Navigating Next.js: Choosing Between Link and useRouter for Optimal Performance

Table of contents
- Introduction
- The Evolution of Client-Side Navigation in Next.js
- Deep Dive: The Link Component
- Deep Dive: The useRouter() Hook
- Strategic Decision Framework: When to Use Each Approach
- Real-World Implementation Patterns
- Performance Considerations
- Best Practices for Production Applications
- Conclusion: The Strategic Approach
- What's Next?
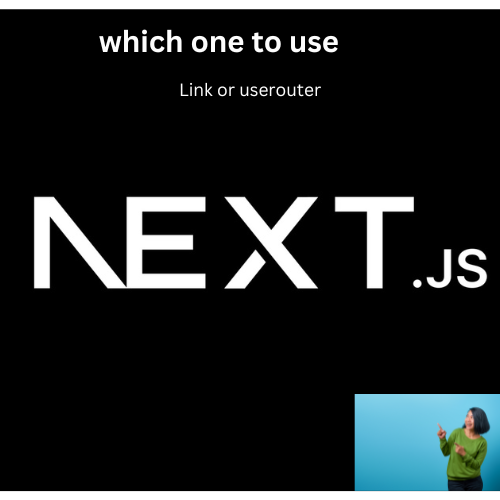
Introduction
Navigation is the backbone of user experience in web applications. In the Next.js ecosystem, developers often face a critical decision: should they use the Link
component or the useRouter()
hook? This choice isn't merely syntactic—it impacts performance, developer experience, and application architecture.
In this comprehensive guide, we'll dissect both approaches, providing you with actionable insights to make informed decisions in your Next.js projects.
The Evolution of Client-Side Navigation in Next.js
Before diving into the specifics, let's understand why this matters. Next.js revolutionized React applications by introducing a hybrid rendering model, but its client-side navigation capabilities truly set it apart from traditional multi-page applications.
The framework offers two primary navigation mechanisms:
The declarative
Link
componentThe imperative
useRouter()
hook
Each serves distinct use cases and comes with its own set of advantages.
Deep Dive: The Link Component
The Link
component is Next.js's built-in solution for declarative navigation between routes . It wraps the HTML <a>
tag while preventing full page reloads.
import Link from 'next/link'
export default function Navigation() {
return (
<nav className="flex gap-4 p-4 bg-gray-100">
<Link
href="/"
className="font-medium hover:text-blue-600 transition-colors"
>
Home
</Link>
<Link
href="/blog"
className="font-medium hover:text-blue-600 transition-colors"
>
Blog
</Link>
<Link
href="/products"
className="font-medium hover:text-blue-600 transition-colors"
>
Products
</Link>
</nav>
)
}
Advanced Link Features You Should Know
1. Automatic Prefetching
One of Link's most powerful features is automatic prefetching. When a Link component appears in the viewport, Next.js automatically prefetches the linked page in the background . This creates near-instantaneous page transitions for users.<Link href="/dashboard">Dashboard</Link>
<Link href="/dashboard">Dashboard</Link>
2. Dynamic Route Handling
The Link component elegantly handles dynamic routes with both string interpolation and URL objects :
// Method 1: String interpolation
<Link href={`/products/${product.id}`}>
View {product.name}
</Link>
// Method 2: URL object (more explicit for complex routes)
<Link
href={{
pathname: '/products/[id]',
query: { id: product.id, filter: 'active' },
}}
>
View {product.name}
</Link>
3. Scroll and History Management
Link provides fine-grained control over scroll behavior and history stack management:
// Replace current history entry instead of adding a new one
<Link href="/terms" replace>Terms & Conditions</Link>
// Disable automatic scrolling to top
<Link href="/gallery" scroll={false}>Photo Gallery</Link>
Deep Dive: The useRouter() Hook
The useRouter()
hook provides programmatic access to Next.js's router instance, offering imperative navigation control and access to route information.
import { useRouter } from 'next/router'
export default function LoginForm() {
const router = useRouter()
const [credentials, setCredentials] = useState({ email: '', password: '' })
async function handleSubmit(e) {
e.preventDefault()
const success = await authenticateUser(credentials)
if (success) {
// Programmatic navigation after form submission
router.push('/dashboard')
}
}
return (
<form onSubmit={handleSubmit}>
{/* Form fields */}
<button type="submit">Log In</button>
</form>
)
}
Advanced useRouter Features
1. Complete Router API Access
The useRouter hook provides access to the full router object, including:
const router = useRouter()
// Current route information
console.log(router.pathname) // e.g., '/products/[id]'
console.log(router.query) // e.g., { id: '123', filter: 'active' }
console.log(router.asPath) // e.g., '/products/123?filter=active'
// Navigation methods
router.push('/dashboard')
router.replace('/login')
router.back()
router.reload()
2. Shallow Routing for UI State
Shallow routing allows URL changes without triggering data fetching methods like getServerSideProps
or getStaticProps
:
function ProductFilters() {
const router = useRouter()
const { category, sort } = router.query
function updateFilters(newFilters) {
// Update URL without triggering a full page reload or data refetch
router.push(
{
pathname: router.pathname,
query: { ...router.query, ...newFilters },
},
undefined,
{ shallow: true }
)
}
return (
<div className="filter-controls">
<select
value={sort || 'newest'}
onChange={(e) => updateFilters({ sort: e.target.value })}
>
<option value="newest">Newest</option>
<option value="price-asc">Price: Low to High</option>
<option value="price-desc">Price: High to Low</option>
</select>
</div>
)
}
3. Router Events for Loading States
The router exposes events that let you create sophisticated loading experiences:
import { useEffect } from 'react'
import { useRouter } from 'next/router'
export default function LoadingIndicator() {
const router = useRouter()
const [loading, setLoading] = useState(false)
useEffect(() => {
const handleStart = () => setLoading(true)
const handleComplete = () => setLoading(false)
router.events.on('routeChangeStart', handleStart)
router.events.on('routeChangeComplete', handleComplete)
router.events.on('routeChangeError', handleComplete)
return () => {
router.events.off('routeChangeStart', handleStart)
router.events.off('routeChangeComplete', handleComplete)
router.events.off('routeChangeError', handleComplete)
