Comparing React Native (Expo) and Flutter: A Developer's Perspective
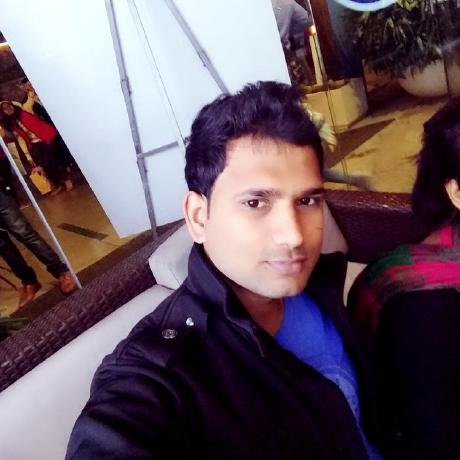
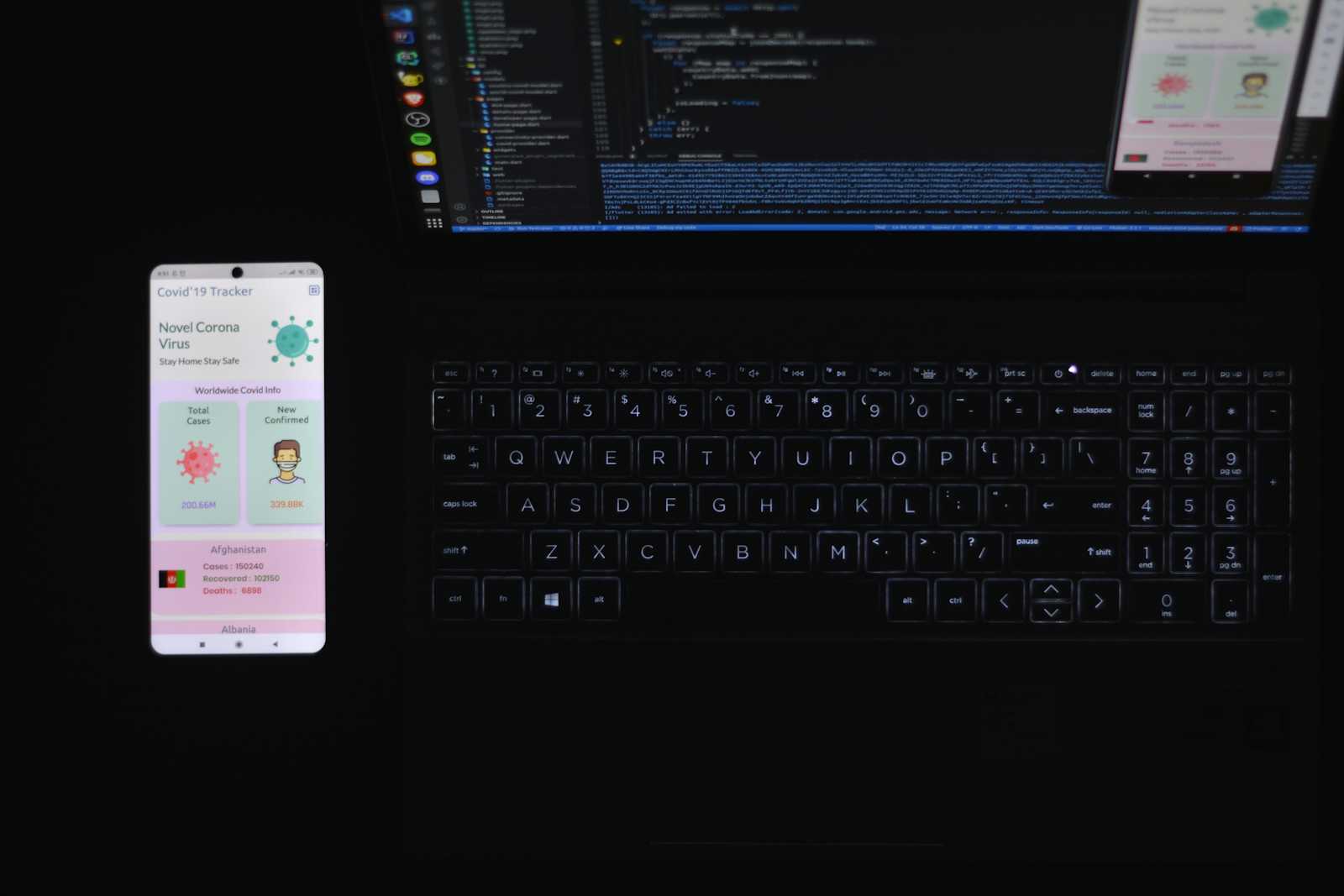
When it comes to cross-platform mobile development, two frameworks stand out prominently: Expo (built on React Native) and Flutter. Both promise to deliver excellent cross-platform applications, but they differ significantly in several aspects. Let's compare them across key parameters that matter to developers.
Performance: Numbers Don't Lie
Flutter
Flutter's performance is often considered exceptional due to its direct compilation to native code and its rendering engine.
Benchmarks:
Startup Time: Flutter apps typically launch in 350-450ms on modern devices
UI Rendering: Flutter's custom rendering engine (Skia) achieves ~60fps consistently, even with complex animations
Memory Usage: Average memory footprint of ~190MB for a typical app
// Flutter animation example - extremely performant even with complex animations
AnimatedContainer(
duration: Duration(seconds: 1),
curve: Curves.fastOutSlowIn,
width: _isExpanded ? 300.0 : 100.0,
height: _isExpanded ? 300.0 : 100.0,
color: _isExpanded ? Colors.blue : Colors.red,
child: FlutterLogo(size: 75),
)
Expo
Expo, being built on React Native, relies on JavaScript bridges to communicate with native components.
