Simplify Role-Based Access Control (RBAC) with "authz" Checker
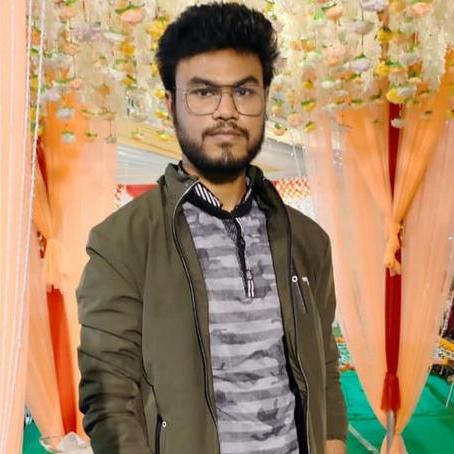
Introduction
Managing user permissions is a critical aspect of web applications, especially when dealing with multiple user roles such as Admins, Moderators, and Users. Instead of hardcoding permission logic into different parts of your code, a structured Role-Based Access Control (RBAC) system makes it easier to manage and scale your application securely.
Introducing Authorization Checker, an easy-to-use NPM package that helps you implement RBAC effortlessly in your Node.js applications.
Why Use Authorization Checker?
Scalability: Easily add or modify roles and permissions.
Security: Prevent unauthorized actions with a centralized permission system.
Simplicity: A lightweight and intuitive API.
Customizable: Define your own roles and access levels.
Installation
Installing the package is straightforward using npm or yarn:
npm install @biswarup598/authz
or
yarn add @biswarup598/authz
How It Works
Authorization Checker works by defining roles and their associated permissions. Then, using a simple function, you can check if a user is authorized to perform a specific action on a resource.
Example Usage
Import the module
import { checkPermission } from '@biswarup598/authz';
Define a user and check permissions
const user = { role: 'admin' };
const action = 'create';
const resource = 'post';
if (checkPermission(user, action, resource)) {
console.log('Access granted');
} else {
console.log('Access denied');
}
Role and Permission Breakdown
Admin Permissions
- Can view, create, update, and delete all resources, including posts, comments, likes, reports, follows, blocks, and users.
Moderator Permissions
- Can manage posts, comments, likes, reports, follows, and blocks but cannot modify admin settings.
User Permissions
- Can view, create, update, and delete their own posts, comments, likes, follows, and blocks.
API Reference
checkPermission(user, action, resource)
user: An object containing the
role
property.action: The action to be performed (e.g.,
create
,update
,delete
).resource: The resource on which the action is performed (e.g.,
post
,comment
).Returns:
true
if the user has permission, otherwisefalse
.
Why RBAC Matters in Modern Applications
RBAC is a standard practice in securing applications. It minimizes the risk of unauthorized actions, ensures compliance, and simplifies permission management. By using a dedicated authorization system like Authorization Checker, you reduce security vulnerabilities and streamline your codebase.
Conclusion
Authorization Checker is a simple yet powerful tool for implementing RBAC in Node.js applications. With minimal setup, you can ensure a secure and scalable permission management system for your users.
๐ Check out authz on NPM and start implementing RBAC today!
Subscribe to my newsletter
Read articles from Biswarup Naha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
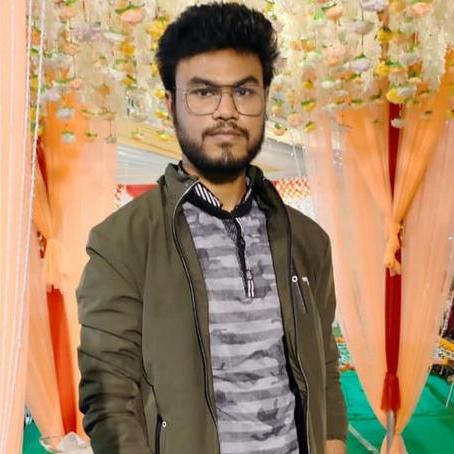