AWS CDK First Try
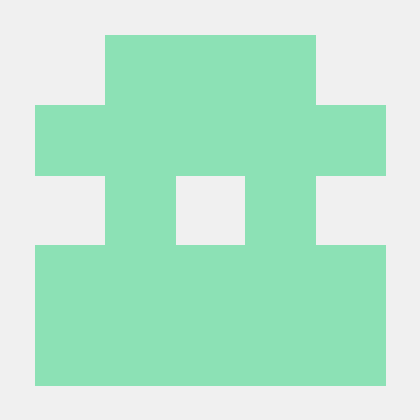
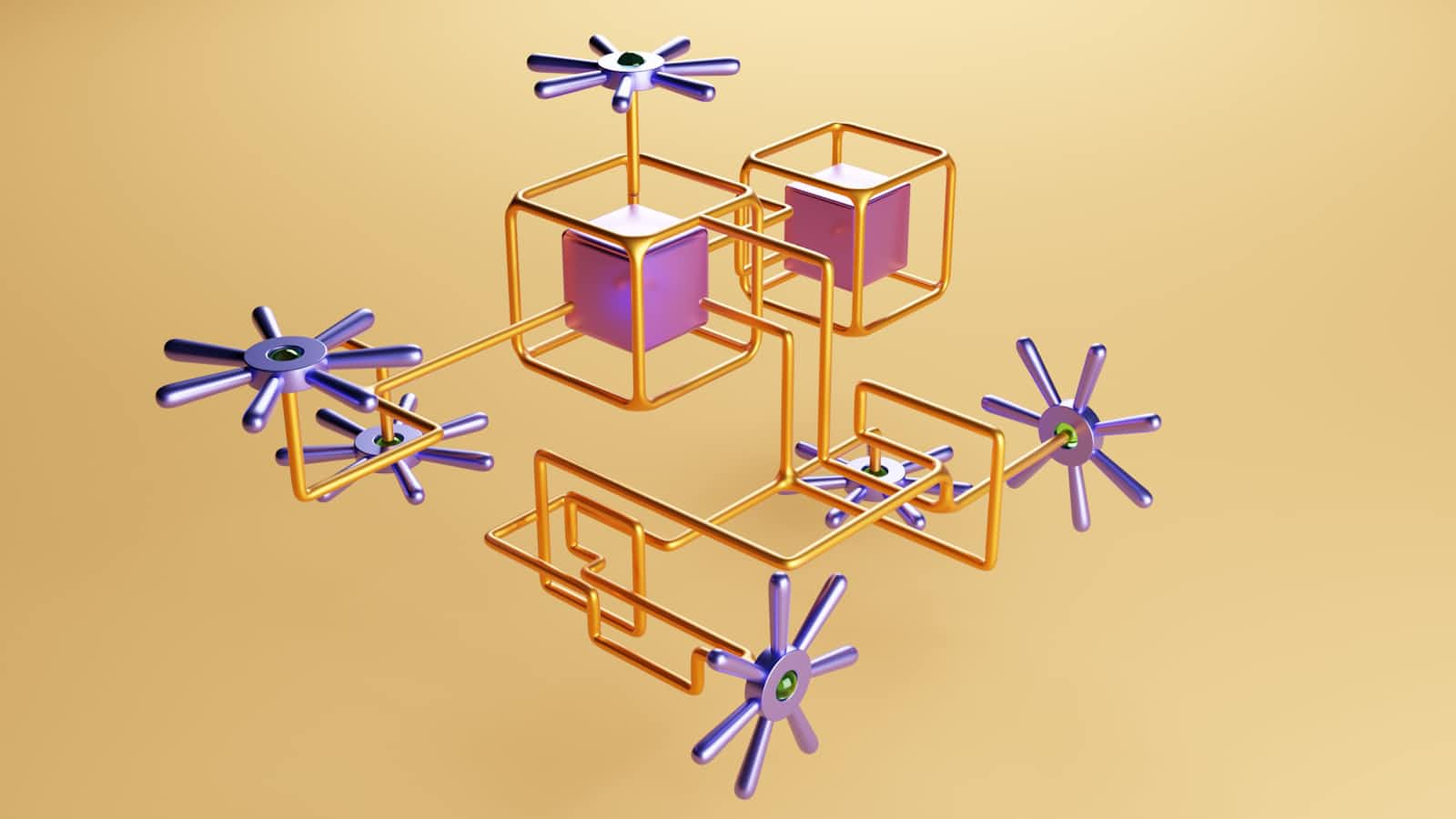
The AWS CDK really surprised me. Using only code, I was able to quickly and easily create a simple AWS serverless API.
