The Basics of PHP for Web Development

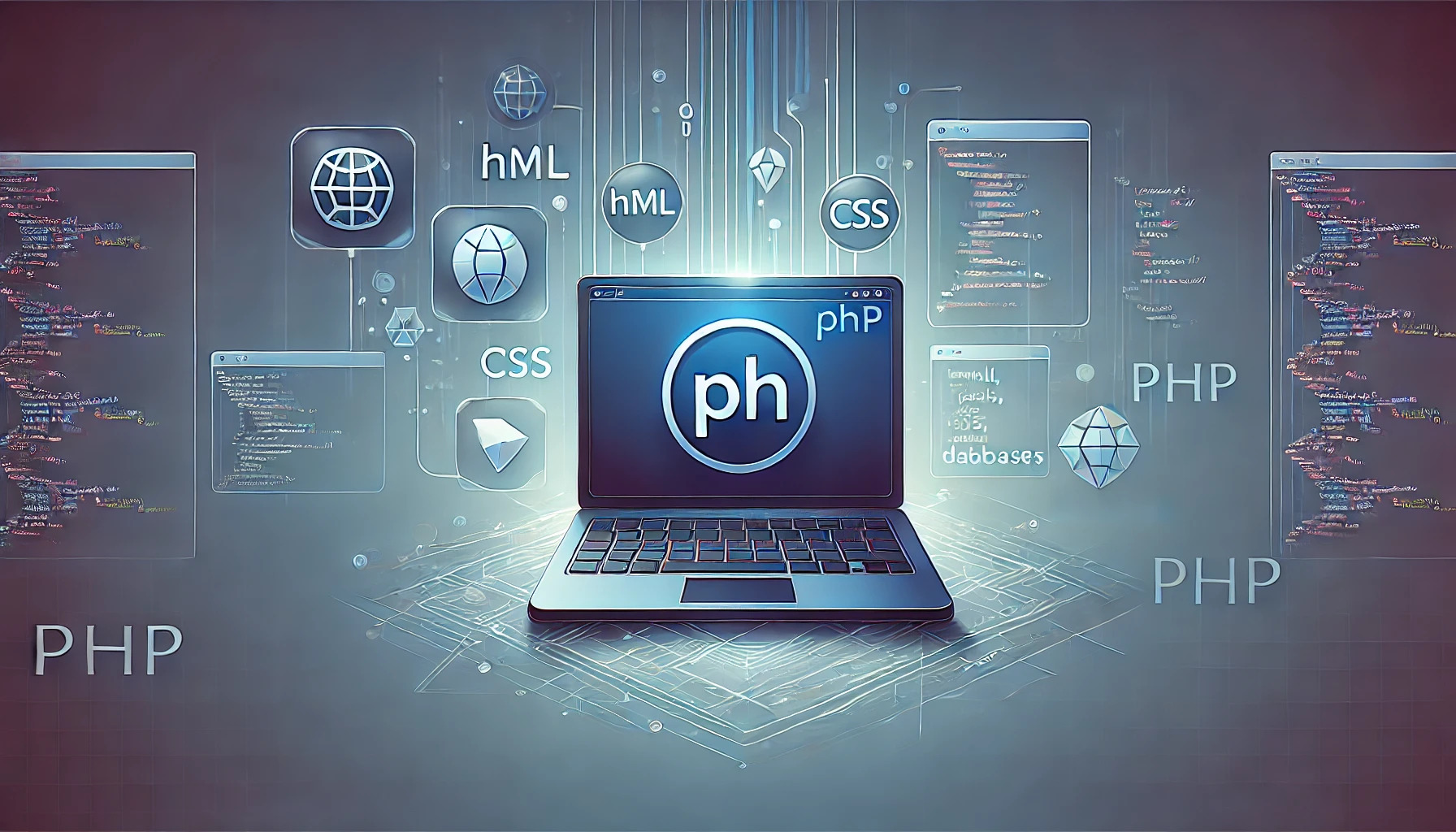
The Basics of PHP for Web Development
PHP (Hypertext Preprocessor) is one of the most widely used server-side scripting languages for web development. It powers over 77% of all websites with a known server-side programming language, including major platforms like WordPress, Facebook, and Wikipedia. If you're looking to dive into web development or enhance your programming skills, PHP is an excellent place to start. In this article, we’ll cover the basics of PHP, its key features, and how you can use it to build dynamic websites. Plus, if you're interested in monetizing your programming skills, platforms like MillionFormula offer great opportunities to make money online—completely free, with no credit or debit cards required.
