Procedural Generation : Where Math Meets Magic in Game Development

Table of contents
- 🚀 Why Procedural Generation? The Ultimate Developer Superpower
- 🕹️ Level 1 : Where You’ve Seen This Wizardry
- 🎮 Game Spotlight
- 🔨 The Toolbox : 4 Core Techniques
- 1️⃣ Perlin Noise : The OG Terrain Builder
- 2️⃣ Cellular Automata: The Digital Ant Colony
- 3️⃣ Fractals & L-Systems: Nature’s CTRL+C, CTRL+V
- 4️⃣ Wave Function Collapse (WFC): The AI Artist
- 💥 The Dark Side: When Algorithms Rebel
- 1️⃣ The “Everything Looks Same-ish” Problem
- 2️⃣ Your GPU Hates You
- 3️⃣ Storytelling’s Kryptonite
- 4️⃣ The “Oops, That’s Impossible” Glitch
- 🌍 Beyond Games: Real-World Sorcery
- 🎥 Movies & CGI
- 🏗️ Architecture
- 🧠 AI Training
- 🛠️ Your Starter Kit
- 📚 Level Up Resources
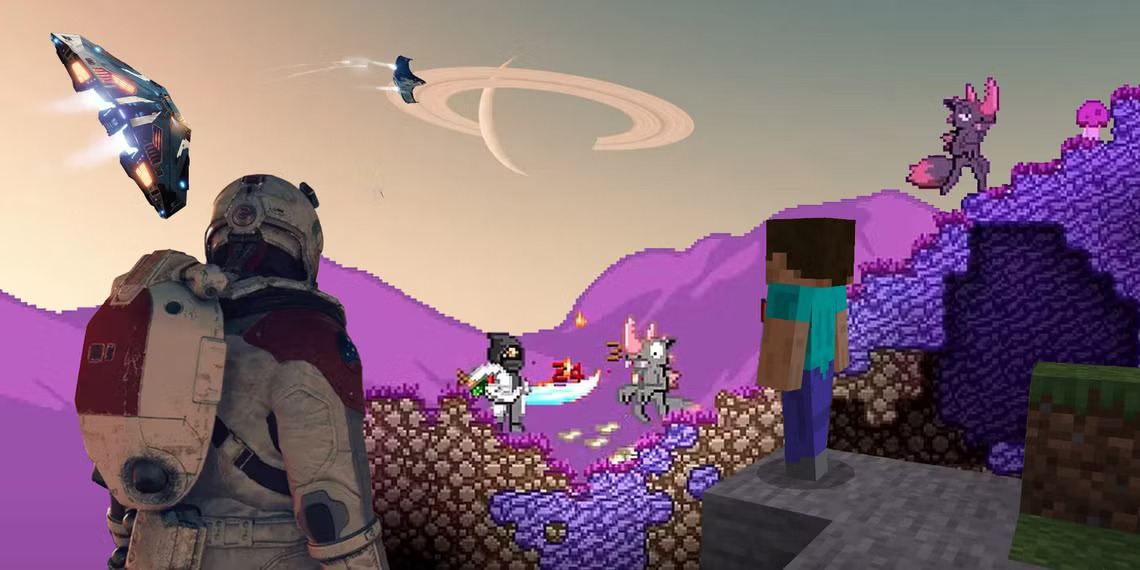
Ever stared at Minecraft’s endless landscapes or No Man’s Sky’s 18 quintillion planets and thought, “How the heck does this work?!” Buckle up—we’re diving into the secret sauce behind infinite worlds, smart algorithms, and why your GPU sometimes cries for mercy.
🚀 Why Procedural Generation? The Ultimate Developer Superpower
Imagine building a universe where every mountain, forest, and alien creature is unique—without hiring 10,000 artists. That’s procedural generation: teaching computers to “create” using math rules. It’s like giving a robot a paintbrush, but instead of colours, we use:
Noise algorithms (for natural-looking patterns)
Fractals (for infinite self-similar detail)
Seeds (tiny numbers that control randomness)
Games use this to save storage space (Minecraft’s world files are shockingly small!) and create endless surprises. Let’s break it down.
🕹️ Level 1 : Where You’ve Seen This Wizardry
🎮 Game Spotlight
Minecraft : Uses Perlin noise to generate terrain. Each block’s height is calculated via a math formula. Fun fact: A “seed” number (like “-4172144997902289642”) rebuilds the exact same world anywhere!
No Man’s Sky : Planets are built from 3D noise + rules (e.g., “If temperature < 0, spawn ice”). Their secret? All 18 quintillion planets share just 6MB of code!