I have created simple ToDo API that allows basic CRUD operations.
How to start with AWS CDK?
First you need to install AWS CLI. Here you could find how to do that https://docs.aws.amazon.com/cli/latest/userguide/getting-started-install.html
Once the AWS CLI is installed, it's time to set it up.
https://docs.aws.amazon.com/cli/latest/userguide/getting-started-quickstart.html
When AWS CLI is installed and configured, time to install with AWS CDK.
npm install -g aws-cdk
Now time to play with AWS CDK project. In your choosen path invoke following commands.
We are going to work with Java project so please install Java JDK and Maven library on your machine first.
mkdir TodoCdkProject && cd TodoCdkProject
cdk init app --language java
The second, CDK one command creates for us AWS CDK project using Java language.
Project I have created could be found here
https://github.com/jrsokolow/aws-serverless-todo-api
You could see that it is maven based project with pom.xml file already created
In pom.xml we already have dependencies required to work with AWS CDK.
Additionally in src directory we already have two classes created
TodoApiStack.java where we define AWS stack e.g DynamoDB, Lambda, API Gateway
TodoCdkApp.java where we are instantiating app and stack to run the project
The cdk.json file is a configuration file at the root of your AWS CDK project. It tells the CDK CLI how to run your application and can also include context values that customize your stack's behavior. Key aspects include:
App Command:
The file typically contains an"app"
property that specifies the command to execute your CDK app. For example:{ "app": "mvn -e -q compile exec:java", }
Now it is good time to install all project dependencies
mvn clean install
Let’s see the code now
First we will see TodoCdkApp class, please check comments which explains code
package com.myorg;
import software.amazon.awscdk.App;
public class TodoCdkApp {
public static void main(final String[] args) {
App app = new App(); //instantiates AWS CDK App
new TodoApiStack(app, "TodoApiStack"); //instantiates stack class. In constructor passing app and stack id
app.synth(); //converts your CDK code into a deployable CloudFormation template
}
}
Let’s check now TodoApiStack class
package com.myorg;
import software.amazon.awscdk.App;
import software.amazon.awscdk.Duration;
import software.amazon.awscdk.Stack;
import software.amazon.awscdk.StackProps;
import software.amazon.awscdk.services.dynamodb.Attribute;
import software.amazon.awscdk.services.dynamodb.AttributeType;
import software.amazon.awscdk.services.dynamodb.BillingMode;
import software.amazon.awscdk.services.dynamodb.Table;
import software.amazon.awscdk.services.lambda.Code;
import software.amazon.awscdk.services.lambda.Function;
import software.amazon.awscdk.services.lambda.Runtime;
import software.amazon.awscdk.services.apigateway.LambdaRestApi;
//First important thing we are extending here AWS CDK Stack class
public class TodoApiStack extends Stack {
public TodoApiStack(final App scope, final String id) {
this(scope, id, null);
}
//In constructor we are defining what AWS services we want to use
public TodoApiStack(final App scope, final String id, final StackProps props) {
super(scope, id, props);
// Create a DynamoDB table to store TODO items.
// DynamoDB is NoSQL database so we don't have to define whole table schema
// Below we are defining only partition key and that's it
// We could put into table todo items of what ever structure
Table todoTable = Table.Builder.create(this, "TodoTable")
.tableName("TodoTable")
//here we are defining billing method!!!!
.billingMode(BillingMode.PAY_PER_REQUEST)
.partitionKey(Attribute.builder()
.name("id")
.type(AttributeType.STRING)
.build())
.build();
// Create a Lambda function to handle CRUD operations.
