Why Upgrade to Java 24? What you need to know
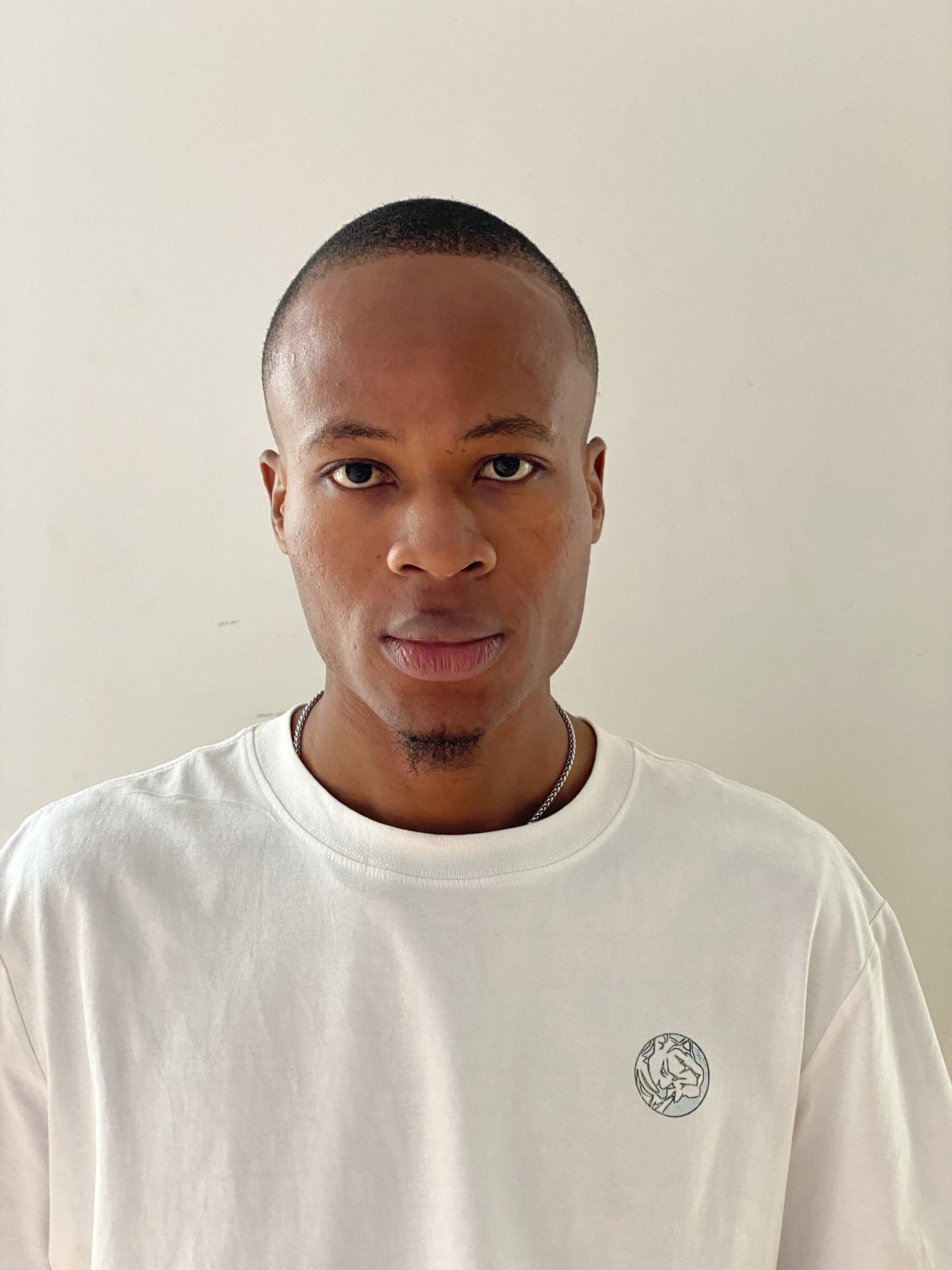
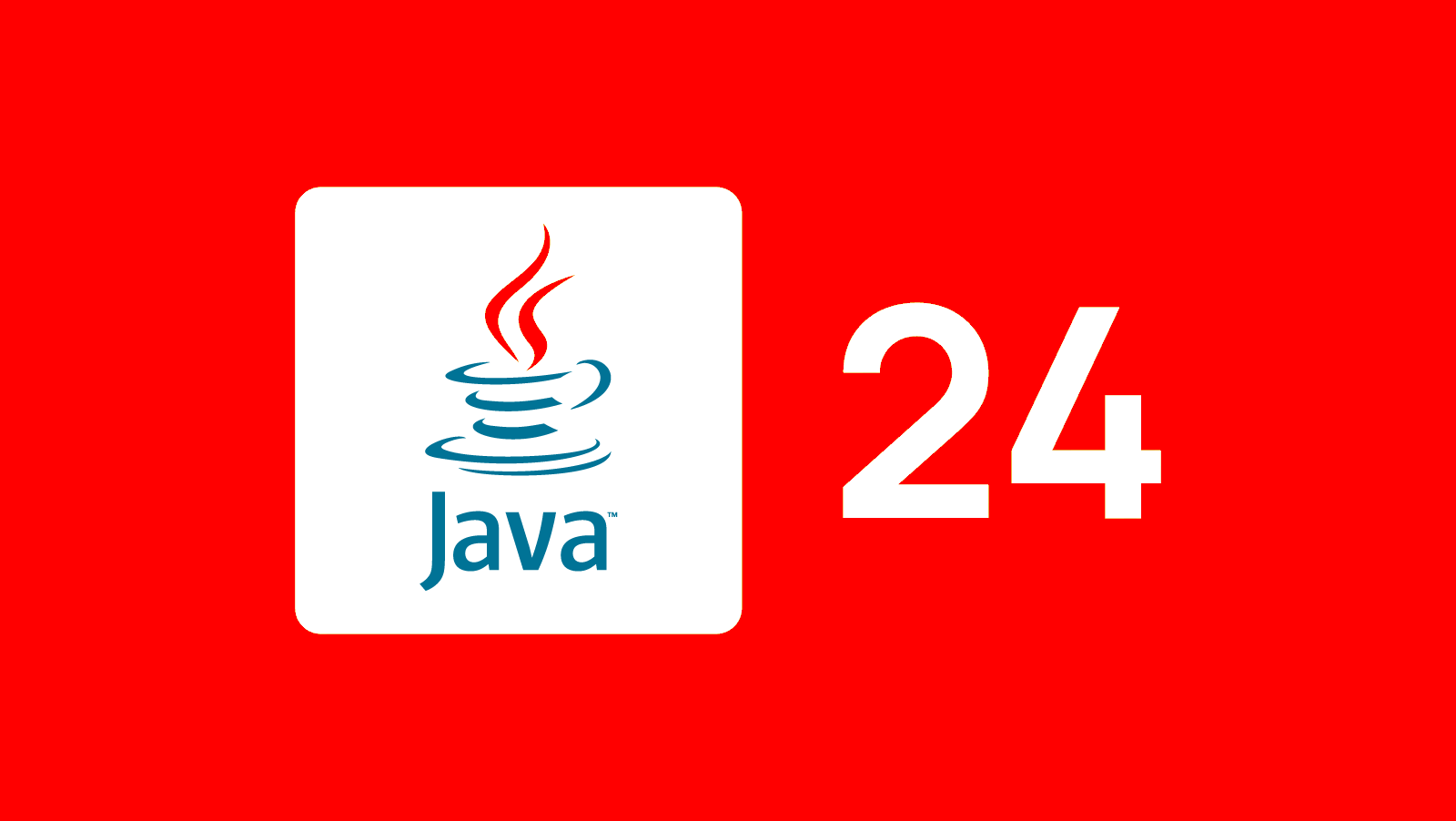
Java will celebrate its 30-year anniversary later this year, and given the rate at which it is evolving, especially with the Java 24 upgrade, it is safe to say it is not slowing down anytime soon. After reviewing the Java 24 Enhancement Proposals (JEPs), it is clear that this release builds directly on Java 23, finalizing several previews and rolling out key features. If you need a refresher on Java 23, you can check its features here
Unlike Java 23, which received fewer upgrades, Java 24 includes a broader range of improvements aimed at increasing developer productivity and efficiency. While it lacks long-term support (LTS), it does provide a glimpse into what the next stable version will bring, and it seems promising.
If you're wondering when the next LTS release will arrive, it’s expected to be Java 25, scheduled for release before October 2025.
