FPGAs Part III - Final
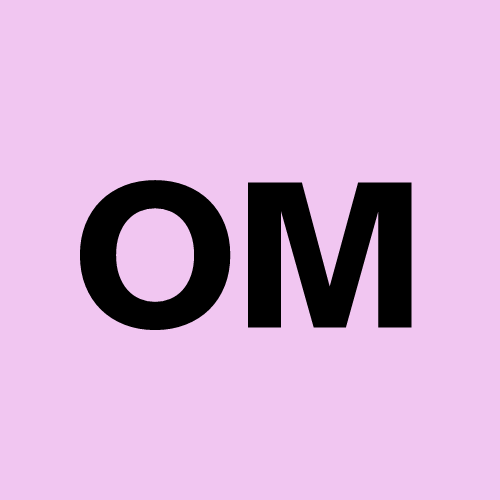
Prerequisites - Execute steps in Part II
After install - You may have to restart your runtime (if errors occur) and execute the following:
# Basic setup steps
pip install openvino-dev
pip install numpy tensorflow torch
Note: You must execute Step 1 in FPGAs Part II before proceeding:
# Simple OpenVINO-FPGA vision pipeline
from openvino.runtime import Core
import cv2
def create_vision_pipeline():
ie = Core()
model = ie.read_model("vision_model.xml")
compiled = ie.compile_model(model, "FPGA")
return compiled
# Initialize webcam and run the pipeline
cap = cv2.VideoCapture(0) # 0 for the primary camera
model = create_vision_pipeline()
while True:
ret, frame = cap.read()
if not ret:
break
# Preprocess frame as required by your model
# For example, resizing to model's input size
processed_frame = cv2.resize(frame, (224, 224)) # Adjust size
processed_frame = processed_frame.transpose(2, 0, 1) # Channels first
processed_frame = processed_frame.reshape(1, 3, 224, 224)
# Run inference
results = model([processed_frame])[0]
# Example: Display results (customize based on your use case)
print("Inference Results:", results)
cv2.imshow("Webcam Feed", frame)
if cv2.waitKey(1) & 0xFF == ord("q"): # Press 'q' to quit
break
cap.release()
cv2.destroyAllWindows()
#END
Boom! You did it!
You used an emerging FPGA framework to unlock a world of possibilities. Computational power could not be more accessible for the dreamers, disrupters, pioneers and next-gen of inference engineers.
“May your models converge and your deadlines be generous.” - Claude 3.5 Sonnet
Comparison with Traditional ML Acceleration
Feature | FPGA (OpenVINO) | GPU (CUDA) | CPU |
Setup Complexity | Medium | Low | Low |
Performance | High for specific tasks | General high performance | Baseline |
Power Efficiency | Excellent | Moderate | Moderate |
Flexibility | Highly configurable | Fixed architecture | Fixed architecture |
Development Time | Longer | Quick | Quick |
Common Pitfalls to Avoid
Resource Overallocation
FPGAs have limited resources
Monitor utilization
Use profiling tools
Performance Bottlenecks
Check data transfer overhead
Optimize I/O operations
Consider pipeline parallelism
Start with pre-optimized models
Use quantization when possible
Monitor FPGA resource usage
Batch processing for better throughput
Guide Summary:
This guide provides a practical entry-level path for ML engineers to start working with FPGAs, focusing on actual implementation rather than just theory. In the article we took a step-by-step approach to setting up an OpenVINO-FPGA vision pipeline for machine learning applications. It covers basic installation, webcam initialization, and running an inference model using OpenVINO with FPGA acceleration. The guide highlights the advantages of using FPGAs over traditional GPUs and CPUs, emphasizing high performance, power efficiency, and configurability for specific AI tasks. It also includes optimization tips, pitfalls to avoid, and the unique benefits of FPGAs for edge computing and real-time processing.
Remember: While FPGAs require more initial setup than GPUs, they offer unique advantages for specific AI applications, especially in edge computing and real-time processing scenarios. The key is to begin with high-level tools like OpenVINO and gradually move to more complex optimizations as needed.
Subscribe to my newsletter
Read articles from Omar Morales directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
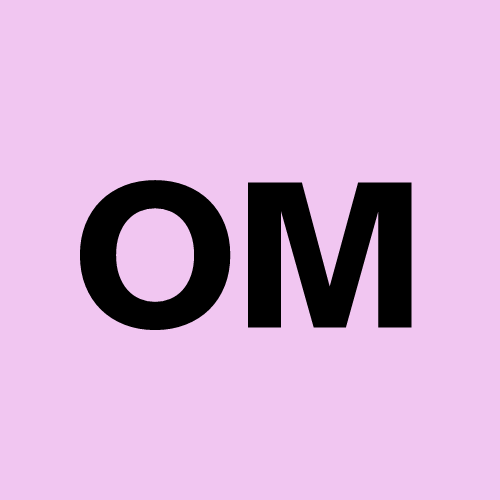
Omar Morales
Omar Morales
Driving AI Innovation, Cloud Observability, and Scalable Infrastructure - Omar Morales is a Machine Learning Engineer and SRE Leader with over a decade of experience bridging AI-driven automation with large-scale cloud infrastructure. His work has been instrumental in optimizing observability, predictive analytics, and system reliability across multiple industries, including logistics, geospatial intelligence, and enterprise cloud services. Omar has led ML and cloud observability initiatives at Sysco LABS, where he has integrated Datadog APM for performance monitoring and anomaly detection, cutting incident resolution times and improving SLO/SLI compliance. His work in infrastructure automation has reduced cloud provisioning time through Terraform and Kubernetes, making deployments more scalable and resilient. Beyond Sysco LABS, Omar co-founded SunCity Greens, a small and local AI-powered agriculture and supply chain analytics indoor horticulture farm that leverages predictive modeling to optimize farm-to-market logistics serving farm-to-table chefs and eateries. His AI models have successfully increased crop yield efficiency by 30%, demonstrating the real-world impact of machine learning on localized supply chains. Prior to these roles, Omar worked as a Geospatial Applications Analyst Tier 2 at TELUS International, where he developed predictive routing models using TensorFlow and Google Maps API, reducing delivery times by 20%. He also has a strong consulting background, where he has helped multiple enterprises implement AI-driven automation, real-time analytics, ETL batch processing, and big data pipelines. Omar holds multiple relevant certifications and is on track to complete his Postgraduate Certificate (PGC) in AI & Machine Learning from the Texas McCombs School of Business. He is deeply passionate about AI innovation, system optimization, and building highly scalable architectures that drive business intelligence and automation. When he’s not working on AI/ML solutions, Omar enjoys virtual reality sim racing, amateur astronomy, and building custom PCs.