Function todoLambda = Function.Builder.create(this, "TodoLambda")
//Defining Java version
//Important to build project with the same Java version
//As the one used to run project in AWS
.runtime(Runtime.JAVA_17)
.handler("com.myorg.TodoHandler::handleRequest")
// Path to your built Lambda jar
.code(Code.fromAsset("target/todo-api-lambda-0.1.jar"))
// when testing project in AWS I had to add this line to avoid timeouts
.timeout(Duration.seconds(10))
.build();
// Grant the Lambda function read/write permissions on the DynamoDB table.
todoTable.grantReadWriteData(todoLambda);
// Create an API Gateway REST API backed by the Lambda function.
LambdaRestApi api = LambdaRestApi.Builder.create(this, "TodoApi")
.handler(todoLambda)
.restApiName("Todo Service")
.build();
}
}
Now let’s see lamba handler implementation
package com.myorg;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder;
import com.amazonaws.services.dynamodbv2.document.DynamoDB;
import com.amazonaws.services.dynamodbv2.document.Item;
import com.amazonaws.services.dynamodbv2.document.ItemCollection;
import com.amazonaws.services.dynamodbv2.document.ScanOutcome;
import com.amazonaws.services.dynamodbv2.document.Table;
import com.amazonaws.services.dynamodbv2.document.spec.ScanSpec;
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
import com.fasterxml.jackson.databind.ObjectMapper;
public class TodoHandler implements RequestHandler<Map<String, Object>, ApiResponse> {
private static final String TABLE_NAME = "TodoTable";
// Create a DynamoDB client and instantiate a DynamoDB object.
private static final AmazonDynamoDB client = AmazonDynamoDBClientBuilder.standard().build();
private static final DynamoDB dynamoDB = new DynamoDB(client);
private static final Table table = dynamoDB.getTable(TABLE_NAME);
@Override
public ApiResponse handleRequest(Map<String, Object> input, Context context) {
// Log the received event (you can see it in CloudWatch logs).
context.getLogger().log("Received event: " + input);
// Determine the HTTP method and route from the input.
String httpMethod = (String) input.get("httpMethod");
// Based on the HTTP method, call different functions.
switch (httpMethod) {
case "GET":
// Return list of TODOs or a specific TODO.
return handleGet(context);
case "POST":
// Create a new TODO.
return handlePost(context, input);
case "PUT":
// Update an existing TODO.
return handlePut(input);
case "DELETE":
// Delete a TODO.
return handleDelete(input);
default:
return new ApiResponse(400, "Unsupported HTTP method");
}
}
// Sample implementations (to be fleshed out with actual DynamoDB logic)
private ApiResponse handleGet(Context context) {
List<Map<String, Object>> itemsAsMap = new ArrayList<>();
try {
// Scan the table for all items.
ScanSpec scanSpec = new ScanSpec();
ItemCollection<ScanOutcome> itemsCollection = table.scan(scanSpec);
for (Item item : itemsCollection) {
itemsAsMap.add(item.asMap());
}
} catch (Exception e) {
context.getLogger().log("Failed to scan table: " + e.getMessage());
return new ApiResponse(500, "Failed to fetch items: " + e.getMessage());
}
// Convert the list of item maps to JSON.
