How to Install and Import Modules in Python: A Step-by-Step Guide

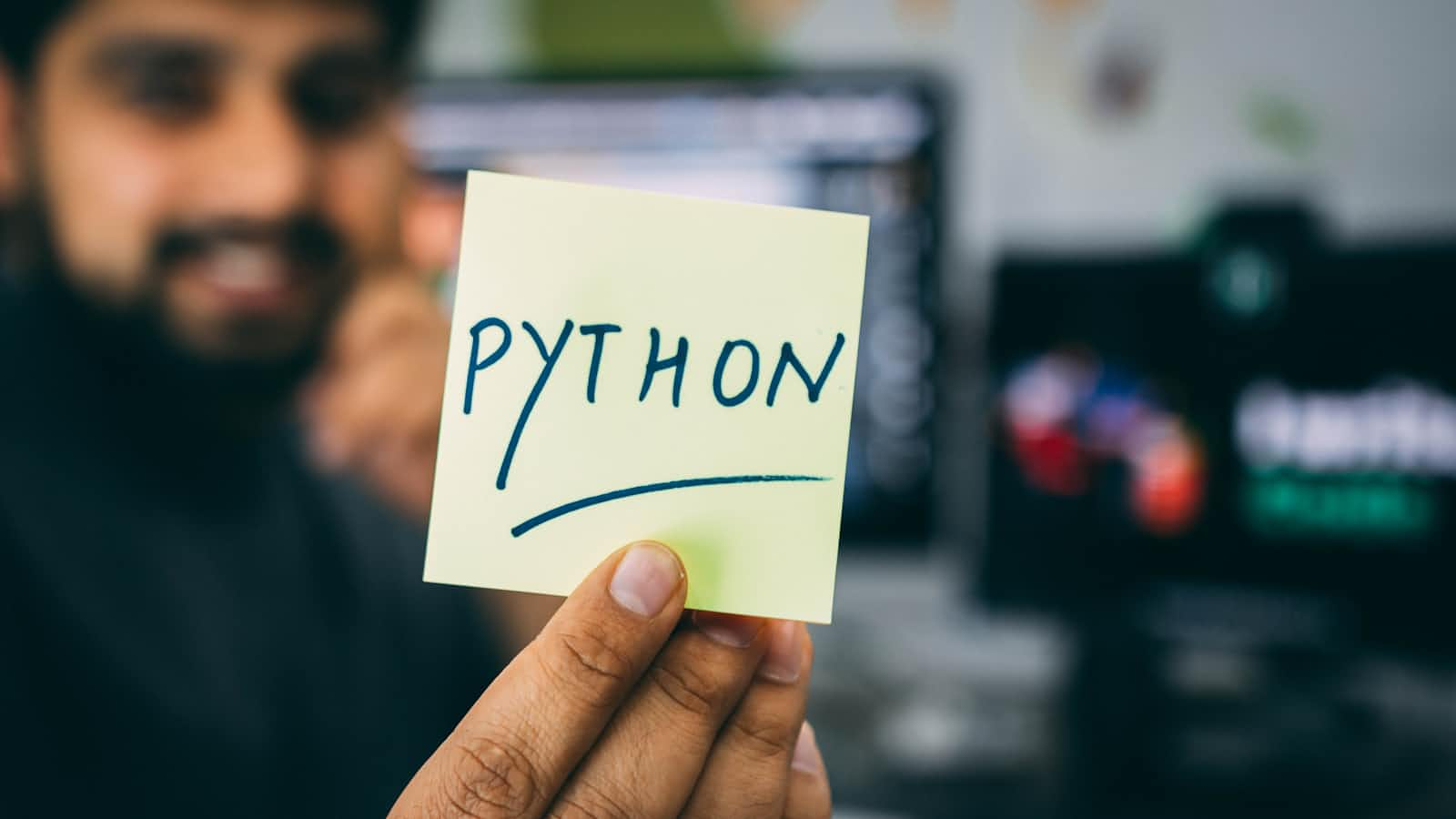
Python’s versatility stems from its vast ecosystem of modules and packages. These pre-written code libraries help developers streamline their workflow by providing ready-to-use functions and tools. In this guide, you’ll learn how to install and import modules in Python, along with troubleshooting common issues.
1. What Are Python Modules?
A module is a file containing Python code (functions, variables, or classes) that can be reused in other programs. Modules help organize code logically and promote reusability. For example:
The
math
module provides mathematical functions.The
requests
library simplifies HTTP requests.numpy
offers advanced numerical computing tools.
2. Installing Python Modules
Using pip (Python Package Installer)
Most third-party modules are installed using pip, Python’s default package manager. Follow these steps:
Step 1: Check if pip is Installed
Open a terminal (Command Prompt, PowerShell, or Terminal) and run:
pip --version
If pip isn’t installed, download it from pip.pypa.io.
Step 2: Install a Module
Use the following command to install any package (replace package-name
with the module name, e.g., requests
):
pip install package-name
Example:
pip install numpy
3. Importing Modules
Once installed, modules can be imported into your Python script or you can use in your code.
Importing Standard Library Modules
Python’s standard library (e.g., math
, os
, sys
) requires no installation:
import math
print(math.sqrt(25)) # Output: 5.0
4. Common Issues & Solutions
ModuleNotFoundError
Cause: The module isn’t installed or is misspelled.
Fix: Install it via
pip
or check the spelling.
5. Best Practices
Use Virtual Environments: Keep projects isolated.
Update Packages: Run
pip install --upgrade package-name
.Leverage Official Documentation: Check module docs for usage examples.
6. Conclusion
Mastering module installation and import techniques is essential for efficient Python development. By leveraging tools like pip
, virtual environments, and proper import syntax, you can harness the power of Python’s ecosystem. Start exploring popular libraries like pandas
, matplotlib
, and flask
to supercharge your projects!
Popular Modules to Try:
Data Science:
pandas
,numpy
,matplotlib
Web Development:
flask
,django
,requests
Automation:
selenium
,beautifulsoup4
Now go forth and code! 🐍✨
Subscribe to my newsletter
Read articles from Abhishek Pratap Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Pratap Singh
Abhishek Pratap Singh
DevOps Advocate | Passionate about bridging development & operations to build seamless, scalable systems. Let’s connect and geek out over DevOps, open-source, or the latest in cloud innovation! ✨ Building the future, one pipeline at a time.