Macros in Rust
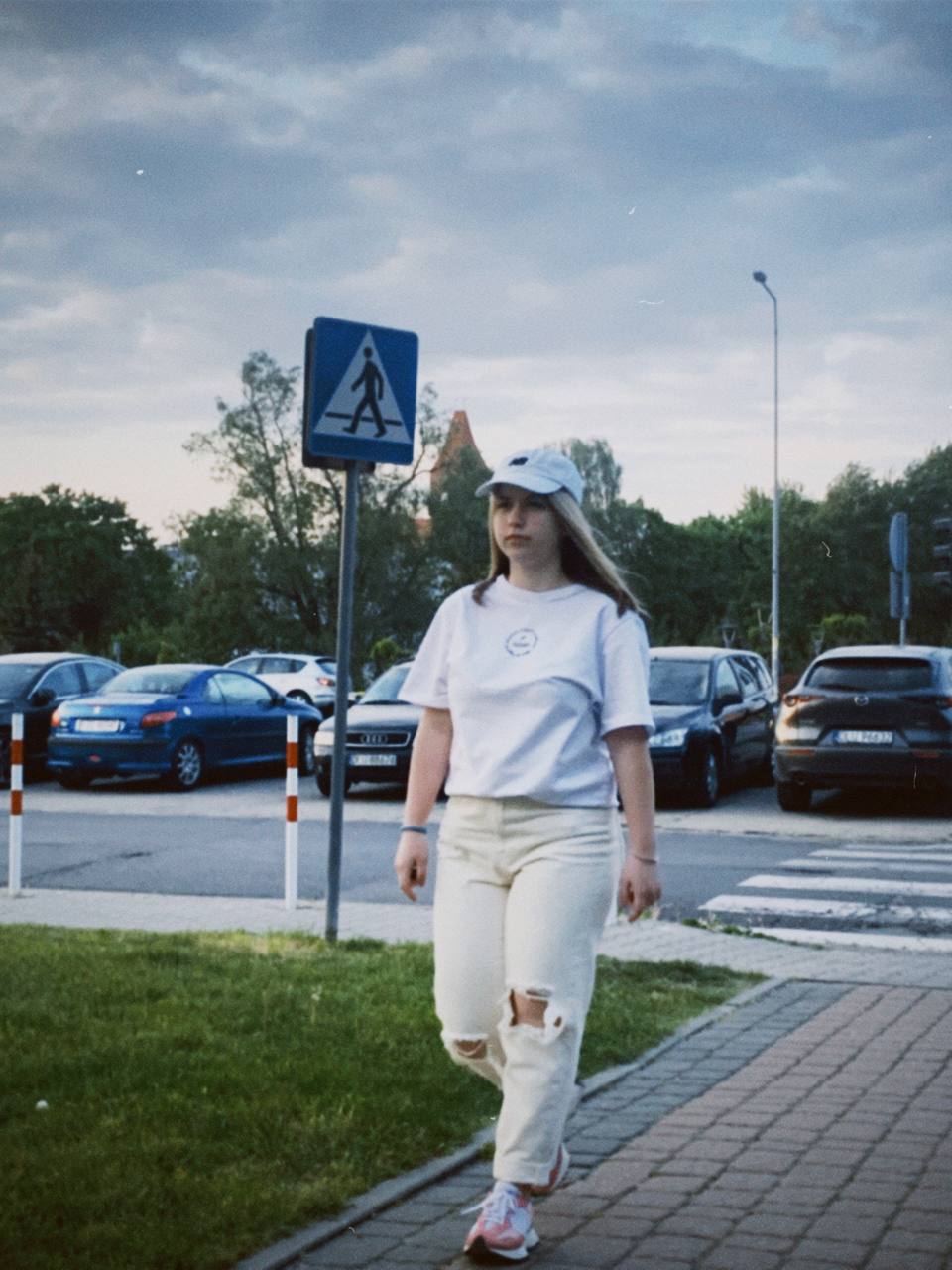
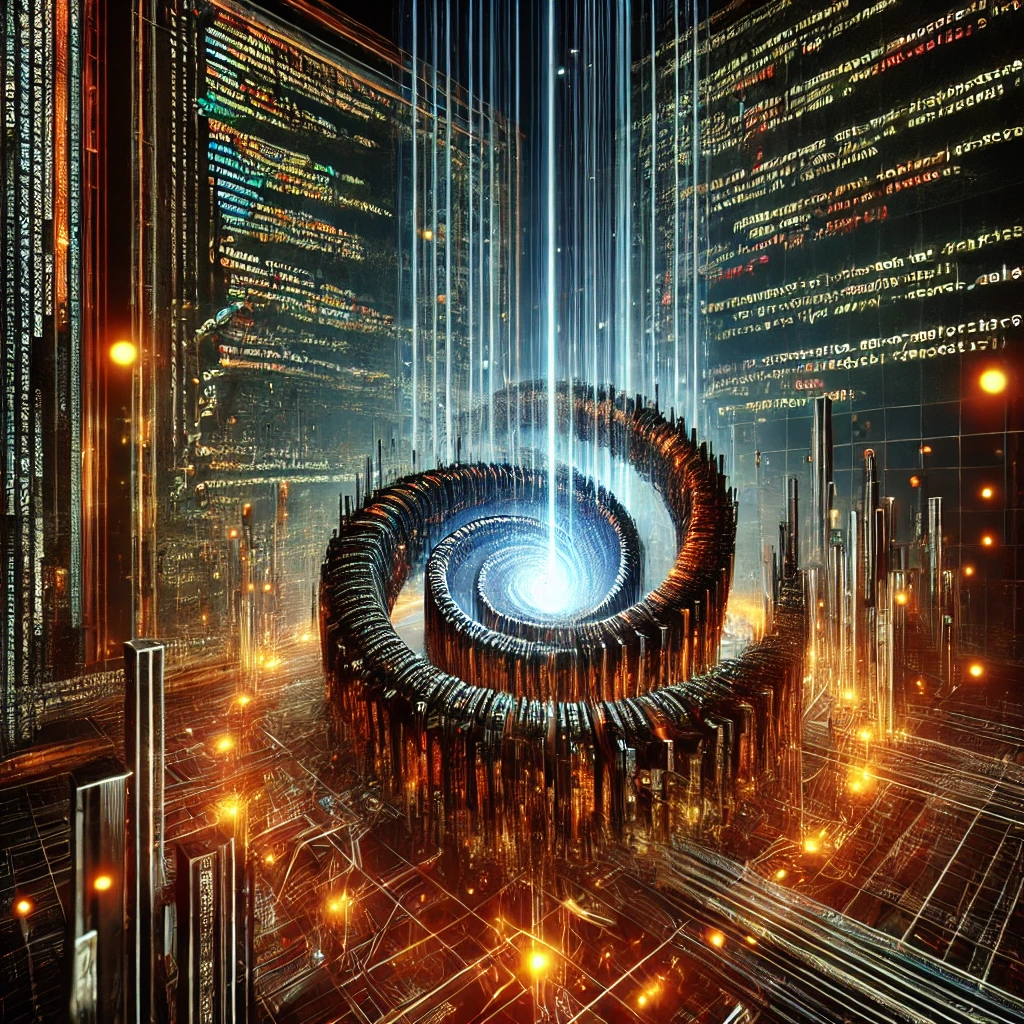
Introduction
In Rust macros are very powerful tools that generate code at compile time. Unlike functions, which execute predefined operations at runtime, macros write code, allowing you to dynamically create code during compilation.
The biggest advantage of using them - is their flexibility. You don’t need to set a fixed number of inputs, as they can accept a variable number of inputs. This capability helps reduce boilerplate and lets you write more expressive and concise code.
In this article, we’ll explore how Rust macros work and how they differ from functions.
Macros Types
There are 2 types of macros in Rust:
Procedural Macros
Declarative Macros
Procedural Macros
Procedural macros - take code as input → operate on that code → produce new code
Procedural macros look similar to a Rust function taking in a token streams and returning a token stream.
Procedural macro:
Derive
Function-like
Attribute
Derive macros
A derive procedural macro lets you create custom behaviour when a user writes #[derive(YourMacroName)] on a struct and enum. The macro receives the annotated item’s code as input, processes it, and then produces additional code that is added to your program.
