Using ls in C

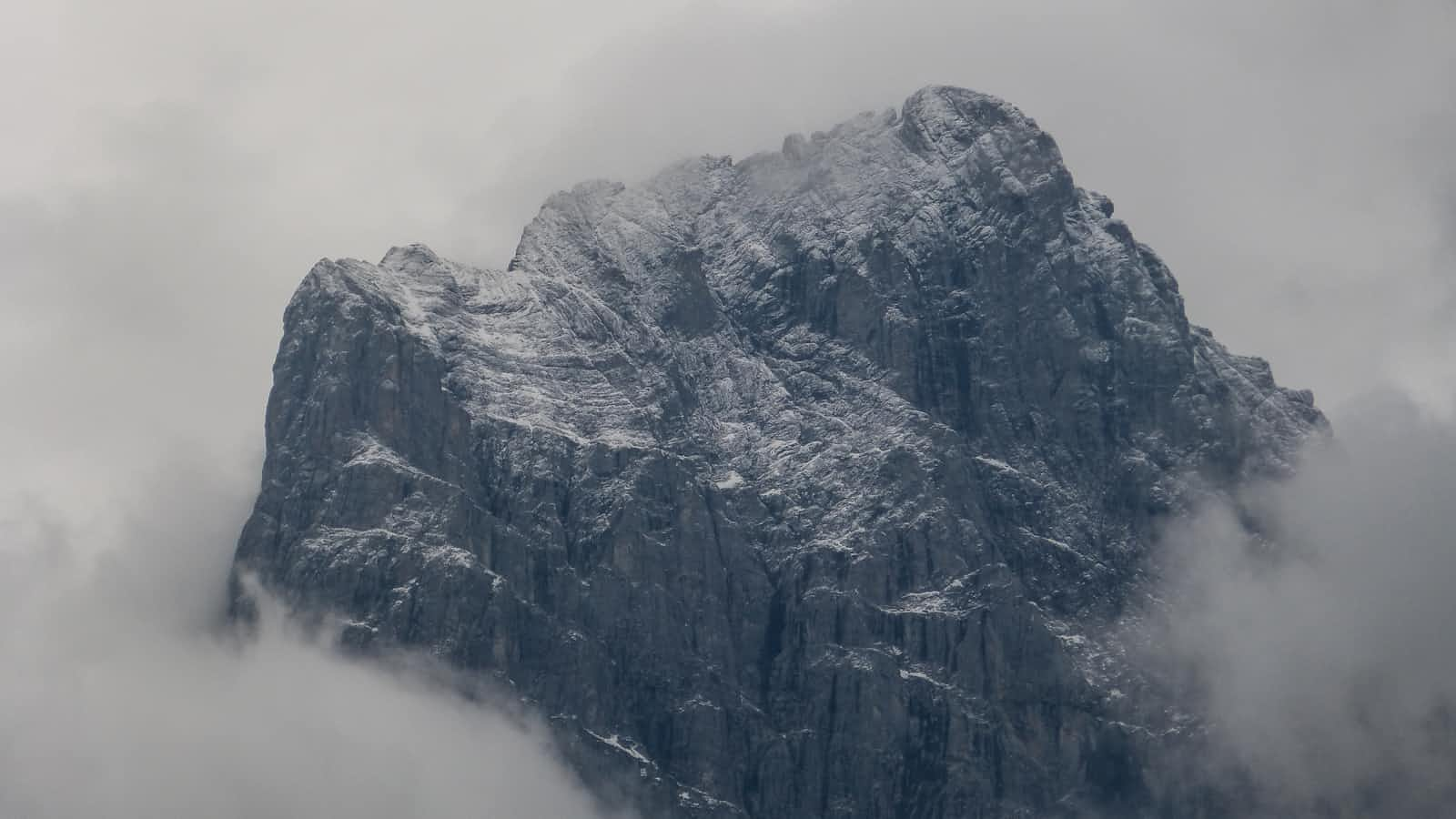
This program prints the contents of a directory using the ls -l command. It checks initially if a directory path is supplied as a command-line argument. If an argument is supplied, it sets that path to the dirpath variable. Otherwise, it uses the current directory. The program then uses execlp to substitute the current process with the ls -l command, displaying the directory contents in long format. If execlp returns unsuccessfully, an error message is output with perror, and the program terminates with a failure status. The return statement at the end never gets called if execlp executes successfully because execlp substitutes the current process.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
const char *dirpath;
// If a directory path is provided as an argument, use it
if (argc > 1) {
dirpath = argv[1];
}
else{
dirpath=".";
}
// Use execlp to call "ls -l <directory>"
if (execlp("ls", "ls", "-l", dirpath, (char *)NULL) == -1) {
perror("execlp"); //Here is the main part of the code
exit(EXIT_FAILURE);
}
return 0;
}
Hope you learnt something new today.
Happy Coding…
Subscribe to my newsletter
Read articles from Gagan G Saralaya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
