Complete Auth Using MongoDB

Table of contents
- What We’ll Learn:
- Why Authentication is Important?
- Step-by-Step Guide:
- Step 1: Setting Up the Project
- Step 1: Create a MongoDB Atlas Account
- Step 2: Create a New Cluster
- Step 3: Set Up Database Access
- Step 4: Set Up Network Access (IP Whitelisting)
- Step 5: Get Your Connection String
- Step 2: Define the MongoDB Schema
- This code is a pre-save middleware in Mongoose that hashes the password before saving the user document to the database. Let's break down each part:
- Explanation:
- Why It Works:
- Step 3: Email Setup with Nodemailer
- 1. User Registration and Email Verification
- 2. Email Verification
- 3. User Login
- 4. Logout
- 5. Forgot Password
- 6. Reset Password
- Conclusion
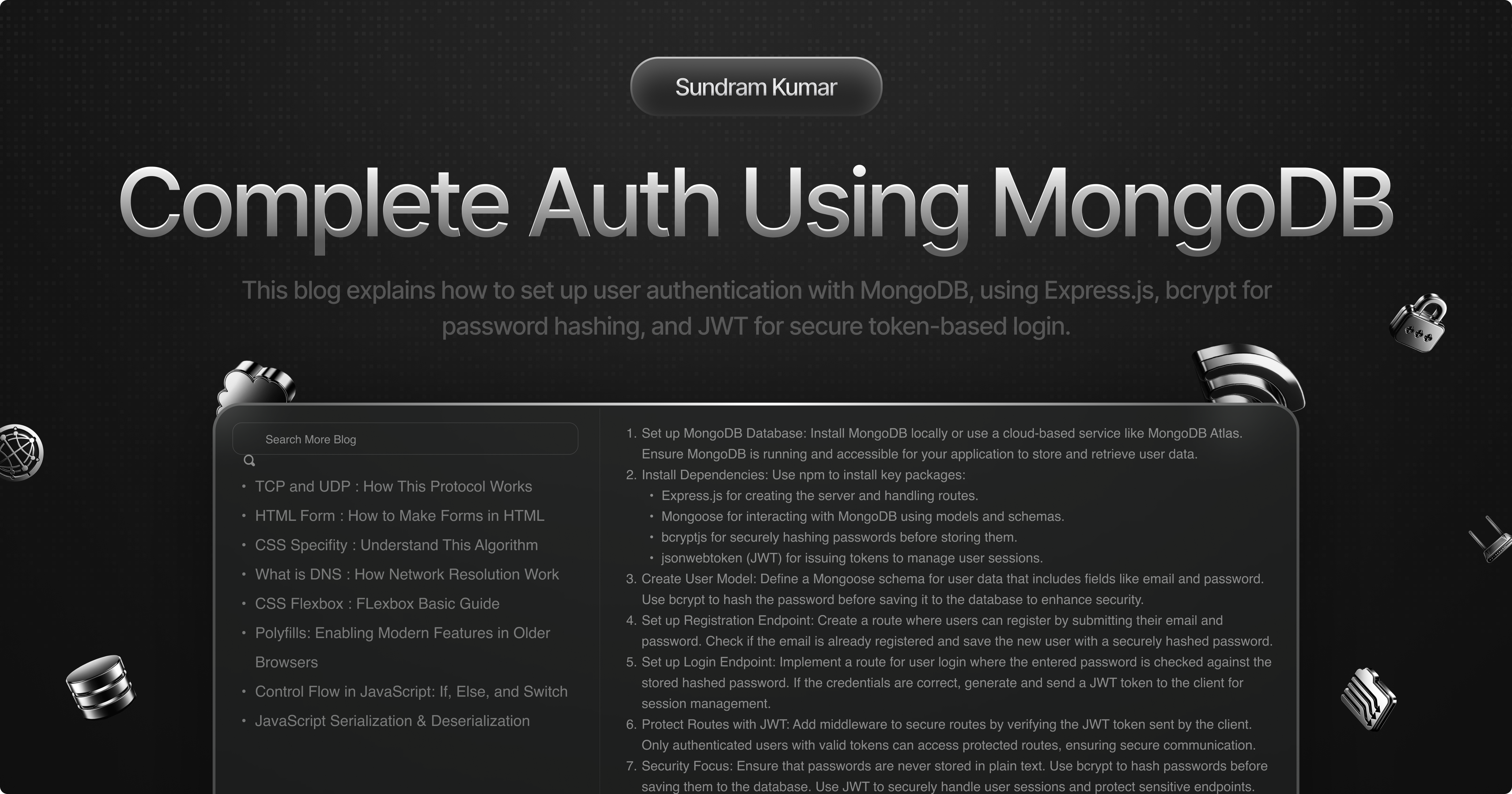
Overview:
Authentication is one of the most essential components of web applications. It helps secure user data, ensures only authorized access to specific resources, and keeps malicious actors at bay. But if you're new to auth or feeling confused about how all the pieces fit together, this blog is for you!
