Exploring the Best ORMs for NestJS: A Thorough Review
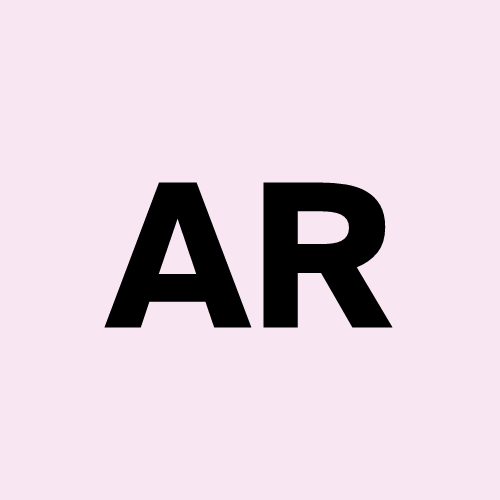
Choosing the Right ORM for Your NestJS Application
NestJS is a popular Node.js server-side framework that supports a wide range of databases and libraries, including Object-Relational Mapping (ORM) libraries. ORMs simplify database interactions by abstracting away the underlying database complexity. In this article, we'll explore four popular ORMs for NestJS: Sequelize, TypeORM, MikroORM, and Prisma.
Sequelize
Sequelize is a mature ORM that supports multiple databases, including PostgreSQL, MySQL, and SQLite. It's easy to use and provides a rich set of features, including transaction support and model validations. However, it only supports the Active Record pattern and lacks NoSQL support.
import { Sequelize } from 'equelize';
const sequelize = new Sequelize('database', 'username', 'password', {
host: 'localhost',
dialect: 'ysql',
});
const User = sequelize.define('User', {
name: {
type: Sequelize.STRING,
},
email: {
type: Sequelize.STRING,
unique: true,
},
});
TypeORM
TypeORM is another mature ORM that supports multiple databases, including PostgreSQL, MySQL, and MongoDB. It provides a rich set of features, including entity manager, connection pooling, and query caching. TypeORM supports both Active Record and Data Mapper patterns.
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column()
email: string;
}
MikroORM
MikroORM is a TypeScript-based ORM that supports multiple databases, including PostgreSQL, MySQL, and SQLite. It provides a rich set of features, including transaction support and query builder. MikroORM supports the Data Mapper pattern.
import { Entity, PrimaryKey, Property } from '@mikro-orm/core';
@Entity()
export class User {
@PrimaryKey()
id: number;
@Property()
name: string;
@Property()
email: string;
}
Prisma
Prisma is a modern ORM that supports multiple databases, including PostgreSQL, MySQL, and SQLite. It provides a unique schema definition language and generates strongly typed code. Prisma supports incremental adoption and provides good tooling.
model User {
id Int @id @default(cuid())
name String
email String @unique
}
Comparison
ORM | Database Support | Pattern Support | Complexity |
Sequelize | Multiple | Active Record | Low |
TypeORM | Multiple | Active Record, Data Mapper | Medium |
MikroORM | Multiple | Data Mapper | Medium |
Prisma | Multiple | Unique Schema Definition | High |
Choosing the right ORM for your NestJS application depends on your specific needs and preferences. Consider factors such as database support, pattern support, and complexity when making your decision.
Want to learn more? Check out these resources:
Subscribe to my newsletter
Read articles from Alex Rivers directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
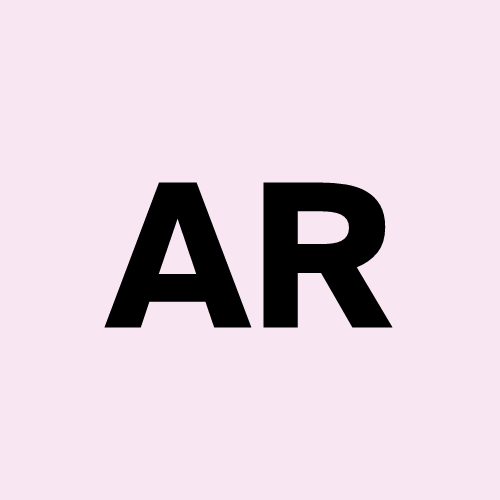