π₯ JavaScript Closures: A Simple Guide for Beginners π

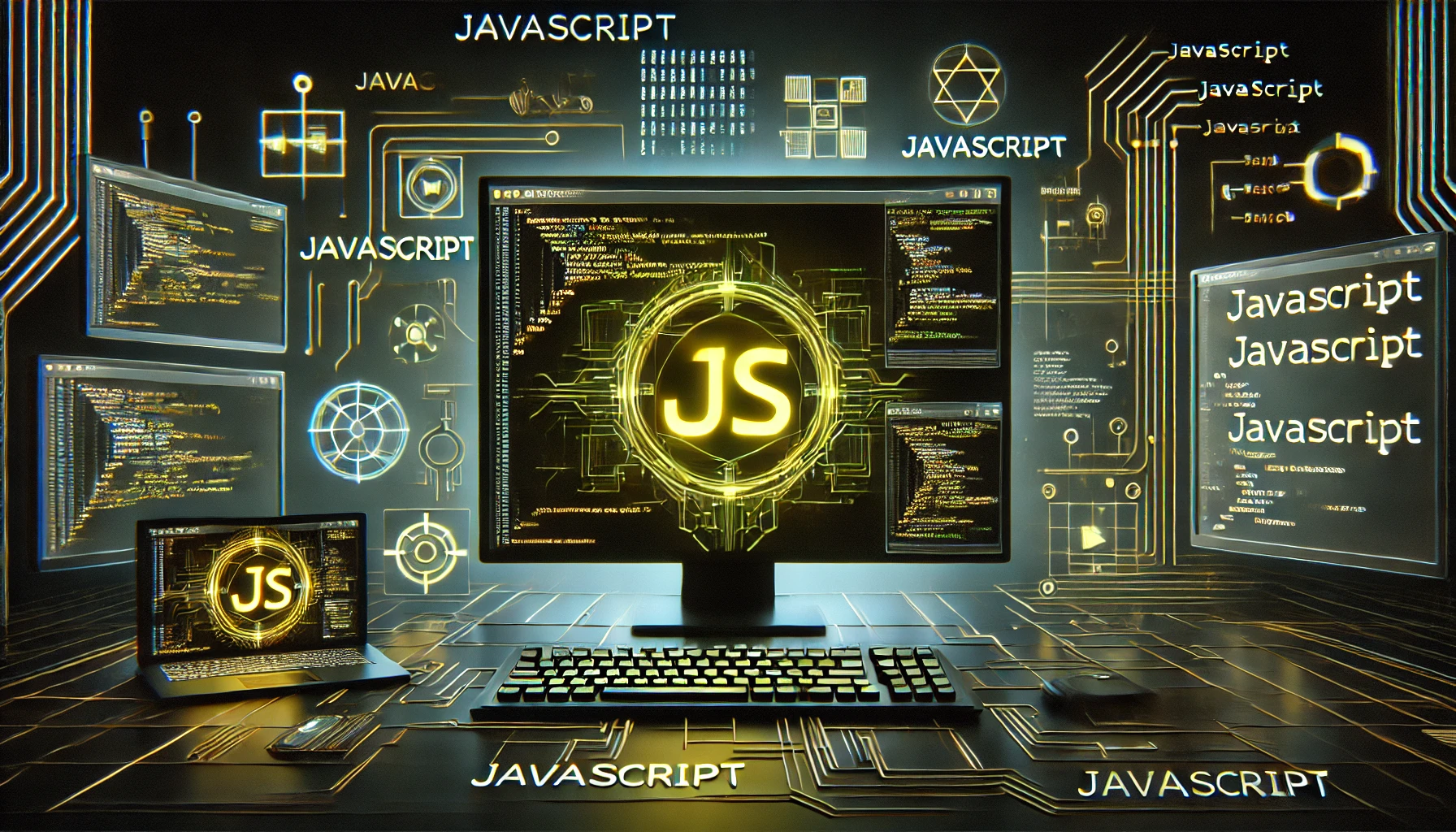
If you are learning JavaScript, then "Closures" is an important concept that you must understand. At first, it may seem a bit confusing, but once you get it, coding becomes much more interesting! Let's break it down with simple explanations and practical examples. π
π What is a Closure?
In JavaScript, a closure is a function that remembers the scope of its parent function, even after the parent function has executed. In simple terms, if a function inside another function accesses the parent functionβs variables, it forms a closure. π
π A Simple Example:
function outerFunction() {
let count = 0; // Variable inside the parent function
function innerFunction() {
count++;
console.log("Count: ", count);
}
return innerFunction;
}
const counter = outerFunction();
counter(); // Output: Count: 1
counter(); // Output: Count: 2
counter(); // Output: Count: 3
π§ Explanation:
outerFunction()
is a function with a variablecount
.innerFunction()
accessescount
, which is insideouterFunction()
.When
outerFunction()
is called, it returnsinnerFunction
.Every time
counter()
is called, thecount
value increases becauseinnerFunction
retains access tocount
via closure.
π‘ Why Are Closures Important?
β Data Encapsulation: Helps keep variables private. β State Management: Useful when a function needs to retain state. β Event Handlers & Callbacks: Essential for JavaScript events and asynchronous functions.
π― A Real-World Example: Button Click Counter
function createCounter() {
let count = 0;
return function () {
count++;
document.getElementById("countDisplay").innerText = "Clicks: " + count;
};
}
const buttonCounter = createCounter();
document.getElementById("myButton").addEventListener("click", buttonCounter);
This creates a button click counter that increases the count without using global variables! π₯
π Conclusion
Closures are a powerful feature in JavaScript that make your code more efficient and structured. If you understand them, you can write better and cleaner functional programs. β¨
If you have any questions or doubts, feel free to ask in the comments! Happy coding! ππ
π Next Topic: JavaScript Promises π€
Stay tuned for our next blog, where we will explore JavaScript Promises and how they help handle asynchronous operations efficiently! π
Subscribe to my newsletter
Read articles from Vivek varshney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vivek varshney
Vivek varshney
Full-Stack Web Developer | Blogging About Tech, React.js, Angular, Node.js & Web Development. Turning Ideas into Code & Helping Businesses Grow!