Working with Query Parameters in Vue 3

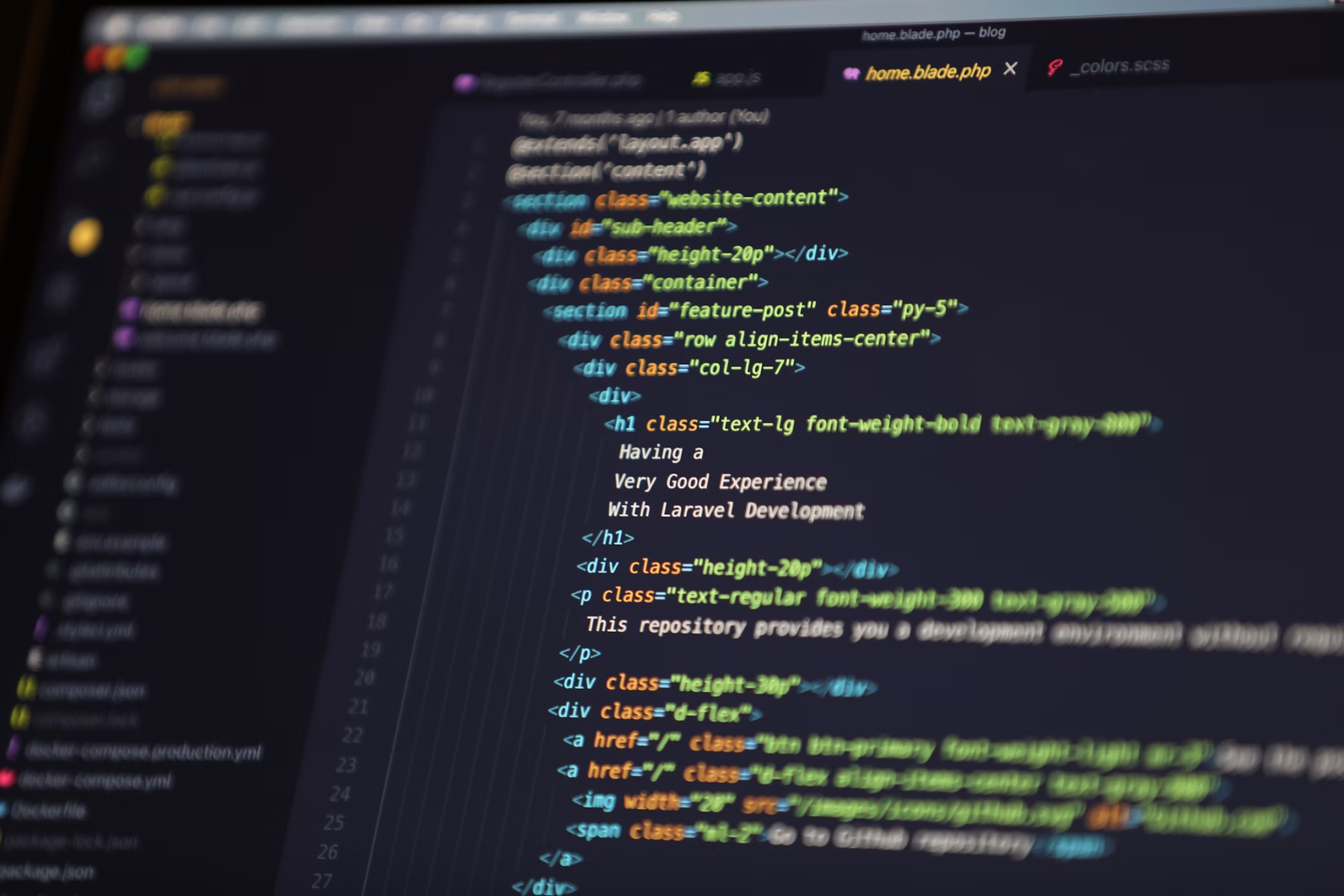
I started working with Vue about three weeks ago, and so far, it's going great. The learning curve is not as steep as React's, and the way it handles certain aspects within components makes things move smoother. I had the grand chance to finally work with the Router, hence this article.
Query Parameters
Query parameters allow you to add more information to a URL when making requests to a server. A very easy example where we can see this is a simple search on Google. Immediately you type in the query and hit the search button, it becomes a long string in the search bar with equal signs.
I needed to perform a pagination function in my Vue app, whereby after filling and submitting a form in my form component, I would be automatically navigated to the last page of a paginated table in a separate table component. To solve this, I decided to use query parameters, and I'll show you how I did that in this article.
Working with Query Parameters
Router
Assuming that we already have our Vue app set up, we want to install Vue Router with npm install vue-router@latest
. The latest version at the time of writing is 4.
You can create a separate file for your router as I like to do, so in an index.js
file, I define my routes.
import { createRouter, createWebHistory } from "vue-router";
import ListOfCommandsView from "@/views/ListOfCommandsView.vue";
import CreateCommandView from "@/views/CreateCommandView.vue";
const router = createRouter({
history: createWebHistory(import.meta.env.BASE_URL),
routes: [
{
path: "/",
name: "home",
component: ListOfCommandsView,
},
{
path: "/create",
name: "create",
component: CreateCommandView,
},
],
});
export default router;
My components have already been created. I have a CreateCommandView
for my form, and I have a ListOfCommandsView
for my table.
Form component
When I submit the form, I want to be navigated back to the table component so that the form data is added to my table as a row (we won't go into the details of that here as it is not the point).
For the submit function in my form component,
<script setup>
import { useRouter } from 'vue-router'
const totalNumberOfPages = ref(3)
const addRowVal = () => {
// do something like check for empty fields
// navigate back to home
router.push({
name: 'home',
query: { currentPage: totalNumberOfPages.value }
})
}
</script>
<template>
<!-- form template -->
</template>
After running and performing whatever action the addRowVal
function needs to perform, the router.push
function pushes a new entry into the history stack and takes us to the home page where our table is. The query
property of the object is where we define the query, and it is the totalNumberOfPages
in this case. And that would make sense because we are navigating to the last page of the table.
Once our form is submitted and the navigation takes us back to the CreateCommandView
component where our table is, the URL changes to http://localhost:5173/?currentPage=3
`
Getting query parameters from route
Grabbing the query parameters and using them is something we might also want to do. Doing this is also just as easy as setting them.
<script setup>
import { useRoute } from 'vue-router'
const route = useRoute()
console.log(route.query.currentPage)
</script>
Thats it. The useRoute
composable returns the current route and via that, we can access the query which in this case is our currentPage
.
That's it...
Besides the sneaky fact that this article is a test run for my new blog, it's something I very much felt like writing on the whim. The Vue Router docs do an amazing job of explaining everything surrounding the router already, so this article only scratches the surface of query parameters. As I learn (and burn with the need to FINALLY publish something on my blog), I hope to put out simple articles or even bigger ones on my experience with Vue.
Subscribe to my newsletter
Read articles from Njong Emy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Njong Emy
Njong Emy
Short girl swimming her way through the tech ocean, avoiding sharks, and staying on the javascript boat. I write bite sized articles that show you the ropes around simple tech notions :)