Redux VS Zustand : The Ultimate Beginner’s Guide

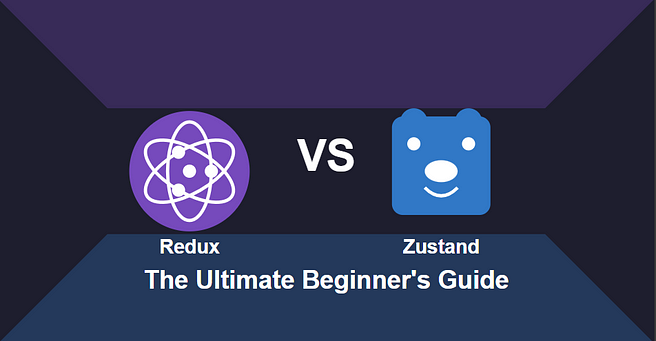
Introduction
State management is a crucial part of modern frontend development. If you’ve built applications with React, you’ve probably heard of Redux — one of the most popular state management libraries. However, Zustand has recently gained popularity for being simpler and more intuitive.
So, how do these two compare? Which one should you use? Let’s break it down in a way that even a child can understand!
What is State Management?
Imagine you are playing a game where you collect coins. The number of coins you collect needs to be stored somewhere so that it doesn’t reset every time you move to a new level.
State management in React is like a notebook that keeps track of your game’s progress. It helps you store and update information across different parts of your app.
Now, Redux and Zustand are two different ways of managing this notebook. Let’s see how they work!
What is Redux?
Redux is like a strict teacher who makes sure everything is organized. It follows a set of rules:
You store your data in a single object called the store.
To change data, you have to ask for permission using something called actions.
These actions are handled by reducers, which are like rule books that decide how the state should change.
How Redux Works
Imagine you have a piggy bank, and you want to add or remove coins.
Store → The piggy bank where coins are stored.
Action → A note saying “Add 10 coins” or “Remove 5 coins”.
Reducer → The rule book that says, “If the action says ‘add,’ increase the number of coins.”
Dispatch → A messenger that sends the action to the reducer.
Redux Code Example
import { createStore } from “redux”;
// Step 1: Define the initial state
const initialState = { count: 0 };
// Step 2: Create a reducer (rule book)
function counterReducer(state = initialState, action) {
switch (action.type) {
case “INCREMENT”:
return { count: state.count + 1 };
case “DECREMENT”:
return { count: state.count — 1 };
default:
return state;
}
}
// Step 3: Create the store
const store = createStore(counterReducer);
// Step 4: Dispatch actions (asking for state change)
store.dispatch({ type: “INCREMENT” });
console.log(store.getState()); // { count: 1 }
store.dispatch({ type: “DECREMENT” });
console.log(store.getState()); // { count: 0 }
🔴 Problem with Redux
Too much boilerplate (reducers, actions, dispatch).
More setup for simple state management.
Can be overkill for small projects.
What is Zustand?
Zustand is like a friendly notebook where you can just write down whatever you need, without needing a middleman (reducers, actions).
No boilerplate — just a simple function!
Direct updates — you don’t need reducers or actions.
Lightweight and fast — works with small and large projects.
How Zustand Works
Imagine you have a magic notebook where you can write and update your state directly.
Store → A notebook where you store your information.
State Updater → A simple function to update the notebook.
Zustand Code Example
import { create } from “zustand”;
// Step 1: Create the store
const useCounterStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count — 1 })),
}));
// Step 2: Use the store in a React component
function Counter() {
const { count, increment, decrement } = useCounterStore();
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>Increase</button>
<button onClick={decrement}>Decrease</button>
</div>
);
}
export default Counter;
🟢 Zustand Advantage Over Redux
Much simpler than Redux!
No reducers, no actions, just state and functions.
Differences B/W Redux and Zustand
When to Use Redux vs Zustand?
Use Redux if:
You are working on a large-scale app.
You need strict state management with middleware.
Your team already uses Redux.
Use Zustand if:
You want a simple, easy-to-use state manager.
You are working on small to medium-sized apps.
You don’t want extra boilerplate code.
Conclusion
Both Redux and Zustand are great tools, but Zustand is much simpler and easier to use for most projects. If you are a beginner, start with Zustand and learn Redux when you need more complex state management.
🔵 Zustand → Simple and fast!
🔴 Redux → Powerful but complex!
Subscribe to my newsletter
Read articles from Harshit Ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