Major Enhancement that comes with Java 24
Ahead of time Class loading and linking (JEP 483)
Stream Gatherers (JEP 485)
Late Barrier expansion for G1 (JEP 475)
Introduction of Key Derivation Function API (Preview) (JEP 478)
Permanently disable the Security Manager (JEP 486)
ZGC:Removal of the Non Generational mode (JEP 490)
Removal Of the Windows 32-bit x86 port (JEP 479)
Synchronize Virtual threads without Pinning (JEP 491)
Class File API (JEP 484)
Linking runtime images without JMODs (JEP 493)
Quantum-Resistant Cryptography (JEP 496 & 497)
Prepare to restrict the use of Java Native Interface (JNI) (JEP 472)
Generational shenandoah (Experiment) (JEP 404)
Compact Object Headers (Experiment) (JEP 450)
Ahead Of Time Class Loading and Linking
This feature enhances startup performance by preloading and linking classes before execution. This reduces the workload of the Just-In-Time (JIT) compiler during runtime while also minimizing class-loading overhead in the Java Virtual Machine. As a result, applications, particularly large ones like Spring Boot applications, can start faster and become responsive more quickly.
Stream Gatherers
Stream::gather(Gatherer) is a new intermediate stream operation that processes the elements of a stream by applying a Gatherer implementation. Stream Gatherers improves the Stream API by providing ways for collecting data more efficiently into a mutable container.
This feature is extremely important for gathering large volumes of data because it reduces overhead and improves efficiency. Given the availability of a calculator, why would you want to compute your numbers using an abacus?
code example:
public static void main(String[] args) {
List<List<String>> windows1 =
Stream.of("a","b","c","d","e","f","g","h").gather(Gatherers.windowFixed(3)).toList();
List<List<Integer>> windows2 =
Stream.of(1,2,3,4,5,6,7,8).gather(Gatherers.windowSliding(2)).toList();
System.out.println(windows1 + "\n" + windows2);
}
THE RESULT WILL LOOK LIKE THIS
[[a, b, c], [d, e, f], [g, h]]
[[1, 2], [2, 3], [3, 4], [4, 5], [5, 6], [6, 7], [7, 8]]
Late Barrier Expansion for G1
This feature improves G1 Garbage Collection (GC) by delaying when memory barriers are added. Instead of the typical early barrier (which can slow things down), it waits and adds them only when and where necessary. This makes garbage collection more efficient, reducing GC-related delays and improving overall application performance.
Key Derivation Function API (Preview)
This feature improves Java security by enhancing cryptographic data protection. Previously, Java lacked a built-in Key Derivation Function (KDF) API, requiring developers to use custom implementations or third-party libraries like Bouncy Castle or Google Tink, which added complexity. The KDF API in Java 24 simplifies secure key generation, allowing developers to implement strong encryption protocols with ease. This API provides a standardized, consistent, and secure method for deriving cryptographic keys for encryption and authentication.
code example:
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.PBEKeySpec;
import java.util.Base64;
public class KDFExample {
public static void main(String[] args) throws Exception {
String password = "SecurePassword";
byte[] salt = "RandomSalt".getBytes();
var spec = new PBEKeySpec(password.toCharArray(), salt, 100_000, 256);
var factory = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA256");
var key = factory.generateSecret(spec).getEncoded();
System.out.println("Derived Key: " + Base64.getEncoder().encodeToString(key));
}
} //KDF API used in generating key that later got encoded to base 64
Permanently Disable The Security Manager
Since Java 17, the Security Manager has been deprecated due to its outdated architecture and performance limitations. With the evolution of the Java ecosystem, developers are encouraged to adopt modern security practices that provide better efficiency, flexibility, and stronger protection.
ZGC: Removal Of The Non Generational Mode
The default transition to generational mode is driven by the goal of simplifying maintenance: maintaining both generational and non-generational modes requires significant resources. By focusing solely on generational ZGC, the OpenJDK team aims to fine-tune performance, creating a more streamlined and robust GC that meets the demands of modern Java applications and their complex memory usage patterns.
Removal Of The Windows 32-bits x86 Port
With the evolution of the Java ecosystem and its focus on performance enhancements, the removal of support for Windows 32-bit systems was only a matter of time. By fully embracing 64-bit systems, Java enables developers to leverage modern features without the burden of maintaining 32-bit fallbacks, leading to better performance, scalability, and compatibility with current hardware and software improvements.
Synchronize Virtual Threads Without Pinning
This feature directly addresses the pinning issue that occurs when using a synchronized block inside a virtual thread.
