CrossFi’s Scalability Secrets: Simulating High-Throughput Transactions in Solidity

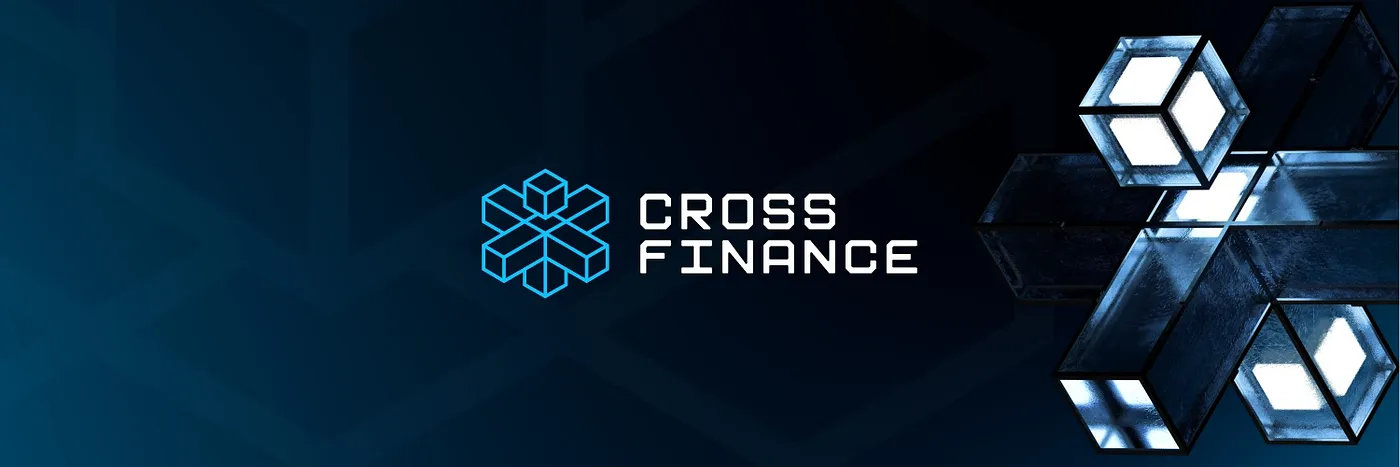
In the rapidly evolving world of blockchain technology, scalability remains a critical hurdle — particularly for systems that span multiple chains [i.e., cross-chain interactions]. CrossFi, a conceptual framework blending cross-chain interoperability with financial innovation, offers a compelling approach to processing high volumes of transactions efficiently. By leveraging Solidity, the programming language powering Ethereum smart contracts, CrossFi demonstrates how to simulate and optimize transaction throughput in a cross-chain environment.
This article takes a look at Transactions with high-throughput simulation in solidity, CrossFi’s simulation strategies, cross-chain mechanisms, and practical Solidity implementations.
The Scalability Challenge in Cross-Chain Systems
Blockchain networks, while secure and decentralized, often struggle to match the transaction speeds of traditional systems. Ethereum, for instance, processes around 20–30 transactions per second (TPS), far below the thousands managed by centralized payment networks. This challenge intensifies in cross-chain scenarios, where assets or data must move between distinct blockchains with unique protocols and performance limits. CrossFi addresses this by focusing on two core principles:
High-Throughput Simulation: Modeling large-scale transaction processing using Solidity.
Cross-Chain Efficiency: Streamlining interactions between blockchains to minimize delays and costs.
Let’s examine these strategies in detail.
Strategy 1: Simulating High-Throughput Transactions in Solidity
Simulation is key to understanding how a system behaves under load. CrossFi uses Solidity smart contracts to emulate high-throughput scenarios, allowing developers to stress-test cross-chain transaction flows without real-world costs. This involves batching transactions, optimizing gas usage, and leveraging off-chain computation where possible.
Solidity Example: Transaction Batching
Here’s a simplified Solidity contract that batches multiple transactions into a single call, reducing the number of on-chain operation:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract TransactionBatcher {
address public administrator;
uint256 public processedTransactions;
event BatchCompleted(uint256 transactionCount, uint256 totalValue);
constructor() {
administrator = msg.sender;
}
// Process multiple transactions in a single call
function executeBatch(address[] memory recipients, uint256[] memory amounts) external {
require(msg.sender == administrator, "Restricted to administrator");
require(recipients.length == amounts.length, "Input arrays must match");
uint256 totalValue = 0;
for (uint256 i = 0; i < recipients.length; i++) {
require(recipients[i] != address(0), "Invalid recipient address");
require(amounts[i] > 0, "Amount must be greater than zero");
totalValue += amounts[i];
processedTransactions++;
}
emit BatchCompleted(recipients.length, totalValue);
}
}
How It Works:
The executeBatch function accepts lists of recipients and amounts, processing them as a single unit.
