Understanding Extends, Implements, and With in Flutter's Dart: A Comprehensive Guide

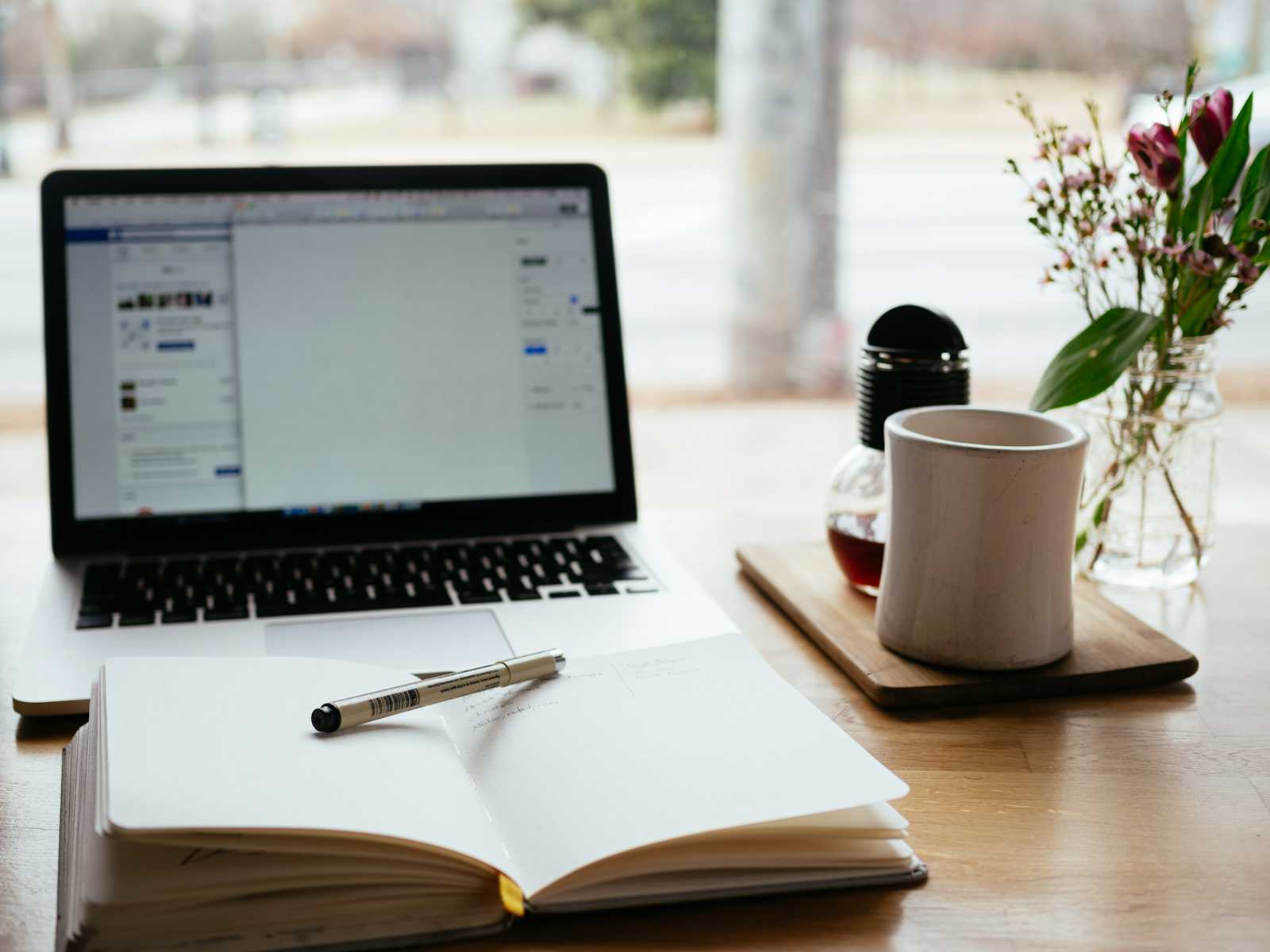
The key to understanding the differences between extends, with and implements lies in understanding three fundamental patterns of Object Oriented Programming(OOP): Inheritance, Interfaces an mixins.
If you have been using extends, implement and with in your code without actually knowing what they are, i wrote this article for you. So what exactly are these concepts?
Inheritance: Building on the Foundation of Existing Classes
Inheritance, in the world of object-oriented programming, is a mechanism that allows a child class to acquire properties and behaviors from another class called the parent or superclass.
For a moment, Imagine you're building toys..
Think of inheritance like making a new car toy that is a special version of an existing toy. Let’s say a car toy.
You start with a parent Car toy, and you create a child toy that is the same as that parent Car toy, but with some extra features or changes. In the context of programming, this is like saying, "This new class I'm making is a special kind of the old class." You do this by using the extends keyword.