Prior to Java 24, when a virtual thread entered a synchronized block, it may block (pin) the underlying carrier thread, preventing it from handling other virtual threads. This resulted in wasted resources, lower concurrency, and poor scalability.
With this enhancement, virtual threads no longer pin carrier threads when using synchronized, allowing them to pause and resume efficiently without blocking system resources. This results in better performance, higher scalability, and improved responsiveness in high-concurrency applications.
Class File API
Previously, tools that analyzed Java bytecode relied on third-party libraries like ASM or BCEL. However, with changes in bytecode formats across Java versions, these tools often broke and required updates.
The Class-File API provides a standardized way for parsing, generating, and transforming Java class files, as well as tracking the JVM-defined class file format, making bytecode analysis and modification more dependable and future-proof.
A company using a custom security compliance tool to inspect Java classes might experience breakages when upgrading from JDK 17 to JDK 23 due to bytecode changes. The class-file API eliminates this issue by providing a stable, official API, ensuring the tool works seamlessly across Java versions without major rewrites.
Linking Runtime Images Without Jmods
This feature increases efficiency by allowing the jlink tool to create custom runtime images without requiring JMOD files. Developers can now link a runtime image from modular JARs, standalone modules, or previously linked runtime images.
However, it is not enabled by default. It must be explicitly enabled when the JDK is built, and some JDK vendors may choose not to support it.
Quantum Resistant Cryptography
This feature strengthens Java security in preparation for quantum computing threats. It introduces the lattice-based Key Encapsulation Mechanism (ML-KEM) to secure symmetric keys over insecure channels using public key cryptography, as well as the lattice-based Digital Signature Algorithm (ML-DSA), which detects unauthorized data modifications and authenticates signatories. It is like building a fighter jet before a war.
Prepare to restrict the use of Java Native Interface (JNI)
Java 24 introduces stricter controls on JNI through JEP 472 to enhance security and maintainability. While JNI remains a standard way for Java to interact with native code, developers are encouraged to transition to the Foreign Function & Memory (FFM) API, which provides a safer and more modern alternative for native interoperation.
The reason for the FFM API is intended to allow Java programs to use external code and handle data safely, avoiding the issues that are frequently encountered when using JNI, which include complexity, safety risks, and loss of platform independence.
Java 24 introduces the --illegal-native-access
option to warn or restrict unauthorized JNI usage, preparing for stricter enforcement in future releases.
Generational shenandoah(Experiment)
This feature is still experimental, meaning it may undergo modifications, and it requires enabling via JVM options. It enhances the Shenandoah Garbage Collector with Generational Collection, which categorizes objects into young and old generations. This improves performance and reduces pause times by optimizing memory reclamation based on object lifetimes.
Young Generation: Short-lived objects are collected more frequently.
Old Generation: Long-lived objects are collected less often
Compact Object Headers (Experiment)
This feature is still in the experimental stage and must be enabled using JVM options. It optimizes memory usage by reducing the size of object headers between 96 and 128 bits down to 64 bits on 64-bit architectures, allowing more efficient memory allocation. By minimizing header overhead, Java applications can achieve better memory density, which is particularly beneficial for applications with a large number of small objects.
FEATURES IN REVIEW
Deprecation Of Memory Access Methods in sun.misc.Unsafe (JEP 498)
Scoped Values (Fourth Preview) (JEP 487)
Primitive Types in Patterns, instanceof, and switch (Second Preview) (JEP 488)
Vector API (Ninth Incubator) (JEP 489)
Flexible Constructor Bodies (Third Preview) (JEP 492)
Module Import Declarations (Second Preview) (JEP 494)
Simple Source Files and Instance Main Methods (Fourth Preview) (JEP 495)
Structured Concurrency (Fourth Preview) (JEP 499)
Most of them have been explained in the previous version articles 22 & 23.
CONCLUSION
If there’s one thing I can say for sure, it’s that Java 24 equips developers with the right tools to build cutting-edge, AI-powered applications. In fact, its preparation for the era of quantum computing speaks volumes about how Java has evolved over the past 30 years.
The new release's extensive range of capabilities will help developers enhance productivity, allowing them to deliver feature-rich apps to their businesses and customers faster and more effectively. It is worth noting that Java 24 is a considerable improvement in developer productivity, security, and performance. Despite not offering long-term support, it is nevertheless worthwhile to explore.