In this blog, we’ll go step by step, covering the core concepts and implementation of user authentication using MongoDB, Node.js, and JWT (JSON Web Tokens). By the end of this blog, you’ll have a clear understanding of the authentication flow, including registration, login, password hashing, email verification, and more.
If you’ve ever wondered:
"How does authentication actually work?"
"How do I securely store passwords?"
"What role do tokens play in authentication?"
"How do I manage user sessions securely?"
"How do I ensure that emails are verified before granting access?"
This blog will answer all your questions in a structured, hands-on manner!
What We’ll Learn:
In this blog, we will cover the following concepts and technologies step by step:
What is Authentication?
A clear understanding of what authentication is and why it's crucial.
The difference between authentication and authorization.
How to Set Up MongoDB with Node.js
Integrating MongoDB Atlas (cloud-hosted MongoDB service) into your project.
How to configure MongoDB connection in a Node.js application.
Hashing Passwords
- How to hash passwords securely using bcryptjs to ensure that no plaintext passwords are stored.
JWT (JSON Web Tokens)
Generating JWT tokens for securing user sessions.
How to send and receive JWT tokens using cookies.
User Registration & Login Flow
A full registration flow (with validation and email verification).
How to handle user login with JWT authentication.
Email Verification
Sending verification emails using Nodemailer and Mailtrap.
How to generate a unique token for email verification.
Password Reset Flow
- Implementing the forget password and reset password flow with email tokenization.
Middleware and Routing
- Protecting routes with middleware to ensure that users are authenticated before accessing certain routes.
Testing & Postman
- How to test all your authentication routes using Postman and ensure your app is secure.
Why Authentication is Important?
In any web application, authentication is the process of verifying who a user is, whereas authorization refers to what they can access once logged in.
Imagine a banking application. Authentication ensures that only the legitimate user can access their bank account, while authorization defines what operations the user is allowed to perform within the account (e.g., viewing balance, transferring money, etc.).
In the context of our blog, we’ll focus on the authentication process, where we’ll create an authentication system that will:
Register new users.
Verify their identity during login.
Issue tokens to keep users logged in securely.
Protect resources with middleware to ensure only authenticated users can access certain pages.
Step-by-Step Guide:
Let's break the flow down into simple steps to understand the full authentication system.
Step 1: Setting Up the Project
Install Dependencies
You’ll need to set up your environment with Node.js, Express, and MongoDB. Key dependencies include:mongoose
(for MongoDB connection)bcryptjs
(for password hashing)jsonwebtoken
(for JWT generation)nodemailer
(for sending verification emails)cookie-parser
(to manage cookies for JWTs)
Run this command in your terminal:
install mongoose bcryptjs jsonwebtoken nodemailer cookie-parser
MongoDB Atlas Setup
- MongoDB Atlas is a cloud-based database service. We’ll use it to host our MongoDB database and connect it securely to our Node.js application. You’ll need to create an account on MongoDB Atlas and get your connection string.
