Learning JDBC: A Step-by-Step Introduction to Java Database Connectivity
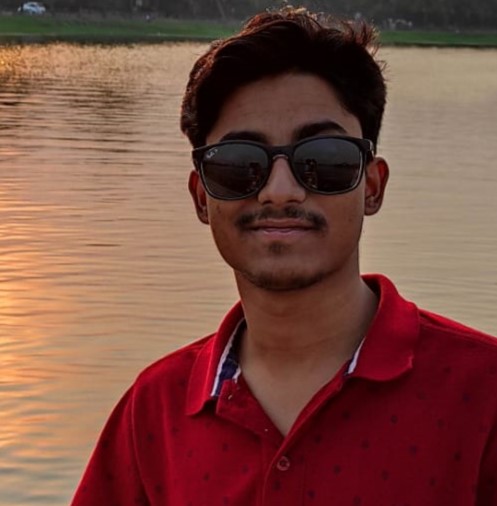
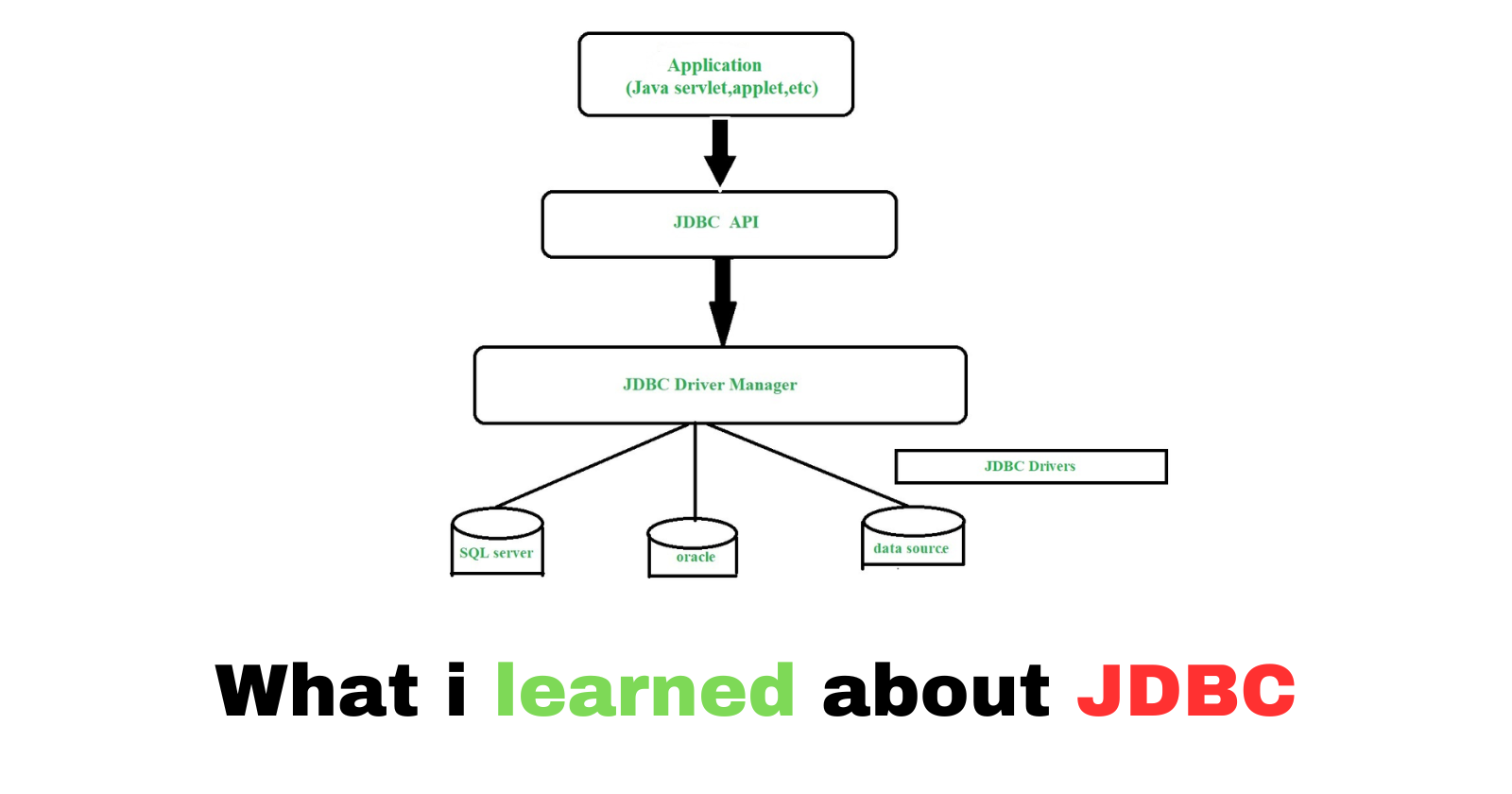
A few weeks ago, I started learning JDBC (Java Database Connectivity) to understand how Java interacts with databases. Coming from a JavaScript background, where I used ORMs like Mongoose in Node.js, JDBC initially felt low-level and manual, but as I delved deeper, I realized that JDBC gives me full control over database operations, offering both power and flexibility. If you're also learning JDBC, this guide will walk you through everything I learned, from setting up a connection to handling transactions, using Prepared Statements, and more. This is not an expert's guideβjust a learnerβs perspective on figuring things out. π
π Resources I used while learning JDBC:
πΊ YouTube Video 1 β Quick overview of JDBC
πΊ YouTube Playlist β In-depth JDBC tutorials
π GeeksForGeeks Article β Great written explanation
π» All my practice codes π GitHub Repo
1οΈβ£ What is JDBC? (My First Confusion π€―)
At first, I thought JDBC was some kind of ORM (like Mongoose or Hibernate), but it's actually just an API that allows Java applications to communicate with databases.