If you enjoyed my article, please consider asking for my account number; don't buy me coffee. I wouldn't wanna pretend like I love coffee when I actually prefer hot pap. And please stay tuned for more articles about programming, technologies, java, and AI. 🧑💻
Reach out to me through:
LinkedIn: LinkedIn
Mail: salamkorede345@gmail.com
Subscribe to my newsletter
Read articles from Salami Korede directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
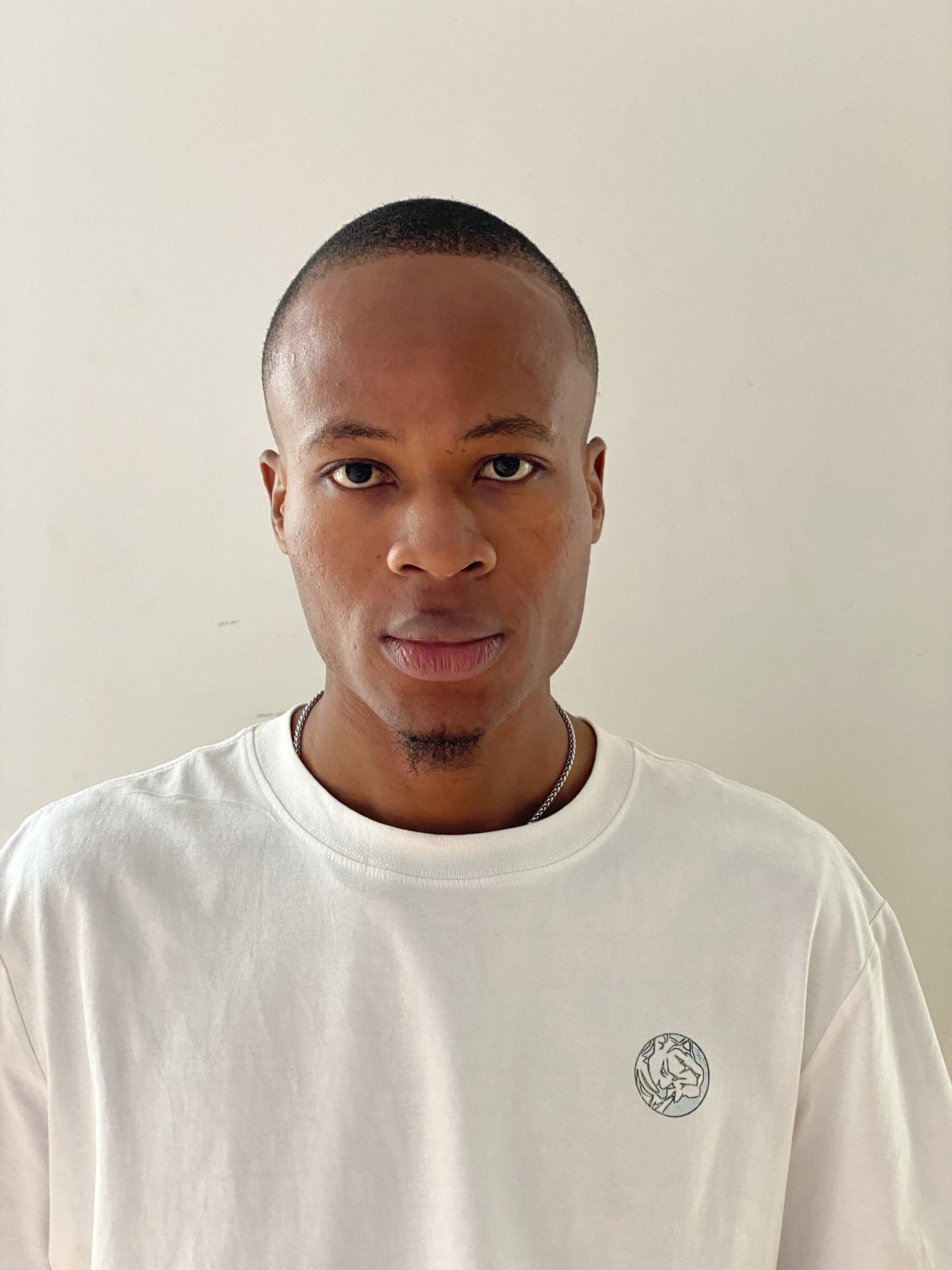
Salami Korede
Salami Korede
Software Engineer from Nigeria ready to collaborate on projects and help people learn