}
}, [router])
return loading ? <div className="global-spinner" /> : null
}
Strategic Decision Framework: When to Use Each Approach
Choose Link When:
Building Navigation UI Elements
Menus, navigation bars, pagination, breadcrumbs
Internal links within content
Any clickable element that navigates to a predefined route
Optimizing for Performance
Taking advantage of automatic prefetching
Improving Core Web Vitals metrics
Enhancing perceived performance
Prioritizing Accessibility
Ensuring proper semantic HTML
Supporting keyboard navigation
Maintaining compatibility with screen readers
Choose useRouter() When:
Implementing Complex Navigation Logic
Conditional redirects based on user state
Multi-step forms or wizards
Authentication flows
Managing Application State in URLs
Filter and sort controls
Pagination parameters
Search queries
Creating Advanced UX Patterns
Custom loading indicators
Transition animations between routes
Confirming navigation (preventing accidental navigation away from forms)
Real-World Implementation Patterns
Pattern 1: Hybrid Navigation in Product Cards
import Link from 'next/link'
import { useRouter } from 'next/router'
export default function ProductCard({ product }) {
const router = useRouter()
async function handleAddToCart() {
try {
await addToCart(product.id)
// Show mini cart or navigate to cart
router.push('/cart')
} catch (error) {
// Handle error
}
}
return (
<div className="product-card border rounded-lg p-4 flex flex-col">
{/* Declarative navigation for the product details */}
<Link href={`/products/${product.id}`} className="group">
<div className="aspect-square bg-gray-100 mb-4 overflow-hidden rounded">
<img
src={product.imageUrl || "/placeholder.svg?height=300&width=300"}
alt={product.name}
className="w-full h-full object-cover group-hover:scale-105 transition-transform"
/>
</div>
<h3 className="font-medium text-lg group-hover:text-blue-600 transition-colors">
{product.name}
</h3>
<p className="text-gray-700">${product.price.toFixed(2)}</p>
</Link>
{/* Imperative navigation after an action */}
<button
onClick={handleAddToCart}
className="mt-auto bg-blue-600 text-white py-2 rounded hover:bg-blue-700 transition-colors"
>
Add to Cart
</button>
</div>
)
}
Pattern 2: Authentication-Aware Navigation Guard
import { useEffect } from 'react'
import { useRouter } from 'next/router'
import { useAuth } from '@/lib/auth'
export default function ProtectedRoute({ children }) {
const router = useRouter()
const { user, loading } = useAuth()
useEffect(() => {
// Skip during initial load
if (loading) return
// Redirect if not authenticated
if (!user) {
router.replace({
pathname: '/login',
query: { returnUrl: router.asPath }
})
}
}, [user, loading, router])
// Show loading state or children based on auth state
if (loading || !user) {
return <div className="p-8 text-center">Loading...</div>
}
return children
}
Performance Considerations
Link Component Performance Benefits
Automatic Code Splitting: Next.js only loads the JavaScript needed for the linked page
Prefetching: Reduces perceived loading time
Optimized Bundle Size: The Link component is lightweight
Router Hook Performance Considerations
Event Listeners: Be careful with router events to avoid memory leaks
Shallow Routing: Use it to prevent unnecessary data fetching
Route Change Callbacks: Keep them lightweight to avoid jank during transitions
Best Practices for Production Applications
Consistent Navigation Patterns
Use Link for standard navigation
Reserve useRouter for complex scenarios
Document your team's conventions
Error Handling
Implement fallbacks for failed navigations
Consider retry mechanisms for critical flows
Analytics Integration
Track page views and navigation patterns
Measure performance metrics
// Example of router events for analytics
useEffect(() => {
const handleRouteChange = (url) => {
analytics.pageView(url)
performance.mark('route-change-complete')
}
router.events.on('routeChangeComplete', handleRouteChange)
return () => {
router.events.off('routeChangeComplete', handleRouteChange)
}
}, [router])
Conclusion: The Strategic Approach
The choice between Link and useRouter isn't binary—it's contextual. Professional Next.js applications leverage both approaches strategically:
Link for its declarative simplicity and performance optimizations
useRouter for its flexibility and programmatic control
By understanding the strengths and use cases of each approach, you can create more intuitive, performant, and maintainable navigation experiences in your Next.js applications.
What's Next?
As Next.js continues to evolve, stay updated with the latest navigation patterns and best practices. The introduction of the App Router has brought new considerations to this discussion, which we'll explore in a future post.
Sources:
https://nextjs.org/docs/pages/building-your-application/routing/dynamic-routes
https://nextjs.org/docs/pages/building-your-application/routing/linking-and-navigating
https://nextjs.org/docs/app/building-your-application/routing/dynamic-routes
if you want to learn through videos .here are some clean youtube videos.
What navigation patterns have you found most effective in your Next.js projects? Share your experiences in the comments below!
Subscribe to my newsletter
Read articles from Arab Amer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arab Amer
Arab Amer
I'm a passionate Frontend and Java Developer with a strong focus on building modern, scalable web applications. Currently, I work at a startup, where I contribute to creating dynamic user experiences using Next.js and React.js. I love sharing my knowledge through blogs, helping developers learn and grow in the ever-evolving world of frontend development. Constantly exploring new technologies, I aim to blend performance, design, and functionality in every project I work on.