Building a Simple Calculator Using HTML, CSS, and JavaScript

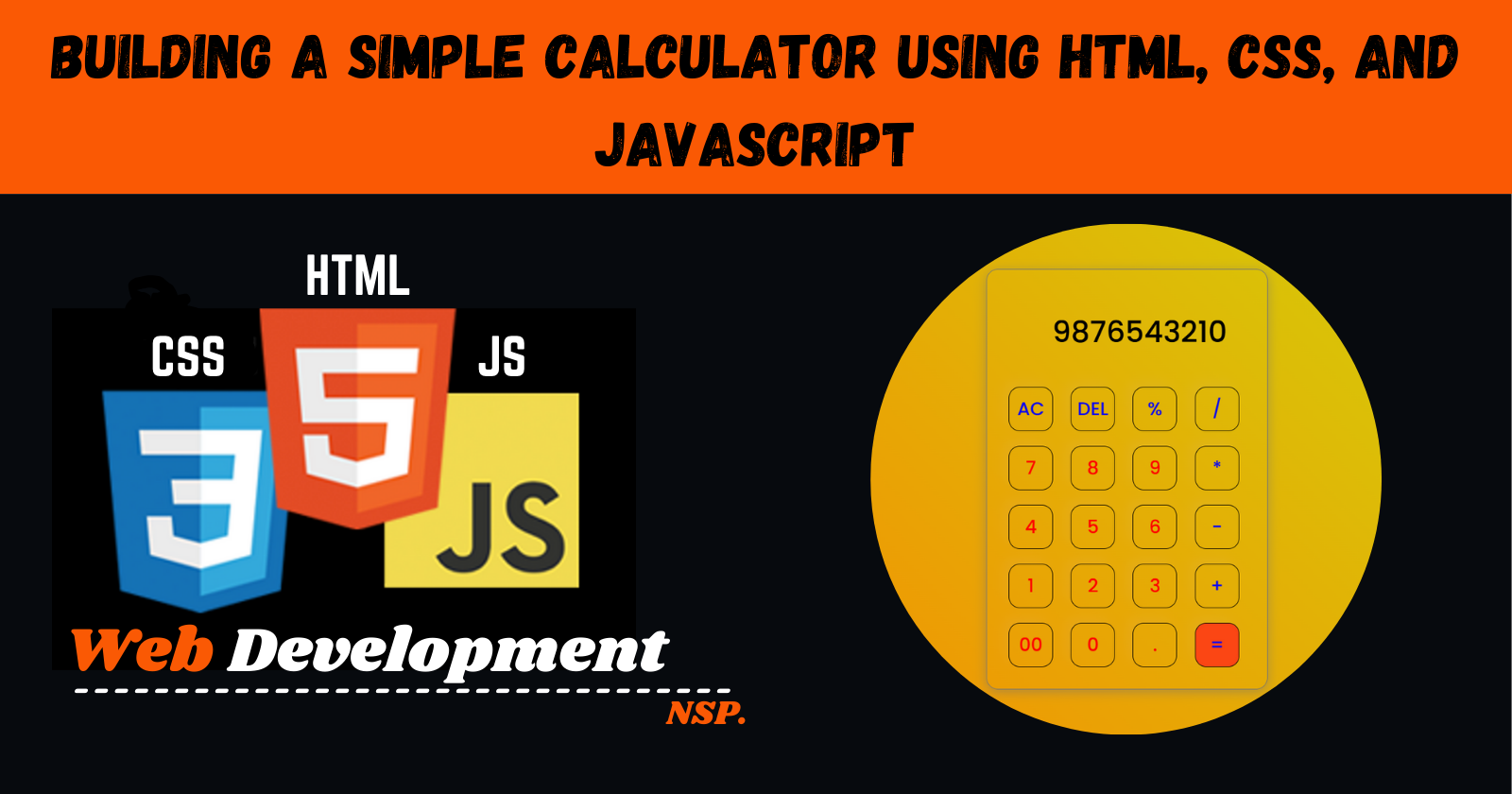
Introduction
- A calculator is a great beginner-friendly project that helps in understanding DOM manipulation, event handling, and styling. In this blog, I'll walk you through building a simple calculator using HTML, CSS, and JavaScript.
Features of My Calculator
Performs basic arithmetic operations (+, -, *, /).
Has AC (All Clear) and DEL (Delete one character) functions.
