsoft .NET stack. Throughout my diverse career, I've accumulated a wealth of experience in various capacities, both technically and personally. The constant desire to create innovative software solutions led me to the world of Low-Code and the OutSystems platform. I remain captivated by how closely OutSystems aligns with traditional software development, offering a seamless experience devoid of limitations. While my managerial responsibilities primarily revolve around leading and inspiring my teams, my passion for solution development with OutSystems remains unwavering. My personal focus extends to integrating our solutions with leading technologies such as Amazon Web Services, Microsoft 365, Azure, and more. In 2023, I earned recognition as an OutSystems Most Valuable Professional, one of only 80 worldwide, and concurrently became an AWS Community Builder.