Benchmarks:
Startup Time: Typically 700-900ms (standard Expo apps)
UI Rendering: ~55-60fps for most UI interactions, but can drop with complex animations
Memory Usage: Average memory footprint of ~230MB
// Expo/React Native animation - might experience slight jank with complex animations
import { Animated } from 'react-native';
// In component:
const animatedValue = new Animated.Value(0);
Animated.timing(animatedValue, {
toValue: 1,
duration: 1000,
useNativeDriver: true, // Crucial for performance
}).start();
// Usage with interpolation
<Animated.View
style={{
opacity: animatedValue,
transform: [{ scale: animatedValue }]
}}
>
<SomeComponent />
</Animated.View>
Real-world Impact: In apps with highly complex UI or animations, Flutter generally performs better. However, for standard business applications, the difference is often negligible to users.
Syntax Comparison
Flutter (Dart)
// Flutter stateful widget
class CounterScreen extends StatefulWidget {
@override
_CounterScreenState createState() => _CounterScreenState();
}
class _CounterScreenState extends State<CounterScreen> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Counter')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Counter value:'),
Text('$_counter', style: TextStyle(fontSize: 24)),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
child: Icon(Icons.add),
),
);
}
}
Expo (React Native with JavaScript/TypeScript)
// Expo/React Native functional component with hooks
import React, { useState } from 'react';
import { View, Text, Button, StyleSheet } from 'react-native';
export default function CounterScreen() {
const [counter, setCounter] = useState(0);
return (
<View style={styles.container}>
<Text>Counter value:</Text>
<Text style={styles.counterText}>{counter}</Text>
<Button title="Increment" onPress={() => setCounter(counter + 1)} />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
counterText: {
fontSize: 24,
marginVertical: 18,
},
});
Key Differences:
Language: Flutter uses Dart while Expo uses JavaScript/TypeScript
Style Definition: Flutter uses a widget-based approach with inline styles, while Expo uses StyleSheet objects similar to CSS
Component Structure: Flutter uses class-based StatefulWidget/StatelessWidget paradigm; Expo follows React's functional components with hooks or class components
Ecosystem
Flutter
Package Manager: pub.dev with over 30,000 packages
UI Components: Rich set of Material Design and Cupertino widgets out of the box
Tooling: Excellent developer tools, hot reload, and well-documented Flutter CLI
Community: Strong and growing community with good support from Google
Testing: Built-in testing frameworks for unit, widget, and integration testing
Expo
Package Manager: npm/yarn with over 1.3 million packages (though not all are Expo/RN compatible)
Expo SDK: Pre-built solutions for common mobile features (camera, maps, etc.)
Expo Go: Test apps directly on physical devices without complicated setup
EAS Build: Cloud-based build service (major advantage over vanilla React Native)
Community: Massive JavaScript/React ecosystem; very active community
Updates: Over-the-air updates without App Store/Play Store review
Ecosystem Winner: It's a tie, depending on your needs. Flutter's ecosystem is more cohesive, while Expo leverages the massive JavaScript ecosystem and offers exceptional deployment tools.
Ease of Development
Flutter
Pros:
Excellent documentation
Hot reload that preserves state
Consistent behavior across platforms
Strong typing with Dart
Extensive widget inspector
Cons:
Steeper learning curve if you're not familiar with Dart
More verbose UI code with the widget tree structure
Setting up native functionality requires more platform-specific knowledge
Expo
Pros:
Easy setup (create-expo-app)
Familiar to web developers (JavaScript/React)
Expo Go for instant testing on physical devices
Managed workflow abstracts away native code complexity
Over-the-air updates
Cons:
Performance limitations for complex applications
Limited access to native modules in the managed workflow
Ejecting to bare workflow loses some Expo benefits
Bundle size tends to be larger
Development Experience Winner: Expo has a lower barrier to entry, especially for web developers. Flutter offers more control but requires more investment to master.
Conclusion
Choose Flutter if:
Performance is your top priority
You're building a UI-heavy application with complex animations
You prefer a more structured, typed language
You want a more cohesive development experience
Choose Expo if:
You want to leverage JavaScript/React knowledge
Quick development and iteration cycles are important
You need over-the-air updates
You prefer simplified access to native features
Both frameworks are excellent choices for cross-platform development, with their own strengths and tradeoffs. The best choice depends on your team's background, project requirements, and long-term development goals.
Subscribe to my newsletter
Read articles from Ritesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
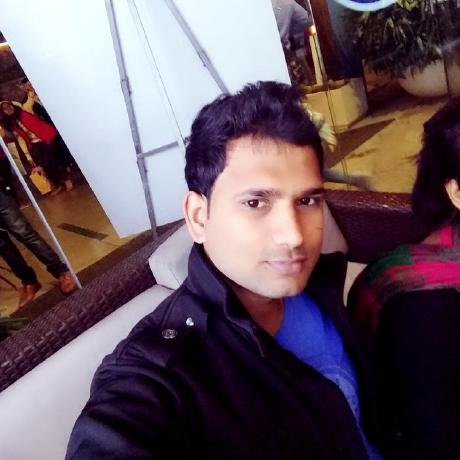