Race Conditions in Frontend API Development

Table of contents
- Common Race Condition Scenarios in Mobile Apps
- 1. Rapid Search or Filter Interactions
- 2. Repeated Button Taps
- 3. Pagination with Quick Scrolling
- 4. Form Submissions with Background Validation
- The Real-World Impact
- Solution 1: Sequence Numbering
- Solution 2: Request Cancellation
- Solution 3: Debouncing and Throttling
- The Optimal System Architecture
- Implementation Considerations
- Parting Shot
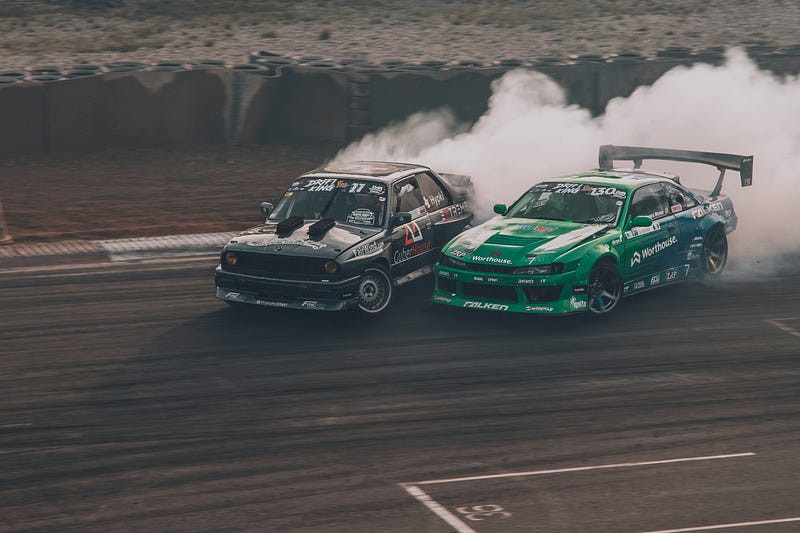
Photo by Ralfs Blumbergs on Unsplash
What Exactly Is a Race Condition?
Imagine the excitement at a Grand Prix starting line: Formula 1 cars revving and pawing the dirt, MotoGP bikes leaning into position, and fighter jets prepping for an air show. Each represents speed, power, and a carefully orchestrated sequence.
But what if the race officials lost track of who crossed the finish line first? What if the photo finish camera captured results out of order, declaring yesterday’s runner-up today’s champion despite clear evidence to the contrary?
This is precisely what happens in a race condition: two or more operations that should happen in a specific sequence end up executing in an unpredictable order, leading to unexpected results. In frontend development using REST APIs, this typically happens when multiple API requests race against each other like thoroughbreds down the stretch — they’re sent in a logical sequence, but their responses return in an unpredictable order based on network conditions, server load, and processing time.
The starting gun fires in sequence, but you can’t predict which horse will cross the finish line first — or which API response will arrive first. And just like declaring the wrong winner would throw the entire race into chaos, accepting outdated API responses can wreak havoc on your application’s state.
Imagine you’re managing a city’s traffic from a central control room. You have cars on the road sending updates about traffic conditions.
Car #1 leaves first on the main route, while Car #2 leaves a moment later but takes a faster alternate route. Although Car #1 departed earlier, Car #2 arrives at its destination first and sends back traffic data. Later, Car #1 finally arrives and sends its (now outdated) traffic report.
If your traffic control system simply displays the most recently received data, it would show the outdated information from Car #1, even though that data is less current than what Car #2 already reported. This is precisely how race conditions work in frontend applications.
Common Race Condition Scenarios in Mobile Apps
1. Rapid Search or Filter Interactions
When a user types quickly in a search field, each keystroke might trigger an API request. The response for “app” might arrive after the response for “apple,” showing incorrect results.
2. Repeated Button Taps
A user taps “Refresh” multiple times, creating several identical requests. The older responses might override newer ones, causing data to appear to “jump backward.”
3. Pagination with Quick Scrolling
Scrolling rapidly through paginated content can trigger multiple page requests that return out of order, showing content from page 2 after content from page 3.
4. Form Submissions with Background Validation
Form fields validate against the server while a user is typing, but responses return in an unexpected order, marking a field as invalid even after it’s been corrected.
The Real-World Impact
Race conditions aren’t just theoretical concerns. They create tangible problems here some of them:
- Flickering UI: Content appears, disappears, then reappears as outdated responses arrive
- Data Inconsistency: Users see incorrect or outdated information
- Wasted Resources: Unnecessary API calls consume bandwidth and server resources
- Degraded User Experience: Unpredictable behavior frustrates users
Solution 1: Sequence Numbering
This approach is the foundation of race condition management:
- Assign a sequential ID or timestamp to each request
- Store the ID of the most recent request for each request type
- When responses return, only process those matching the latest request ID
- Discard responses from older requests, even if they arrive later
This pattern solves the fundamental issue by enforcing a logical order regardless of actual arrival time.
Solution 2: Request Cancellation
When a new request is made:
- Cancel any in-flight requests of the same type
- Only the most recent request will complete and return a response
- Earlier requests never complete, eliminating the possibility of outdated responses
This approach works well when your HTTP client supports cancellation (like fetch with AbortController or Axios with CancelToken).
Solution 3: Debouncing and Throttling
These techniques prevent race conditions by controlling when requests are sent:
Debouncing: Wait until user input pauses before sending a request
- Perfect for search fields and form inputs
- Waits a short period (e.g., 300ms) after the last keystroke before sending
Throttling: Limit how frequently requests can be sent
- Ideal for scroll events or button taps
- Allows at most one request per time interval (e.g., once every 500ms)
The Optimal System Architecture
For the most robust solution, combine these approaches into a cohesive system:
- Input Layer: Apply debouncing to user interactions
- Request Manager: Implement sequence numbering for all requests
- Response Handler: Validate responses against the current sequence state
- State Updates: Only apply updates from the most recent valid responses
This layered approach addresses race conditions at multiple levels, creating a resilient system that maintains data consistency regardless of network timing.
Implementation Considerations
When implementing these solutions:
- Keep It Centralized: Use a single request manager for consistent handling
- Make It Reusable: Create patterns you can apply across your application
- Consider the UX: Add loading indicators to keep users informed
- Handle Edge Cases: Plan for timeout scenarios and error states
Parting Shot
Race conditions in frontend API interactions are a system design challenge that requires architectural thinking rather than just code-level fixes. By understanding the underlying patterns and implementing a structured approach to request management, you can create applications that remain stable and consistent regardless of network behavior.
Remember the traffic control room: always trust the most recent information, not the most recently received information. This principle forms the foundation of reliable frontend-backend communication.
Subscribe to my newsletter
Read articles from Julius Ndegwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
