Shell Basics Guide

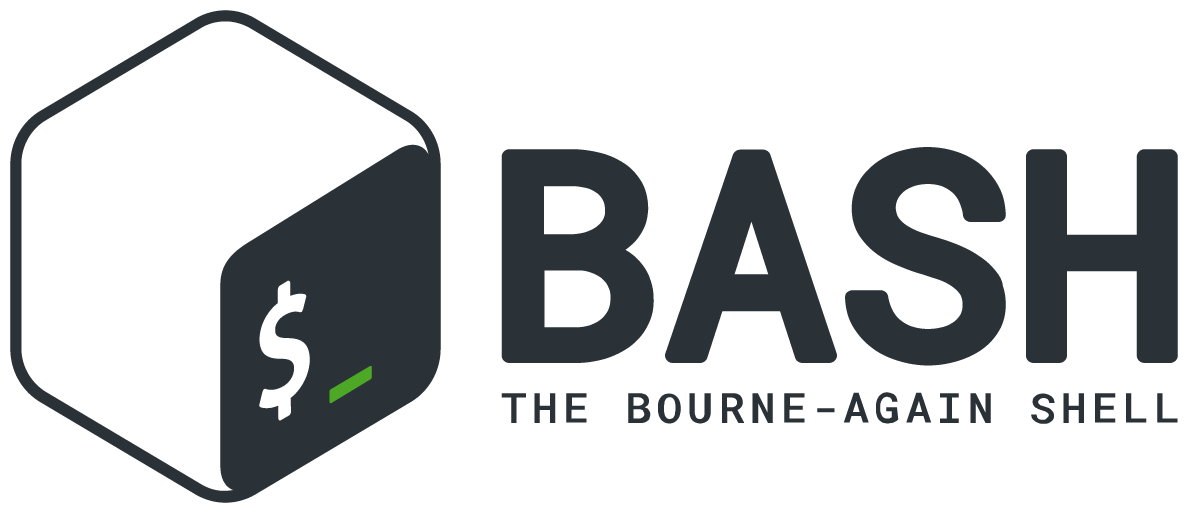
Introduction to Shells
A shell is a command-line interface that allows users to interact with the operating system. It serves as a bridge between the user and the system kernel, enabling command execution, scripting, and automation.
Shell | Description | Common OS |
Bash (bash) | Most widely used, default on many Linux distros | Linux/macOS (older) |
Zsh (zsh) | More advanced than Bash, customizable | macOS (default since Catalina), Linux |
Fish (fish) | User-friendly, auto-suggestions, colorful UI | Linux/macOS |
PowerShell (pwsh) | Object-oriented, designed for Windows | Windows |
Command Prompt (cmd) | Basic Windows shell | Windows |
Types of ShellsGetting Started with a Shell
Checking Your Current Shell
Run:
echo $SHELL
This returns something like /bin/bash or /bin/zsh.
Opening a Shell
Linux/macOS: Open Terminal (Ctrl + Alt + T on Linux, Cmd + Space -> Terminal on macOS).
Windows: Open Command Prompt (cmd), PowerShell, or WSL (for Linux-based shells).
Basic Shell Commands
Command | Description |
pwd | Print current directory |
ls | List files in a directory |
cd <directory> | Change directory |
mkdir <name> | Create a new directory |
rm <file> | Remove a file |
rmdir <dir> | Remove an empty directory |
touch <file> | Create an empty file |
cp <src> <dest> | Copy files |
mv <src> <dest> | Move/Rename files |
cat <file> | View file content |
echo "Hello" | Print output |
whoami | Show current user |
exit | Close the shell |
Shell Scripting Basics
A Shell script is a file containing a series of commands.
Creating a Simple Script
Create a new file:nano myscript.sh
Add the following:
#!/bin/bash
echo "Hello, World!"
Save and exit (Ctrl + X, then Y, then Enter).
Make the script executable: chmod +x myscript.sh
Run it:./myscript.sh
Working with Variables
#!/bin/bash
name="Deepak"
echo "Hello, $name!"
Conditional Statements
#!/bin/bash
if [ $1 -gt 10 ]; then
echo "Greater than 10"
else
echo "Less than or equal to 10"
fi
Loops in Shell
For Loop
for i in 1 2 3 4 5; do
echo "Number: $i"
done
While Loop
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
count=$((count+1))
done
Aliases and Shortcuts
To create a shortcut command, add an alias to your ~/.bashrc or ~/.zshrc:
alias ll="ls -lah"
Then apply changes:
source ~/.zshrc
Next Steps
Learn file permissions (chmod, chown)
Understand pipes (|), redirection (>, <, >>)
Explore environment variables (export VAR=value)
Use functions in scripts
Subscribe to my newsletter
Read articles from Deepak Prakash directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