Step 1: Create a MongoDB Atlas Account
Go to MongoDB Atlas: Open your browser and go to https://www.mongodb.com/cloud/atlas.
Sign Up: Click on the “Start Free” button to sign up. You can use your Google account or email to create an account.
Step 2: Create a New Cluster
Once you're logged into MongoDB Atlas:
Create a Cluster:
In the Atlas dashboard, click on "Build a Cluster".
You’ll be prompted to choose a cloud provider and region for your database. The free tier (M0) is available on most cloud providers (AWS, GCP, or Azure) and regions.
Select the Free Tier (M0) option, which gives you 512MB of storage (ideal for development and testing).
Click "Create Cluster".
-
- The creation process can take a few minutes. Once it's complete, you'll see your cluster listed on the dashboard.
Step 3: Set Up Database Access
You’ll need to set up a database user to access your MongoDB cluster.
Create a Database User:
Click "Add New Database User".
You can set specific permissions for the user. For simplicity, you can give them read and write access to any database (recommended for development).
Click "Add User".
Step 4: Set Up Network Access (IP Whitelisting)
You need to allow your app to connect to MongoDB Atlas from specific IP addresses.
-
Go to the Network Access tab in the Atlas dashboard.
Click "Add IP Address".
You can either:
Whitelist your current IP address by clicking on "Allow Access From Anywhere" (this will allow connections from any IP address).
Or add a specific IP address or IP range from which your app will connect.
Click "Confirm" to add your IP address.
Step 5: Get Your Connection String
Now that your cluster is set up, you need to connect your application to it using a connection string.
-
Go to the Clusters tab in the Atlas dashboard.
Click "Connect" next to your cluster.
Choose "Connect Your Application".
Copy the connection string (it will look like this):
mongodb+srv://<username>:<password>@cluster0.mongodb.net/test
Replace
<username>
and<password>
with the credentials you set up earlier.
- Environment Variables
We'll use thedotenv
package to manage sensitive information like database credentials, API keys, and secrets securely in the.env
file.
Step 2: Define the MongoDB Schema
Start by defining a User
schema in MongoDB using Mongoose. This schema will contain user information like name, email, password, and additional fields for email verification and password reset functionality.
mongoose from "mongoose";
import bcrypt from "bcryptjs";
let userSchema = new mongoose.Schema({
name: String,
email: String,
password: String,
role: {
type: String,
enum: ['user', 'admin'],
default: 'user'
},
isVerified: {
type: Boolean,
default: false
},
verificationToken: String,
resetPasswordToken: String,
resetPasswordExpires: Date
}, { timestamps: true });
Here we’ve set up the schema with the necessary fields. We’ll also hash the password before saving it to the database using a pre-save hook.
This code is a pre-save middleware in Mongoose that hashes the password before saving the user document to the database. Let's break down each part:
userSchema.pre("save", async function(next) {
// If the password field is modified (or newly set)
if (this.isModified("password")) {
// Hash the password using bcrypt and set it to the password field
this.password = await bcrypt.hash(this.password, 10);
}
// Proceed with the save operation after the password is hashed
next();
});
Explanation:
userSchema.pre("save", async function(next) { ... })
:pre('save')
: This is a Mongoose middleware that runs before thesave()
operation on the document. In this case, it is executed before saving the user document to the MongoDB database.async function(next)
: The middleware function is asynchronous because password hashing (usingbcrypt
) involves waiting for promises to resolve (i.e., it requires an asynchronous operation).next
is a function that you must call to pass control to the next middleware or to continue with the save operation.
if (this.isModified("password")) { ... }
:this.isModified("password")
: This checks if thepassword
field has been modified in the current document. This check is important because we don't want to hash the password every time the document is updated (for example, when other fields like thename
oremail
are modified).If the password is modified (or it’s a new user), then we proceed to hash the password.
