Til 2025-03-21
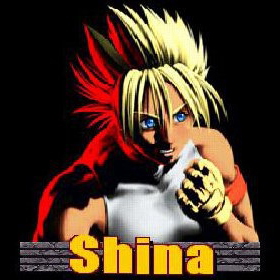
Today I had one issue with React useEffect
and videoJs
.
This initial code has many problems, and one of them is crucial since I didn’t capture it during development time
const { video, videoJsOptions } = props;
const { handleProgress } = useVideoProgress({
videoId: video.id,
});
const options = getVideoPlayerOptions(video, {
...videoJsOptions,
});
useEffect(() => {
// Make sure Video.js player is only initialized once
if (!playerRef.current && videoRef.current) {
// The Video.js player needs to be _inside_ the component el for React 18 Strict Mode.
const videoElement = document.createElement('video-js');
videoElement.classList.add('vjs-big-play-centered');
(videoRef.current as HTMLDivElement).appendChild(videoElement);
const player = videojs(videoElement, options, () => {
let lastUpdateSeconds = -1;
player.on('play', () => {
handlePlay();
});
// Other event listeners...
playerRef.current = player
});
} else {
const player = playerRef.current;
if (player) {
player.autoplay((options as VideoJsOptions).autoplay);
player.src(options.sources);
}
}
}, [options]);
Re-render with object
The most critical issue here is the options
object, it re-renders every time the component re-renders. The issue I faced is whenever the component renders, this options
REFERENCE changes. I didn’t see it in development and only see it when it’s in production.
My useVideoProgress
hook will do some logics that cause this component to re-render, for example, auto-save the user’s progress every 15 seconds, causing this component to re-render.
The result is: the player will be reset to the initial state every 15 seconds.
I was a bit frustrated with this experience. And finally, I can figure out the problem and reproduce the issue by running the production build locally.
The code fixing for it is
const options = useMemo(
() =>
getVideoPlayerOptions(video, {
...videoJsOptions,
}),
[video, JSON.stringify(videoJsOptions)]
);
I don’t care if somebody doesn’t like JSON.stringify
it since they want to import a new fancy library just for comparing objects.
VideoJS problem
The next problem is the rendered videojs, since videojs is NOT a react component, it's just a pure javascript library. These lines have a problem
if (!playerRef.current && videoRef.current) {
// later, inside this if, inside videojs callback
playerRef.current = player
Here is what I faced during the development
The component rendered
The
useEffect
run, first time, thisif
condition matched, videojs trying to initialize a new instanceNext time the
useEffect
run BUTplayerRef
has NOT been assigned yet because we assign only when we init all event listeners, now videojs trying to initialize a new instance. React is fast by default.What I saw in the HTML like this
Then I tried to assign the ref as soon as possible, but it’s still the same issue
Finally, the working code
const player = (playerRef.current = videojs(videoElement, options, () => {});
We assigned both at the same time, and now everything working as expected.
Clean up in useEffect
Adding the clean up in useEffect
return () => {
const player = playerRef.current;
if (player && !player.isDisposed()) {
player.dispose();
playerRef.current = null;
}
};
Finally, update the unit test to cover all these things
Cleanup on unmounted
Memorize the
options
Subscribe to my newsletter
Read articles from ShinaBR2 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
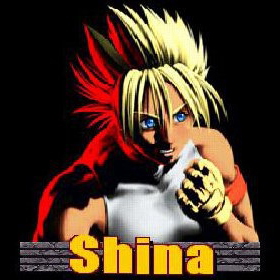
ShinaBR2
ShinaBR2
Enthusiasm for building products with less code but more reliable.