JavaScript this Keyword
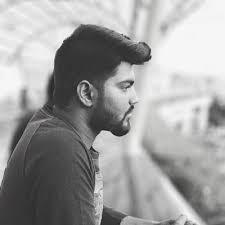
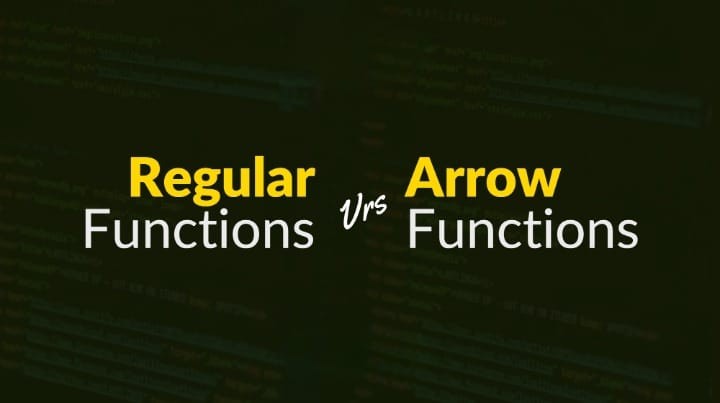
Ever encountered a situation where your this
keyword in JavaScript doesn’t behave as expected? If you’ve used arrow functions, you might have faced this issue. Unlike traditional functions, arrow functions handle this
differently, and it can lead to unexpected bugs in your backend code. In this blog, we’ll explore why this happens, how to fix it, and when to use each type of function. Let’s dive in!
Table of Contents
1. What is the this
Keyword in JavaScript?
In JavaScript, this
refers to the context in which a function is executed. It can change depending on how the function is called. For example:
In a method,
this
refers to the object that owns the method.In a global context,
this
refers to the global object (e.g.,window
in browsers).
2. How Arrow Functions Handle this
Arrow functions do not have their own this
binding. Instead, they inherit this
from the parent scope (lexical scoping). This can be useful in some cases but problematic in others.
Example:
const obj = {
name: "Dev",
greet: () => {
console.log(`Hello, ${this.name}`); // `this` refers to the global object, not `obj`
},
};
obj.greet(); // Output: Hello, undefined
Why does this happen?
Arrow functions don’t bind their own this
, so this.name
looks for name
in the global scope, which doesn’t exist.
3. How Traditional Functions Handle this
Traditional functions have their own this
binding, which depends on how the function is called. You can also explicitly bind this
using .bind()
, .call()
, or .apply()
.
Example:
const obj = {
name: "Dev",
greet: function () {
console.log(`Hello, ${this.name}`); // `this` refers to `obj`
},
};
obj.greet(); // Output: Hello, Dev
Why does this work?
Traditional functions bind this
to the object calling the method, so this.name
correctly refers to obj.name
.
4. Code Demonstration: Arrow vs Traditional Functions
Let’s see a practical example of how this
behaves differently in arrow and traditional functions.
Code:
class User {
constructor(name) {
this.name = name;
}
// Traditional Function
greetTraditional() {
console.log(`Traditional: Hello, ${this.name}`);
}
// Arrow Function
greetArrow = () => {
console.log(`Arrow: Hello, ${this.name}`);
};
}
const user = new User("Alice");
// Works as expected
user.greetTraditional(); // Output: Traditional: Hello, Alice
// Also works, but for a different reason (lexical `this`)
user.greetArrow(); // Output: Arrow: Hello, Alice
// Now let's break it
const traditionalGreet = user.greetTraditional;
const arrowGreet = user.greetArrow;
traditionalGreet(); // Output: Traditional: Hello, undefined (lost `this` binding)
arrowGreet(); // Output: Arrow: Hello, Alice (still works due to lexical `this`)
Explanation:
Traditional functions lose
this
when called without the object context.Arrow functions retain
this
because they inherit it from the parent scope.
5. Common Mistakes to Avoid
Using arrow functions for object methods where
this
is needed.Assuming arrow functions and traditional functions behave the same way.
Forgetting to bind
this
in traditional functions when passing them as callbacks.
6. When to Use Arrow Functions vs Traditional Functions
Use Arrow Functions When:
You need lexical
this
(e.g., in callbacks or closures).You want a shorter syntax for simple functions.
Use Traditional Functions When:
You need dynamic
this
binding (e.g., object methods).You want to use
.bind()
,.call()
, or.apply()
.
7. Conclusion
Understanding how this
works in JavaScript is crucial for writing bug-free code. Arrow functions are great for certain use cases, but they can break your code if you rely on this
binding. Traditional functions, on the other hand, give you more control over this
.
Pro Tip: Always think about the context in which your function will run before choosing between arrow and traditional functions.
Call-to-Action
What’s your experience with this
in JavaScript? Have you ever been bitten by this issue? Share your thoughts in the comments below! If you found this guide helpful, don’t forget to share it with your fellow developers. 🚀
Additional Resources
Your Feedback Matters! 💬
Thank you for taking the time to read this blog! I hope you found it insightful and helpful in understanding the nuances of the this
keyword in JavaScript. If you have any questions, thoughts, or additional tips to share, I’d love to hear from you in the comments below. Your feedback not only helps me improve but also enriches the discussion for fellow developers.
If you enjoyed this post, please consider:
Liking this article ❤️ to let me know it was valuable to you.
Sharing it with your network on Twitter, LinkedIn, or any platform where developers hang out. Spreading knowledge is what makes our community stronger! 🌟
Following my blog for more tutorials, tips, and deep dives into programming concepts. Your support motivates me to keep creating content that matters to you.
Let’s keep the conversation going—drop a comment, share your experiences, or suggest topics you’d like me to cover next. Together, we can learn and grow as developers! 🚀
Happy coding! 😊
Abhinav Rastogi
Subscribe to my newsletter
Read articles from Abhinav Deep Rastogi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
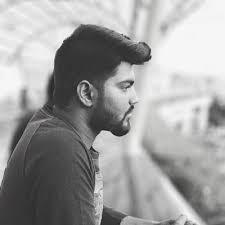