this.password = await bcrypt.hash(this.password, 10)
:bcrypt.hash(this.password, 10)
: This hashes the password using thebcrypt
library.this.password
refers to the current value of the password field in the document.10
: This is the number of salt rounds used bybcrypt
to hash the password. The higher the number of rounds, the more computationally expensive the hash will be, making it harder for attackers to brute-force. A typical value is 10.await
: We useawait
becausebcrypt.hash()
is an asynchronous function, meaning it returns a promise. We need to wait for the password to be hashed before proceeding.
next()
:next()
: This function is called to pass control to the next middleware in the chain or to continue with the save operation once the password is hashed. Without this call, the save operation will be blocked, and the document won't be saved to the database.
Why It Works:
When a user’s password is modified or created, this middleware ensures that the password is hashed before saving it to MongoDB.
If the password hasn’t been changed (i.e., it was not modified), this hook does nothing, and the save operation proceeds as usual without altering the password.
Step 3: Email Setup with Nodemailer
To send verification and password reset emails, we'll use Nodemailer. First, you need to install it:
install nodemailer
Then, set up the email service. You can use services like Mailtrap (for development) or SendGrid (for production). Here's a sample setup with Nodemailer using Mailtrap:
nodemailer from 'nodemailer';
const transporter = nodemailer.createTransport({
service: 'smtp.mailtrap.io',
auth: {
user: process.env.MAILTRAP_USER,
pass: process.env.MAILTRAP_PASS
}
});
const sendVerificationEmail = (user, verificationToken) => {
const mailOptions = {
from: 'your-email@example.com',
to: user.email,
subject: 'Email Verification',
text: `Click the following link to verify your email: http://sundramkumar.com/verify/${verificationToken}`
};
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.log(error);
} else {
console.log('Verification email sent:', info.response);
}
});
};
You'll need to replace the MAILTRAP_USER
and MAILTRAP_PASS
values with your Mailtrap credentials (or the credentials for whatever service you're using). This function sends the verification email when a user registers.
1. User Registration and Email Verification
In this section, we handle the process of registering a new user and verifying their email address.
Why Do We Need This?
Before users can access protected routes, they need to be registered. After registration, we send an email with a verification link, ensuring that the user is who they say they are.
Steps in the Registration Process:
Collect User Input: We first grab the
name
,email
, andpassword
from the user's request body. If any of these are missing, we return a 400 error and prompt the user to provide all fields.let { name, email, password } = req.body; if (!name || !email || !password) { return res.status(400).json({ message: "All fields are required" }); }
Check for Existing User: We then check if there’s already an existing user with the same email. If we find one, we return an error message saying the user already exists.
let existingUser = await User.findOne({ email }); if (existingUser) { return res.status(400).json({ message: "User already exists" }); }
Create a New User: If no user exists, we proceed to create a new user in the database. At this point, we also generate a verification token that will be sent to the user's email.
let user = await User.create({ name, password, email });
Send Verification Email: After successfully creating the user, we generate a token using
crypto
and send a verification email using Nodemailer. This email contains a link that, once clicked, verifies the user's account.let token = crypto.randomBytes(32).toString("hex"); user.verificationToken = token; await user.save(); const transporter = nodemailer.createTransport({ host: process.env.MAILTRAP_HOST, port: process.env.MAILTRAP_PORT, secure: false, auth: { user: process.env.MAILTRAP_USERNAME, pass: process.env.MAILTRAP_PASSWORD, }, }); let mailOptions = { from: '"Magic Elves" <from@example.com>', to: user.email, subject: "Verify Your Email", text: `Please click on the following link to verify your account: ${process.env.BASE_URL}/api/v1/users/verify/${token}` }; await transporter.sendMail(mailOptions);
What Happens Next?
