Practicality of useState in React

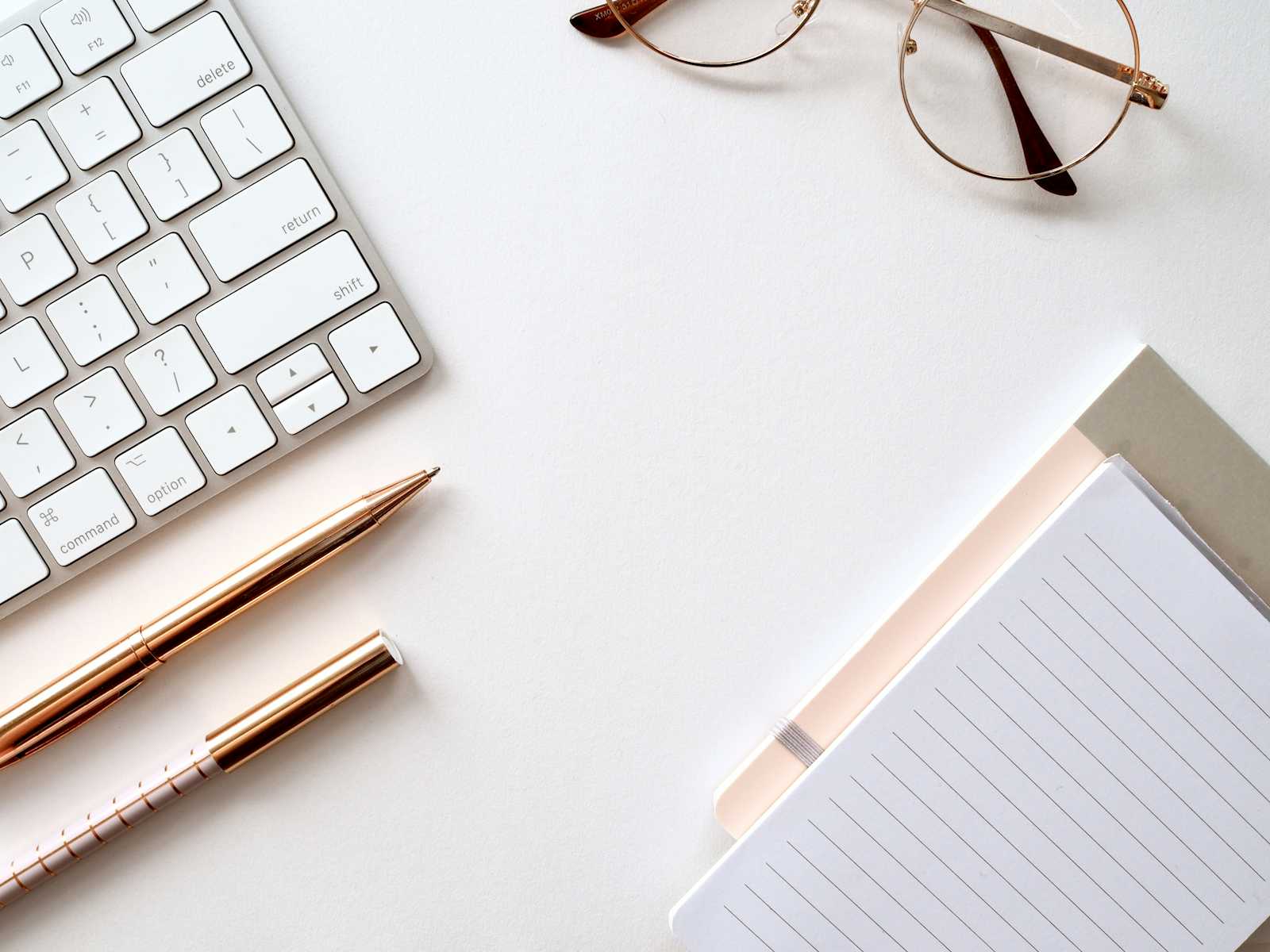
Let's dive into React's useState hook and see how to use it effectively in real-world web applications. We'll cover its practical usage and avoiding common mistakes for better state management.
We will discuss:
🌟 Simple use cases of useState
🌟 Common mistakes and how to avoid them
Let’s understand useState,
First and foremost, we need an idea of what useState is and why we need it. I found a useful point in an article by a senior engineer. He mentioned that first, we need to understand "what problem we are going to solve" before, "how we are going to solve it."
Let's think about updating a count variable when the user presses the button.
We use useState to declare a state variable count with an initial value of 0.
When the button is clicked, the setCount function updates the state.
function Counter() {
const [count, setCount] = useState(0); // Declaring state for count
return (
<div>
<p>Count: {count}</p> {/* UI automatically updates with state */}
<button onClick={() => setCount(count + 1)}>Increment</button> {/* State update triggers re-render */}
</div>
);
}
But someone might wonder why we need this when we can write a regular function inside our React application, like this:
function Counter() {
let count = 0; // A regular JavaScript variable (not state)
function increment() {
count++; // Increment the count (but no re-render)
console.log(count); // Log count value (this shows increment happens)
}
return (
<div>
<p>Count: {count}</p> {/* UI won't update automatically */}
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
The problem here is that when we run this code, the console shows the real-time updates of the count variable, but the DOM itself doesn't reflect these changes. To update the DOM, we'd have to write additional code to manually manipulate the DOM elements. As the application grows, this approach becomes harder to maintain because we would need to manually track and update the DOM each time the state changes.
This is where the useState hook comes in to solve the problem. It’s really simple to understand: it gives us a variable and a function to set a new value to that variable. The magic of useState is that when the state changes, React automatically triggers a re-render, updating the DOM for us. This makes our code cleaner, more efficient, and easier to scale!
Common Mistakes and how to avoid them
◽Directly Modifying State
A common mistake is trying to mutate the state directly, instead of updating it properly using the setter function returned by useState.
❌ Wrong approach
const [count, setCount] = useState(0);
// Directly modifying state count++;
count++;
✔️ Correct approach
const [count, setCount] = useState(0);
// Using the setter function to update state
setCount(count + 1);
React relies on the setter function setCount to track state updates and trigger re-renders. Directly modifying state doesn’t trigger a re-render.
◽what happened if we called two times set function when button clicked?
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1); // First update
setCount(count + 1); // Second update (not based on the first update)
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
}
export default Counter;
When we see this, we might think that when the button is clicked, the count will increase by two because the function is called twice. However, this won't update the DOM as expected. This happens because React uses a batch processing technique to keep our application efficient by avoiding unnecessary re-renders. You can learn more about React's batch-processing using the link below.
https://www.geeksforgeeks.org/what-is-automatic-batching-in-react-18/
➡️ If multiple setState calls happen in a single event, React processes them together instead of re-rendering on each update.
To overcome this challenge, we need to do it the following way:,
const handleClick = () => {
// Use functional update to ensure the correct value is used for each update
setCount(prevCount => prevCount + 1); // First update
setCount(prevCount => prevCount + 1); // Second update
};
The key is to use the functional form of the setCount function, which takes the previous state as an argument and ensures that updates are based on the most up-to-date state.
I would love to hear your thoughts! Feel free to share your insights, questions, or experiences in the comments below. 😊
Subscribe to my newsletter
Read articles from Harshana Prabhath directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
