How PostgreSQL Transforms Your Query into a Parse Tree π³

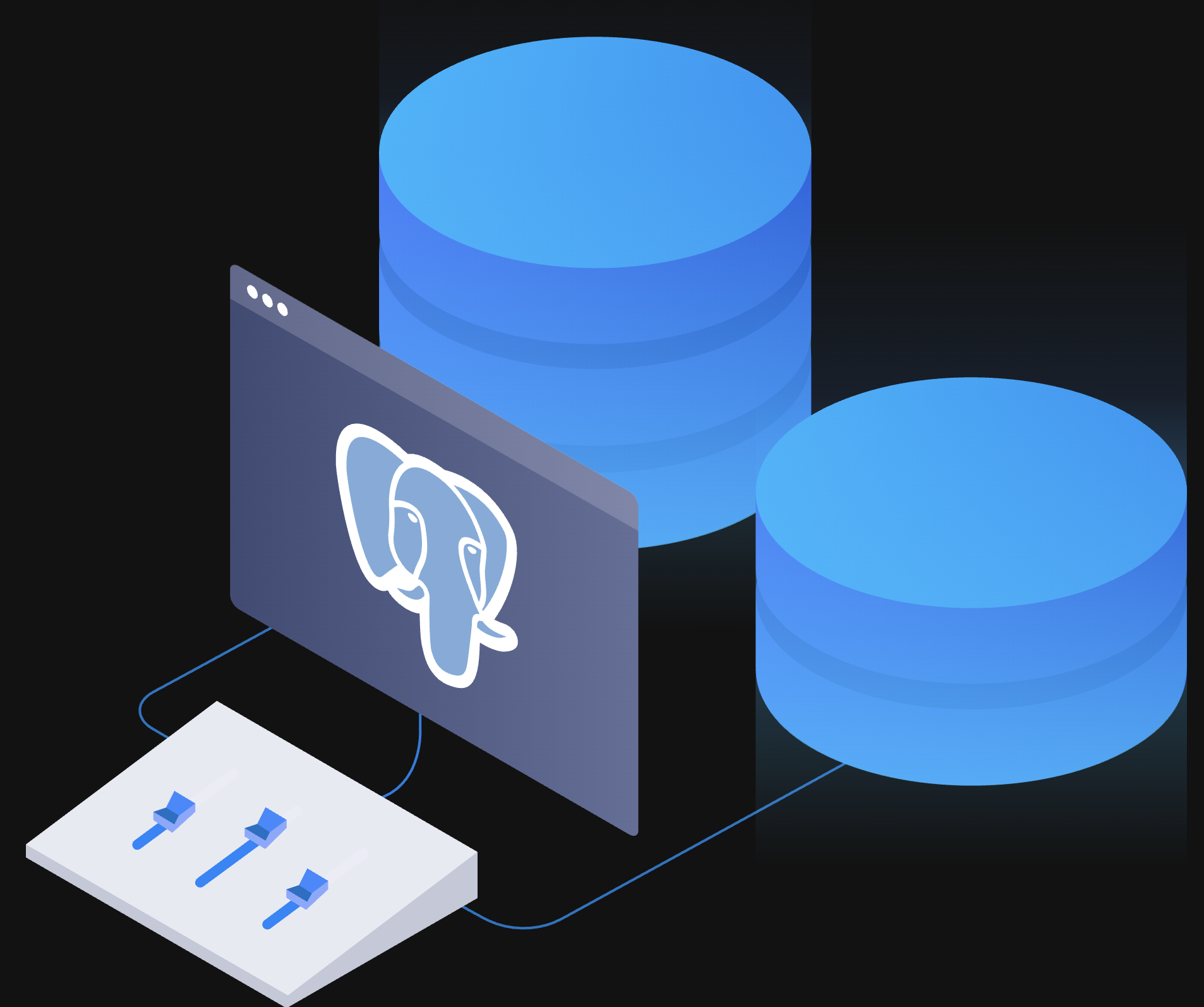
Ever wondered how PostgreSQL understands your SQL query before executing it? It doesnβt just βreadβ your query and magically return results. Instead, PostgreSQL goes through multiple steps to interpret, validate, and optimize your SQL query. π
One of the first and most crucial steps is Parsing, where PostgreSQL builds a Parse Tree to represent your SQL statement in a structured way. Let's break this down step by step! π
π The SQL Query
Consider this simple SQL query:
SELECT name, age FROM users WHERE age > 18;
Before PostgreSQL executes this, it needs to understand the query structure. Here's how it happens:
π Step 1: Lexical Analysis (Breaking Query into Tokens)
PostgreSQL first breaks down the SQL query into tokens:
SELECT
β a keywordname
,age
β column namesFROM
β a keywordusers
β table nameWHERE
β a keywordage
,>
,18
β condition elements
This step is like taking a sentence and breaking it into words.
π³ Step 2: Parsing (Building a Parse Tree)
Once PostgreSQL has the tokens, it structures them into a Parse Tree, which visually represents the SQL statement:
SelectStmt
βββ targetList
β βββ ColumnRef: name
β βββ ColumnRef: age
βββ fromClause
β βββ RangeVar: users
βββ whereClause
β βββ OpExpr: ">"
β β βββ ColumnRef: age
β β βββ Const: 18
Breaking it down:
SelectStmt
β The root node (this is aSELECT
query).targetList
β The columns being selected (name
,age
).fromClause
β The table being queried (users
).whereClause
β The filtering condition (age > 18
).OpExpr
β The operation (>
).ColumnRef
β The column (age
).Const
β The constant value (18
).
This structured representation allows PostgreSQL to process your query efficiently.
β Step 3: Semantic Analysis (Error Checking)
Before moving forward, PostgreSQL validates the query:
Does the
users
table exist?Do the
name
andage
columns exist?Is
SELECT *
a valid SQL syntax?
If everything checks out, the query moves to the next step. Otherwise, PostgreSQL throws an error.
β‘ Step 4: Query Planning & Optimization
Now, PostgreSQL decides the best way to execute the query:
Should it scan the whole table?
Should it use an index to find results faster?
Should it sort the data first?
These decisions impact query performance and speed.
π― Step 5: Execution (Getting the Data)
Finally, PostgreSQL executes the query and returns the result.
π‘ Why Should You Care?
Understanding the Parse Tree is useful for: β
Debugging: Helps in spotting errors in complex queries.
β
Performance: Query optimizations start after parsing.
β
Deeper DB Knowledge: Helps understand PostgreSQL internals better.
π οΈ Try It Yourself!
Want to see the Parse Tree for your queries? Run this command inside PostgreSQL:
EXPLAIN (VERBOSE, FORMAT JSON) SELECT name, age FROM users WHERE age > 18;
This will output the Parse Tree, showing exactly how PostgreSQL understands your query.
π Whatβs the most interesting thing youβve learned about PostgreSQL internals? Letβs discuss in the comments! π
Subscribe to my newsletter
Read articles from Eshaan Bagga directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