Once the user clicks the verification link in their email, they are redirected to a page where their email gets verified, and they are granted access to the application.
2. Email Verification
Once the user registers, they need to confirm their email. This step is essential to ensure the user’s email is valid and that they are not a bot or malicious user.
Why Do We Need This?
This prevents unauthorized users from accessing the system and ensures that the user is indeed the owner of the email they registered with.
Steps in the Email Verification Process:
Retrieve the Token: The token sent in the email is included in the URL, and we extract it from the request parameters.
let { token } = req.params;
Check for Token Validity: We look for a user in the database whose verification token matches the one in the URL. If no user is found or if the token is invalid, we return an error.
let user = await User.findOne({ verificationToken: token }); if (!user) { return res.status(400).json({ message: "Invalid token" }); }
Mark User as Verified: Once the user is found, we update the
isverified
status totrue
, indicating that the email has been confirmed. We then remove the token to prevent reuse.user.isverified = true; user.verificationToken = undefined; await user.save();
Send Success Response: Finally, we send a success message to the user, confirming their email verification.
res.status(200).json({ message: "User verified successfully", success: true });
What Happens Next?
Once the user’s email is verified, they are able to log in and access protected routes.
3. User Login
After the user registers and verifies their email, they can log in to the system.
Why Do We Need This?
Logging in allows users to authenticate themselves and access secure areas of the application.
Steps in the Login Process:
Receive User Credentials: The user provides their email and password. If any of these are missing, we return a 400 error.
const { email, password } = req.body; if (!email || !password) { return res.status(400).json({ message: "All fields are required" }); }
Check User Existence: We check if the email exists in the database. If the email is invalid, we return an error.
let user = await User.findOne({ email }); if (!user) { return res.status(400).json({ message: "Invalid email or password" }); }
Verify Password: Using bcrypt, we compare the password provided by the user with the one stored in the database. If the passwords don't match, we return an error.
let isMatch = await bcrypt.compare(password, user.password); if (!isMatch) { return res.status(400).json({ message: "Invalid email or password" }); }
Check Email Verification: If the user hasn't verified their email, we return an error instructing them to verify their email first.
if (!user.isverified) { return res.status(400).json({ message: "Please verify your email." }); }
Generate JWT Token: If everything checks out, we generate a JWT token that the user can use for subsequent requests. This token will expire in 24 hours.
let token = jwt.sign( { id: user._id }, process.env.JWT_TOKEN, { expiresIn: "24h" } );
Send Token in Cookie: We send the JWT token as a cookie to the user's browser. This allows the user to remain logged in across requests.
const cookieOptions = { httpOnly: true, secure: true, maxAge: 24 * 60 * 60 * 1000 }; res.cookie("token", token, cookieOptions);
Send Success Response: Finally, we return the user’s details and the JWT token, confirming a successful login.
res.status(200).json({ success: true, message: "Login successful", token, user: { id: user._id, name: user.name, role: user.role }, });
What Happens Next?
Now that the user is logged in, they can access routes protected by authentication middleware, which verifies the JWT token before granting access.
4. Logout
When the user wants to log out, we simply clear their JWT token stored in the cookie.
Steps in the Logout Process:
Clear the Cookie: We remove the
token
cookie from the user's browser, effectively logging them out.res.cookie('token', '', { httpOnly: true, expires: new Date(0) // Expire immediately });
Send Success Response: Finally, we send a response confirming that the logout was successful.
res.status(200).json({ success: true, message: "Logged out successfully" });
What Happens Next?
The user is logged out and needs to log in again to access protected routes.
5. Forgot Password
The Forgot Password route allows a user to request a password reset if they forget their password.
Why Do We Need This?
This feature is critical for account recovery. If a user forgets their password, they can request a reset email with a link to reset their password and regain access to their account.