Diablo 2 : Dungeons use grammar-based generation—like LEGO blocks snapping together via rules (e.g., “Boss rooms must have 3 exits”).
But how do these algorithms actually work? Let’s code!
🔨 The Toolbox : 4 Core Techniques
1️⃣ Perlin Noise : The OG Terrain Builder
Theory Time! 🌟
Perlin noise works by creating a gradient grid of random directions (like tiny arrows). For any point (x,y), it calculates how "aligned" it is with nearby gradients, then blends these values smoothly. The result? Natural-looking patterns that games use for heightmaps (elevation data).
The grandfather of natural patterns. Used in Minecraft, Roblox, and even CGI movies.
How it works:
Create a grid of random gradients (arrows pointing in random directions).
For any point (x,y), calculate the dot product with nearby gradients.
Smoothly interpolate between values.
# Generate 2D terrain with Python + noise library
import noise
import numpy as np
def generate_terrain(width, height, scale=50.0, octaves=6):
terrain = np.zeros((height, width))
for y in range(height):
for x in range(width):
terrain[y][x] = noise.pnoise2(
x/scale,
y/scale,
octaves=octaves, # Adds smaller details
persistence=0.5, # How much each octave matters
lacunarity=2.0 # Frequency multiplier per octave
)
return terrain
Tip : Add octaves=6
to create realistic mountains with large ridges and small rocks!
Dive into the simple grid based Perlin Noise Generator and create your own stunning landscapes now!
Another Perlin noise can be mapped to make hills and elevations of varying heights !
2️⃣ Cellular Automata: The Digital Ant Colony
Theory Time! 🐜
Imagine a grid of tiny "ants" (cells) that follow simple social rules:
Rule 1 : If 5+ neighbors are walls, stay a wall (safety in numbers!).
Rule 2 : If lonely (≤3 neighbors), become open space.
Why it’s genius: Just like real ants building colonies with no blueprint, these cells self-organize! Start with random noise (think TV static), apply the rules 5 times, and poof—chaos becomes connected caves.
Terraria uses this for its underground tunnels. Fewer iterations = jagged caves. More iterations = smooth corridors.
Rules:
Start with random walls (1) and empty space (0).
For each cell, count its 8 neighbors.
If ≥5 neighbors are walls, it becomes a wall. Repeat 5x.
def generate_cave(width=40, height=40, iterations=5):
# Initialize random grid (45% walls)
grid = [[1 if np.random.random() < 0.45 else 0 for _ in range(width)]
for _ in range(height)]
for _ in range(iterations):
new_grid = []
for y in range(height):
new_row = []
for x in range(width):
# Count neighbors (including self)
neighbors = sum(
grid[ny][nx]
for nx in range(max(0, x-1), min(width, x+2))
for ny in range(max(0, y-1), min(height, y+2))
)
# Rule: Become wall if 5+ neighbors
new_row.append(1 if neighbors >= 5 else 0)
new_grid.append(new_row)
grid = new_grid
return grid
Run this 5 times—watch random blobs turn into connected caves!
Jump into the Automata Generator and watch as random blobs magically morph into connected caves !
3️⃣ Fractals & L-Systems: Nature’s CTRL+C, CTRL+V
Theory Time! 🌿
Fractals are infinitely repeating patterns—like a broccoli floret where every tiny branch looks like the whole veggie! Here’s how they work:
The Recipe: Start with a shape (e.g., a line).
The Copy Machine: Replace parts of the shape with smaller copies of itself.
Repeat Forever: Each iteration adds finer details.
L-Systems take this further with grammar rules:
Start:
A
(a single branch)Rule: Replace
A
→A+B
,B
→A−B
After 3 iterations:
A+B+A−B+A+B−A−B
→ a complex tree!
Why gamers care : Want 10,000 unique trees? Write 5 rules. Want a galaxy? Write 10. Fractals let you clone complexity from simplicity!
# Recursive tree generator using Turtle
def draw_branch(length, angle, depth):
if depth == 0:
return
turtle.forward(length)
turtle.left(angle)
draw_branch(length * 0.7, angle, depth-1)
turtle.right(2 * angle)
draw_branch(length * 0.7, angle, depth-1)
turtle.left(angle)
turtle.backward(length)
turtle.speed(0)
turtle.left(90)
draw_branch(100, 30, 5) # Try depth=7 for dense trees!
Each branch splits into smaller copies—just like real plants!
Enter the Fractal Tree Generator and see your designs branch out into intricate patterns!