You will understand it better with this code:
//You have a "Car" toy. You can make a "RaceCar" toy that extends "Car."
// The "RaceCar" is a "Car" but it also has a spoiler.. it goes faster.
// It gets all the basic "Car" stuff (name, moving, reversing, etc.) automatically.
/// ---Normal car
class Car {
String name;
Car(this.name);
void move() {
//Implement moving logic
}
void reverse() {
//Implement reversing logic
}
}
///---Race car
class RaceCar extends Car {
RaceCar(super.name);
void goTurboMode() {
//Implement TURBO MODE logic
}
}
In Dart, when we use the extends keyword we are merely performing inheritance. When a class extends another class, it inherits all the properties and methods of the parent class. And it is of the same type as the parent class, meaning they can switch places, like twins, without worries and nobody would be able to tell the difference.
While some languages support multiple inheritance, dart only supports single inheritance. This means, a class can only extend one class.
Interface: Crafting Blueprints for Consistent Behavior
In object-oriented programming an Interface is a class that defines a contract that classes must follow without specifying how the behavior is implemented. This principle is called abstraction.
Let us use, again, the toy analogy..
Think of Interfaces like following a set of instructions to build a toy.
You have a toy blueprint (an interface) that says what a toy should be able to do. You can make different toys that all follow that same blueprint, but they might be built in completely different ways.
An interface says, "If you want to follow me, you must have these methods and properties." It does not actually have an implementation for the declared methods. To use an Interface in dart we use the Implements keyword.
//You have a Toy blueprint.
// It says that any Toy toy must have a move() and makeSound() action.
// You can make a Car toy that implements Toy,
// and you can also make a Robot toy that implements Toy.
//They both follow the same rule, but they move and make sounds in completely different ways.
abstract class Toy {
void move();
void makeSound();
}
class DogToy implements Toy {
@override
void makeSound() {
//Implement dog sound logic => barking, that is.
}
@override
void move() {
//Implement Dog movement logic
}
}
class CarToy implements Toy {
@override
void makeSound() {
//Implement Car sound logic => blaring horns
}
@override
void move() {
//Implement Car movement logic
}
}
class RobotToy implements Toy {
@override
void makeSound() {
//Implement robot sound logic => maybe talking robotically.
}
@override
void move() {
//Implement robot movement logic
}
}
When a class implements an interface, it is promising to provide all the methods defined in that interface. It's like a contract. The class looks like the interface, but it's not a type of the interface. In Dart, a class can implement multiple interfaces.
Mixins: Enhancing Classes with Reusable Abilities
In simple terms, mixins are a way to add to extra abilities to a class. In more elaborate terms, a mixin is like the grey between Inheritance and Interface. A way to add extra functionality to a class when we do not want direct inheritance or have need to make an interface.
Mixins are all about reusing code. Instead of writing the same set of methods in multiple classes, you write them once in a mixin and then mix them in wherever you need them.
Let’s look at it from the toy angle once again..
Imagine you have a basic “Robot” toy that can move, and then you attach a "flying" mixin to it. Now, that robot can also fly without directly inheriting a class that has a “flying” method or implementing a “Flying” interface.
Mixins are like reusable modules of behavior that can be mixed in to multiple classes. Pun intended. To use a mixin in dart we use the with keyword.
//To use a mixin you must first define that mixin.
//Below, we have defined a Fly mixin.
//We have also defined a Human class.
//Now to make a Superhuman we extend the Human class and then mix in the Fly mixin.
mixin Fly {
void fly() {
print("Flying");
}
}
class Human {
void walk() {
print("Walking");
}
void talk() {
print("Talking");
}
void eat() {
print("Eating");
}
}
//Using Inheritance: Making a "super human" would involve inheritance,
//because it is a type of human.
//Using Mixin: Giving that human the ability to fly would need to use a mixin because
//Flying isn't what humans are capable of, but it's an extra ability you can add.
class SuperHuman extends Human with Fly {
@override
void fly() {
// TODO: implement fly
super.fly();
}
}
//The SuperHuman automatically inherits everything that the Human class can do
//But with the help of the Fly mixin, it can also fly
void main(){
SuperHuman superHuman = SuperHuman(); //create a super human instance
superHuman.walk(); //super human can walk because it is a type of Human
superHuman.talk(); //super human can talk because it is a type of Human
superHuman.fly(); //super human can fly because it has the Fly mixin
}
In Dart we can use multiple mixins in a single class. Dart has a clean and dedicated implementation of mixins. When defining a mixin it must not extend any class other Object.
In conclusion, mixins are all about adding special abilities to classes. Inheritance is all about creating special version of existing classes while interfaces are all about creating blueprints that other classes must follow to achieve certain things.
I hope this makes it clearer for you!
In the next post we will get to see a real life usage of extends, implements and with in a real app. Make sure to hit the follow button if you want to see that post.
Kusoma!
Subscribe to my newsletter
Read articles from Iwarifgha Toby directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