Function-like macros
This type of macros considers one of the easiest out of 3.
Example of function-like macros in Rust:
use proc_macro::TokenStream;
#[proc_macro]
pub fn fuction_like_macros(data: TokenStream) -> TokenStream {
data
}
Attribute-like macros
Basically, attribute-like procedural macros let you attach custom behavior to your code by simply adding an annotation above it. When you write something like #[my_macro(...)] above a function, struct or another item, the macro takes two parts: the extra details you might include inside the parentheses (if any), and the actual code item itself. It then processes these inputs and returns new code that replaces the original item.
There is an example of how to implement a procedural macro attribute called log_calls. This macro wraps a function’s body so that whenever the function is called, it prints messages before and after executing the original code. In this example, the macro is defined in its own crate and then used in a binary crate.
1. Procedural Macro Crate (log_calls_macro)
Cargo.toml
[package]
name = "log_calls_macro"
version = "0.1.0"
edition = "2021"
[lib]
proc-macro = true
[dependencies]
syn = { version = "1.0", features = ["full"] }
quote = "1.0"
proc-macro2 = "1.0"
src/lib.rs
extern crate proc_macro;
use proc_macro::TokenStream;
use quote::quote;
use syn::{parse_macro_input, ItemFn};
/// This attribute macro wraps a function so that it logs when the function is entered and exited.
#[proc_macro_attribute]
pub fn log_calls(_attr: TokenStream, item: TokenStream) -> TokenStream {
// Parse the input tokens into a function syntax tree.
let mut input = parse_macro_input!(item as ItemFn);
let fn_name = input.sig.ident.clone();
// Save the original function block.
let original_block = input.block;
// Create a new block that wraps the original block with log statements.
input.block = syn::parse_quote!({
println!("Entering function: {}", stringify!(#fn_name));
let __log_calls_result = { #original_block };
println!("Exiting function: {}", stringify!(#fn_name));
__log_calls_result
});
// Return the modified function as a TokenStream.
TokenStream::from(quote! {
#input
})
}
2. Binary Crate Using the Macro (log_calls_example)
Cargo.toml
[package]
name = "log_calls_example"
version = "0.1.0"
edition = "2021"
[dependencies]
log_calls_macro = { path = "../log_calls_macro" }
src/main.rs
use log_calls_macro::log_calls;
#[log_calls]
fn add(a: i32, b: i32) -> i32 {
a + b
}
#[log_calls]
fn say_hello(name: &str) {
println!("Hello, {}!", name);
}
fn main() {
let sum = add(2, 3);
println!("Sum is {}", sum);
say_hello("Alice");
}
Explanation
• Procedural Macro Crate:
The log_calls macro parses the input function (using the syn crate) to obtain its name and body. Then it creates a new block that prints a message before executing the original function body and another message afterward. Finally, it returns the modified function using the quote crate.
• Binary Crate Usage:
In the log_calls_example crate, the macro is applied to two functions: add and say_hello. When these functions are called, the console will display log messages indicating when each function is entered and exited, along with the original behaviour (calculating a sum or printing a greeting).
This implementation provides a straightforward way to see how attribute procedural macros can modify function behaviour by injecting additional code without changing the function’s original logic.
Declarative Macros
Declarative macros - match patterns → replace code with other code
#[macro_export] - attribute which makes the macro visible outside the crate where it is defined. So, if you define the macro inside a submodule, #[macro_export] automatically re-exports it at the root of the crate. This allows us to call macro directly without specifying its module path.
mod example {
#[macro_export]
macro_rules! example_macro {
() => {
println!("This is our created macro");
};
}
}
fn main() {
// We can call the macro directly even tho it is inside the "example" module,
// as #[macro_export] re-exports it to the crate root.
example_macro!();
}
macro_rules! is a tool in Rust that lets you create custom shortcuts for code. Instead of writing the same code over and over, you define a rule that tells the compiler to automatically replace a certain pattern with a block of code you specify.
example_macro - in our particular case is a name of our macros, but you can call how you want.
Rules syntax
($matcher) => {$expansion};
Matcher is a template which tells the compiler what pattern to look for.
Expansion is the block of code that gets substituted in its place.
Rules should be separated using semicolons (last one can be optional).
