useReducer in React: When and Why to Use It Over useState
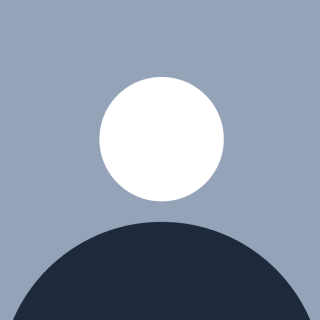
When managing state in React, most developers start with useState. However, as state logic grows complex—especially when multiple values need to change based on specific actions—useReducer becomes a more structured and scalable alternative.
In this post, we’ll dive into:
✅ What useReducer is and how it works.
✅ When to use useReducer instead of useState.
What is useReducer?
useReducer is a React Hook that helps manage state transitions in a more predictable way using a reducer function. Instead of updating state directly like useState, useReducer follows a pattern inspired by Redux:
const [state, dispatch] = useReducer(reducer, initialState);
The Reducer Function
A reducer function determines how state should change based on the action it receives:
const reducer = (state, action) => { switch (action.type)
{
case "INCREMENT":
return { count: state.count + 1 };
case "DECREMENT":
return { count: state.count - 1 };
case "RESET":
return { count: 0 }; default: return state; } };
Basic Example: Counter
Here’s how you can use useReducer to manage a counter:
import { useReducer } from "react";
const initialState = { count: 0 };
const reducer = (state, action) => { switch (action.type) { case "INCREMENT": return { count: state.count + 1 }; case "DECREMENT": return { count: state.count - 1 }; case "RESET": return { count: 0 }; default: return state; } };
export default function Counter() { const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<h2>Count: {state.count}</h2>
<button onClick={() => dispatch({ type: "INCREMENT" })}>+</button>
<button onClick={() => dispatch({ type: "DECREMENT" })}>-</button>
<button onClick={() => dispatch({ type: "RESET" })}>Reset</button>
</div>
);
}
Instead of modifying state directly, we call dispatch({ type: "ACTION_NAME" }), and the reducer function determines the new state.
When to Use useReducer Instead of useState
You should use useReducer when:
✔️ State transitions involve multiple actions.
✔️ New state depends on the previous state.
✔️ You want to consolidate state updates in one function.
Subscribe to my newsletter
Read articles from Enofua Etue Divine directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Enofua Etue Divine
Enofua Etue Divine
I'm a tech enthusiast . I'm a web developer and a student at Altschool Africa currently learning Frontend engineering ...