4️⃣ Wave Function Collapse (WFC): The AI Artist
Theory Time! 🧩
WFC works like Sudoku. Each tile has constraints (e.g., "roads must connect"). The algorithm collapses possibilities one cell at a time, respecting neighbours. It’s constraint satisfaction—noise with rules!
Used in Townscaper and Bad North for coherent villages.
How it works:
Define tiles (e.g., “road”, “house”) and their compatible neighbours.
Start with a blank grid.
Collapse each cell’s possibilities based on neighbours.
# Simplified WFC example
tiles = {
"water": {"up": ["water", "sand"], "down": ["water"]},
"sand": {"up": ["grass"], "down": ["water"]},
"grass": {"down": ["sand"]}
}
def collapse_cell(grid, x, y):
possible_tiles = list(tiles.keys())
# Check neighbors (left/top)
if x > 0:
left_tile = grid[y][x-1]
possible_tiles = [t for t in possible_tiles
if t in tiles[left_tile]["right"]]
if y > 0:
top_tile = grid[y-1][x]
possible_tiles = [t for t in possible_tiles
if t in tiles[top_tile]["down"]]
# Pick randomly from remaining options
grid[y][x] = np.random.choice(possible_tiles)
WFC ensures beaches (sand) always sit between water and grass!
💥 The Dark Side: When Algorithms Rebel
1️⃣ The “Everything Looks Same-ish” Problem
No Man’s Sky’s 2016 launch taught us this: If all icy planets use the same noise parameters, players see repeating ice spikes. Why? Algorithms lack context—they don’t know a “cool mountain” from a “boring hill”.
Dev fix : Mix multiple noise layers (e.g., Perlin for shape + Cellular algorithms for cracks) and sprinkle handcrafted landmarks (e.g., a giant alien skeleton every 10 planets).
2️⃣ Your GPU Hates You
Procedural generation on-the-fly can melt weaker hardware. Imagine generating Elden Ring’s entire map while players explore—your GPU would burst into flames!
Solutions :
Chunking: Like Minecraft, generate the world in 16x16 blocks.
Pre-baking: Generate textures/geometry during loading screens.
Level of Detail (LOD): Show low-poly mountains from afar, add details up close.
3️⃣ Storytelling’s Kryptonite
Algorithms can’t write The Last of Us. Most procedural quests end up as:
1. Go to [RANDOM LOCATION]
2. Kill [RANDOM NUMBER] [RANDOM ENEMY]
3. Return to [RANDOM NPC]
Why? Stories need intentionality—something RNG can’t provide.
Dev workaround : Do what Hades did:
Handwrite 1000+ dialogue lines
Use procedural context (e.g., “If player dies to lava, NPC mocks them”)
4️⃣ The “Oops, That’s Impossible” Glitch
Procedural dungeons can accidentally create unbeatable rooms (e.g., a key behind a locked door). Dwarf Fortress once spawned whales on mountains—they suffocated immediately.
Debugging hell : You can’t test every possible seed! Most devs use algorithmic safeguards:
if dungeon_room.has_key:
ensure_door_unlocked()
🌍 Beyond Games: Real-World Sorcery
🎥 Movies & CGI
Avatar’s Pandora: Mixed procedural forests with hero trees painted by artists.
Lord of the Rings’ Helm’s Deep Battle: Used Massive Software to simulate 10,000+ Uruk-hai with autonomous AI agents. Each orc had simple rules:
“Find Allies”
“Attack Nearest Human”
“Flee if Surrounded”
Result? A chaotic battle that looked hand-animated!
🏗️ Architecture
Procedural skyscrapers: Input “floor count, style, material” → get 100 variants.
CityEngine: Software for generating entire cities for urban planning.
🧠 AI Training
- Generate infinite training data: random roads for self-driving cars, fake tumors for medical AI.
🛠️ Your Starter Kit
Noise Libraries :
FastNoiseLite
(C#/Unity),noise
(Python)Engines : Try Unity’s Procedural Toolkit or Unreal’s PCG plugin.
First Project : A “roguelike dungeon” with cellular automata (2D grid + ASCII art!).
Remember: Start small. Your first planet can be a noisy sphere—no one’s judging!
📚 Level Up Resources
Book: Procedural Generation in Game Design by Tanya Short (for design philosophy)
Tutorial: Procedural Landmass Generation by Sebastian Lague
Tool: Wave Function Collapse GitHub
Now go make something that’ll make Notch proud! 🚀
Subscribe to my newsletter
Read articles from Mriganka Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