πΉ It helps Java interact with MySQL, PostgreSQL, Oracle, and other relational databases.
πΉ It provides methods for executing SQL queries directly from Java.
πΉ Unlike ORMs, JDBC doesn't abstract SQLβyou write raw SQL queries inside Java code.
How JDBC Works (Basic Flow)
Load JDBC Driver π οΈ β Allows Java to talk to a database.
Establish Connection π β Connect Java to MySQL, PostgreSQL, etc.
Execute Queries πΎ β Perform CRUD operations (Insert, Read, Update, Delete).
Process Results π β Read and use data from the database.
Close Connection πͺ β Free up resources.
Now that I understood the basics, my next step was to connect Java to MySQL using JDBC.
2οΈβ£ Setting Up JDBC β Connecting Java with MySQL
Before writing any Java code, I had to set up a database and install the JDBC driver.
πΉ Step 1: Install MySQL and Create a Database
First, I installed MySQL and created a simple database:
CREATE DATABASE student_db;
USE student_db;
CREATE TABLE students (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50),
age INT
);
πΉ Step 2: Add MySQL JDBC Driver to Java Project
To let Java talk to MySQL, I downloaded the MySQL JDBC Driver (Connector/J) and added it to my project.
For Maven projects, I added this dependency to pom.xml
:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.33</version>
</dependency>
πΉ Step 3: Establish a Connection in Java
Finally, I wrote this Java code to connect to MySQL:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/student_db";
String user = "root";
String password = "your_password";
try {
Connection conn = DriverManager.getConnection(url, user, password);
System.out.println("Connected to the database!");
conn.close(); // Always close the connection
} catch (SQLException e) {
System.out.println("Connection failed: " + e.getMessage());
}
}
}
π Success! My Java program was connected to MySQL.
3οΈβ£ Executing SQL Queries in JDBC
Once the connection was working, I learned how to execute SQL queries using JDBC. There are three main ways:
πΉ 1. Using Statement
(Basic but Risky)
This is the simplest way, but itβs vulnerable to SQL Injection (which I'll cover later).
import java.sql.*;
public class InsertData {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/student_db";
String user = "root";
String password = "your_password";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement()) {
String sql = "INSERT INTO students (name, age) VALUES ('John Doe', 22)";
stmt.executeUpdate(sql);
System.out.println("Data inserted successfully!");
} catch (SQLException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
β Works well, but not safe for user inputs.
4οΈβ£ PreparedStatement β Safe and Efficient β
Instead of writing SQL directly inside the Java code, PreparedStatement allows us to pass parameters safely.
import java.sql.*;
public class SafeInsert {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/student_db";
String user = "root";
String password = "your_password";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement("INSERT INTO students (name, age) VALUES (?, ?)")) {
pstmt.setString(1, "Alice");
pstmt.setInt(2, 21);
pstmt.executeUpdate();
System.out.println("Data inserted safely!");
} catch (SQLException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
β Prevents SQL Injection
β More readable and maintainable
5οΈβ£ Transaction Management β Avoiding Data Loss
JDBC supports transactions, which means we can execute multiple queries as a single unit. If one query fails, everything is rolled back to prevent partial changes.
πΉ Example: Handling Transactions
import java.sql.*;
public class TransactionExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/student_db";
String user = "root";
String password = "your_password";
try (Connection conn = DriverManager.getConnection(url, user, password)) {
conn.setAutoCommit(false); // Disable auto-commit
try (Statement stmt = conn.createStatement()) {
stmt.executeUpdate("UPDATE students SET age = 25 WHERE name = 'Alice'");
stmt.executeUpdate("DELETE FROM students WHERE name = 'Bob'");
conn.commit(); // Commit transaction
System.out.println("Transaction committed!");
} catch (SQLException e) {
conn.rollback(); // Rollback if any error occurs
System.out.println("Transaction rolled back!");
}
} catch (SQLException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
β Prevents half-executed queries in case of failure.
In a Nutshell π
β JDBC lets Java talk to databases via SQL queries.
β Basic flow: Load driver β Connect β Execute Queries β Close connection.
β Use PreparedStatement
instead of Statement
for security.
β Transactions help avoid data corruption.
β JDBC gives full control but requires writing raw SQL.
π Check out all my JDBC practice codes here π GitHub Repo
If you're also learning JDBC, let me know what confused you the most! ππ
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
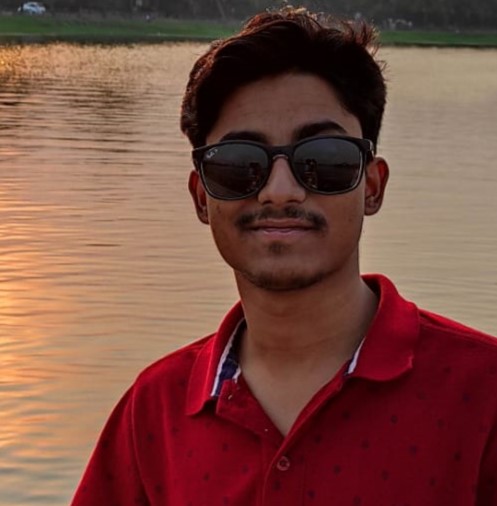
Arkadipta Kundu
Arkadipta Kundu
Iβm a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, Iβm focused on sharpening my DSA skills and expanding my expertise in Java development.