Mastering Kotlin Coroutines Channels

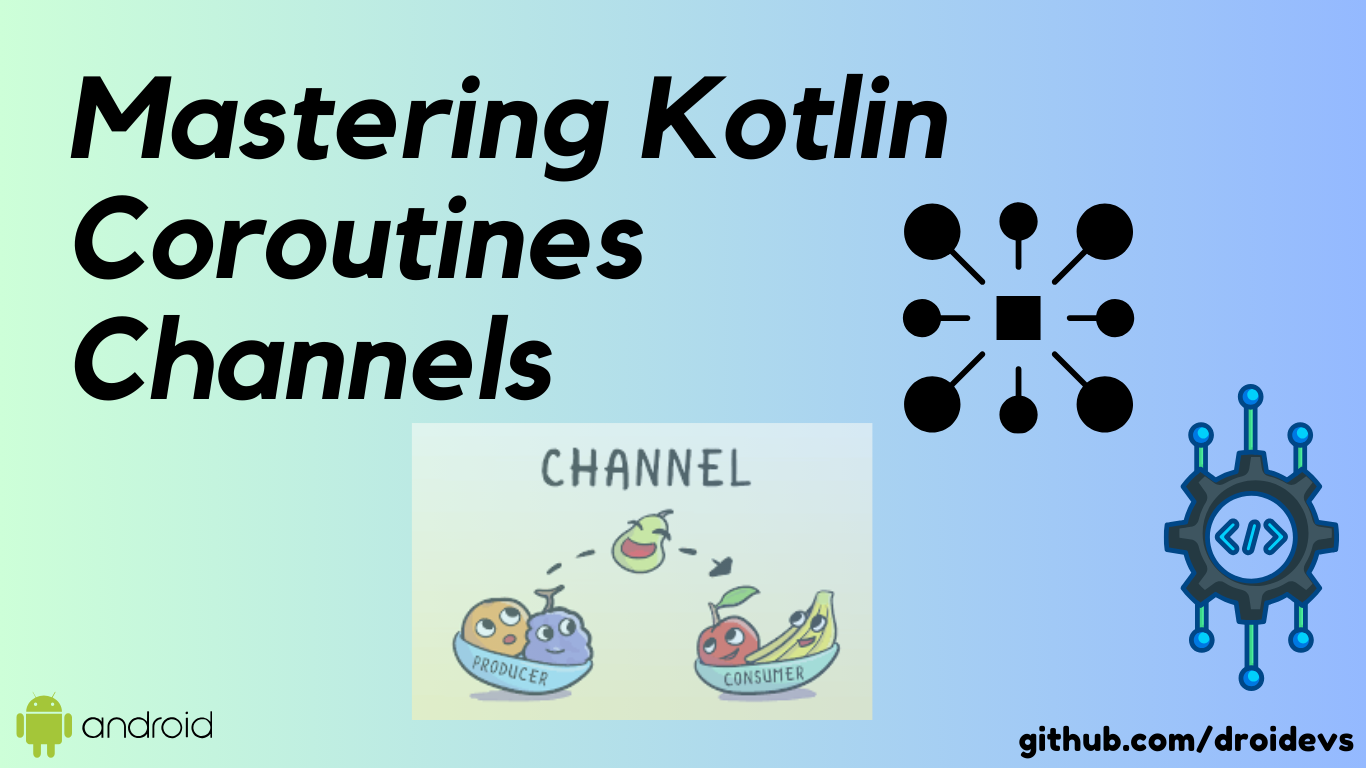
Introduction
Kotlin Coroutines revolutionized asynchronous programming by making it structured and intuitive. However, handling concurrent data streams efficiently requires more than just suspending functions and flows. This is where Coroutines Channels come in.
Similar to Java's BlockingQueue, Kotlin Coroutines Channels provide a way to send and receive data between coroutines asynchronously. Unlike traditional callbacks or shared mutable state, channels enable a safe and structured approach to concurrent communication.
What Are Coroutines Channels?
