Mix: A Beginner’s Guide to Elixir’s Build Tool and Dependency Manage
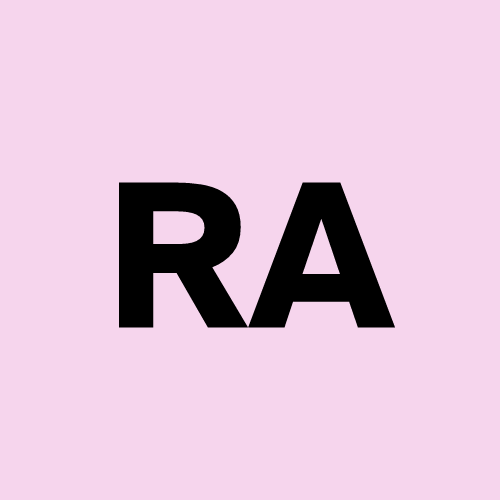
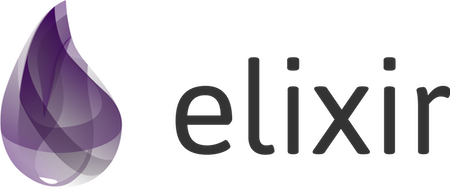
Introduction
Mix is Elixir’s default build tool, designed to automate common tasks like project creation, compilation, testing, and dependency management. While similar to tools like dotnet
CLI, Mix offers deeper extensibility and is tightly integrated with Elixir’s ecosystem.
Core Features
- Creating a Project
Generate a new Elixir project with a single command:
mix new friends
This creates a structured project with a mix.exs file at its core.
- The mix.exs File
This file defines project settings, dependencies, and tasks. Here’s a minimal example:
defmodule Friends.MixProject do
use Mix.Project
def project do
[
app: :friends,
version: "0.1.0",
elixir: "~> 1.18",
start_permanent: Mix.env() == :prod,
deps: deps()
]
end
def application do
[extra_applications: [:logger]]
end
defp deps do
[] # Dependencies go here
end
end
Dependency Management
Adding Dependencies
Edit the deps function in mix.exs:
defp deps do
[
{:ecto_sql, "~> 3.0"},
{:postgrex, ">= 0.0.0"}
]
end
Note: Unlike npm or cargo, Mix lacks a mix deps.add
command—edit mix.exs manually.
Restoring Dependencies
mix deps.get # Fetches dependencies
mix deps.compile # Compiles them
Updating Dependencies
Update a single package:
mix deps.update ecto_sql
Update all packages:
mix deps.update --all
Mix uses a mix.lock
file to ensure consistent versions across environments.
Common Workflows
Compiling the Project
mix compile
Running Tests
mix test
Extending Mix
Aliases
Mix aliases are custom shortcuts defined in your mix.exs file to simplify or combine repetitive tasks. They allow you to:
Chain multiple Mix commands into one.
Create project-specific workflows.
Simplify complex build/test/deploy processes.
def project do
[
...,
aliases: aliases()
]
end
defp aliases do
[
build_test: ["compile", "test"],
"build:test": ["compile", "test"]
]
end
Run with:
mix build_test
Custom Tasks
Create reusable tasks (e.g., lib/mix/tasks/hello.ex
):
defmodule Mix.Tasks.Hello do
@moduledoc "Prints a greeting: `mix help hello`"
use Mix.Task
@shortdoc "Prints 'hello' to the console"
def run(_args) do
IO.puts("hello")
end
end
Run with:
mix hello
Why Mix Shines
Extensible: Frameworks like Phoenix and Ecto add their own tasks (e.g., mix ecto.gen.repo).
Batteries Included: Built-in tasks for testing, compilation, and dependency management.
Community-Driven: Most Elixir libraries integrate seamlessly with Mix.
Conclusion
Mix is the backbone of Elixir development. Its simplicity for common tasks and flexibility for customization make it indispensable.
Next Steps:
Explore Mix’s official documentation.
Learn advanced workflows with Elixir School.
Subscribe to my newsletter
Read articles from Rafael Andrade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
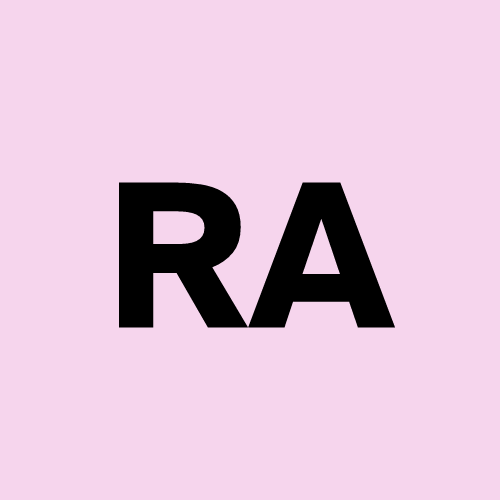