ObjectMapper mapper = new ObjectMapper();
String jsonResponse;
try {
jsonResponse = mapper.writeValueAsString(itemsAsMap);
} catch (Exception e) {
context.getLogger().log("Failed to convert items to JSON: " + e.getMessage());
return new ApiResponse(500, "Error converting items to JSON: " + e.getMessage());
}
return new ApiResponse(200, jsonResponse);
}
private ApiResponse handlePost(Context context, Map<String, Object> input) {
// Extract the body from the input event
String body = (String) input.get("body");
if (body == null || body.isEmpty()) {
return new ApiResponse(400, "Missing request body");
}
// Use Jackson ObjectMapper to parse the JSON payload
ObjectMapper mapper = new ObjectMapper();
Map<String, Object> payload;
try {
payload = mapper.readValue(body, Map.class);
} catch (Exception e) {
return new ApiResponse(400, "Invalid JSON in request body: " + e.getMessage());
}
// Validate required fields (e.g., title)
String title = (String) payload.get("title");
if (title == null || title.isEmpty()) {
return new ApiResponse(400, "Missing or empty 'title' field");
}
String description = (String) payload.get("description");
// Generate a unique ID for the new TODO item
String id = java.util.UUID.randomUUID().toString();
try {
// Build the new item and insert it into the table
Item newItem = new Item()
.withPrimaryKey("id", id)
.withString("title", title)
.withString("description", description != null ? description : "")
.withBoolean("status", false);
table.putItem(newItem);
} catch (Exception e) {
return new ApiResponse(500, "Error inserting item: " + e.getMessage());
}
// Build the response with the created item details
Map<String, Object> responseMap = new java.util.HashMap<>();
responseMap.put("id", id);
responseMap.put("title", title);
responseMap.put("description", description);
String jsonResponse;
try {
jsonResponse = mapper.writeValueAsString(responseMap);
} catch (Exception e) {
return new ApiResponse(500, "Error generating JSON response: " + e.getMessage());
}
return new ApiResponse(201, jsonResponse);
}
private ApiResponse handlePut(Map<String, Object> input) {
// TODO: Implement PUT logic to update a TODO item
return new ApiResponse(200, "PUT method called");
}
private ApiResponse handleDelete(Map<String, Object> input) {
// TODO: Implement DELETE logic to remove a TODO item
return new ApiResponse(200, "DELETE method called");
}
}
The handlePut and handleDelete methods still need implementation. Feel free to copy my repo and implement these.
This is almost all code required to implement simple ToDo API working well on AWS.
Now time to deploy that project on AWS.
To do that we need to invoke following commands
# install all project dependencies defined in pom.xml file
mvn clean install
# builds whole app into target directory
mvn clean package
# automatically executes your app (which includes the app.synth() call) to generate the CloudFormation template
cdk synth
# deploying your CDK application to AWS
cdk deploy
After these commands execution our ToDo API should be available and ready to be tested via rest client.
Example cURL request to add ToDo item
curl -X POST \
'https://<your-api-id>.execute-api.<region>.amazonaws.com/prod' \
--header 'Accept: */*' \
--header 'User-Agent: Thunder Client (https://www.thunderclient.com)' \
--header 'Content-Type: application/json' \
--data-raw '{
"title": "today task",
"description": "clean car"
}'
Example cURL request to list ToDo items
curl -X GET \
'https://<your-api-id>.execute-api.<region>.amazonaws.com/prod' \
--header 'Accept: */*' \
--header 'User-Agent: Thunder Client (https://www.thunderclient.com)'
As our serverless API is not secured and available publicly worth to destroy it at the end using this simple command
cdk destroy <stack-name>
Thanks to this command, we can observe our lambda logs without using CloudWatch. It's a very convenient way to investigate issues during development.
new TodoApiStack(app, "TodoApiStack");
Another useful command is this one
aws logs tail /aws/lambda/<lambda-id> --follow
Thanks to this command, we can observe our lambda logs without using CloudWatch. It's a very convenient way to investigate issues during development.
To summarize, a clear advantage is how quickly I can build and deploy a serverless API using just code. What I don't like is the tight coupling to AWS libraries. Specifically, in TodoHandler, I am surprised that I have to use AWS classes to add and read to-do list items from a DynamoDB table. If we switch to another cloud provider, the code would need to be completely rewritten.
Subscribe to my newsletter
Read articles from Jakub Sokolowski directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
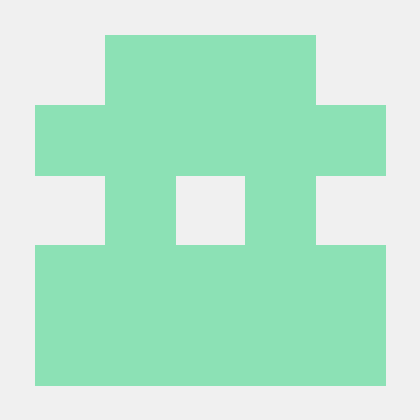