Exploring Embedded Systems: Programming PSoC with C
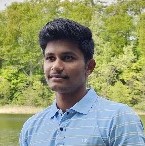
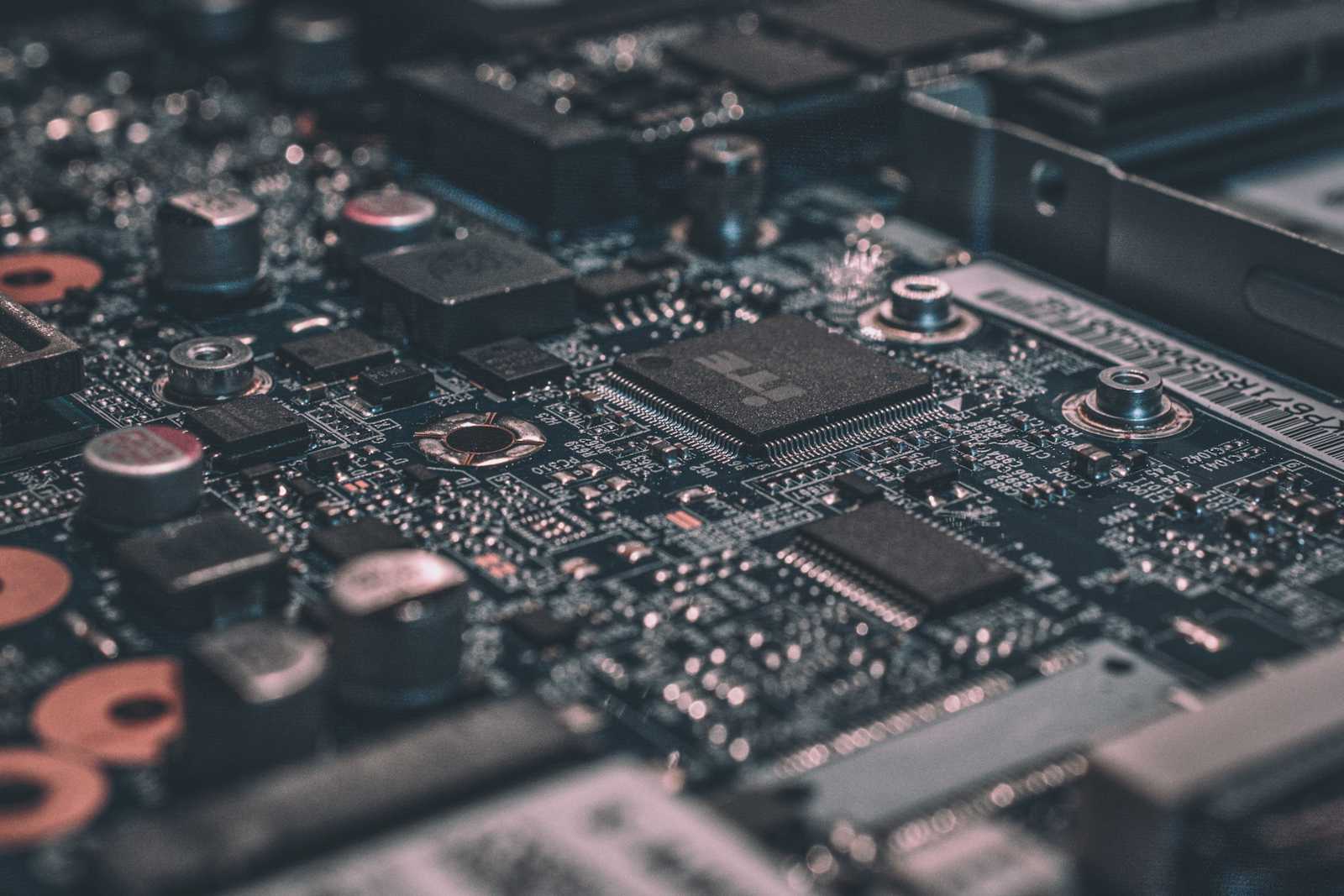
Introduction
Embedded systems play a crucial role in modern electronics, from consumer gadgets to industrial applications. As a Computer Engineering Technology student, I had the opportunity to work with PSoC (Programmable System on Chip) and write a C program to generate and display random characters on an LCD. This blog details my journey through coding, debugging, and testing an embedded system project.
Understanding the Project
The goal of this project was to use the PSoC microcontroller to generate random delays and display characters on an LCD screen. The Pseudo-Random Sequence (PRS) module was used for generating random numbers, which were then used to create dynamic time intervals for character display.
Writing the C Code
The program was written in C and uploaded to the PSoC microcontroller. Below is a breakdown of the code:
Defining Constants and Variables
A message (e.g., "Wednesday") was stored as a character array.
Two unsigned integer variables (
uint8_t
anduint16_t
) were defined for random delay calculations.
Initializing Components
The LCD display and clock were initialized at the start.
The PRS module was activated to generate random numbers.
Main Loop Execution
The program continuously generates random delays between 200ms and 600ms.
Characters from the message are displayed one by one at these intervals.
A 500ms delay is added after the full message is displayed before restarting the loop.
Code Snippet
#include "project.h"
#include <stdio.h>
int main(void)
{
const char Message[] = "THIRSDAY";
uint8 i;
uint16 randomDelay;
LCD_Start();
CLK1_Start();
RandSys_Start();
for (;;)
{
LCD_ClearDisplay();
CyDelay(500);
for (i = 0; i < strlen(Message); i++)
{
randomDelay = (RandSys_Read() % 401) + 200;
LCD_Position(0, i);
LCD_PutChar(Message[i]);
CyDelay(randomDelay);
}
CyDelay(500);
}
}
Testing and Debugging
Once the code was written, it was uploaded to the PSoC microcontroller. Here’s how I tested it:
Ensured the LCD properly initialized and displayed text.
Verified the random delays by monitoring how letters appeared on the screen.
Debugged minor issues related to timing mismatches and display flickering by adjusting delay values.
Confirmed that the infinite loop ran without crashes or memory overflow.
Key Learnings
Importance of PRS: Using a Pseudo-Random Sequence Generator helps create realistic timing variations.
Efficient Memory Usage: Embedded systems have limited resources, so optimizing variable types (
uint8_t
anduint16_t
) is critical.Debugging on Embedded Devices: Unlike traditional programming, debugging in an embedded system requires hardware monitoring and careful observation of output behavior.
Media
Animation
Conclusion
This project enhanced my understanding of real-time embedded programming and hardware-software integration. By working with C programming, PSoC microcontrollers, and LCD displays, I gained hands-on experience with practical applications of embedded systems.
Subscribe to my newsletter
Read articles from Neel Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
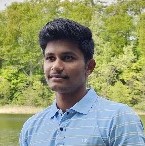