Steps in the Forgot Password Process:
Receive User's Email: The user provides their email address. If this email is not associated with any registered account, we return an error.
const { email } = req.body; let user = await User.findOne({ email }); if (!user) { return res.status(404).json({ success: false, message: "User not found" }); }
Generate Reset Token: We generate a unique token using
crypto.randomBytes()
and store this token in the user's record in the database. The token will be used to identify the reset request.Additionally, we set an expiration time for the token (in this case, 10 minutes), so the user has a limited time to reset their password.
const token = crypto.randomBytes(32).toString("hex"); user.resetPasswordToken = token; user.resetPasswordExpires = Date.now() + 10 * 60 * 1000; // Token expires in 10 mins
Save the Token and Expiration: We save the generated token and the expiration time in the database to ensure they can be validated later.
await user.save();
Set Up Nodemailer for Sending Email: Next, we set up Nodemailer to send an email to the user with a reset link. The link contains the reset token, and the user can click on it to go to the password reset page.
const transporter = nodemailer.createTransport({ host: process.env.MAILTRAP_HOST, port: process.env.MAILTRAP_PORT, secure: false, auth: { user: process.env.MAILTRAP_USERNAME, pass: process.env.MAILTRAP_PASSWORD, }, }); let mailOptions = { from: '"Magic Elves" <from@example.com>', to: user.email, subject: "Password Reset Request", text: `Hello, You requested a password reset. Please click the link below to reset your password: ${process.env.BASE_URL}/api/v1/users/reset/${token}` }; await transporter.sendMail(mailOptions);
Send Success Response: After sending the email, we return a success response to the user confirming that the password reset request has been sent.
res.status(200).json({ success: true, message: "Password reset email sent" });
What Happens Next?
The user receives an email with a reset link. This link includes the token, and they can use it to access the reset password page.
6. Reset Password
The Reset Password route allows a user to set a new password by verifying the token they received in the email. This is the step where the actual password change happens.
Why Do We Need This?
This route ensures that the user can update their password after receiving the reset link and provides a secure way to validate the token before updating the password.
Steps in the Reset Password Process:
Retrieve the Reset Token: We first retrieve the token from the request parameters (
req.params.token
) and the new password from the request body (req.body.newPassword
).const { token } = req.params; const { newPassword } = req.body;
Verify the Token and Expiry Time: We search the database for a user who has the matching reset token and whose reset token has not expired. The token expiration time is checked to ensure that it hasn’t been more than 10 minutes since the reset request was made.
let user = await User.findOne({ resetPasswordToken: token, resetPasswordExpires: { $gt: Date.now() } // Token still valid });
- If the token is invalid or expired, we return an error message stating that the token is invalid or expired.
if (!user) {
return res.status(400).json({ success: false, message: "Invalid or expired token" });
}
Update the User's Password: If the token is valid, we update the user’s password to the new one provided. For security reasons, it's best to hash the password (usually with bcrypt) before saving it. Here, we assume
newPassword
is already hashed or prepared for storage.user.password = await newPassword; // Ideally, hash the password first with bcrypt
Clear the Reset Token and Expiry: After successfully updating the password, we clear the reset token and reset token expiration fields from the user's record in the database. This prevents the token from being used again.
user.resetPasswordToken = undefined; user.resetPasswordExpires = undefined;
Save the Updated User: We save the updated user record with the new password.
await user.save();
Send Success Response: Finally, we return a success response to the user, confirming that their password has been reset successfully.
res.status(200).json({ success: true, message: "Password reset successful" });
What Happens Next?
Once the user’s password has been updated, they can log in using their new password.
Conclusion
This guide provides a complete flow for implementing user authentication using MongoDB, Node.js, and JWT. You’ve learned how to:
Set up MongoDB and create a user schema.
Implement password hashing and JWT authentication.
Handle email verification and password reset functionality.
Protect routes with JWT-based authentication middleware.
By following this guide, you’ll have a solid foundation for building secure user authentication in your Node.js apps!
Subscribe to my newsletter
Read articles from Sundram Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
