Understanding JavaScript Bundlers: Tools for Modern Web Development
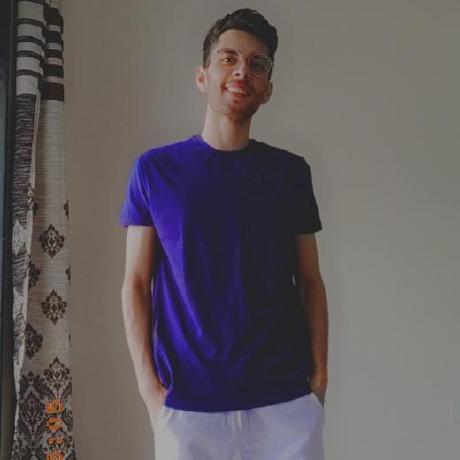
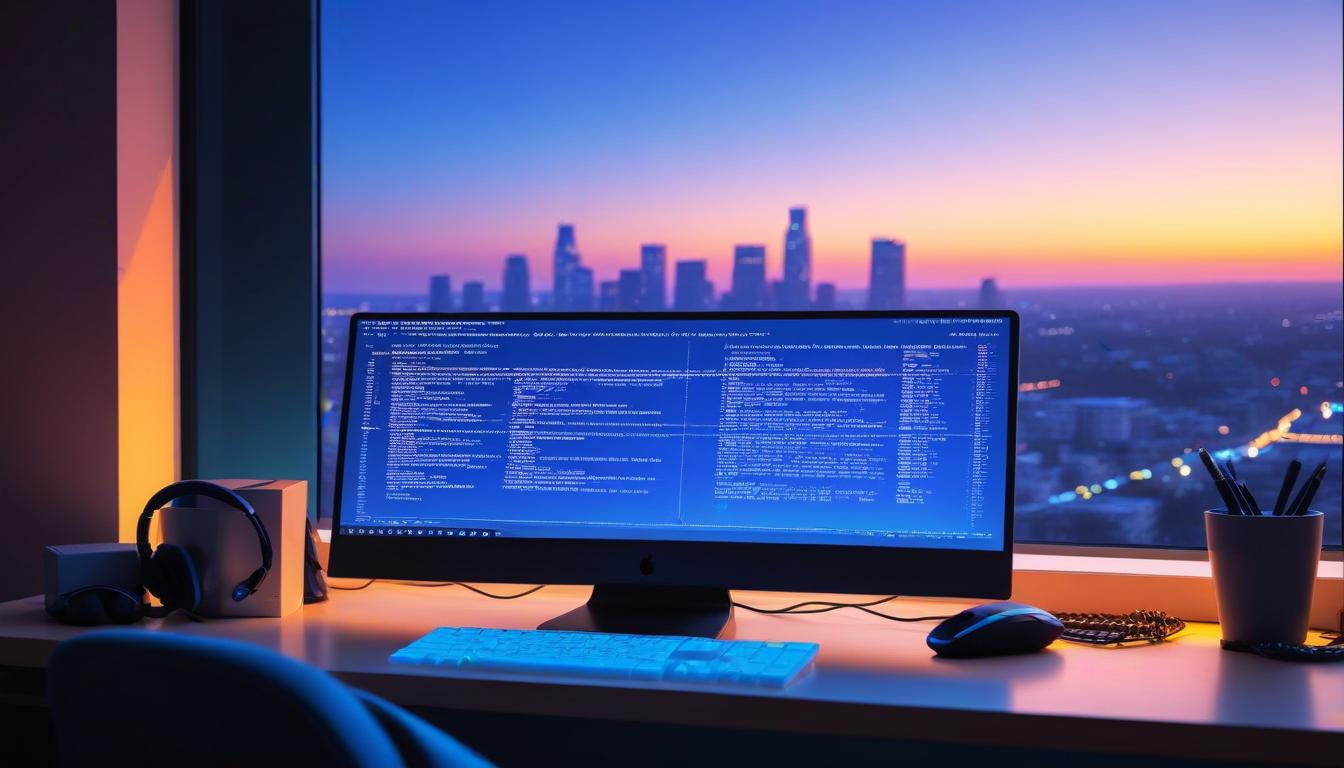
Introduction
Modern JavaScript applications are like high-performance cars—they need a strong engine to run well. That's where bundlers come in! Just as an engine optimizes fuel use for the best performance, bundlers optimize and package our code to ensure smooth, efficient loading in production. In this blog, we'll explore what bundlers are, why they're important, and take a closer look at the core concepts.
What is a bundler?
A bundler is a tool that processes and combines multiple JavaScript files, along with other assets like CSS, images, and fonts, into a single or optimized set of files for production. It ensures that dependencies are properly managed, applies optimizations like minification and compression, and can perform tree shaking (removing unused code) to keep the final bundle as small as possible.
Why use a Bundler?
Let’s say I’m using Flipkart to purchase something. When I visit the homepage, it loads quickly and displays products instantly. However, behind the scenes, Flipkart’s website consists of:
Thousands of JavaScript modules manage search, recommendations, cart, and checkout.