What is PHP?
PHP is an open-source scripting language designed for web development. It is embedded within HTML and executed on the server, generating dynamic content that is sent to the client's browser. Unlike client-side languages like JavaScript, PHP runs on the server, making it ideal for tasks like database interactions, form processing, and user authentication.
Key Features of PHP
Open Source: PHP is free to use and has a large community of developers contributing to its growth.
Cross-Platform: It runs on various operating systems like Windows, Linux, and macOS.
Database Support: PHP integrates seamlessly with databases like MySQL, PostgreSQL, and MongoDB.
Ease of Learning: Its syntax is simple and beginner-friendly, making it a great choice for new developers.
Setting Up PHP
To start coding in PHP, you’ll need a local development environment. Tools like XAMPP or WAMP provide everything you need, including a web server (Apache), a database (MySQL), and PHP itself.
Once installed, create a .php
file (e.g., index.php
) and start writing PHP code. Here’s a simple example:
php
Copy
<?php
echo "Hello, World!";
?>
This script outputs "Hello, World!" to the browser. The <?php
and ?>
tags indicate the start and end of PHP code.
Basic PHP Syntax
PHP syntax is similar to C, Java, and Perl. Here are some fundamental concepts:
Variables
Variables in PHP start with a $
sign. They are loosely typed, meaning you don’t need to declare their data type explicitly. php Copy
<?php
$name = "John Doe";
$age = 25;
echo "My name is $name and I am $age years old.";
?>
Conditional Statements
PHP supports if
, else
, and switch
statements for decision-making. php Copy
<?php
$score = 85;
if ($score >= 90) {
echo "Excellent!";
} elseif ($score >= 70) {
echo "Good job!";
} else {
echo "Keep trying!";
}
?>
Loops
PHP provides for
, while
, and foreach
loops for repetitive tasks. php Copy
<?php
for ($i = 1; $i <= 5; $i++) {
echo "Iteration $i <br>";
}
?>
Working with Forms
One of PHP’s strengths is handling form data. Here’s an example of a simple HTML form processed by PHP:
HTML Form:
html
Copy
<form action="welcome.php" method="post">
Name: <input type="text" name="name"><br>
Email: <input type="text" name="email"><br>
<input type="submit">
</form>
Run HTML
PHP Script (welcome.php): php Copy
<?php
$name = $_POST['name'];
$email = $_POST['email'];
echo "Welcome, $name! Your email is $email.";
?>
This script retrieves data from the form using the $_POST
superglobal and displays it.
Database Interaction with PHP
PHP is often used with MySQL to create dynamic websites. Here’s a basic example of connecting to a MySQL database and fetching data: php Copy
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Fetch data
$sql = "SELECT id, name FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"]. " - Name: " . $row["name"]. "<br>";
}
} else {
echo "0 results";
}
$conn->close();
?>
This script connects to a MySQL database, retrieves data from the users
table, and displays it.
Why Learn PHP?
High Demand: PHP developers are in high demand due to its widespread use in web development.
Cost-Effective: Being open-source, PHP reduces development costs.
Community Support: A large community means plenty of resources, tutorials, and forums for help.
Scalability: PHP powers small blogs and large-scale applications alike.
Monetizing Your PHP Skills
Once you’ve mastered PHP, you can start monetizing your skills. Platforms like MillionFormula provide a free and accessible way to make money online. Whether you’re freelancing, building websites, or creating web applications, MillionFormula connects you with opportunities to earn—no credit or debit cards required.
Conclusion
PHP is a versatile and powerful language for web development. Its simplicity, combined with robust features, makes it a favorite among developers. By learning PHP, you open doors to countless opportunities in the tech industry. And if you’re ready to turn your skills into income, platforms like MillionFormula can help you get started.
Start coding in PHP today, and take the first step toward building dynamic, interactive websites!
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