Uses JavaScript for event handling and real-time calculations.
Responsive and visually appealing design with a gradient background.
Skills Used
HTML → Creates the calculator structure.
CSS → Styles the calculator for a modern look.
JavaScript → Adds interactivity (calculations, button clicks).
HTML Code (Structure of Calculator)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Calculator - By Sriparthu</title>
</head>
<body>
<div class="container">
<div class="calculator">
<input type="text" id="inputBox" placeholder="0" />
<div>
<button class="button operator">AC</button>
<button class="button operator">DEL</button>
<button class="button operator">%</button>
<button class="button operator">/</button>
</div>
<div>
<button class="button">7</button>
<button class="button">8</button>
<button class="button">9</button>
<button class="button operator">*</button>
</div>
<div>
<button class="button">4</button>
<button class="button">5</button>
<button class="button">6</button>
<button class="button operator">-</button>
</div>
<div>
<button class="button">1</button>
<button class="button">2</button>
<button class="button">3</button>
<button class="button operator">+</button>
</div>
<div>
<button class="button">00</button>
<button class="button">0</button>
<button class="button">.</button>
<button class="button equalBtn">=</button>
</div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Explanation of HTML Code
The
<input>
field is used to display numbers and results.Buttons are arranged in a grid layout using
<div>
elements.Buttons like *AC, DEL, %, /, , -, +, = have an operator class for special styling.
The equal button (
=
) has a separate class (equalBtn
).The
<script>
tag includesscript.js
for adding interactivity.
CSS Code (Styling the Calculator)
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@500&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background: linear-gradient(45deg, #f98603, #cfd90a);
}
.calculator {
border: 1px solid #717377;
padding: 20px;
border-radius: 16px;
background: transparent;
box-shadow: 0px 3px 15px rgba(113, 115, 119, 0.5);
}
input {
width: 320px;
border: none;
padding: 24px;
margin: 10px;
background: transparent;
font-size: 40px;
text-align: right;
color: #000;
}
input::placeholder {
color: #000;
}
button {
width: 60px;
height: 60px;
margin: 10px;
border-radius: 15px;
background: transparent;
color: red;
font-size: 25px;
box-shadow: -8px -8px 15px rgba(223, 185, 153, 0.1);
cursor: pointer;
}
.equalBtn {
background-color: #fb4614;
color: #0e0aee;
}
.operator {
color: #0e0aee;
}
Explanation of CSS Code
Poppins font is used for a modern look.
The background has a gradient color for an attractive UI.
Buttons are given rounded corners and shadows.
The
equalBtn
has a different background color for emphasis.
JavaScript Code (Adding Functionality)
let input = document.getElementById('inputBox');
let buttons = document.querySelectorAll('button');
let string = "";
let arr = Array.from(buttons);
arr.forEach(button => {
button.addEventListener('click', (e) => {
if (e.target.innerHTML == '=') {
try {
string = eval(string);
input.value = string;
} catch {
input.value = "Error";
}
}
else if (e.target.innerHTML == 'AC') {
string = "";
input.value = string;
}
else if (e.target.innerHTML == 'DEL') {
string = string.slice(0, -1);
input.value = string;
}
else {
string += e.target.innerHTML;
input.value = string;
}
});
});
Explanation of JavaScript Code
Selecting Elements
inputBox
→ The display area of the calculator.buttons
→ All buttons in the calculator.
Handling Button Clicks
If
=
is clicked, it evaluates the expression usingeval()
.If
AC
is clicked, it clears the input field.If
DEL
is clicked, it removes the last character using.slice(0, -1)
.Otherwise, it appends the clicked button’s value to the string.
Output:
Conclusion
- In this blog, we built a fully functional calculator using HTML, CSS, and JavaScript. This project helped me understand:
✅ DOM Manipulation usingquerySelectorAll
✅ Event handling in JavaScript
✅ Styling and UI enhancements with CSS
Happy Learning
Thanks For Reading! :)
-SriParthu💝💥
Subscribe to my newsletter
Read articles from sri parthu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

sri parthu
sri parthu
Hello! I'm Sri Parthu! 🌟 I'm aiming to be a DevOps & Cloud enthusiast 🚀 Currently, I'm studying for a BA at Dr. Ambedkar Open University 📚 I really love changing how IT works to make it better 💡 Let's connect and learn together! 🌈👋