Multiple CSS files style different parts of the website.
High-resolution images are used for product listings and banners.
Third-party libraries handle analytics, payment gateways, and interactive elements.
If Flipkart loaded each of these files separately, it would lead to hundreds of network requests, slowing down the website and creating a frustrating user experience. This is where a bundler comes in to optimize everything, ensuring fast and smooth performance.
How a Bundler Helps Flipkart:
Modular Development – Flipkart’s code is split into small, manageable modules (e.g., separate files for cart, checkout, and recommendations). A bundler combines them efficiently for production.
Performance Optimization – Product images are compressed, JavaScript and CSS files are minified, and unnecessary spaces/comments are removed, reducing file size and improving load speed.
Dependency Management – Flipkart relies on third-party libraries like Moment.js for order date tracking. A bundler ensures these dependencies are organized and resolved automatically, avoiding conflicts.
Tree Shaking & Code Splitting – If Flipkart only uses one function from Moment.js, the bundler removes all unused code/functions. Additionally, the checkout page doesn’t load homepage scripts, ensuring only the essential code is delivered per page. The bundler ensures only the required code loads for each page, making navigation snappy and efficient.
Core Concepts of Bundlers:
No matter which car we drive, the core mechanics remain the same—every car needs an engine, wheels, fuel efficiency, and a transmission. Yes, again a car example, I just love cars. 🏎️
So similarly, every bundler relies on entry points, outputs, loaders, plugins, modes, and browser compatibility to optimize our JavaScript application. Every bundler optimizes JavaScript applications by processing and packaging code efficiently. While each bundler has its own approach, they all share some fundamental concepts.
Let’s take an example of Webpack, the bundler used in Create React App(depreciated).
- Entry: The Starting Point:
The entry point is the main file from which the bundler starts processing dependencies. It defines what should be included in the final bundle.
In Webpack, this is explicitly set in webpack.config.js
:
module.exports = {
//default is index.js, but we can specify a different (or multiple) entry points
entry: './path/to/my/entry/file.js'',
};
- Output: The Final Bundled Code:
After processing, the bundler needs to save the final output. The output configuration decides the file name and where the final bundled code will be stored.
module.exports = {
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'my-first-webpack.bundle.js',
},
};
- Loaders: Handling Different File Types:
JavaScript applications often include CSS, images, TypeScript, and other assets. Bundlers use loaders to transform these files into modules that JavaScript can understand.
The test
property identifies which file or files should be transformed. The use
property specifies which loader should be used to perform the transformation.
module.exports = {
module: {
rules: [
{
// A CSS loader in Webpack transforms CSS files
test: /\.css$/,
use: ["style-loader", "css-loader"],
},
],
},
};
- Plugins: Extending Bundler Capabilities:
While loaders transform specific types of modules, plugins can handle a wider range of tasks, such as optimizing bundles, managing assets, and injecting environment variables. To use a plugin, we need to use require.
In Webpack, the HtmlWebpackPlugin
automatically injects bundled files into an HTML template:
const HtmlWebpackPlugin = require('html-webpack-plugin');
const webpack = require('webpack'); //to access built-in plugins
module.exports = {
plugins: [new HtmlWebpackPlugin({ template: './src/index.html' })],
};
- Mode: Development vs. Production:
Bundlers offer different build modes, the default value is production
.
Development Mode: Prioritizes speed, includes source maps for debugging.
Production Mode: Minifies and optimizes code for better performance.
module.exports = { mode: 'development', };
- Browser Compatibility: Ensuring Smooth Execution:
Web applications need to run smoothly across different browsers. Bundlers ensure compatibility by transpiling modern JavaScript (ES6+) into older versions when necessary.
Webpack and Parcel use Babel for this. We will explore Babel in another blog. 😅
No matter which bundler you use—Webpack, Parcel, or Vite—their main function is the same: to efficiently process, optimize, and package our JavaScript for smooth performance.
Bonus
Personally I like using Parcel for its zero-configuration setup and built-in optimizations. Give it a try and share your experience
Conclusion:
Bundlers are essential in modern web development for optimizing and managing JavaScript applications. While Webpack is still the industry standard, newer bundlers like Vite and Turbopack (by Vercel) offer better performance and simplicity. Choosing the right bundler depends on the project's complexity, performance requirements, and ecosystem support.
Subscribe to my newsletter
Read articles from Syed Sibtain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
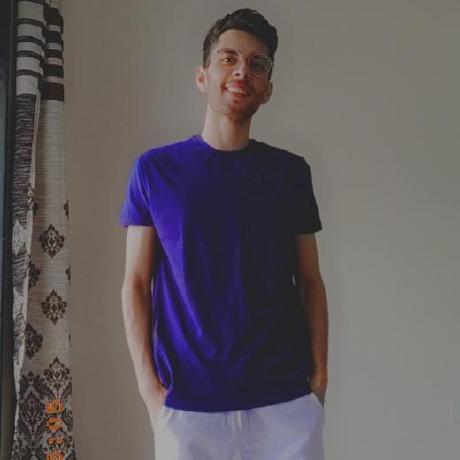