Instead of submitting each transaction individually (costly in gas and time), they’re processed in a single call..
In a real deployment, this could integrate with a token contract (e.g., ERC-20) to execute transfers.
Scalability Benefit: Batching reduces the number of individual blockchain operations, lowering costs and accelerating confirmation times — a cornerstone of CrossFi’s scalability.
Strategy 2: Facilitating Cross-Chain Transfers
Cross-chain interoperability is central to CrossFi’s vision. Moving assets between blockchains requires a mechanism to “lock” them on one chain and “unlock” equivalents on another, ensuring security and speed. CrossFi achieves this through bridge contracts, which coordinate these transfers efficiently.
Solidity Implementation: Cross-Chain Bridge
Below is a basic bridge contract simulating asset locking and unlocking across chains:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract CrossChainBridge {
mapping(address => uint256) public lockedAssets;
address public bridgeOperator;
uint256 public totalLocked;
event AssetLocked(address indexed user, uint256 amount, bytes32 targetChainTx);
event AssetUnlocked(address indexed user, uint256 amount);
constructor() {
bridgeOperator = msg.sender;
}
// Lock assets on the source chain
function lockAssets(uint256 amount, bytes32 targetChainTx) external payable {
require(amount > 0 && msg.value == amount, "Invalid amount");
lockedAssets[msg.sender] += amount;
totalLocked += amount;
emit AssetLocked(msg.sender, amount, targetChainTx);
}
// Unlock assets (called by operator after cross-chain verification)
function unlockAssets(address user, uint256 amount, bytes32 sourceChainProof) external {
require(msg.sender == bridgeOperator, "Only operator can unlock");
require(lockedAssets[user] >= amount, "Insufficient locked funds");
lockedAssets[user] -= amount;
totalLocked -= amount;
payable(user).transfer(amount);
emit AssetUnlocked(user, amount);
}
// Simulate cross-chain verification (placeholder)
function verifyCrossChainTx(bytes32 proof) external view returns (bool) {
// In practice, this would verify a proof from the target chain
return true;
}
}
How It Works:
lockAssets: Users lock funds on the source chain, emitting an event with a targetChainTx identifier for the destination chain.
unlockAssets: The bridge operator (a trusted or decentralized entity) verifies the cross-chain event and releases funds.
In a full implementation, verifyCrossChainTx would integrate with a relay or oracle to confirm the target chain’s state.
Scalability Benefit: By locking assets in bulk and using off-chain verification (e.g., via a relay), CrossFi minimizes on-chain computation, enabling high-throughput cross-chain transfers.
Strategy 3: Optimizing for Efficiency
High transaction volumes demand efficiency. CrossFi optimizes its Solidity contracts to reduce operational costs (known as “gas” on Ethereum) and streamline execution. Techniques include minimizing redundant calculations, leveraging events for off-chain monitoring, and processing tasks in batches wherever possible.
CrossFi’s Unified Approach
CrossFi integrates these strategies into a cohesive system:
Simulation: Solidity-based testing ensures the framework can handle large-scale transaction loads.
Interoperability: Bridge contracts enable seamless asset movement across blockchains.
Efficiency: Optimized designs keep costs low and performance high.
By simulating thousands of transactions per second in a controlled environment, CrossFi refines its architecture before deployment. This proactive strategy ensures scalability without sacrificing security or decentralization — key tenets of the blockchain trilemma.
Conclusion
CrossFi’s scalability secrets lie in its innovative use of Solidity to simulate and execute high-throughput transactions across blockchains. By batching operations, streamlining cross-chain transfers, and prioritizing efficiency, it offers a blueprint for overcoming the performance barriers of decentralized systems.
The examples provided demonstrate practical applications of these concepts, showcasing how CrossFi could transform blockchain interoperability. As the demand for fast, reliable cross-chain solutions grows, CrossFi’s strategies provide a professional and scalable path forward.
Subscribe to my newsletter
Read articles from Abdullahi Raji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