A Channel in Kotlin is a concept from CSP (Communicating Sequential Processes) that acts as a communication pipeline for coroutines. It allows one coroutine to send data and another coroutine to receive it, ensuring safe concurrency.
Key Features of Channels:
Buffered & Unbuffered Communication
Multiple Producers & Consumers
Structured Concurrency
Cancellation & Closing Mechanisms
Backpressure Handling
How to Use Channels in Kotlin?
Kotlin provides the Channel<T>
class, which allows sending and receiving elements.
1. Creating a Channel
import kotlinx.coroutines.*
import kotlinx.coroutines.channels.*
fun main() = runBlocking {
val channel = Channel<Int>()
launch {
for (i in 1..5) {
channel.send(i)
println("Sent: $i")
}
channel.close() // Closing the channel after sending all data
}
launch {
for (value in channel) {
println("Received: $value")
}
}
}
Output:
Sent: 1
Received: 1
Sent: 2
Received: 2
Sent: 3
Received: 3
Sent: 4
Received: 4
Sent: 5
Received: 5
Here, one coroutine sends data into the channel while another receives it, ensuring safe and structured concurrency.
Types of Channels in Kotlin
Channels can be unbuffered or buffered, affecting their behavior regarding send/receive operations.
1. Unbuffered Channel (Default)
Direct handoff between sender & receiver.
Suspends sender if there is no active receiver.
val channel = Channel<Int>() // Unbuffered by default
2. Buffered Channel
Stores elements up to a specified capacity.
Does not suspend sender until the buffer is full.
val bufferedChannel = Channel<Int>(capacity = 3)
3. Conflated Channel
Always keeps the latest value, discarding older ones.
Useful for UI state updates where only the latest value matters.
val conflatedChannel = Channel<Int>(Channel.CONFLATED)
4. Rendezvous Channel
Capacity = 0 (Unbuffered channel behavior).
Ensures that every send is immediately received.
val rendezvousChannel = Channel<Int>(Channel.RENDEZVOUS)
5. Unlimited Channel
Stores elements indefinitely in an unlimited queue.
Risk: High memory consumption if not managed properly.
val unlimitedChannel = Channel<Int>(Channel.UNLIMITED)
Multiple Producers and Consumers
One powerful feature of channels is the ability to have multiple coroutines producing and multiple coroutines consuming data concurrently.
Example: Multiple Producers & Consumers
fun main() = runBlocking {
val channel = Channel<Int>()
repeat(3) { // Three producers
launch {
for (i in 1..5) {
channel.send(i)
println("Producer $it sent: $i")
}
}
}
repeat(2) { // Two consumers
launch {
for (value in channel) {
println("Consumer $it received: $value")
}
}
}
delay(1000)
channel.close()
}
Closing Channels & Handling Exceptions
Closing a Channel
Closing a channel is essential to avoid memory leaks and indicate that no more elements will be sent.
channel.close()
After closing, any further attempts to send data result in an exception.
Handling Channel Exceptions Gracefully
When a receiver tries to collect from a closed channel, it should handle ClosedReceiveChannelException
.
try {
for (value in channel) {
println(value)
}
} catch (e: ClosedReceiveChannelException) {
println("Channel closed, no more data")
}
When to Use Channels vs. Flows?
Feature | Channels | Flows |
Multiple producers | โ Yes | ๐ซ No |
Multiple consumers | โ Yes | ๐ซ No |
Backpressure Handling | โ Yes | โ Yes |
Simpler API | ๐ซ No | โ Yes |
Cold Streams | ๐ซ No | โ Yes |
Use Channels when:
You need bidirectional communication.
There are multiple producers or consumers.
You need manual control over buffering and flow.
Use Flows when:
You need simple and reactive streams.
The stream is cold and starts when collected.
You prefer automatic backpressure handling.
Conclusion
Kotlin Coroutines Channels provide a powerful and safe way to handle concurrent data streams, ensuring efficient producer-consumer communication. While Flows are often the preferred choice for reactive data streams, Channels shine when you need fine-grained control, multiple producers/consumers, or real-time communication.
Subscribe to my newsletter
Read articles from Mouad Oumous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