Basically, when the compiler sees the pattern you defined, it automatically replaces it with the specified code, streamlining repetitive tasks without adding extra visible code to your final program.
Subscribe to my newsletter
Read articles from Yelyzaveta Dymchenko directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
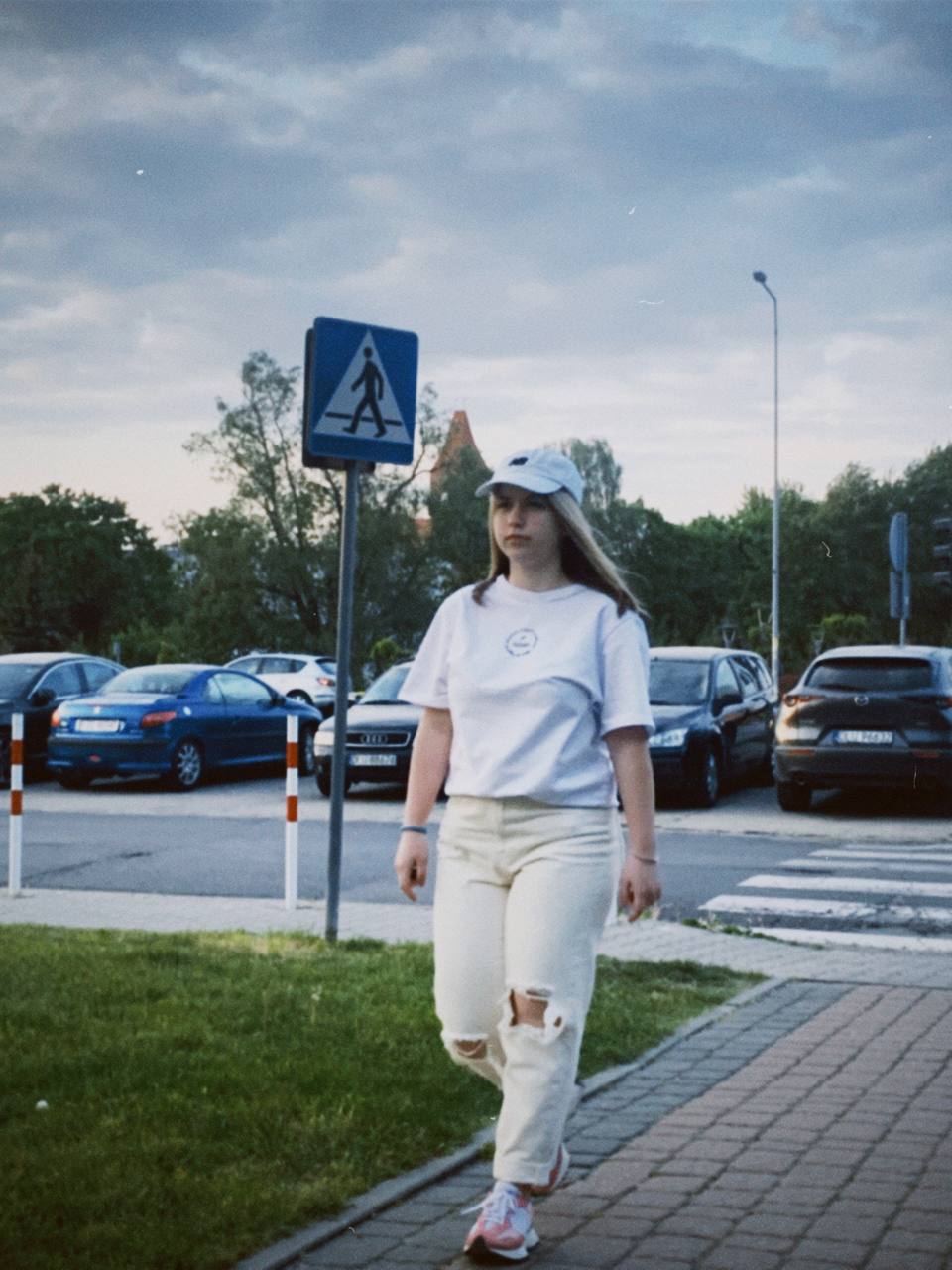